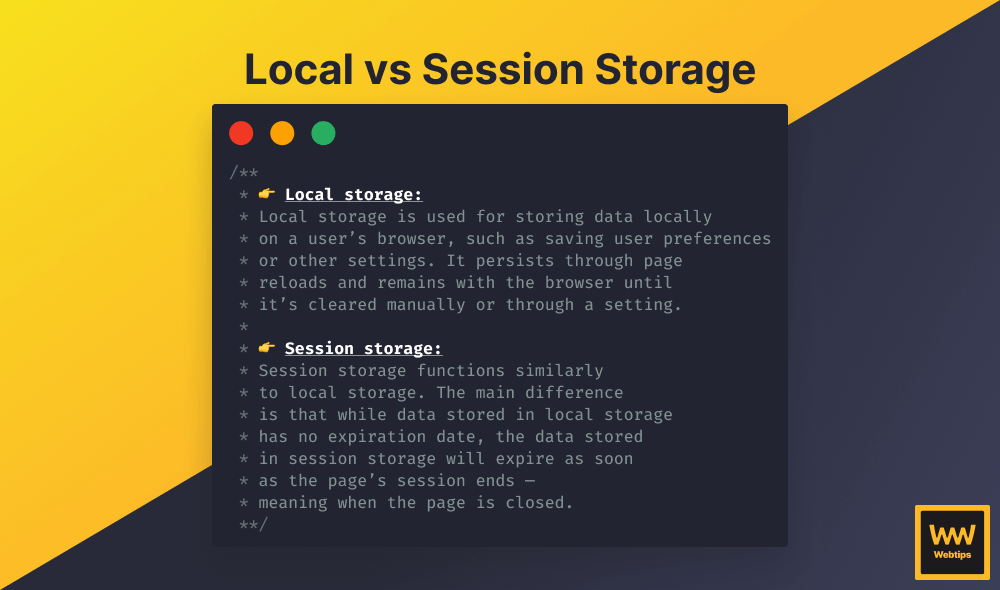
What is The Difference Between Local and Session Storage
When it comes to storing data in the browser, we have multiple APIs we can work with. Two similar-sounding but different APIs are the local storage and session storage APIs. In this quick guide, we'll take a look at the commonalities and the differences between the two. But first, what is Local and Session Storage in one sentence?
- Local storage: Persistent storage in a user's browser, retained through page reloads and across browser sessions until manually cleared.
- Session storage: Temporary storage that holds data for the duration of a single browsing session, automatically clearing it when the session ends, typically by closing the page or tab.
The Differences
It's important to know the difference between Local and Session storage as while they both extend the Storage interface, they behave differently and can be used for different purposes. The main differences can be categorized into three areas:
- Scope of data: The most important difference between the two is the way data is stored. Local Storage persists data indefinitely until explicitly removed by the web application or the user. It's accessible across browser sessions and tabs. On the other hand, data stored in Session Storage lasts only for the duration of the page session, meaning it's lost when the browser tab or window is closed.
- Deleting mechanism: As data in Local Storage is persistent, deleting the data needs to be manually requested by the user. Session Storage handles the deletion of data automatically upon closing the window.
- Sharing data between tabs: By the nature of Local Storage, data can be accessed across multiple tabs or windows of the same browser as it's persistent storage, unlike data stored in Session Storage, which is specific to each tab or window. Changes made in one tab won't be reflected in another.
The Similarities
Even though they can be used for different purposes, which we'll look into in a minute, they also have some commonalities:
- Both are client-side storage: Both Local Storage and Session Storage store data on the client side, meaning the data is located and available in the browser, regardless of server status.
- Storage limit: Although the specific limits can slightly vary between different browsers, they typically have the same storage limit ranging from 5 to 10MB.
- Similar APIs: As they both extend the Storage API, they offer simple APIs to store and retrieve data using key-value pairs, making it easier for new developers to learn both at once.
- Limited security: Lastly, because both types of data are stored in the browser, it's important to mention that you should not store sensitive information in Local or Session storage, such as passwords, credit card details, or personal information.

Use Cases
Now that we know the differences and similarities between the two, let's take a quick look at a couple of use cases for each API.
Local Storage
When dealing with user-specific data, such as user preferences (e.g., theme selection, language preference), or other user data that doesn't need to be stored in a database, we can use Local Storage. The following example demonstrates how we can store a user preference in local storage:
localStorage.setItem('theme', 'dark')
// Later retrieve the theme
const theme = localStorage.getItem('theme')
The localStorage
object is globally available. We can use the setItem and getItem methods to set and retrieve items. We can also handle user authentication, such as storing token information. This allows us to keep users logged in even after closing the browser.
Another way Local Storage can be utilized is by using it for caching purposes. Store frequently accessed data to reduce server load and improve page performance. Although Local Storage only allows us to store strings, we can get around this limitation by converting the data into a JSON object in the following way:
- Line 1: First, we need to convert the JavaScript object into a string that needs to be stored for caching in Local Storage. This can be done by passing the object to
JSON.stringify
. - Line 3: Using the stringified data, we can now set any Local Storage value to arbitrary data.
- Line 5: To retrieve the data, we need to reverse the process. First, we can store the Local Storage value inside a variable. This variable will only hold a JSON string.
- Line 6: To work with the actual data that we stored in Local Storage, we need to parse it back into a JavaScript object using
JSON.parse
.
Using the above approach, we can store any arbitrary data in Local Storage.
Session Storage
Session Storage, on the other hand, should be used for one-time data; data that is only relevant for a single browsing session. A common use case for using Session Storage is to save form data for a single session to prevent loss during page reloads.
We can follow the same approach we did with Local Storage as the API is the same. The only difference is that this time, we need to use the global sessionStorage
object instead of localStorage
:
// Storing data in Session Storage
sessionStorage.setItem('form', 'user=John&age=30')
// Retrieving data from Session Storage
const formData = sessionStorage.getItem('form')
In case we want to store multiple values in a single key, we can follow the same approach we used for Local Storage; convert the object into JSON format, then parse it back to a JavaScript object using JSON.parse
. Another approach presented here is using query strings, where values are concatenated into a single string using ampersand. To convert a query string back to a JavaScript object, we can use the URLSearchParams
object in the following way:
// Returns {user: 'John', age: '30'}
const query = 'user=John&age=30'
const data = Object.fromEntries(new URLSearchParams(query))
Summary
In summary, while both Local and Session storage share a similar API, they are inherently different. Use Local Storage when you need to handle user preferences, authentication, or caching, and prefer Session Storage when dealing with one-time data such as filled form inputs. In both cases, avoid storing any sensitive information.
Is there anything else you'd like to know about client-based storage APIs in JavaScript? Let us know in the comments below! Thank you for reading, and happy coding! If you'd like to learn more about JavaScript, continue with our guided roadmap:
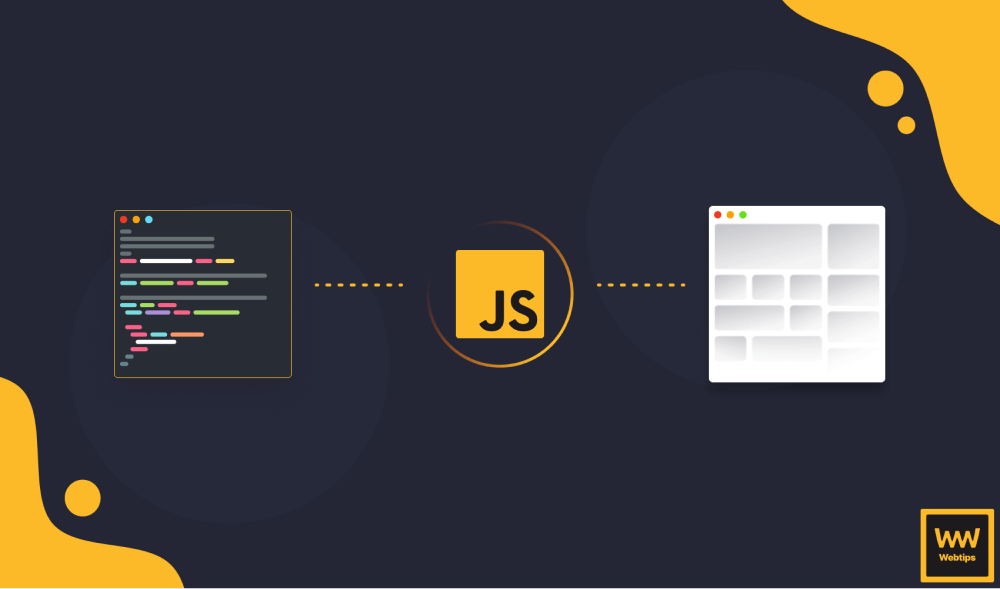
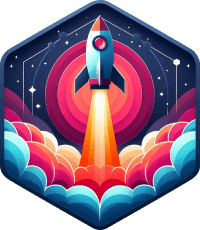
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: