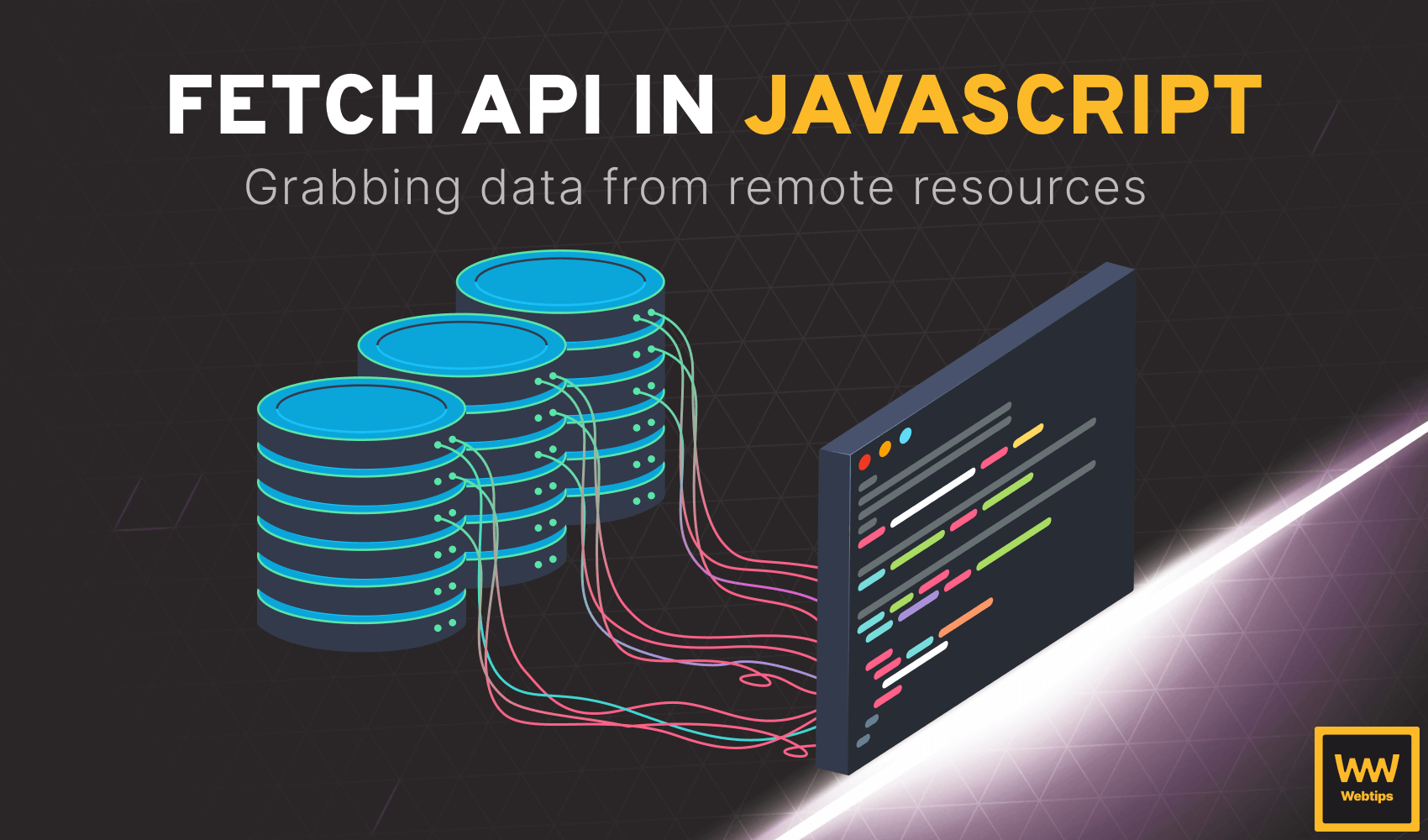
JavaScript
JavaScript is one of the world's most popular programming languages that powers every website on the web, including this page you are looking at right now. It allows you to create interactive and dynamic web applications without the need for extra installation steps. This means that you only need a browser to start using JavaScript.
It can be used for a variety of tasks, whether it is simple things such as manipulating form elements or building complex 3D experiences, JavaScript can do it right in your browser.
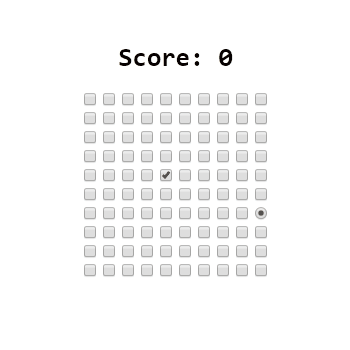

Why Should I Learn JavaScript?#
Still, the question remains: why should I care about learning JavaScript? What's in it for me? Here are five reasons to help you decide whether you should invest your time in learning JavaScript:
- Job opportunities: JavaScript is one of the most in-demand programming languages, with many well-paying job opportunities available, including remote work. This means that you have the potential to work from anywhere without being tied to a specific location.
- Easy to learn: JavaScript is considered easy to learn due to its simpler syntax compared to other languages. This makes it a perfect choice for getting started in the world of programming.
- Versatile: As demonstrated in the two examples above, JavaScript is versatile. It is also cross-platform, meaning you can use it to build desktop or mobile applications, or even games.
- Improved skills: Programming languages require you to think logically and methodically, which improves your problem-solving skills over the long run and boosts your critical thinking.
- A core part of the web: If you are looking to get into web development, there is no way around JavaScript. It is a core part of the web, and even the simplest websites use it to some degree.
Did you know? β JavaScript was originally called Mocha, then LiveScript.
Show Me How JavaScript Works#
Let's take a look at a simple example to see how we can use JavaScript. Suppose we have a dashboard where users can change their email, and we want to reflect the change in real-time. This can be achieved using the following code:
const textInput = document.getElementById('text-input')
const inputValue = document.getElementById('input-value')
textInput.addEventListener('keyup', event => {
inputValue.innerText = event.target.value
})
Change the value inside the input to see the change reflected by JavaScript. Let's break down each line to understand what is going on:
- Line 1-2: We create two variables: one for selecting the input field, and one for selecting the text underneath. The
const
keyword specifies that these values are constants and should not change over time. - Line 4: We add an event listener to the input field using the
addEventListener
function on the variable. By specifying it as "keyup", this listener will be called every time a key is pressed inside the text input. The second parameter of theaddEventListener
is a function that will be called on each keystroke. - Line 5: We assign the value of the input (the value is stored inside
event.target.value
) to the text beneath the input.
Where Should I Start?#
If you are new to JavaScript, you should start with the basics. First, you need to familiarize yourself with the available data types in JavaScript. Next, you should start working with variables and then move on to functions. Once you are familiar with the basics, you can dive deeper into how arrays and objects work. Make sure you understand how the this
keyword works in JavaScript.
JavaScript is asynchronous in nature, and many times you will need to communicate with servers. This can be achieved through the use of promises. At this point, you should start learning how to work with web APIs such as the DOM. We also have a roadmap that guides you through everything you need to know about JavaScript.
Here are some interview questions that can help you get a better understanding of JavaScript, as well as a collection of smaller JavaScript tips and tricks. Additionally, you can filter for JavaScript-related tutorials on our search page.
Last but not least, you can find all of our latest JavaScript tutorials below. Use the filters on the sidebar if you would like to narrow down the results. I wish you good luck with learning JavaScript!
Keep on coding! π¨βπ»
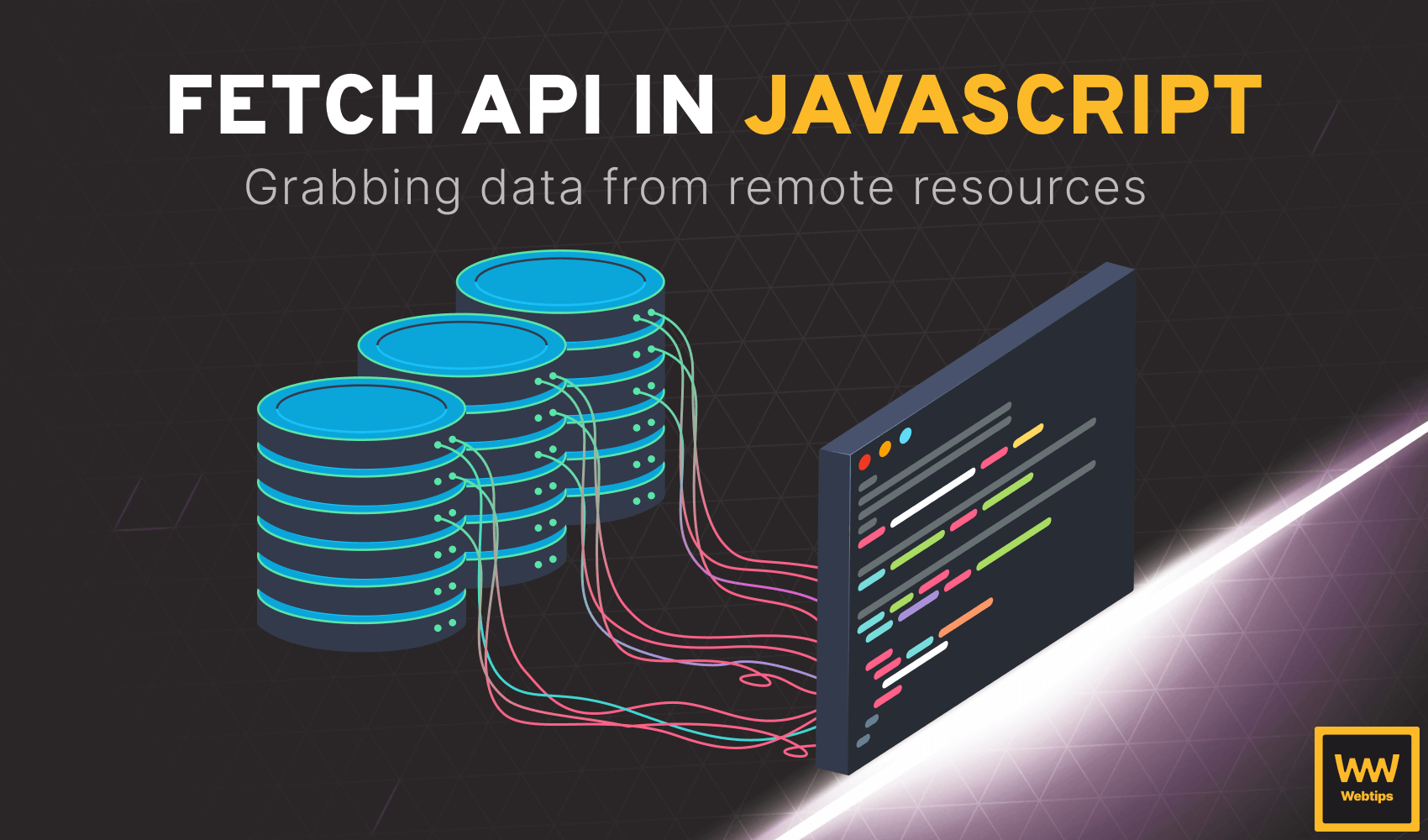
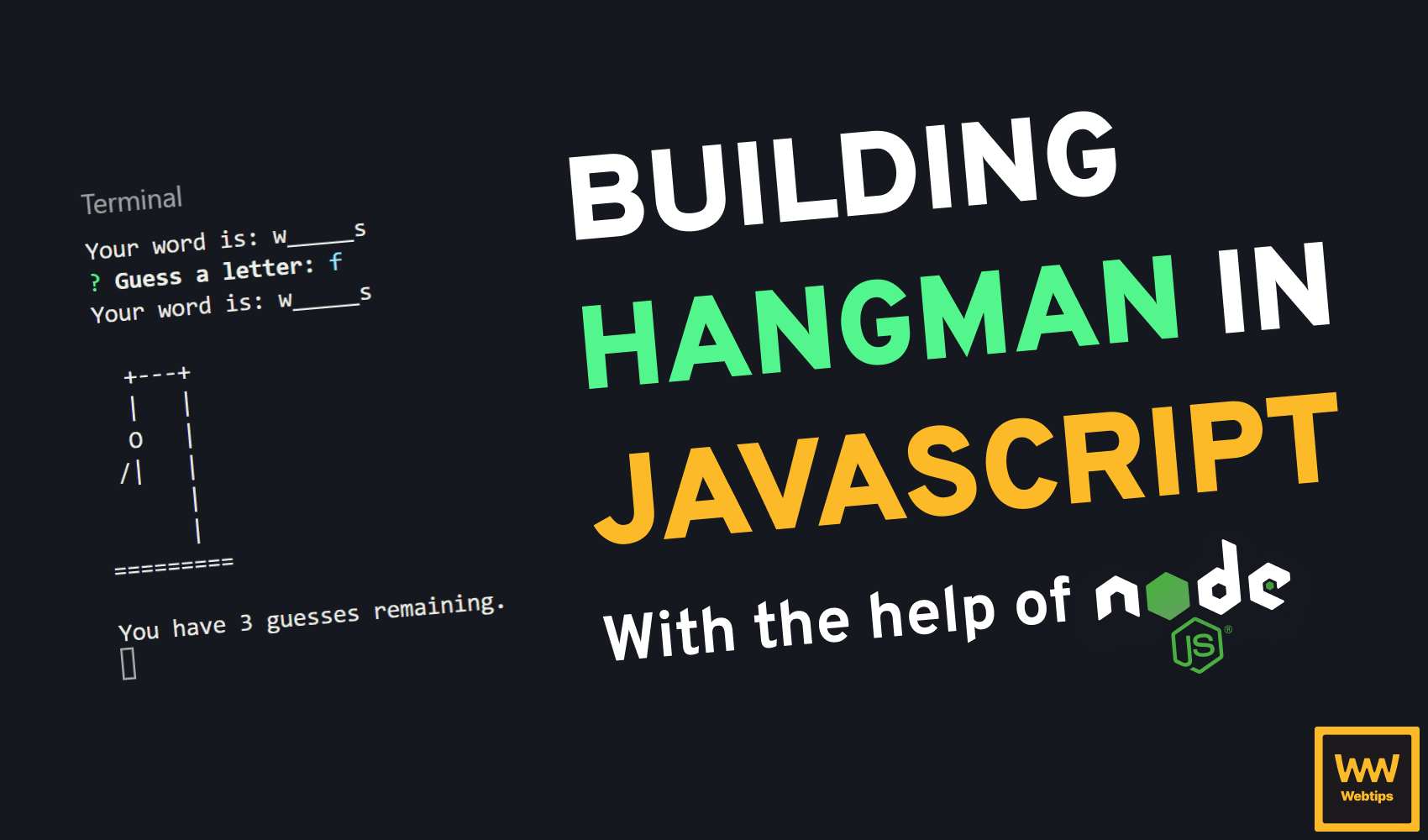
How to Build Hangman in JavaScript From Scratch
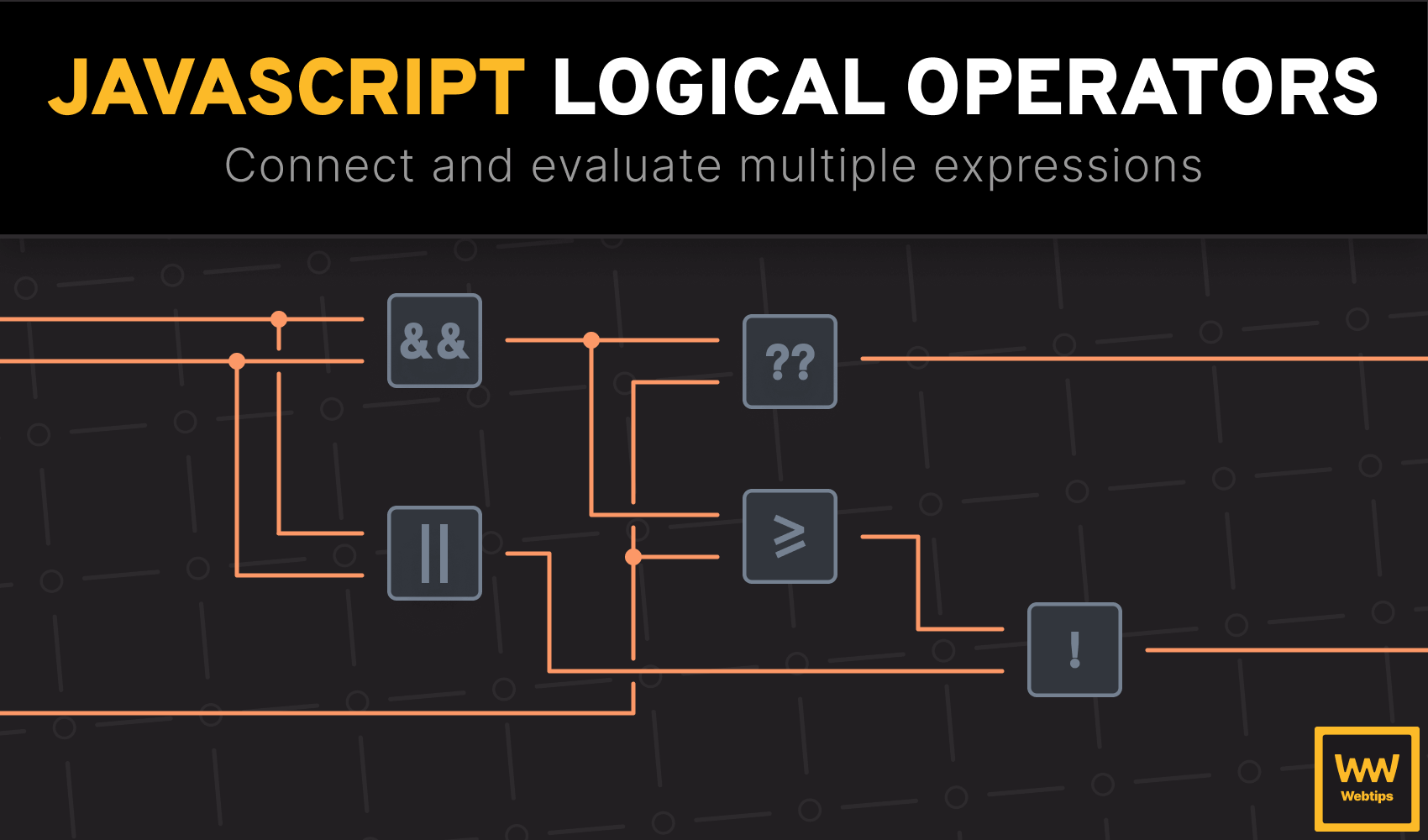
Logical Operators Explained in JavaScript
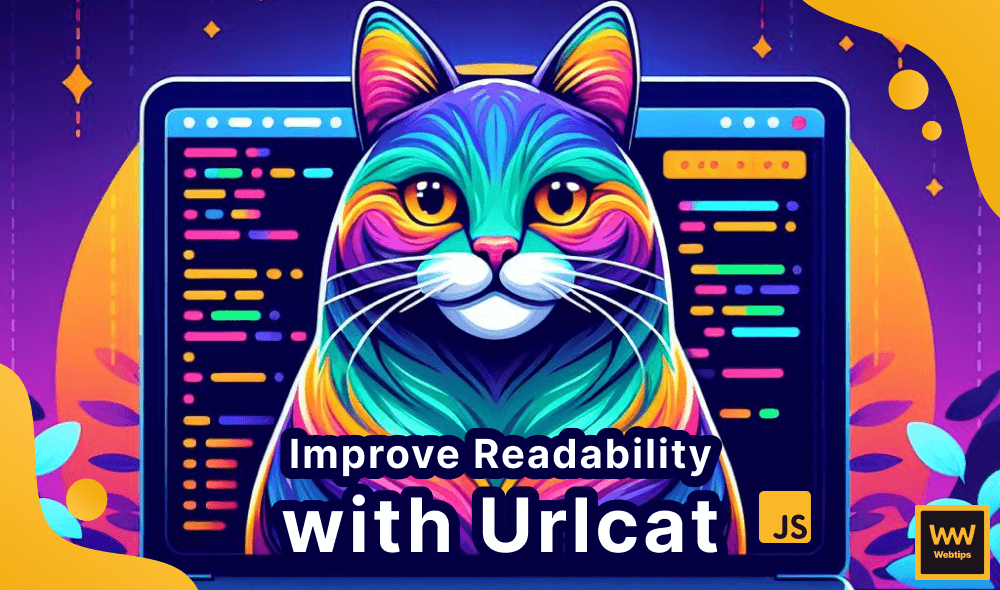
An Introduction to Urlcat
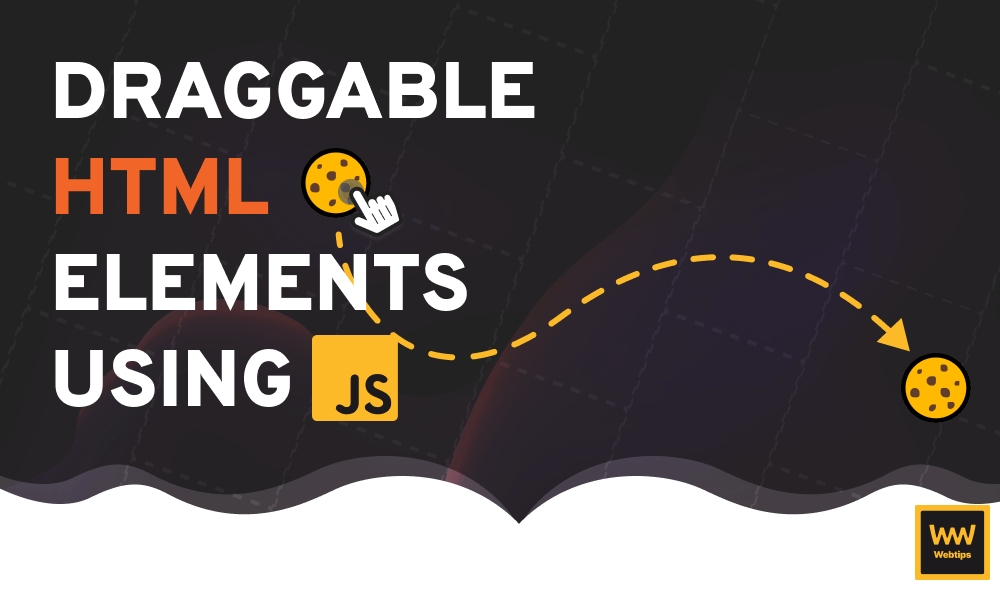
How to Create Draggable HTML Elements With JavaScript
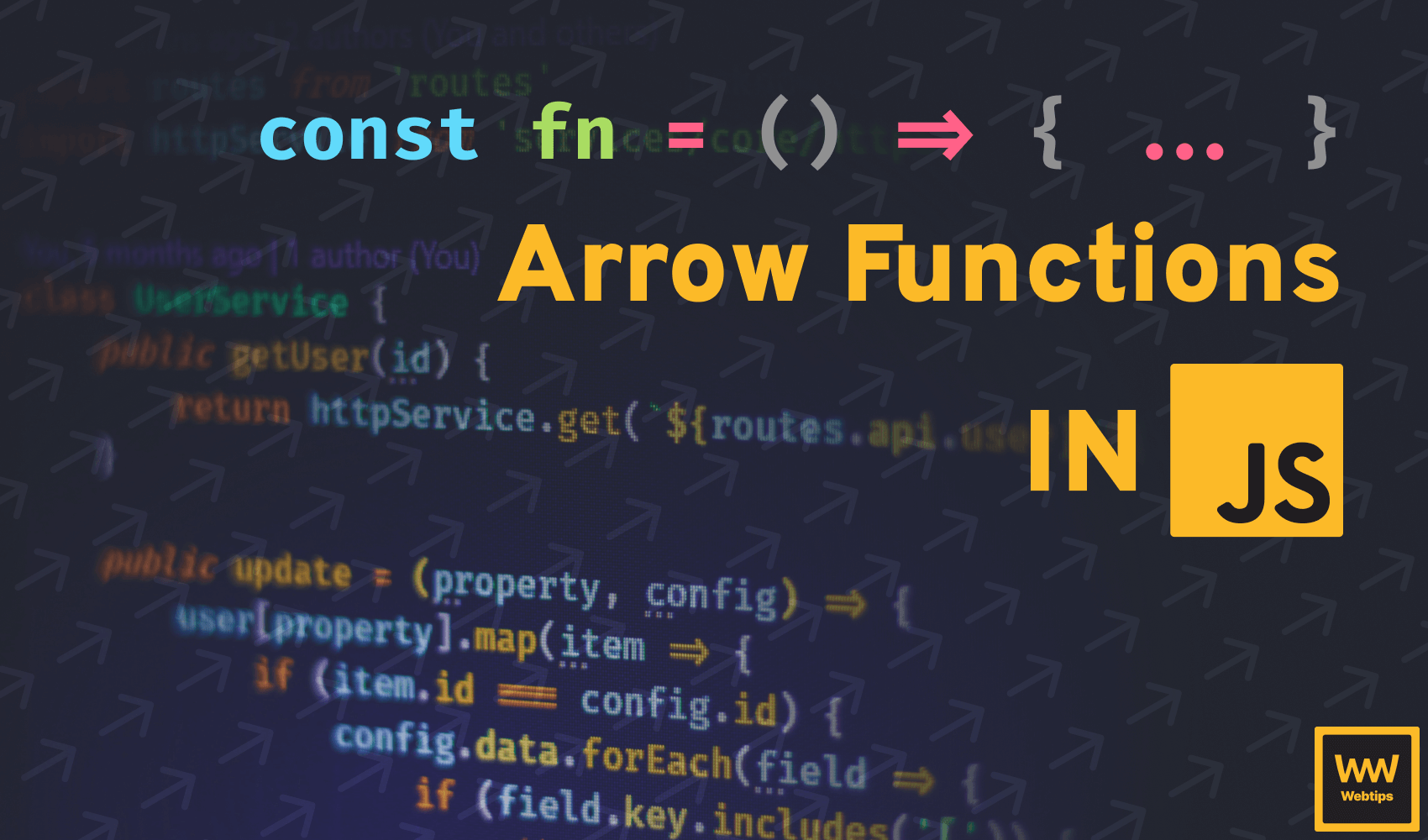
What are Arrow Functions in JavaScript?
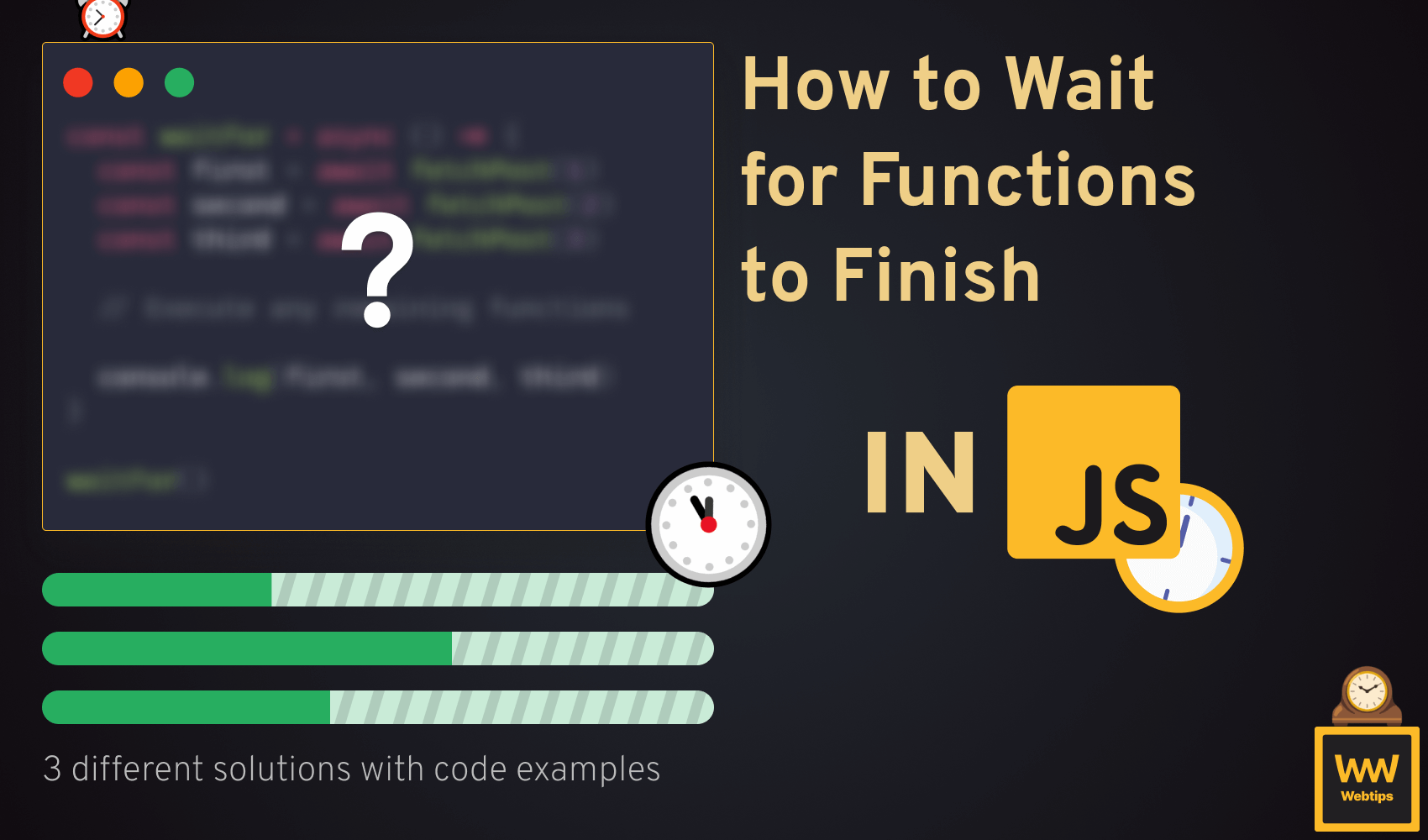
How to Wait for a Function to Finish in JavaScript
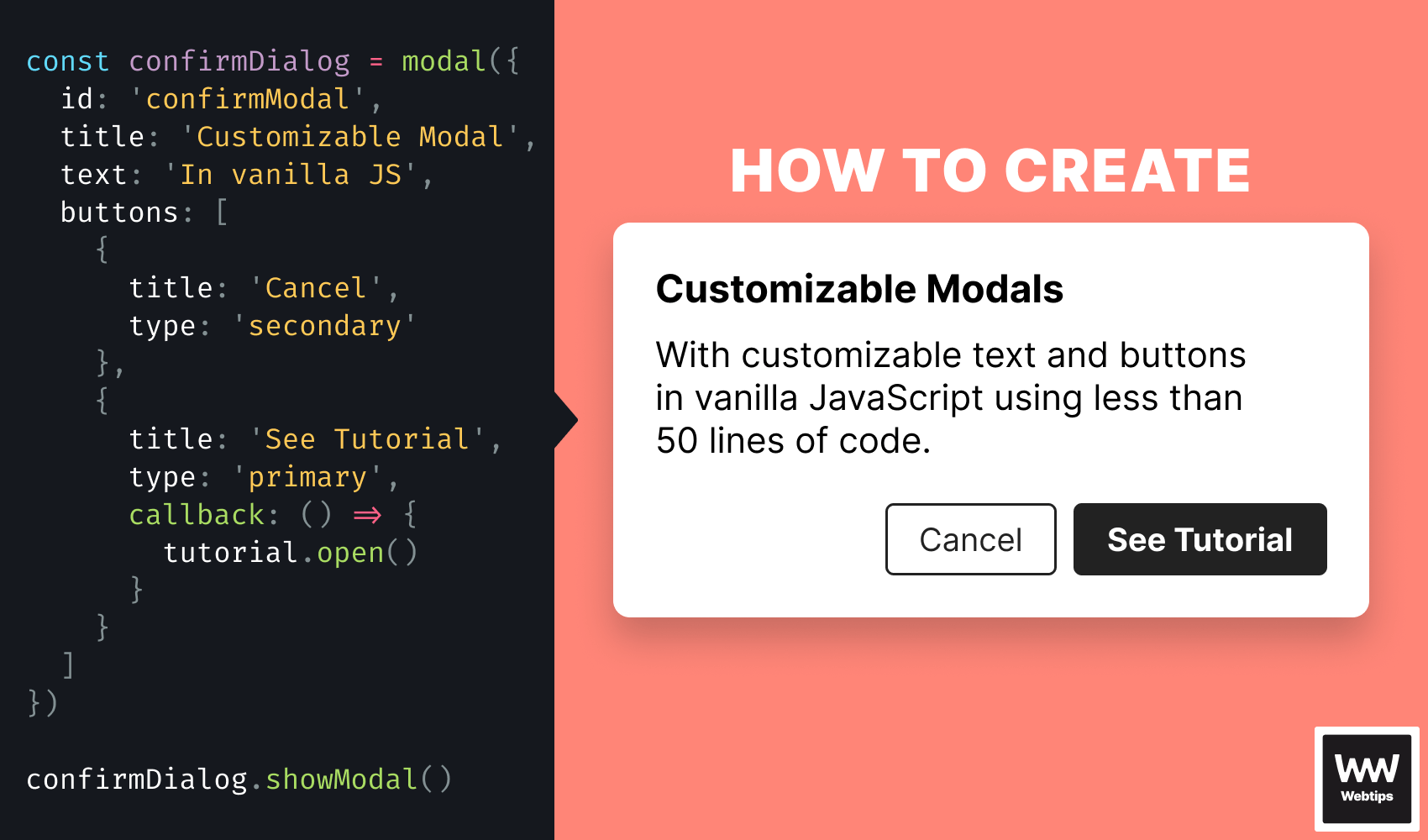
How To Create a Customizable Modal in Vanilla JavaScript
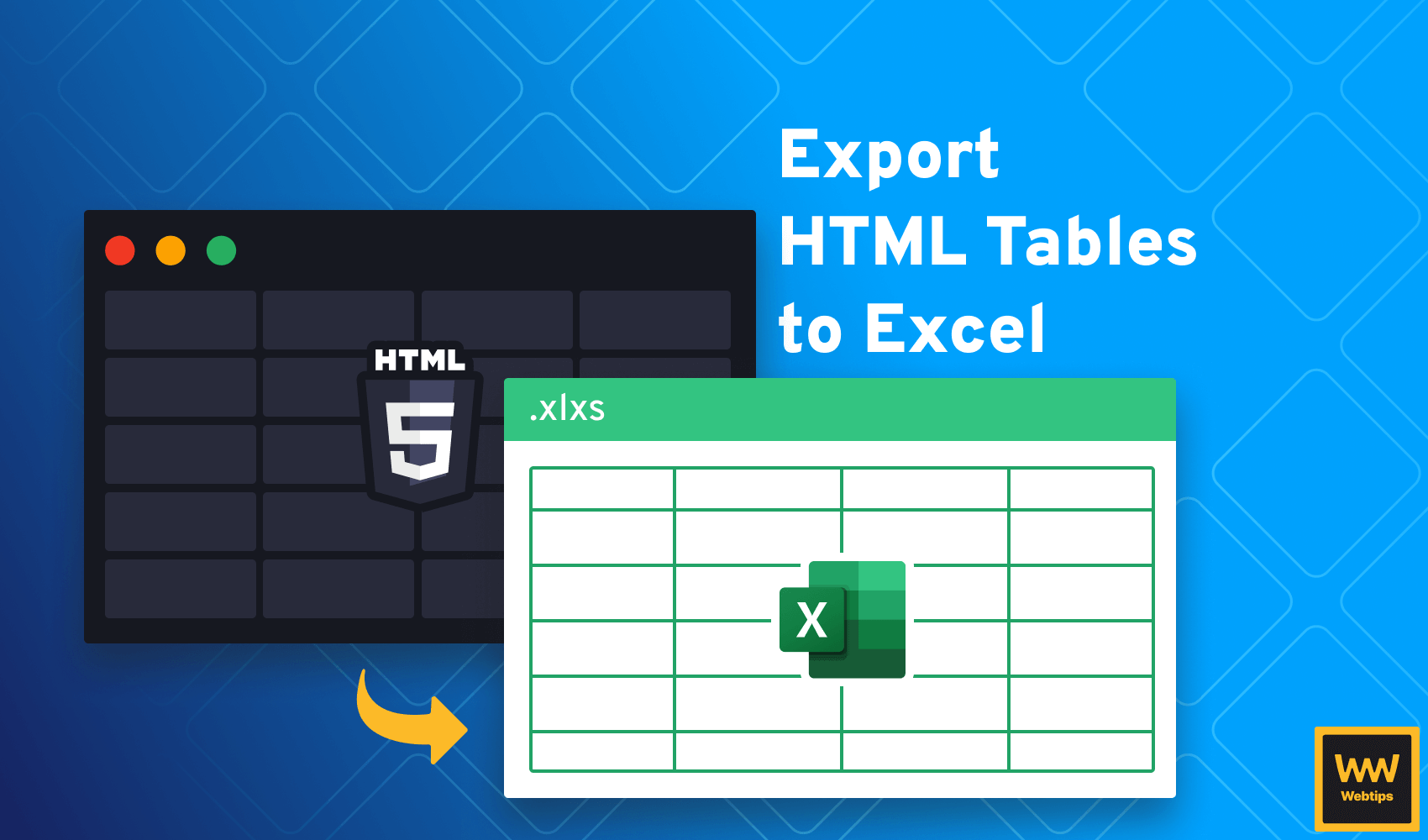
2 Easy Ways to Export HTML Tables to Excel
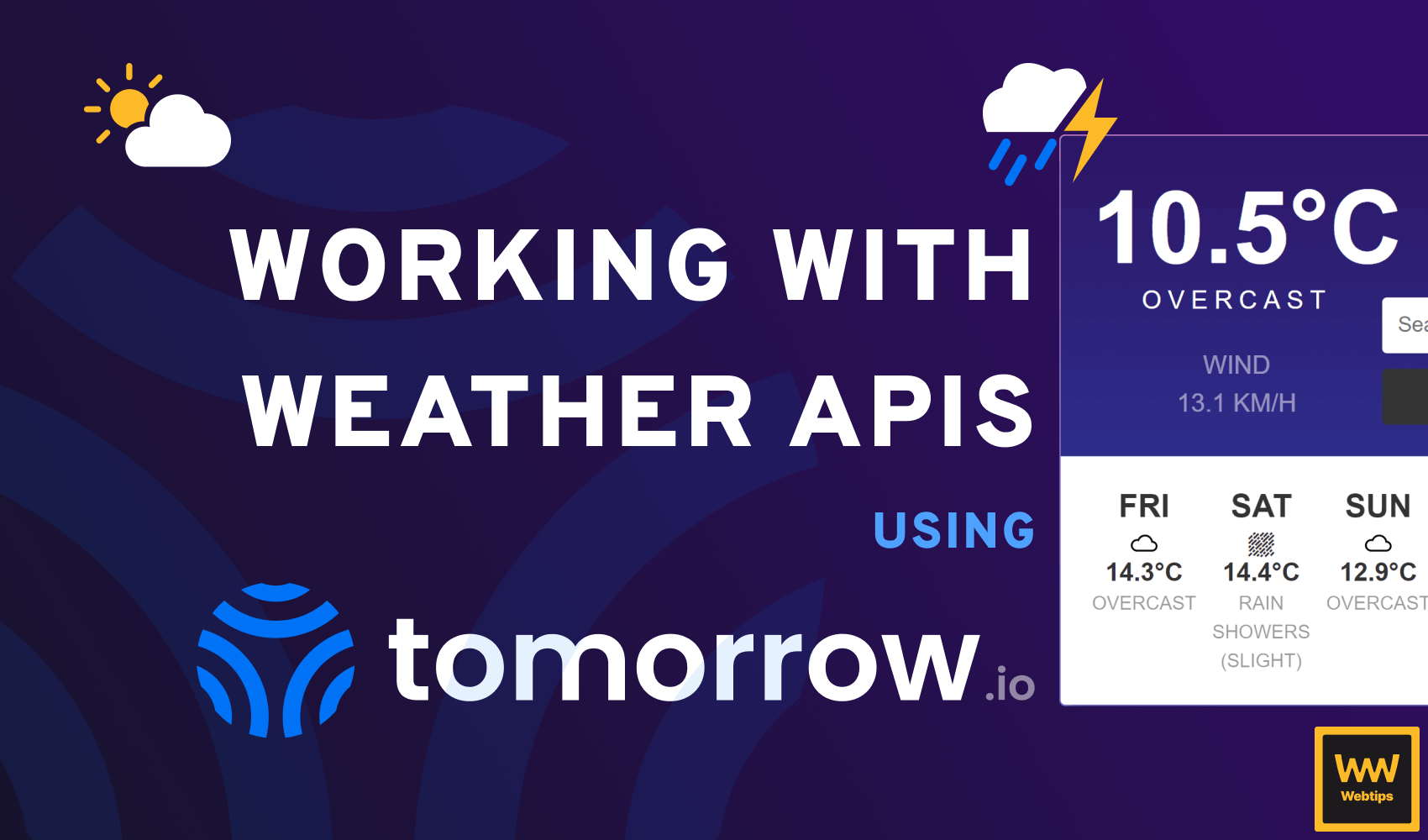
Unleashing the Power of Weather APIs: A Guide for Frontend Developers
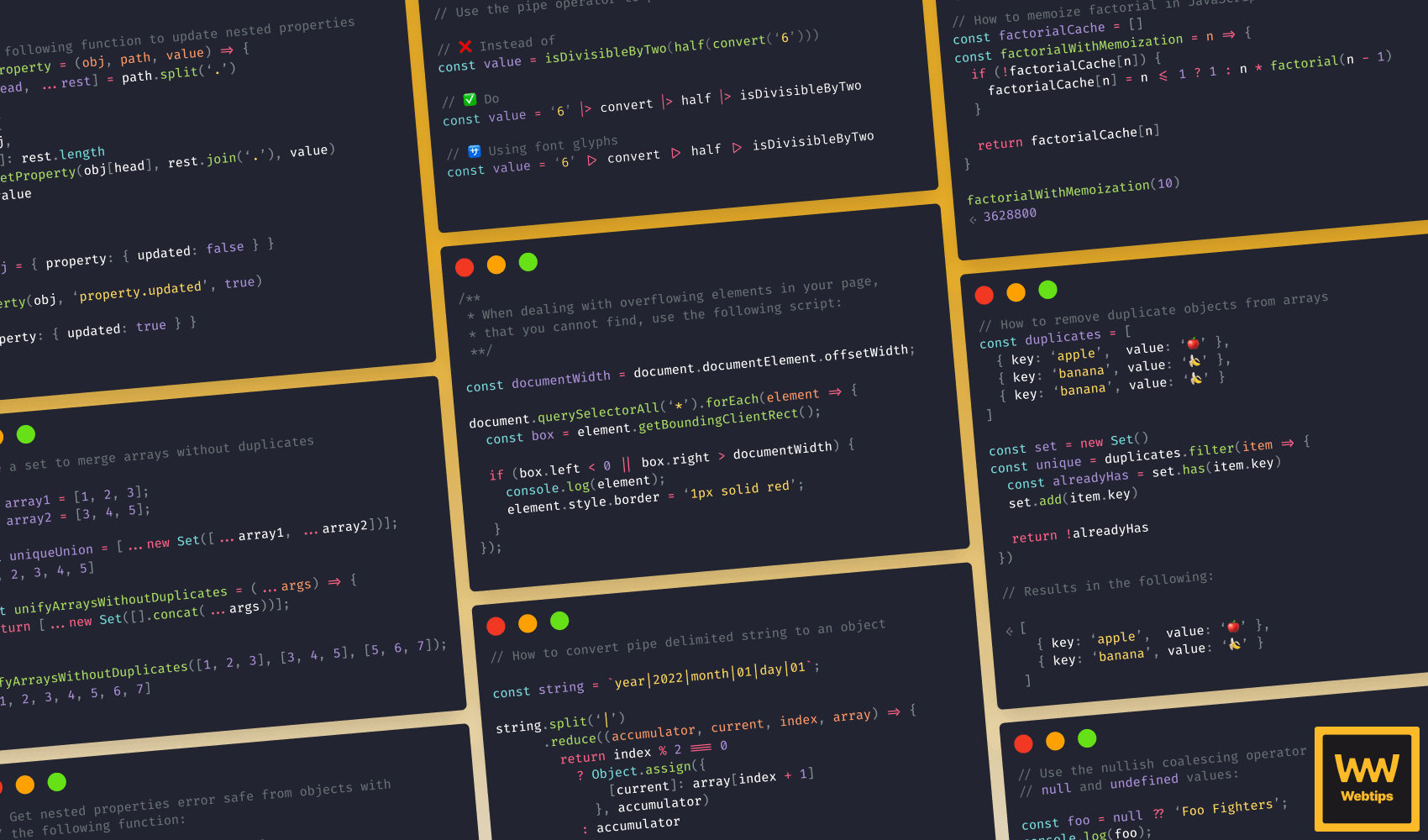
How to Select Everything Between HTML Comments With Regex
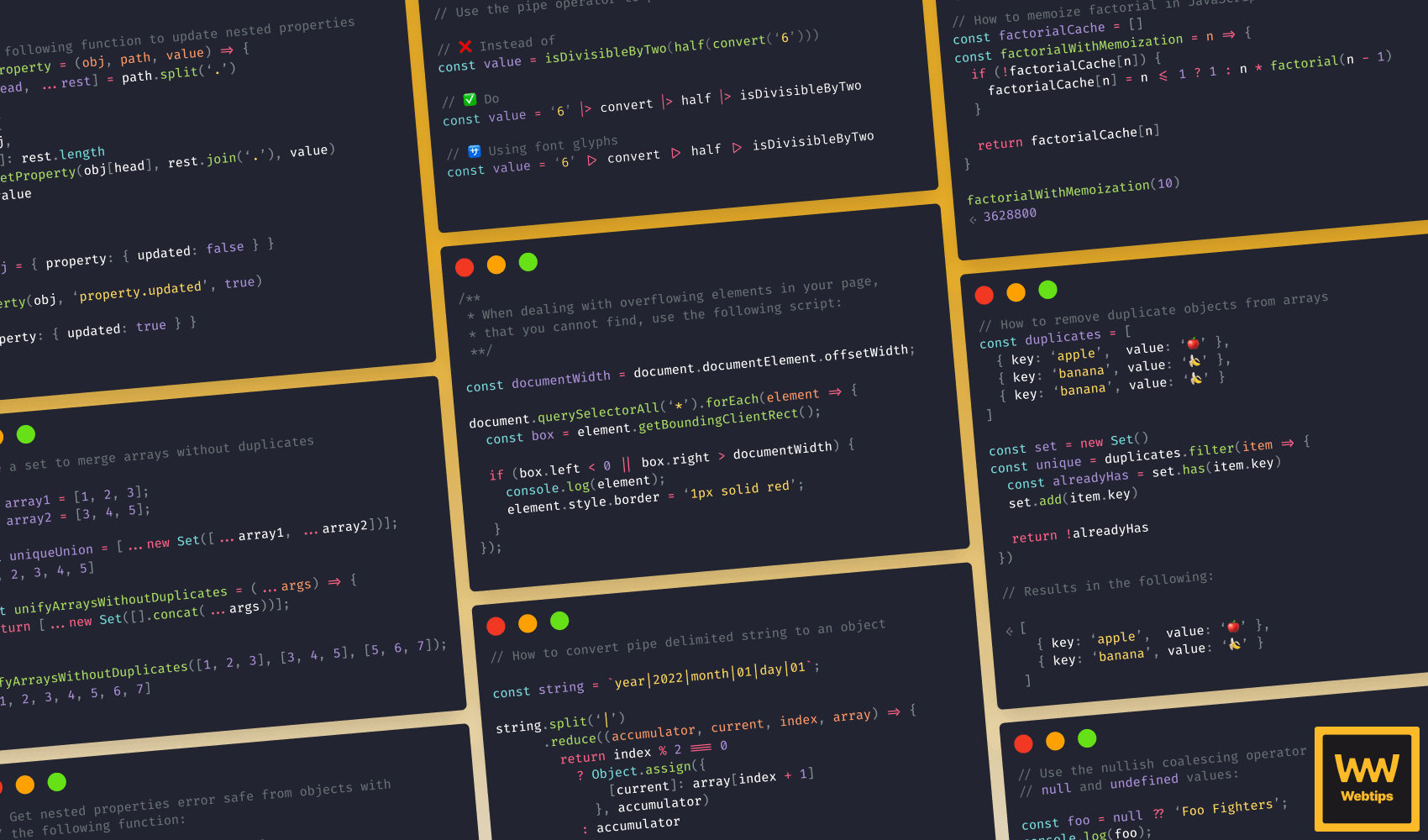
How to Fix "Cannot read property 'then' of undefined" in JS
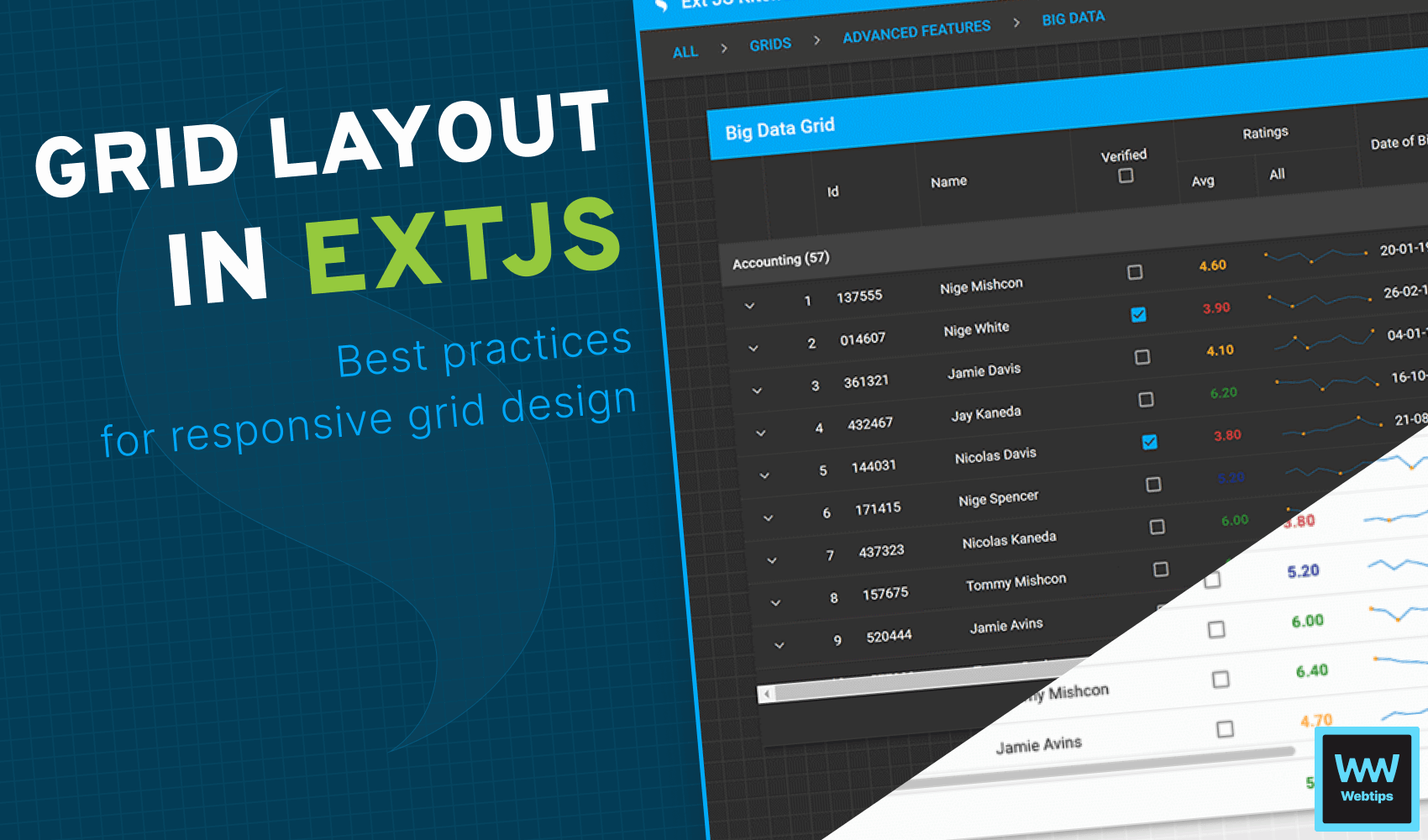
Effective Web App Development with Sencha Ext JS Grid Layout
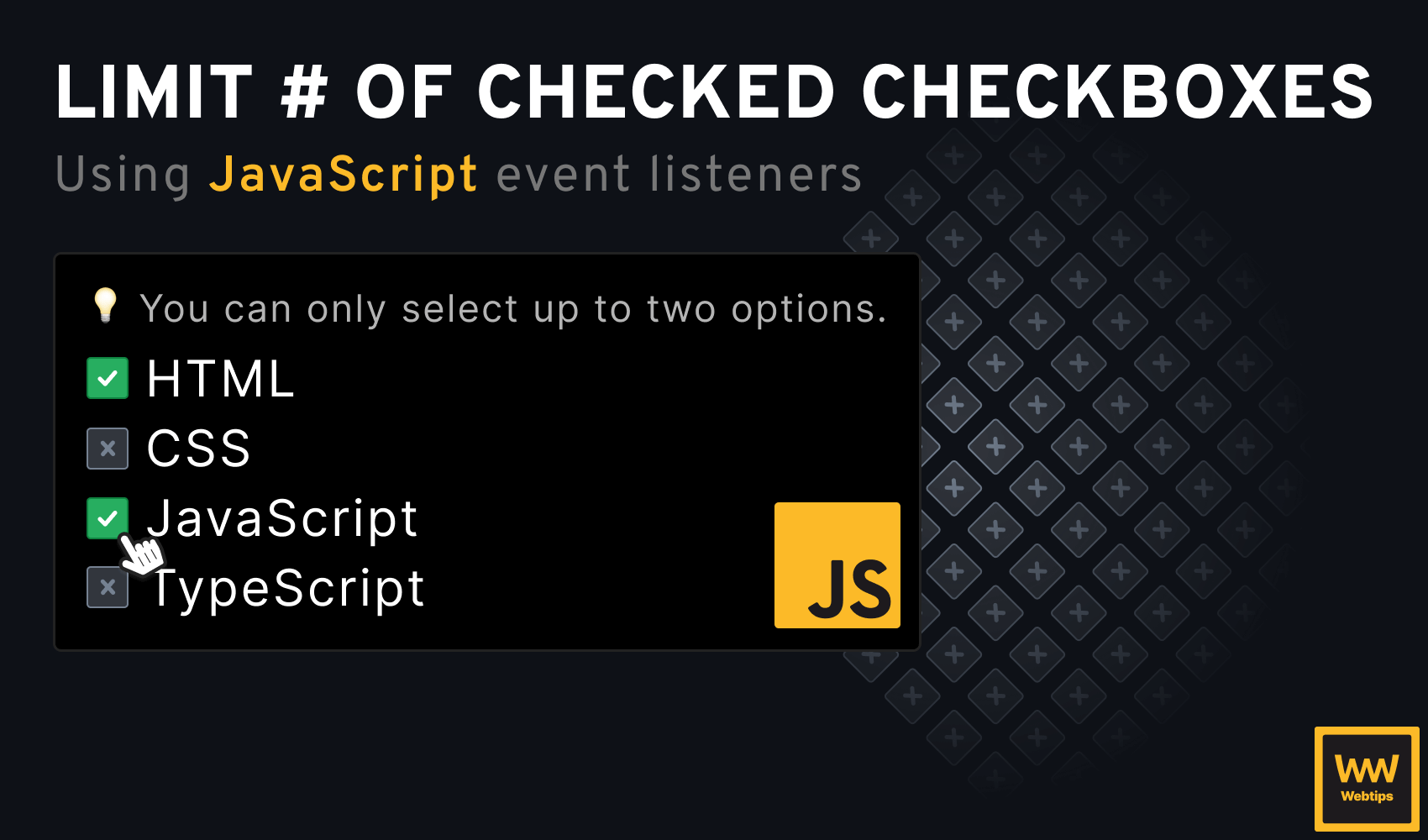
How to Allow Only One Checkbox to Be Checked in HTML
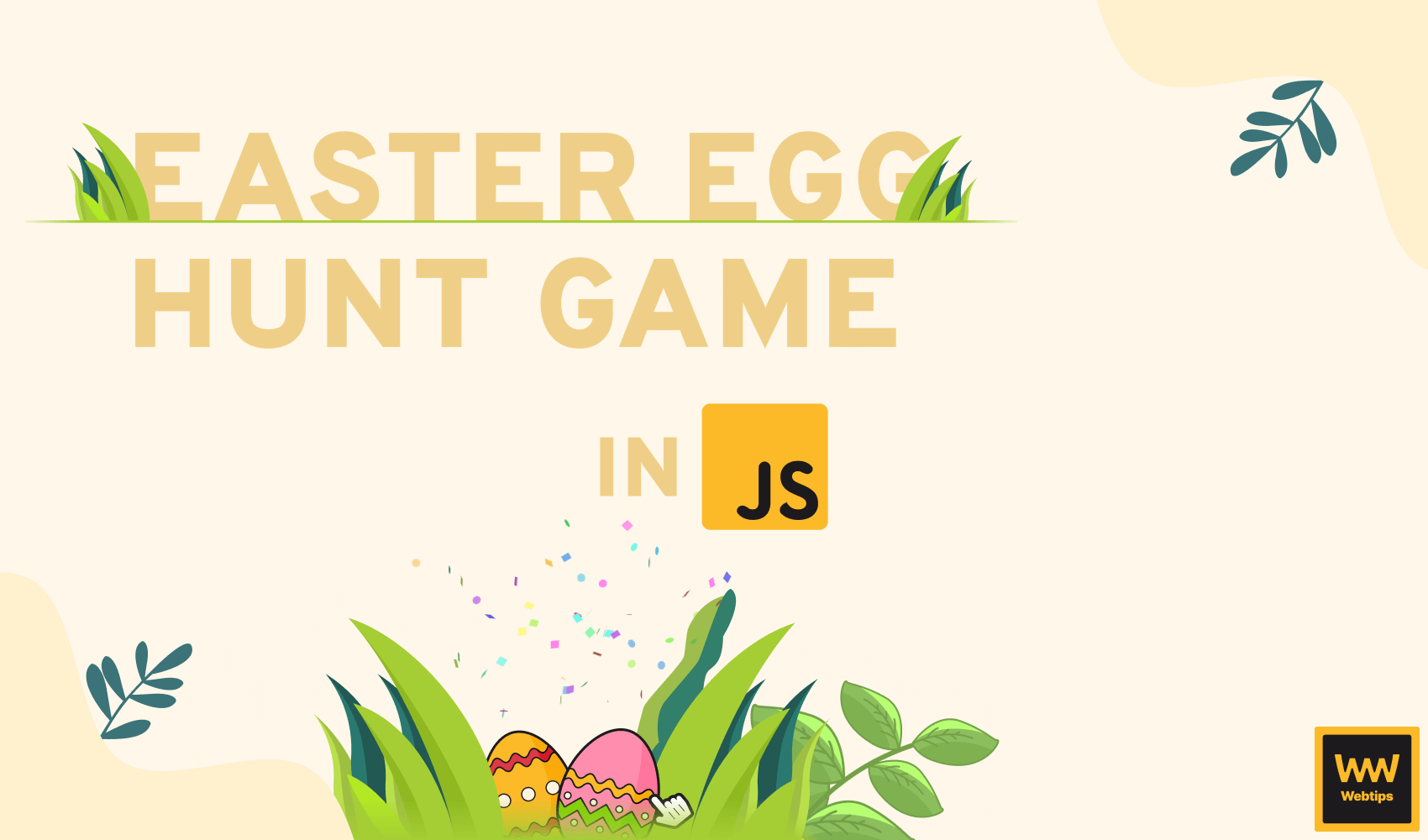
How to Make an Easter Egg Hunt Game in JavaScript
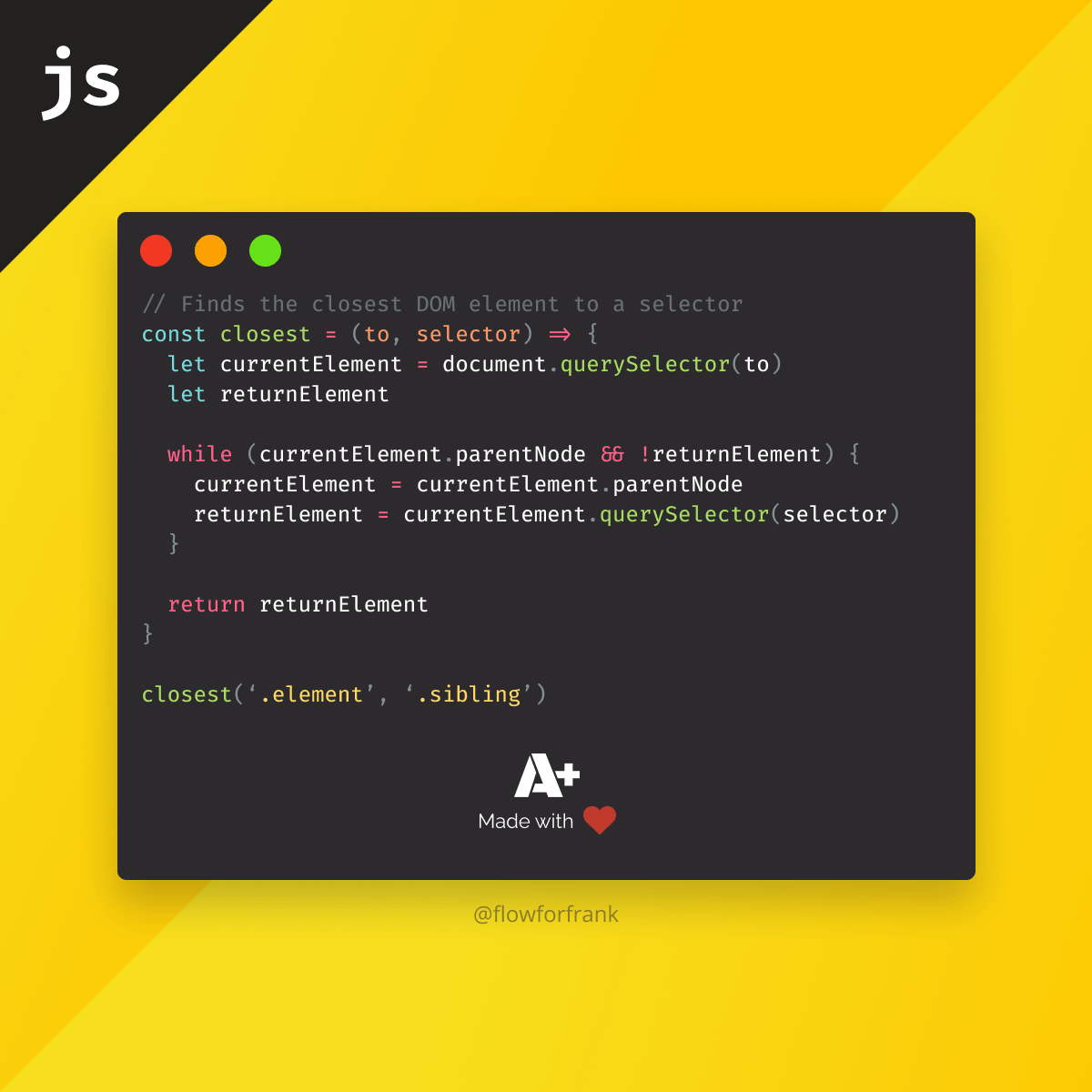
How to Find Any Closest Element in JavaScript
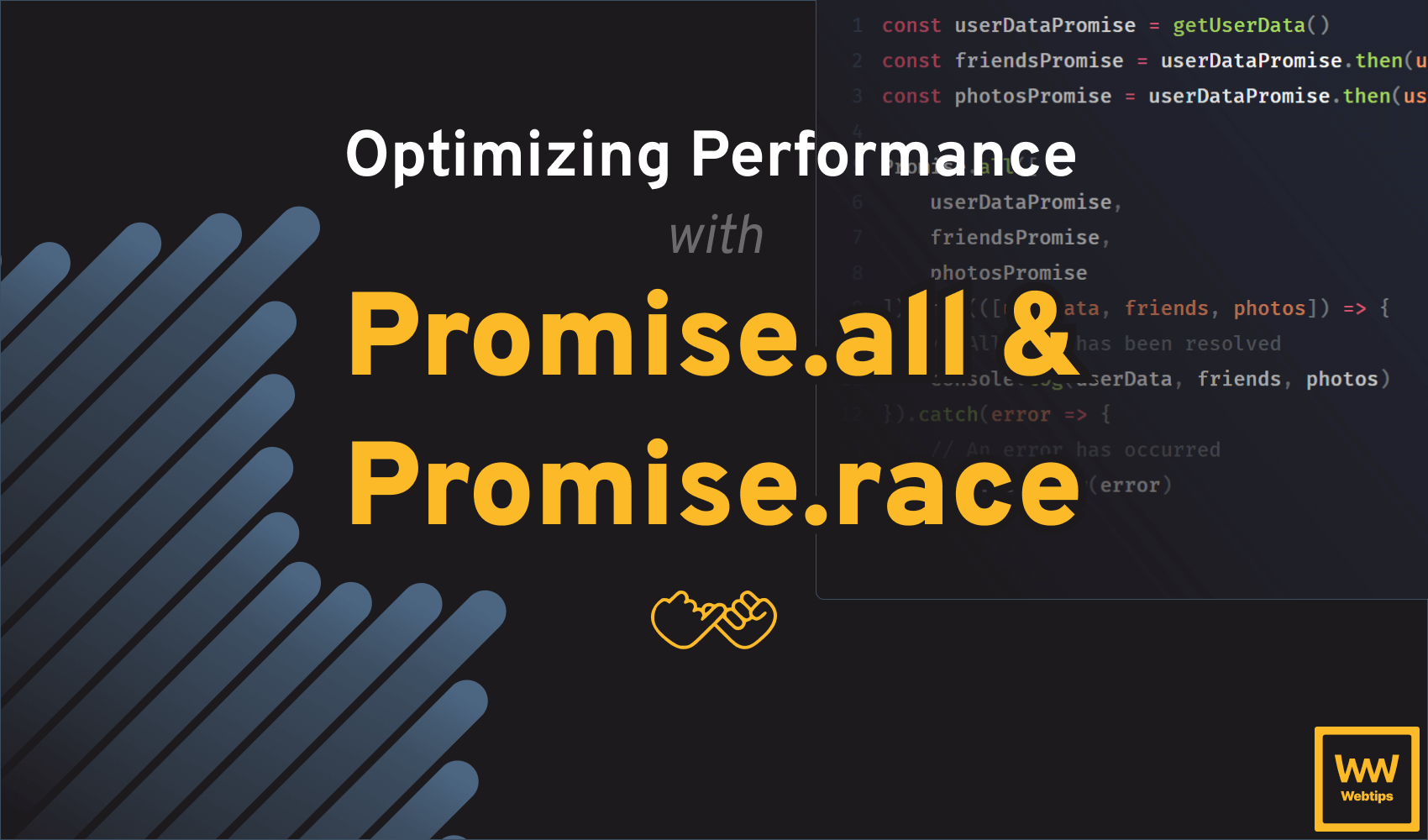
How to Optimize Performance with Promises in JavaScript
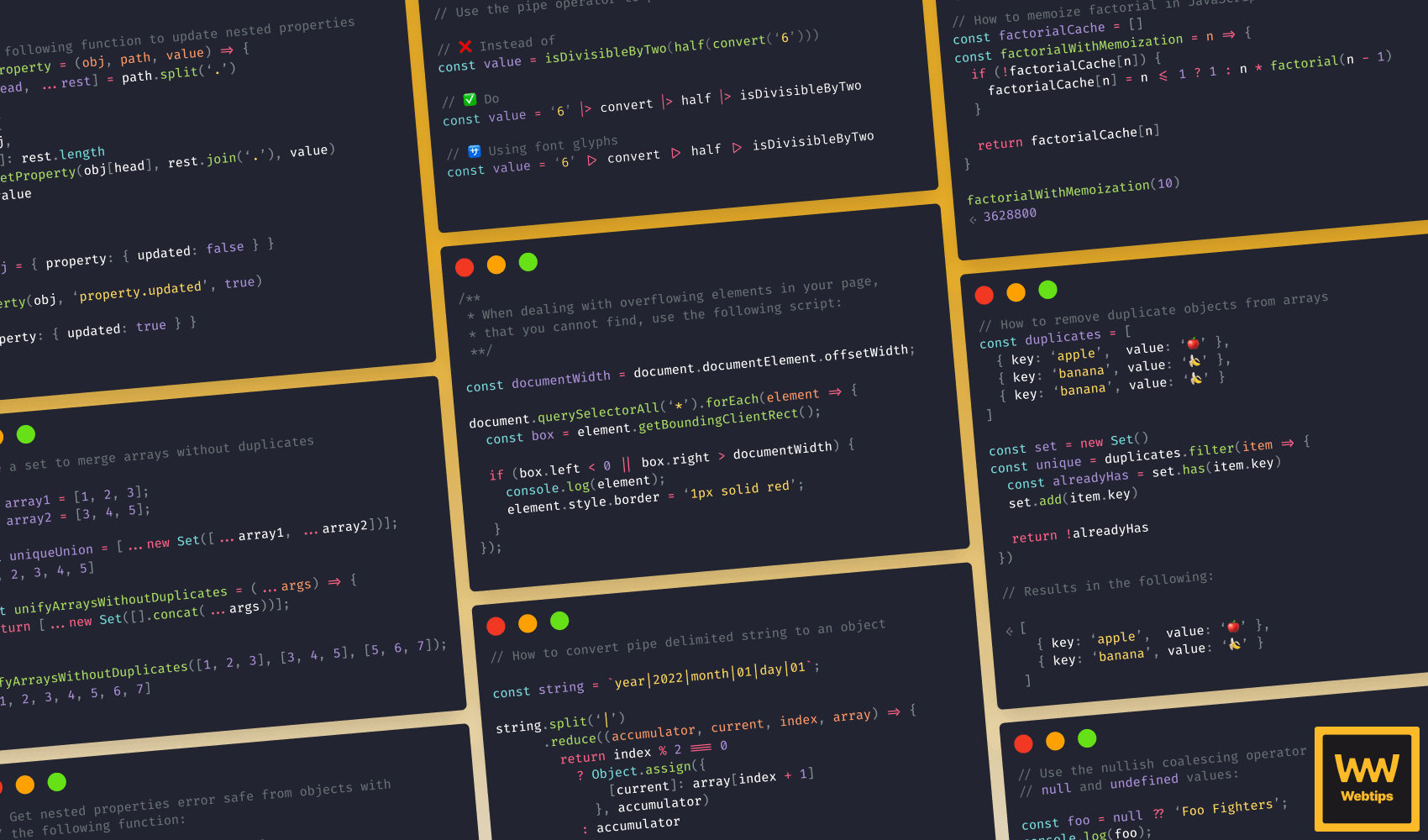
How to Fix "replaceAll is not a function" Errors in JS
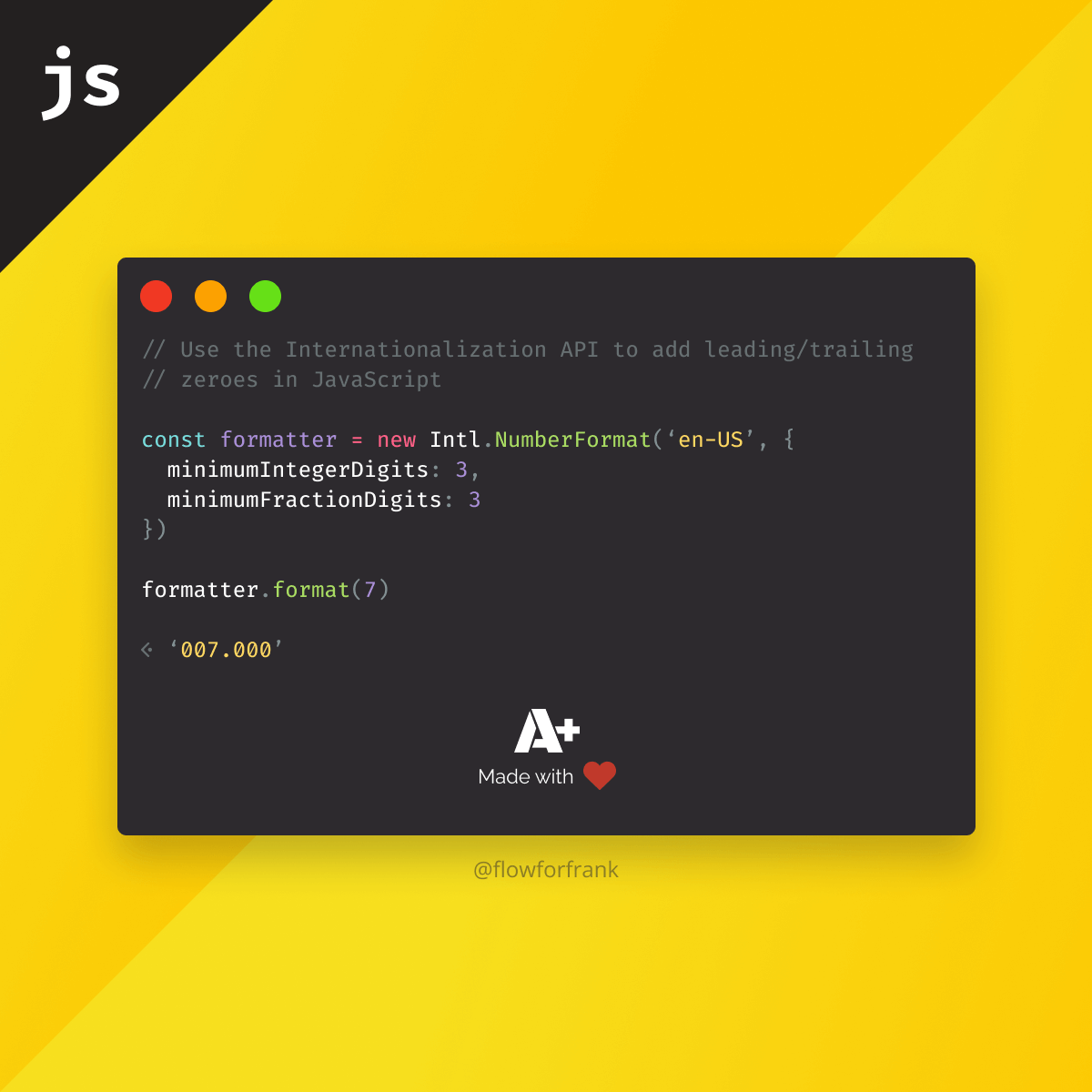
How to Add/Remove Leading/Trailing Zeroes in JavaScript
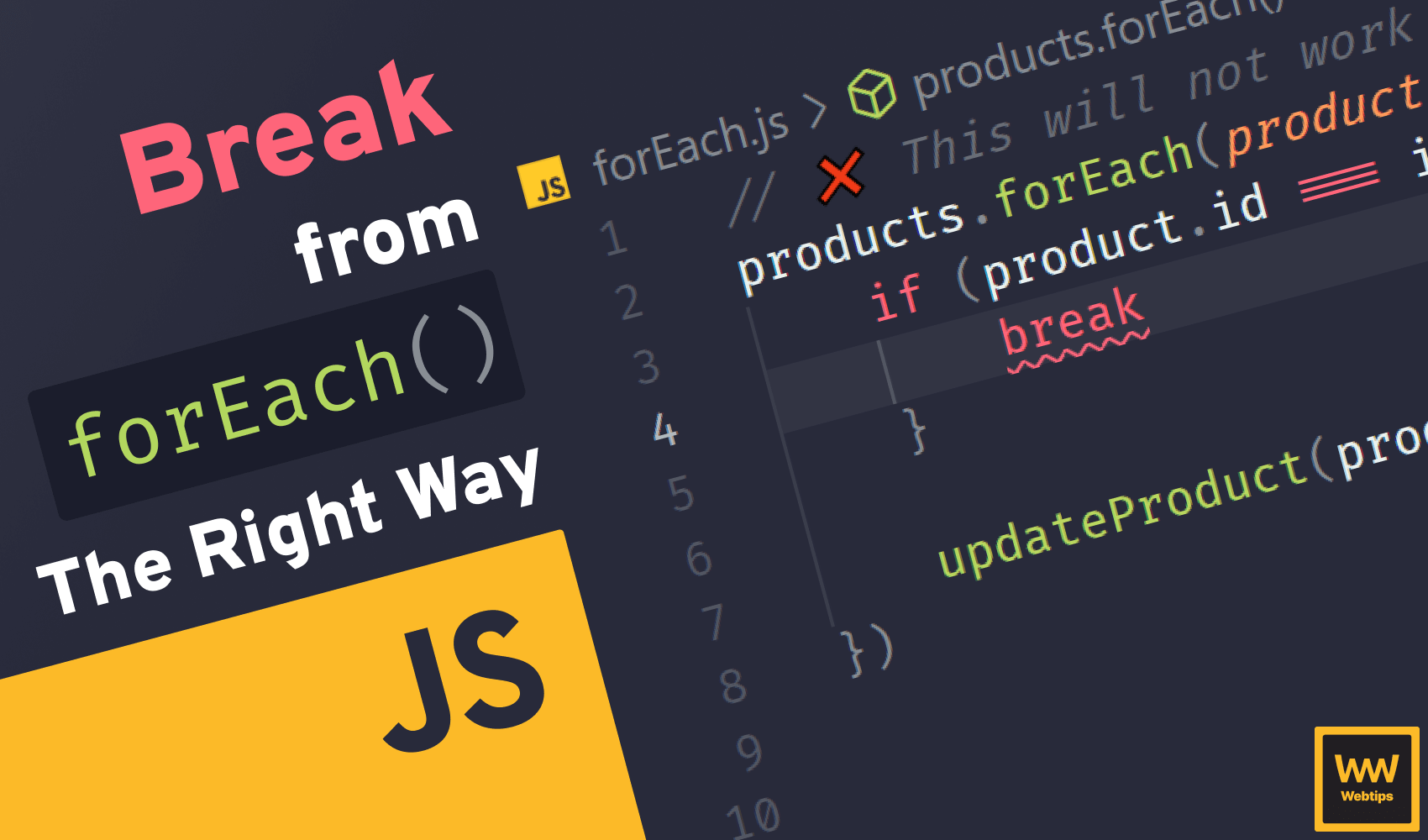
The Right Way to Break from forEach in JavaScript
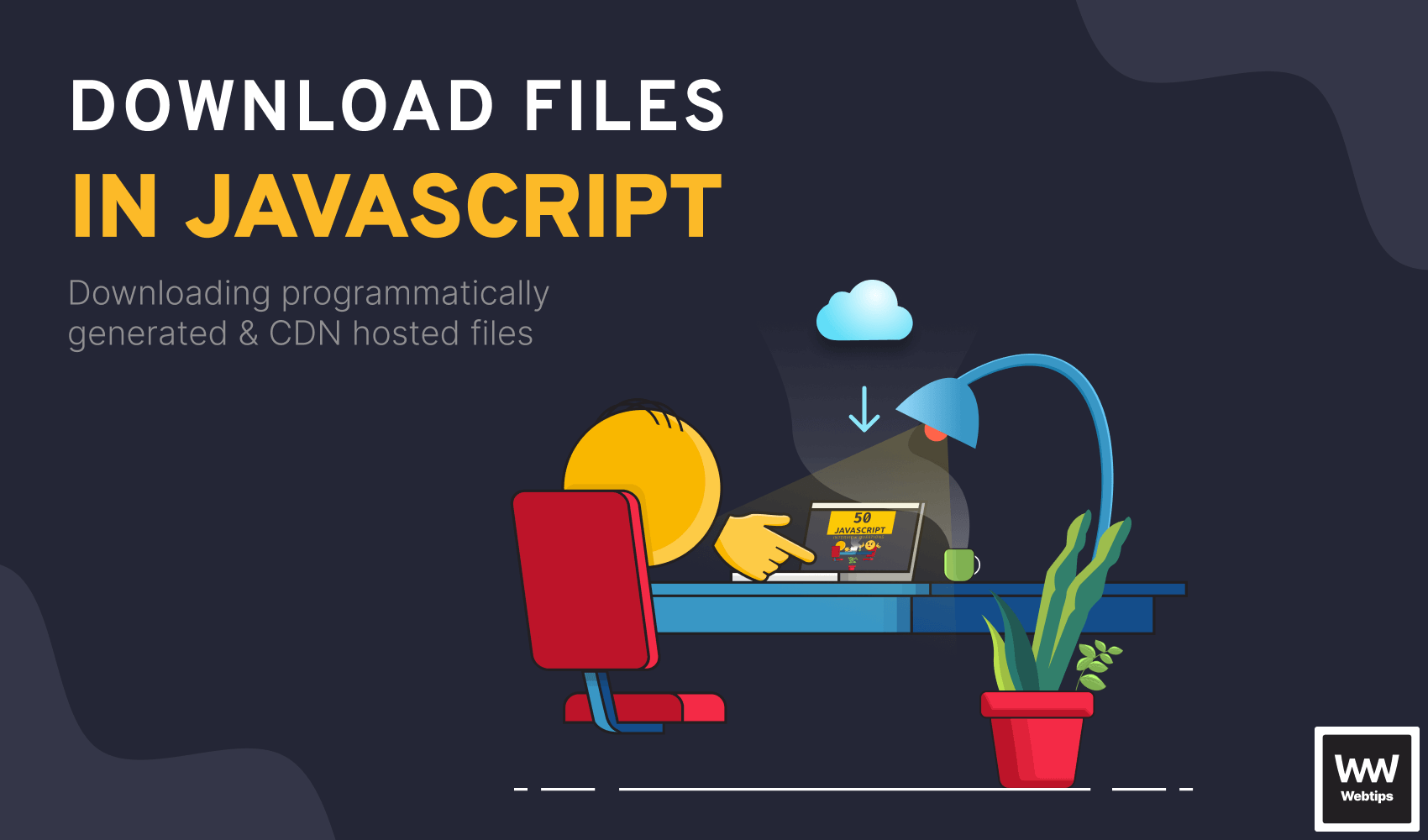
How to Download Any File In JavaScript
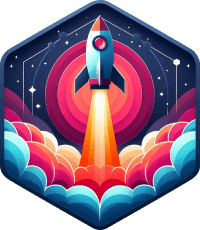
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: