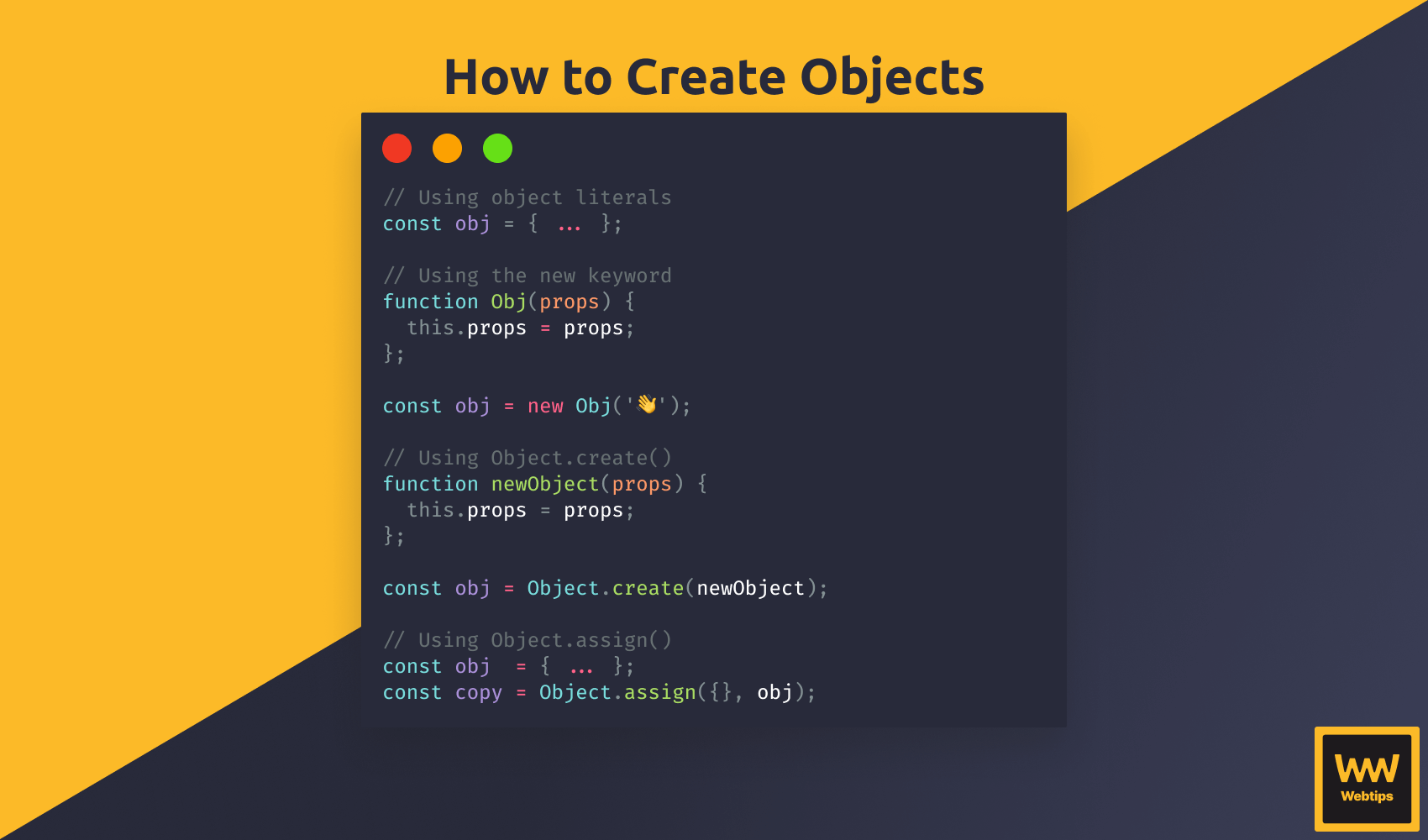
How to Create Objects in JavaScript
Objects are a fundamental data type in JavaScript, allowing us to organize data and behavior into reusable components. There are numerous ways to create objects in JavaScript. In this tutorial, we'll explore five different methods, each with its advantages and use cases. Let's start with the most common one.
Object Literals
Using the object literal syntax is the simplest and most common way to create objects in JavaScript. We can define properties using key-value pairs inside the curly braces:
// "obj" is commonly used as a short form of "Object"
const obj = {
key: 'value'
}
Keys follow the same rules variables do when it comes to naming. Note that we need to use a colon when assigning a value to them, instead of using an equal sign. They can get any type of value, including other objects:
The new Keyword
We can also use the new
keyword, with the built-in global Object
. This will create an empty object:
const obj = new Object()
We can also use the new
keyword on a constructor function. Anything we pass to the function will be available inside it as this.props
:
Here, the this
keyword references the object. We assign the passed properties (usually shortened to props
) to this.props
so it's available anywhere inside the function. Anything we pass to the function whenever we call it with the new
keyword will be available for us to use. Try extending the User
object with additional properties to verify they're logged to the console.

Using Object.create
Another way to create objects is by using the built-in Object.create
method. Whatever we pass to the create method will become the prototype of the object:
const obj = Object.create({})
Here we pass an empty object as the prototype, but we can pass anything we would like, as long as it's an object. For example, let's do the following:
Run the code and also try to log out obj.__proto__
as well to see that now weight
is also available on the prototype. Notice that we can reach the prototype using __proto__
.
If we want to get rid of the prototype, we can pass null
to Object.create
. This way, our object won't have a constructor
and other inherited methods such as hasOwnProperty
or toString
. This is called a pure object because it doesnβt inherit anything.
// Copy and paste the code to the above editor to verify the code
const pure = Object.create(null)
// No prototype will be present
console.log(pure.__proto__)
Using Object.assign
Using Object.assign
is another way of using built-in methods. This, however, copies the values of an existing object into another one. This means we can also use it to merge objects together. The method can take as many objects as we need to merge, the first parameter will be the object that is returned:
const obj1 = { a: 1 }
const obj2 = { b: 2 }
// Assign obj1 and obj2 to an empty object
const copy = Object.assign({}, obj1, obj2)
console.log(copy)
Object.assign
is commonly used to create a deep copy (copy the object and create a new reference) by passing an empty object as the first parameter.
Note that if you pass an existing object to Object.assign
, you also pass the reference, meaning if you modify something in the copy
, the original object will also be changed.
Using Classes
Lastly, we can also use classes. This is equivalent to creating objects in class-based languages. This option also makes use of the new
keyword, just like when using a constructor function. To create a new class, use the class
keyword:
Classes are used for encapsulating logic, properties, and functions into a single entity. In this example, we created a constructor
function that will be automatically called when we create a new class using the new
keyword. This name is reserved in classes and must be called βconstructorβ to be executed automatically. However, classes are usually not used in JavaScript, instead, functions are preferred.
Conclusion
In conclusion, choosing the right method for creating objects in JavaScript depends on your specific requirements and coding style preferences. Here's a quick recap and some recommendations on which one to use:
- Object Literals: Prefer using object literals most of the time. Use when you need a simple, one-off object with straightforward properties and methods.
- new keyword: Perfect for creating objects with customizable properties and methods. It's a versatile approach that excels in scenarios where you need to produce various object instances with similar characteristics.
- Object.assign: Use it when you need to merge multiple objects, or you need to copy an object without overriding the original object (creating a deep copy, the opposite of shallow copy).
- Object.create: Suitable for creating objects with specific prototypes, allowing for more flexible inheritance patterns. Use it when you need finer control over the prototype chain and object relationships.
- Classes: Use it when working on projects that leverage ES6 features, and components are encapsulated into distinct classes.
Is there anything else you'd like to know about object creation in JavaScript? Let us know in the comments below! Thank you for reading through, happy coding! To learn more about JavaScript, continue with our free guided roadmap:
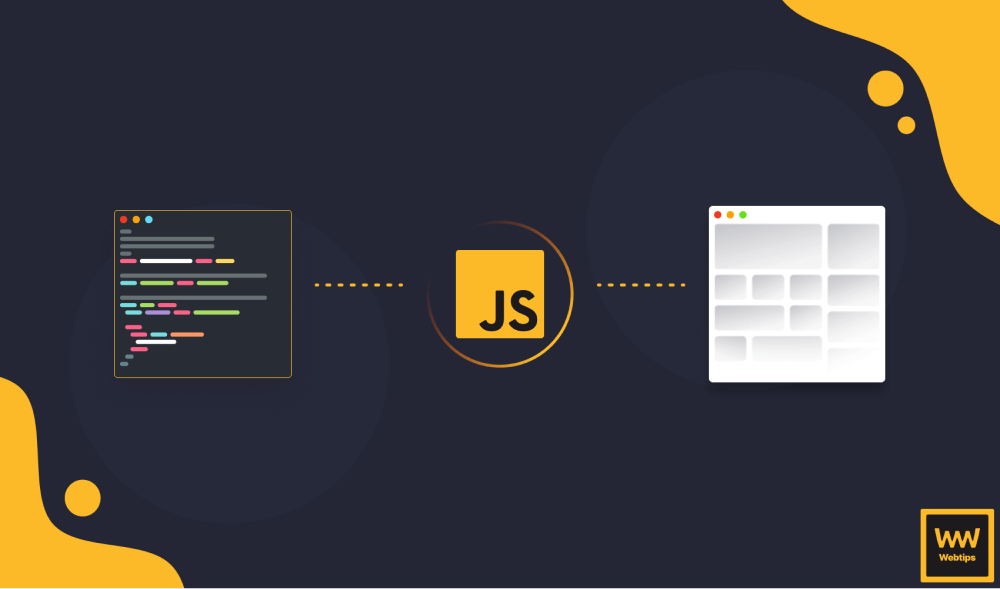
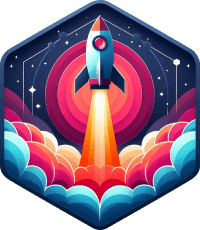
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: