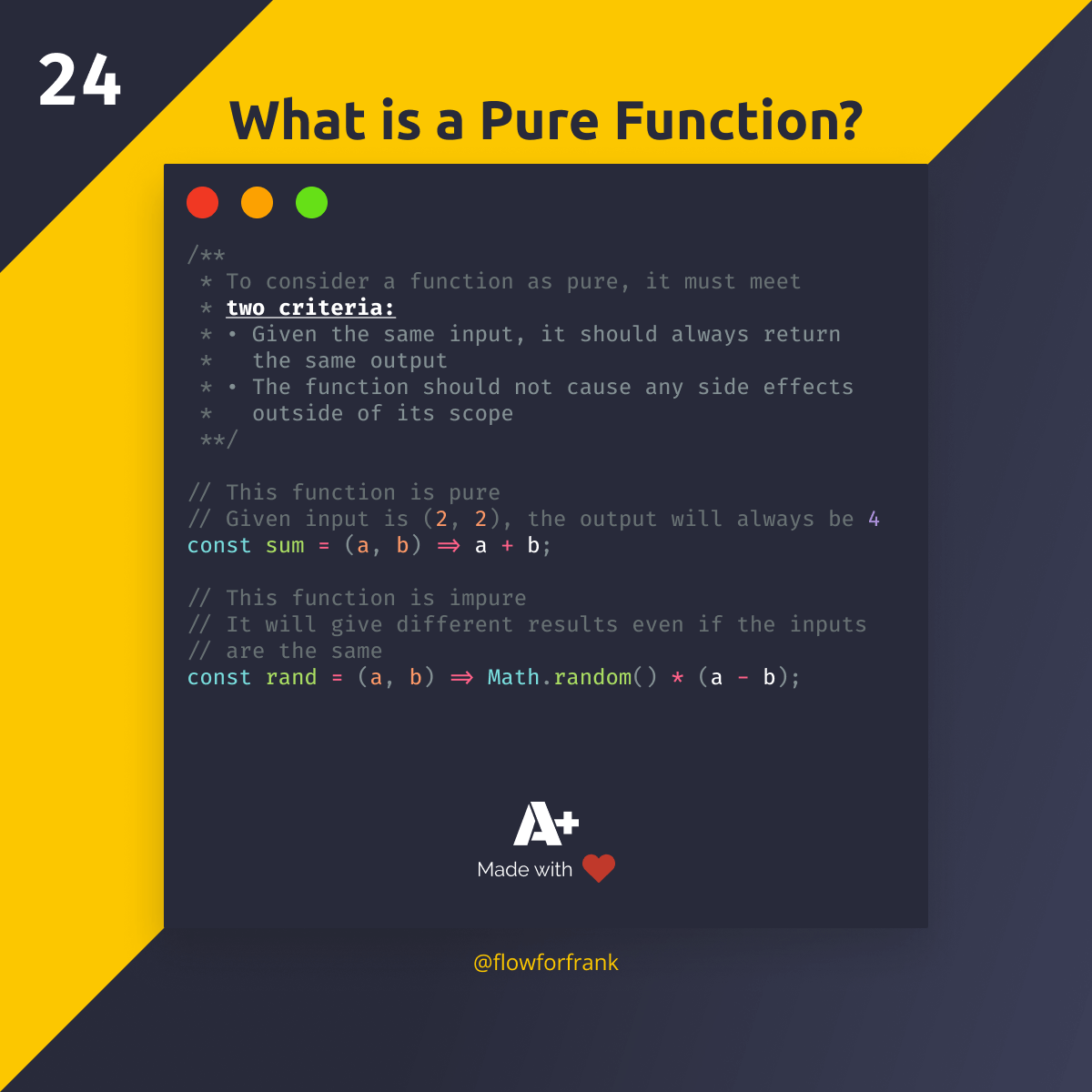
What is a Pure Function?
To consider a function as pure, it must meet two criteria:
- First, it should always return the same output given the same input.
- The function should not cause any side effects outside of its scope.
Side effects means, it doesn't alter data outside of their scope. Take the following as an example:
// This function is pure
// Given the input is 2, 2, the output will always be 4
const sum = (a, b) => a + b;
In the example above, the function is pure. No matter what, if you give it the same inputs over and over again, it will produce the same output. It is deterministic. Now let's take a look at an impure function.
// This function is not pure
// It will give different results even if the inputs are the same
const rand = (a, b) => Math.random() * (a - b);
In the example above, the function is impure. Even if you give it the same input multiple times, it is highly likely it will produce a different output. Also note that if a function doesn't have a return value, it is also considered to be impure.
The advantage of pure functions is that they can be easily covered by unit tests since they are deterministic. However, your goal shouldn't be to make everything pure. For example, you can have network requests that can return different values. These are also impure. Your goal should be about being deliberate about why and when side effects can occur.
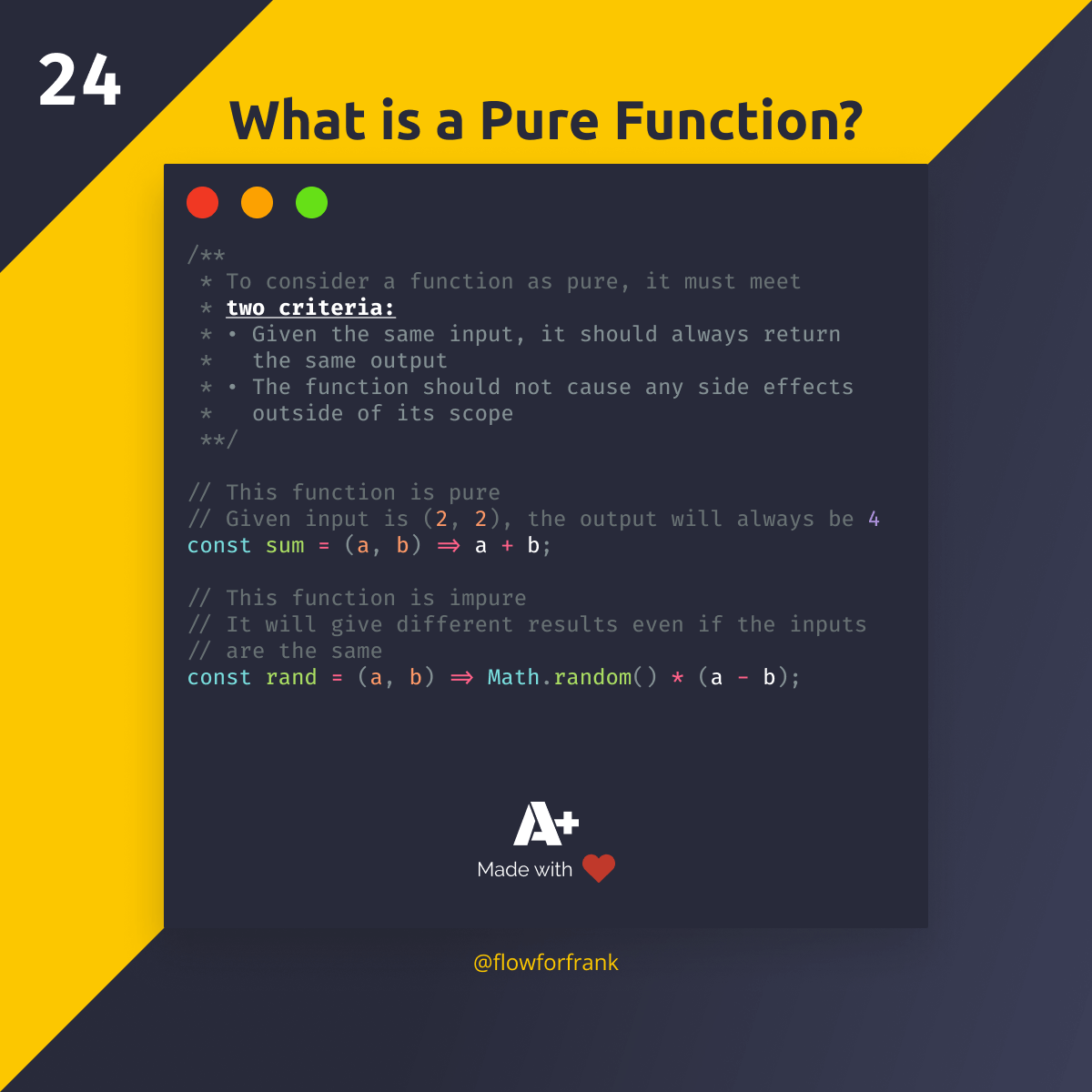
If you would like to learn more about functional programming, make sure you check out the article below.
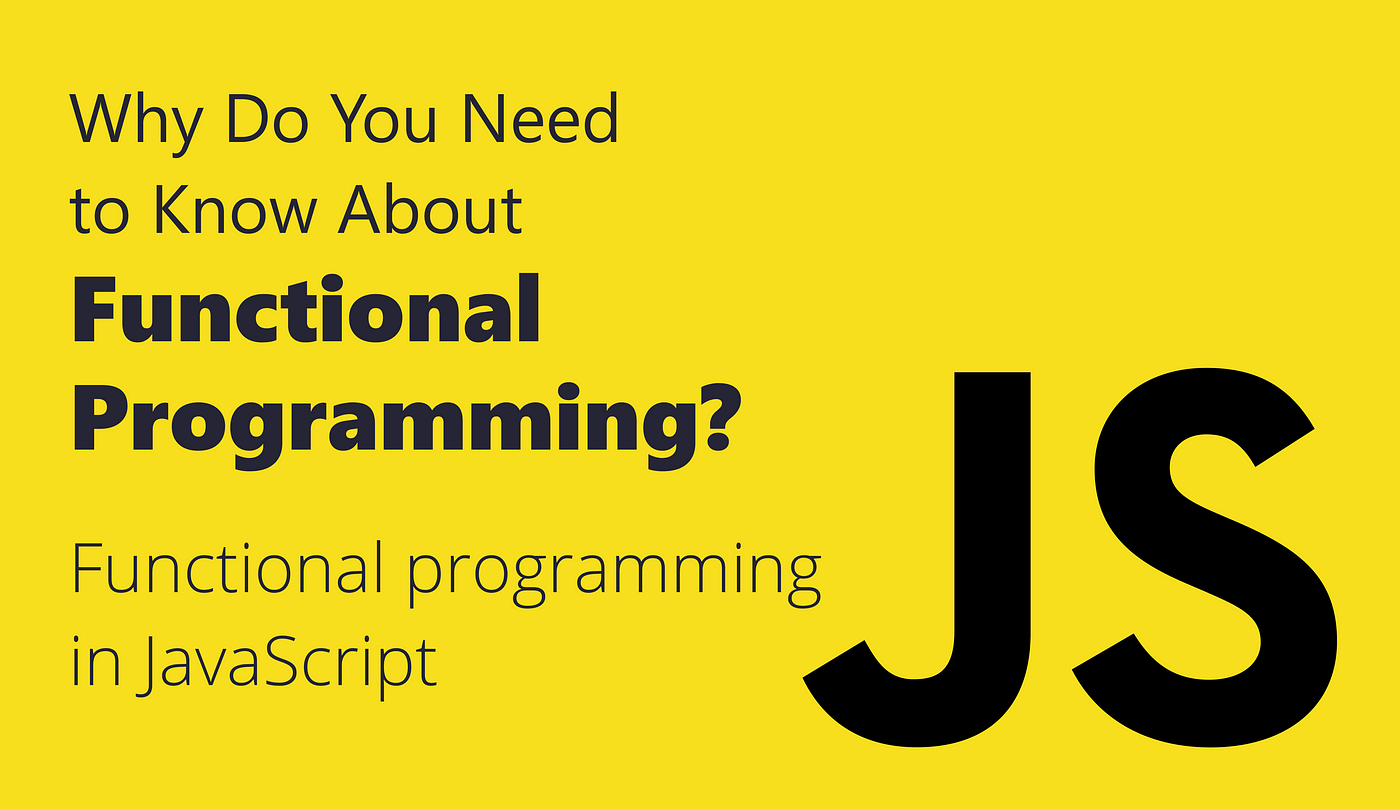
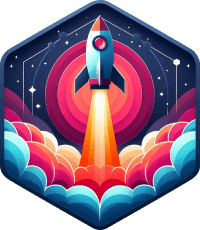
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: