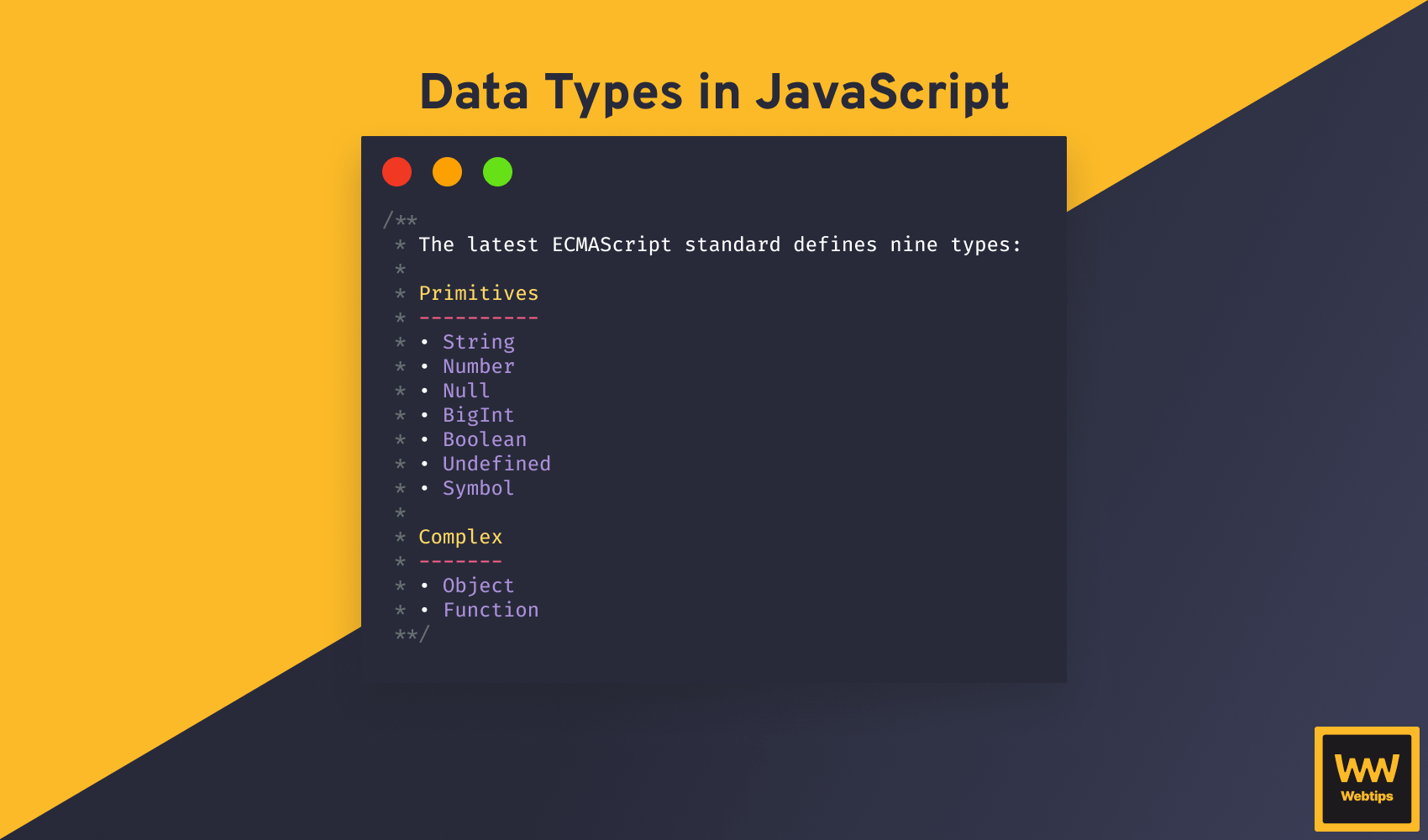
JavaScript Roadmap Other roadmaps
Learn JavaScript with our guided roadmapThis roadmap covers and takes you through the essential concepts in JavaScript, from basic syntax to more advanced concepts like functional and asynchronous programming. By the end of this roadmap, you will have a better understanding of how JavaScript works and how you can apply it to real-world problems.
If you would like to explore more JavaScript tutorials without a direction, you can filter for the tutorials you are interested in on our JavaScript category page.
What is covered?
JavaScript fundamentals
Functional programming
Working with arrays and objects
Understanding
this
,bind
,call
andapply
The event loop
How to use async operations
Browser APIs
Building applications
1Learn what are the fundamental data types in JavaScriptThere are multiple data types in JavaScript. By data type, we simply mean the type of a value. In this tutorial, you will get to know what data types are available in JavaScript. 2Working with Variables
The differences between var, let and constThere are three keywords in JavaScript that you can use for variable declarations: var, let, and const. In this tutorial, you will learn how each can be used differently. 3Logical Operators
Learn logical operators in JavaScirpt under 10 minutesLearn how to use logical operators in JavaScript to connect and evaluate multiple expressions. 4Function Declarations
Function expression vs function declarationIn this tutorial, you will learn what the difference is between a function expression and a function declaration in JavaScript, and why it's important to know about them. 5The Power of Function Composition
A programming paradigm that simplifies your codeJust like object-oriented programming, functional programming has its own concepts too. Learn how you can apply them to JavaScript to make your code simpler. 6The Power of Higher-Order Array Methods
find, some, map, reduce, every, filterLearn how to utilize built-in array methods in JavaScript to easily manipulate arrays. 7How to Create Objects in JavaScript
5 different examples with codeThere are numerous ways to create objects in JavaScript. In this tutorial, you will learn five different ways to create objects. 8Dictionaries in JavaScript
Taking a look at the object data typeUnlike in statically typed languages, there is no Dictionary type in JavaScript. Learn what are the alternative data types to achieve the same. 9The this Keyword in JavaScript
Demystifying JavaScript's this keywordIn this tutorial, we will demystify the this keyword in JavaScript once and for all through a series of practical examples. 10Bind, Call and Apply in JavaScript
Function methods demonstrated through examplesUnderstanding the differences between bind, call, and apply should be an essential toolkit in your pocket. Learn what they mean in JavaScript. 11What is the Event Loop?
How JavaScript is executed in browsersTo fully understand how JavaScript is executed in browsers, you first need to understand the concept of the event loop. 12JavaScript Promises
Putting a stop to callback hellLearn how asynchronous code works in JavaScript, and how we can use promises in place of callbacks to achieve asynchronicity. 13What is the DOM
Understanding the Document Object Model in and outThere are more than 1.5 billion active websites on the internet, all made up of the same model: the Document Object Model or DOM for short. What is it? 14The Fetch API
Learn how to grab data from other resourcesIn this lesson, you are going to learn about the built-in Fetch API which can be used to fetch data in JavaScript using many different ways. 15Building JavaScript Projects
Continue learning by building these projectsLearning is a continuous process. Now that you are familiar with how JavaScript works, it's time to put theory into practice. Solidify your knowledge by building these projects in JavaScript.π Unlock this lesson 16Conditions and Loops
Learn how to work with conditions and loops for repeating codeWorking with conditions and loops is a core part of any programming language. In this lesson, you will learn about if statements, switch statements, for loops, and while loops.π Unlock this lesson 17Arithmetic Operators
Learn how arithmetic operators can help you create various expressionsIn this lesson, we are going to take a closer look at arithmetic operators - the ones that you are already familiar with from your math class, as you will be using them a lot in JavaScript.π Unlock this lesson 18Comparison Operators
Learn strict equality and comparing objectsLearn about comparison operators and how they help you to evaluate expressions.π Unlock this lesson 19Parameters and Arguments
Learn how you can make functions more flexible by using parametersLearn the difference between parameters and arguments, and how you can use them to make your functions versatile.π Unlock this lesson 20Function Returns
Master the use of the return keywordLearn how to use the return keyword to retrieve values from functions, how to return entire functions, and how to write early returns.π Unlock this lesson 21Invoking Functions
Learn about IIFE, closures and built-in functionsIn this lesson, you will learn about the different ways to invoke functions and what built-in functions are available in JavaScript.π Unlock this lesson 22Working with Arrays
Creating, accessing, modifying and looping over arraysArrays in JavaScript are indexed collections that can hold more than one value. Learn why we need arrays in JavaScript and how you can work with them.π Unlock this lesson 23Properties and Methods
Learn how to work with object properties and methodsIn this lesson, we are going to take a look at how to manipulate the properties of objects, as well as how to work with different methods.π Unlock this lesson 24JavaScript Callbacks
Learn what asynchronicity means, and how callbacks can help us achieve itWith the help of callbacks and asynchronous code, we can enhance the way JavaScript works and have functionality that is not possible without it.π Unlock this lesson 25Async/Await in JavaScript
Learn how to make your code work synchronously with async/awaitLearn how you can use async/await in conjunction with Promises to write asynchronous code in a synchronous manner.π Unlock this lesson 26The Local Storage API
Learn how to store data inside the browserThe Local Storage API allows us to save and read data to a user's browser. This data is kept so we can retrieve it, even after the user closes their browser.π Unlock this lesson 27Building an App - Part I.
Learn how you can build an app from scratch in JavaScriptIn this and the following lesson, you will practice the learnings to build a functioning application in JavaScript from the ground up.π Unlock this lesson 28Building an App - Part II.
Finishing the applicationWith this lesson, you are going to finish this roadmap, and you are ready to take your skills to the next level with the provided resources.
πΊοΈ Related roadmaps
Continue learningHTML Roadmap
Learn how to work with HTML using our guided roadmapThe roadmap for guiding you through the fundamentals of working with HTML. Learn how you can write semantic, SEO-friendly HTML documents.Building JavaScript Projects
Learn how to work with JavaScript using our guided roadmapThis roadmap covers and takes you through various projects to help you practice JavaScript while also building a portfolio of applications.React Roadmap
Learn React with our guided roadmapThis roadmap takes you through the essential concepts in React, from JSX, props and state, to concepts like lazy loading, suspense and error boundaries.
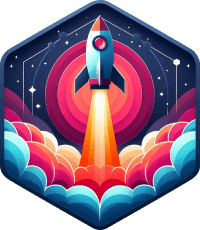
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: