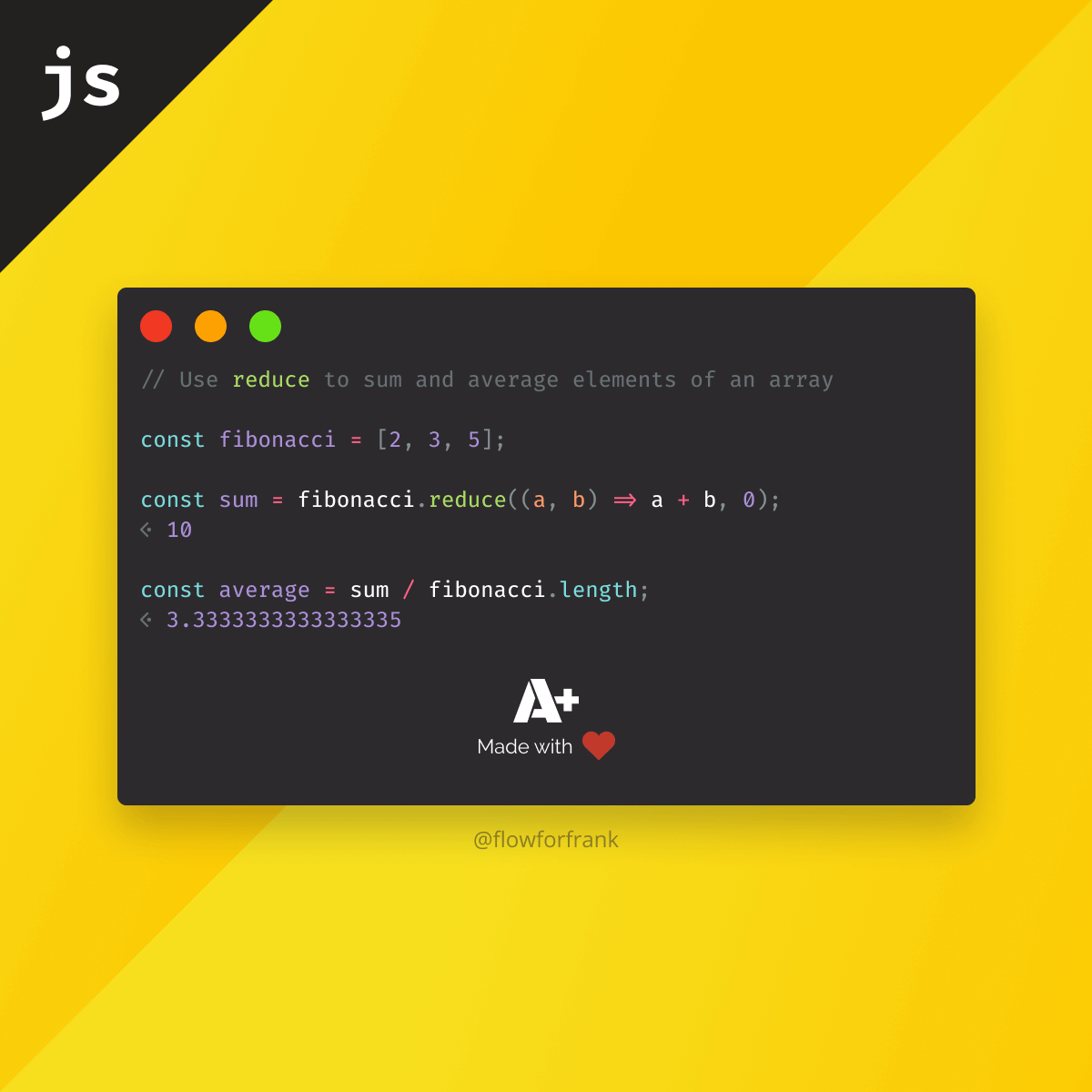
How to Find the Sum and Average of Elements in an Array in JavaScript
If you need to compute the sum or the average of an array of elements in JavaScript, you can use reduce
to get the shortest possible solution:
const fibonacci = [2, 3, 5];
// Will return 10
const sum = fibonacci.reduce((a, b) => a + b, 0);
// Will return 3.3333333333333335
const average = sum / fibonacci.length;
If you are unsure whether the elements of the array are numbers, you can also cast them to one, using the built-in Number
function:
const sum = fibonacci.reduce((a, b) => (Number(a) + Number(b)) || 0, 0);
Explanation
Let's break this code down line-by-line so we fully understand how does it work. We start with this line:
fibonacci.reduce((a, b) => a + b, 0);
The reduce
function takes in two arguments:
- A reducer function that you can define, that results in a single output for the element. This function has two mandatory arguments:
- The accumulator, denoted by
a
. This is the accumulated value returned from the last invocation of the callback. - The current value, denoted by
b
, that is being processed in the array.
- The accumulator, denoted by
- Optionally, you can also provide an initial value that will be used as the first argument to your callback. In the example above, we provided an initial value of 0.
With the example array, these are the values of each argument, step by step:
[2, 3, 5].reduce((0, 2) => 0 + 2, 0); // First iteration -> returns 2
[2, 3, 5].reduce((2, 3) => 2 + 3, 0); // Second iteration -> returns 5
[2, 3, 5].reduce((5, 5) => 5 + 5, 0); // Third iteration -> returns 10
And to find the average, we can divide the sum by the number of numbers. In the second example, when we were unsure about whether we deal with numbers, we have the following:
fibonacci.reduce((a, b) => (Number(a) + Number(b)) || 0, 0);
But why do we need the || 0
at the end? This is because if either a
or b
cannot be converted to a number, the return value will be NaN
. NaN
+ NaN
, or NaN
+ any number will be returned as NaN
, and we don't want that, so instead, we fall back to 0.
β
Number('1') + Number('2') -> 1 + 2 -> 3
π΄ Number('a') + Number('b') -> NaN + NaN -> NaN
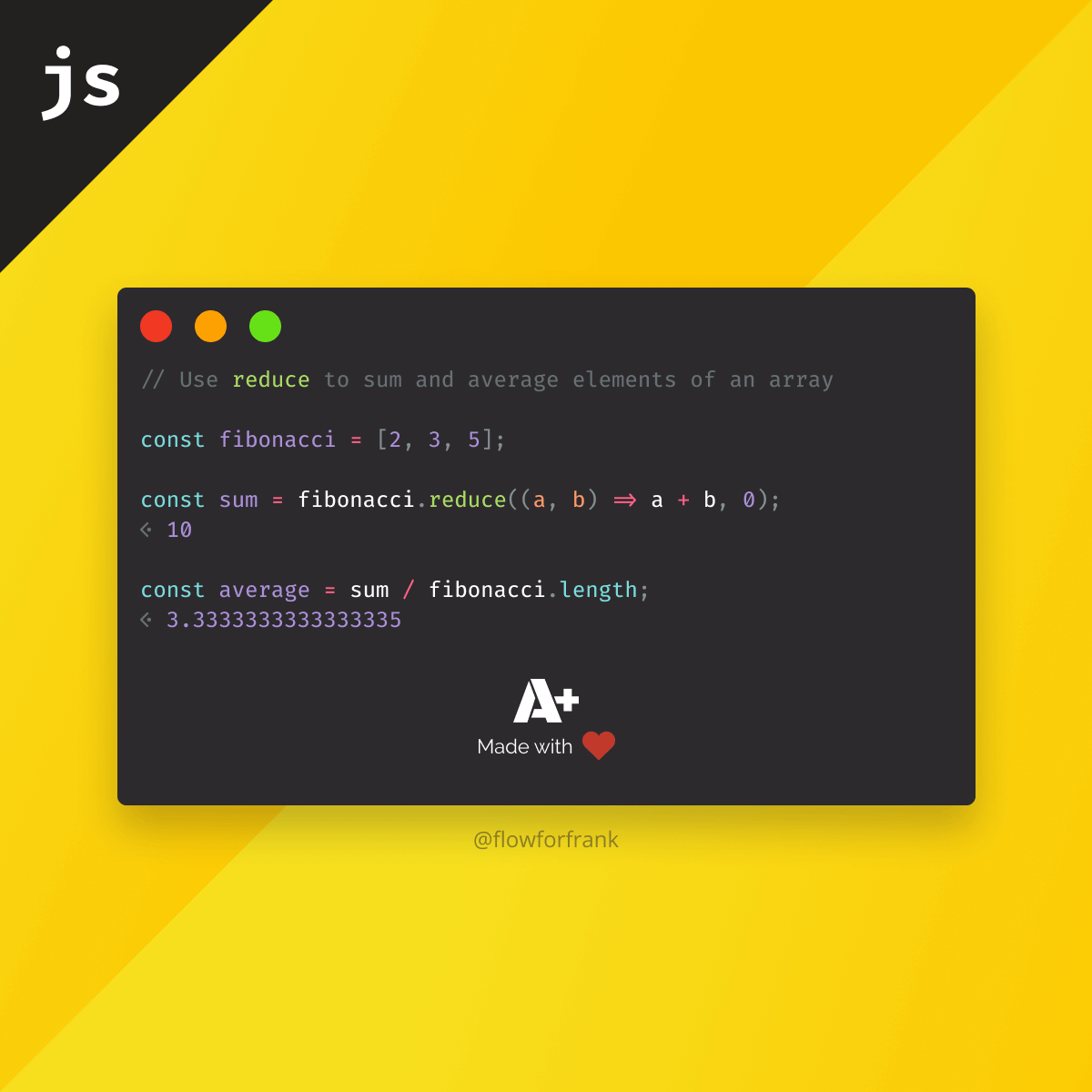
Resources:
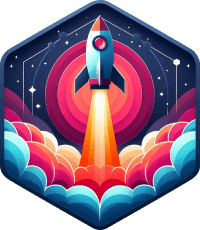
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: