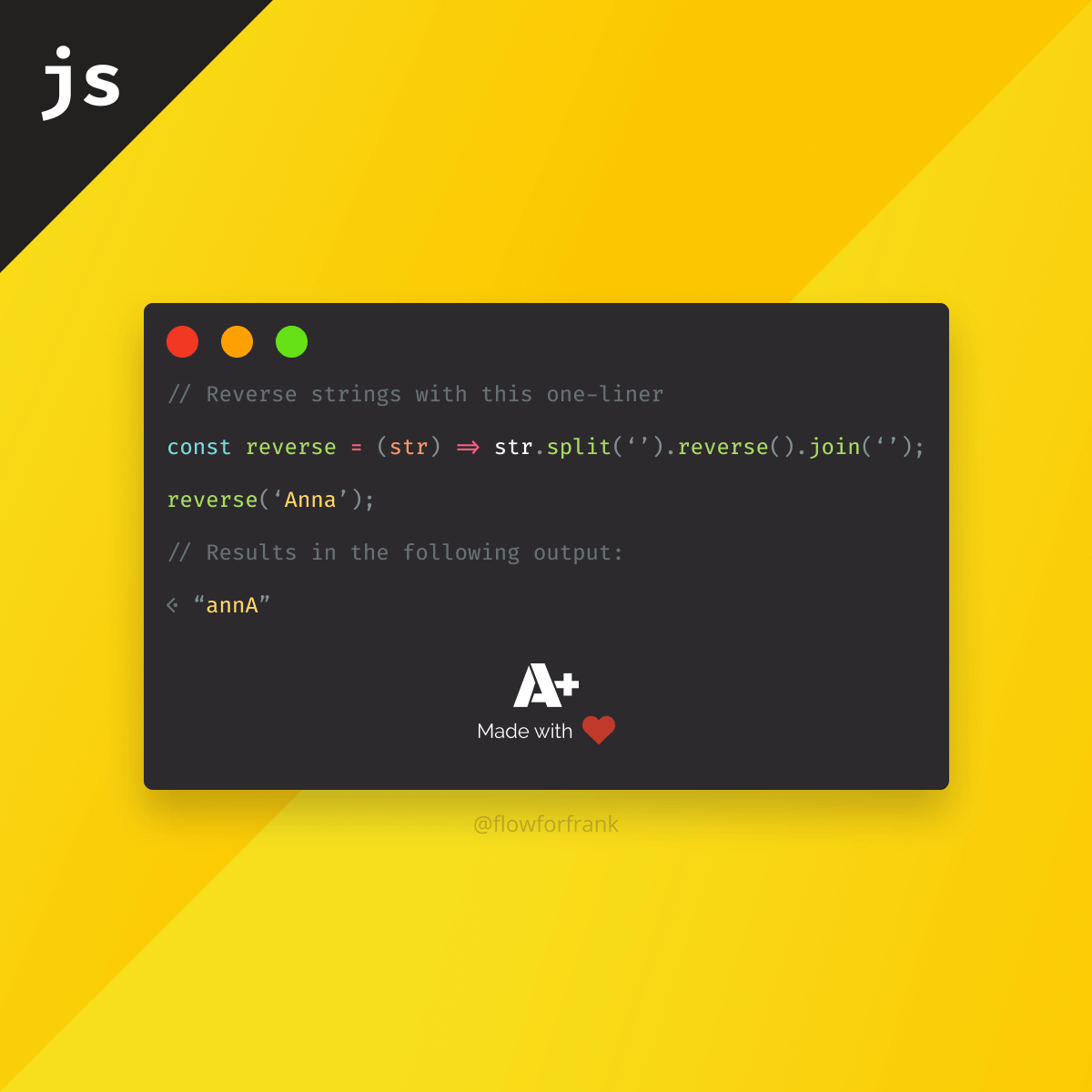
How to Reverse Strings in JavaScript
Want to reverse a string in JavaScript? You can do so with this one-liner:
// Reverse strings with this one-liner
const reverse = str => str.split('').reverse().join('');
// This will return 'annA'
reverse('Anna');
This works by using built in functions, which follows three steps:
- First, the passed string is split into idividual charachters. This results in an array
- The elements of the array is reversed, so the last item becomes the first one, and so on
- The elements of the array are joined together with an empty string, rebuilding the original string in reverse order
But what happens if you try this solution with emojis?
// Using the reverse function on emojis
reverse('πΆπ¨π΄');
// This will result in:
"οΏ½π¨π΄οΏ½"
This is because emojis are multibyte characters, meaning they are made up of multiple bytes.
// If you convert "s" into a byte, you'll get:
73
// If you convert "π¨" into a byte, you'll get:
f0 9f 91 a8
The solution works fine for UTF-8, but not for UTF-16 or UTF-32, which uses different numbers of bytes to represent a character. These multibyte characters cannot be reversed like a UTF-8 string. Instead, you need to split them up, like they were an array:
// Reverse strings with this one-liner
const reverse = str => [...str].reverse().join('');
// This will now return the expected output 'πΆπ¨π΄'
reverse('π΄π¨πΆ');
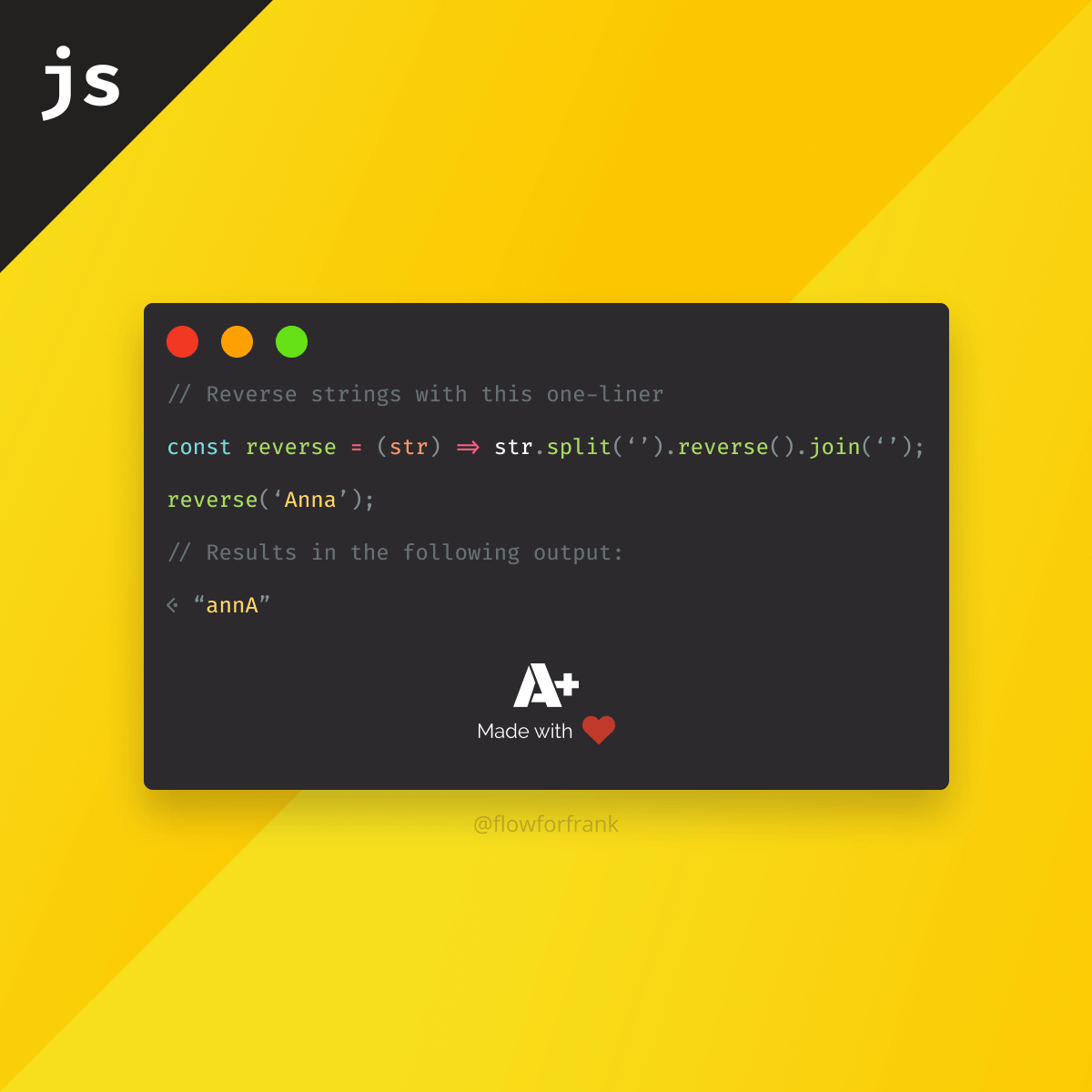
Resources:
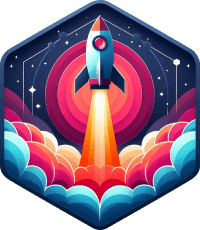
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: