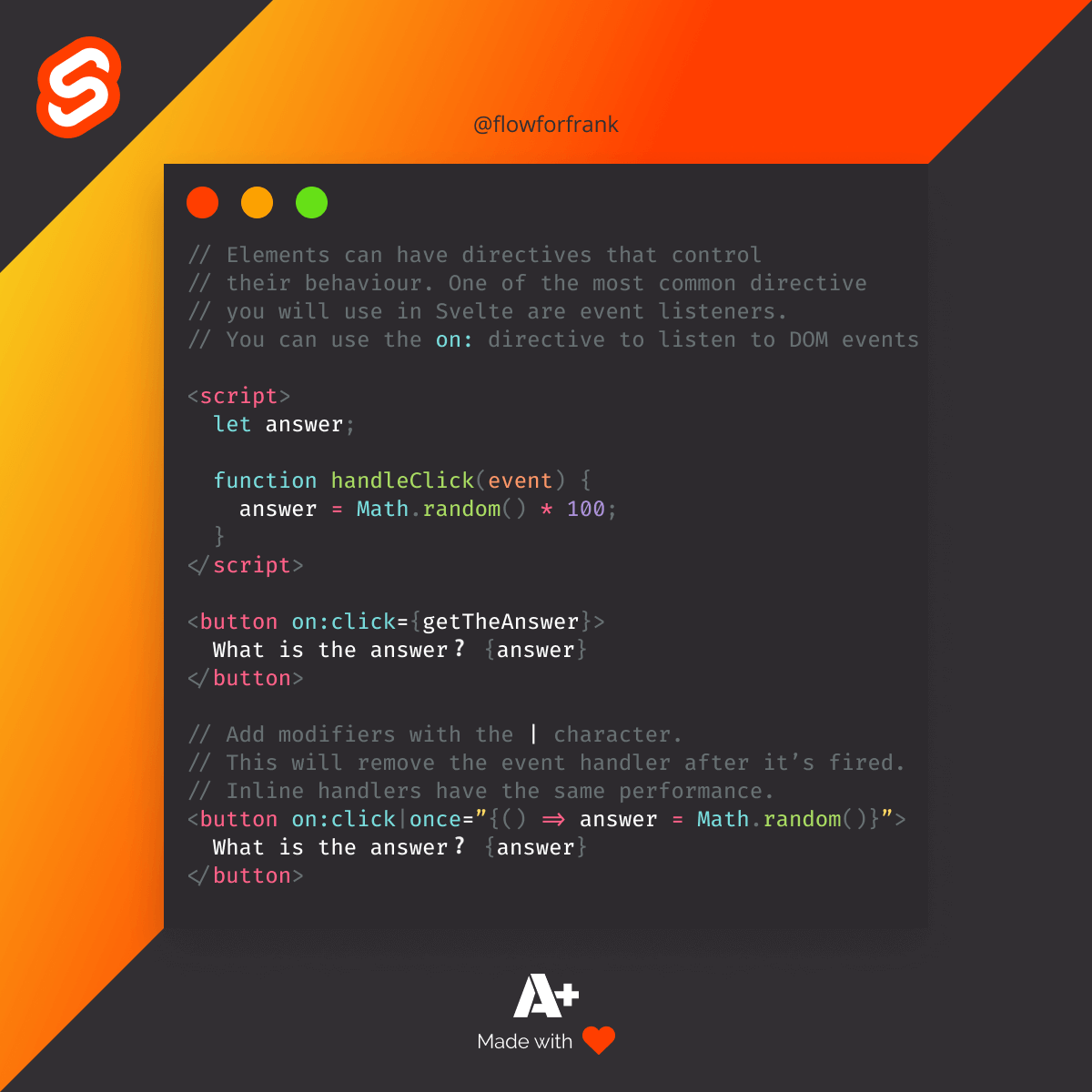
How to Use Event Listeners in Svelte
You can use the on:<eventName>
directive in Svelte to listen to DOM events:
<script>
let answer;
function handleClick(event) {
answer = Math.random() * 100;
}
</script>
<button on:click={getTheAnswer}>
What is the answerβ {answer}
</button>
You can also fire events only once, by adding modifiers with a pipe:
<!-- This will fire once, then the event listener will be removed -->
<button on:click|once="{() => answer = Math.random()}">
What is the answerβ {answer}
</button>
Apart from once
, you can also specify the following modifiers:
preventDefault
stopPropagation
passive
- this is tend to improve scrolling performance for scroll eventscapture
- use event capturing instead of event bubblingself
- only trigger the event if the target is the element itself.
You may also notice that I've declared an inline function. Unlike in React, you don't have to worry about performance if you define an inline handler.
You can also have the same event listener attached multiple times to an element:
<button on:click={getTheAnswer} on:click={trackingClickEvent}>
What is the answerβ {answer}
</button>
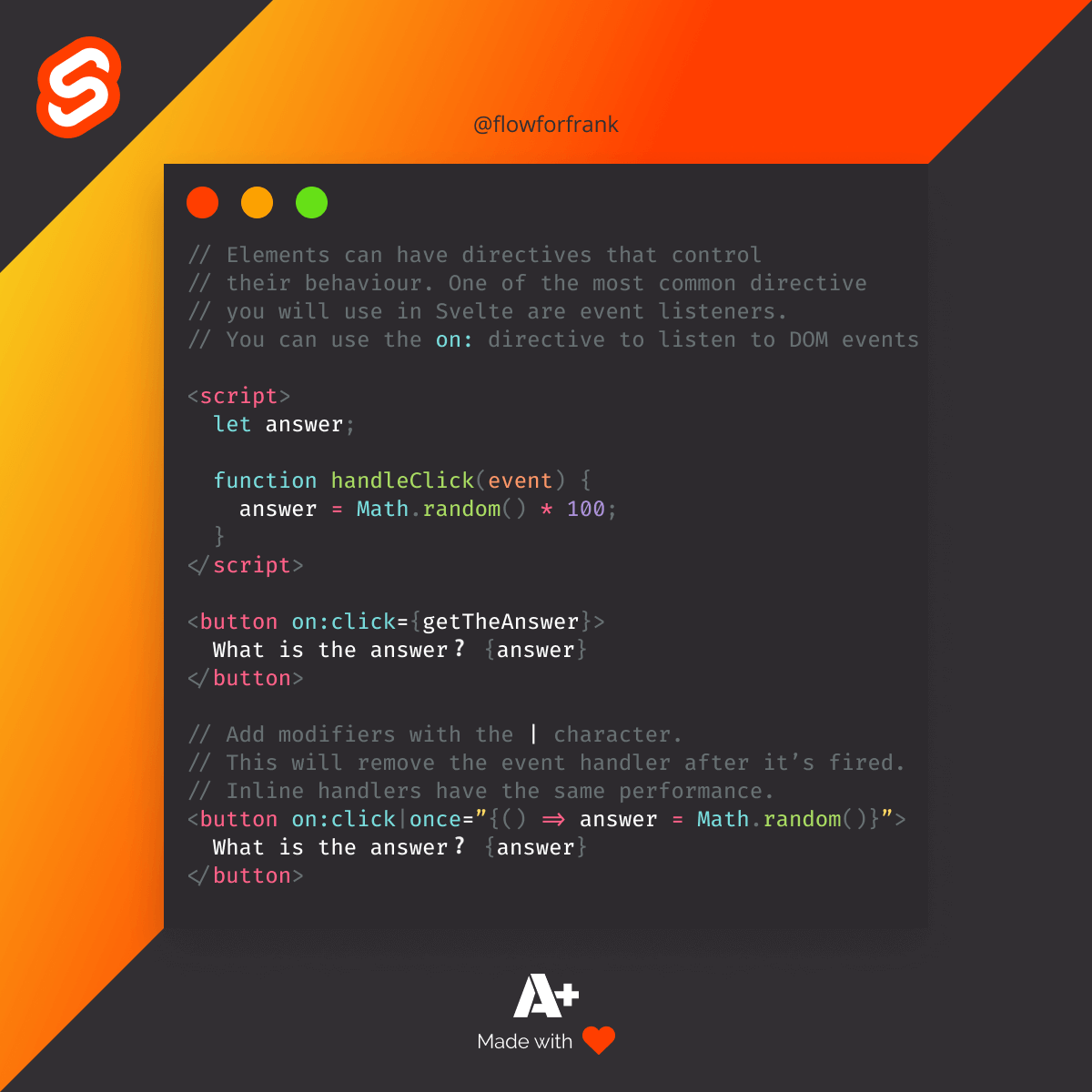
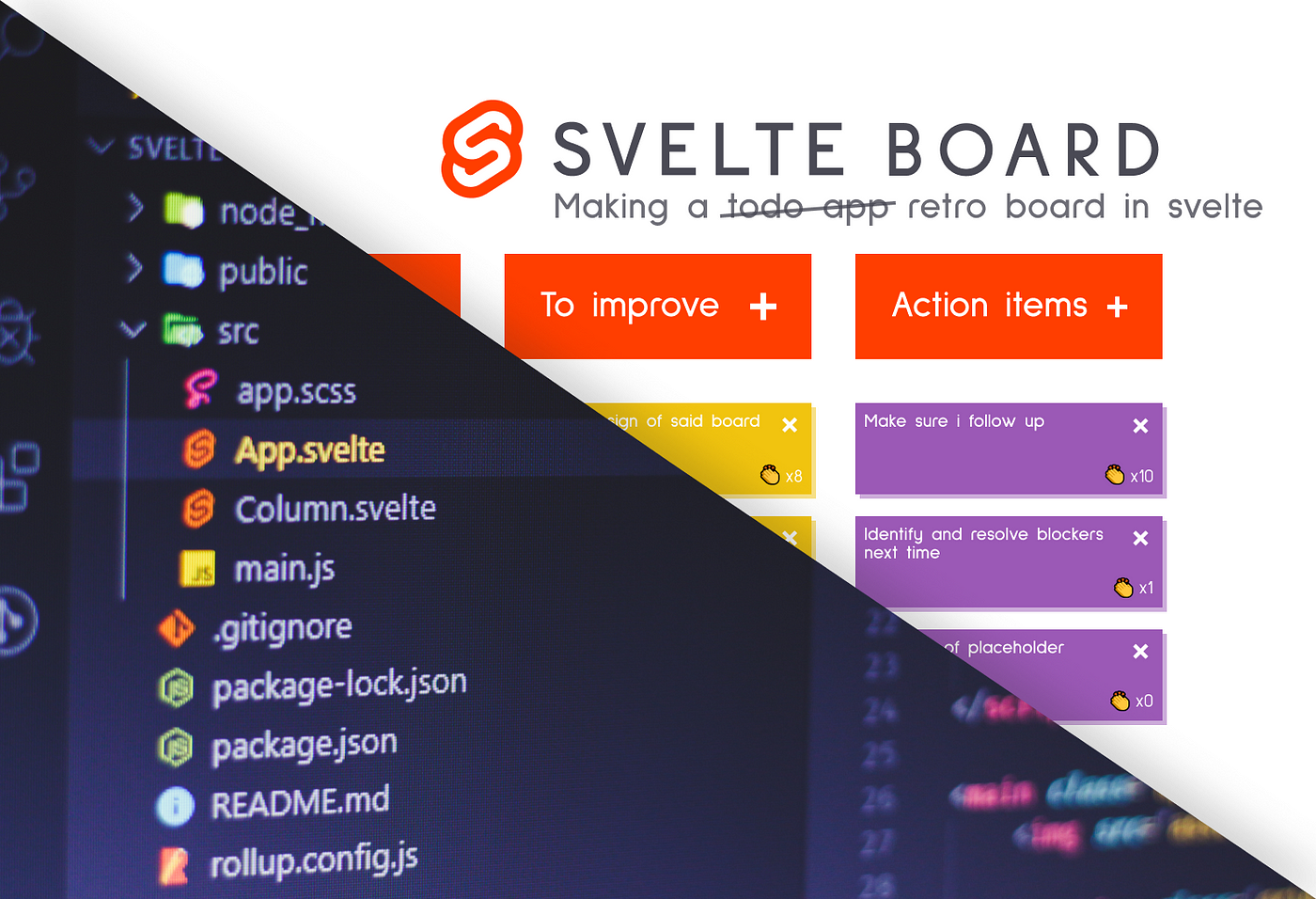
Resources:
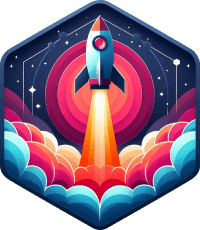
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: