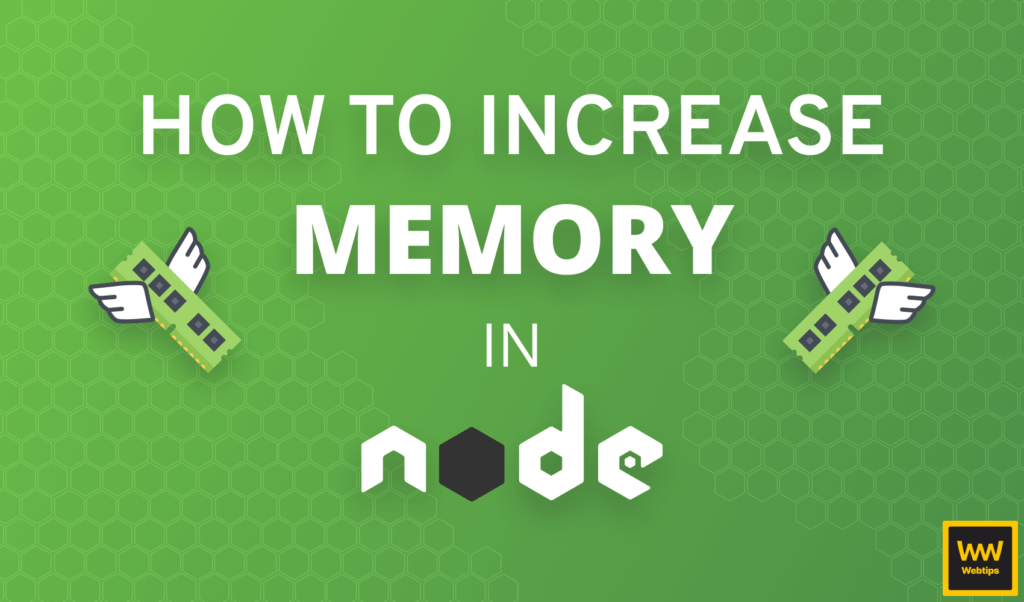
How to Quickly Increase the Memory Limit in Node.js
Running Node.js applications come with a default memory limit. This means that memory-intensive applications can run out of memory by allocating more than the available resource. This results in an error message similar to the one below:
FATAL ERROR: invalid array length Allocation failed - JavaScript heap out of memory
How to Increase the Memory Limit
Luckily, the fix is fairly simple. Use the --max-old-space-size
flag when running a Node.js application to increase the available memory heap.
node --max-old-space-size=1024 app.js # Increase limit to 1GB
node --max-old-space-size=2048 app.js # Increase limit to 2GB
node --max-old-space-size=3072 app.js # Increase limit to 3GB
node --max-old-space-size=4096 app.js # Increase limit to 4GB
node --max-old-space-size=5120 app.js # Increase limit to 5GB
Note that the flag needs to come before calling the file with the node
command.
Of course, keep in mind that you won't be able to increase the memory limit above the physical memory of the machine you are running the Node.js application on. Nonetheless, using the --max-old-space-size
flag is a quick way to fix memory errors in Node.
How to Test Out the Memory Limit
To quickly reach the memory limit and reproduce the error, we can use a while
loop without an exit condition, like the one below:
const array = [];
while (true) {
for (let i = 0; i < 100000; i++) {
array.push(i);
}
console.log(process.memoryUsage());
}
In this code example, we just keep pushing elements to an array that allocates memory, but we never free up that memory, which results in an out-of-memory error. During the execution of your Node.js application, you can use the process.memoryUsage
method in order to get a clear picture of how much memory is used by your Node.js app.
{
rss: 19030016,
heapTotal: 4468736,
heapUsed: 2579432,
external: 855863,
arrayBuffers: 9898
}
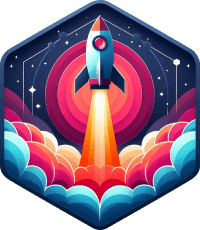
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: