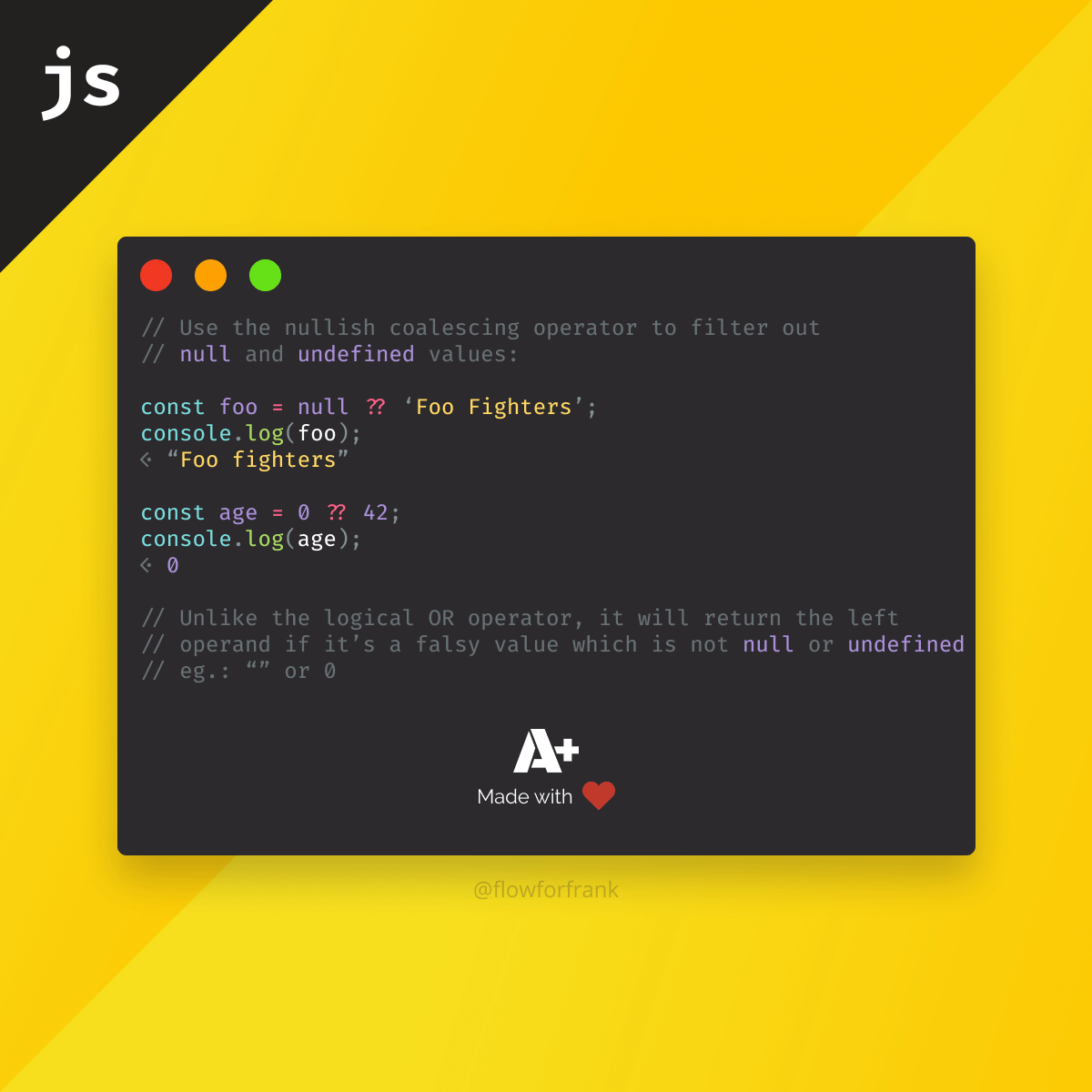
What is the Nullish Coalescing Operator in JavaScript?
The nullish coalescing operator (??
) in JavaScript works similar to the logical OR operator. With logical OR, you can use a short circuit evaluation to provide default values:
// Short circuit (set default values) with logical OR
const fileName = this.fileName || 'πΆ';
// If this.fileName is undefined, πΆ will be used as a fallback value
However, by using the nullish coalescing operator, you can return the left hand side of the operand if it's falsy, but not null
or undefined
:
// Use the nullish coalescing operator to filter out null and undefined values:
const foo = null ?? 'Foo Fighters';
console.log(foo); // This will return βFoo fightersβ
const age = 0 ?? 42;
console.log(age); // This will return 0
// Unlike the logical OR operator, it will return the left
// operand if itβs a falsy value which is not null or undefined
// eg.: ββ or 0
This is not the case for logical OR. It will return the right-hand side, if the value is falsy. (Which means 0
or ""
are also included)
Keep in mind, this is only supported for the latest browsers. You can check the support table at caniuse.com
If you are using babel, you can use the @babel/plugin-proposal-nullish-coalescing-operator plugin for it.
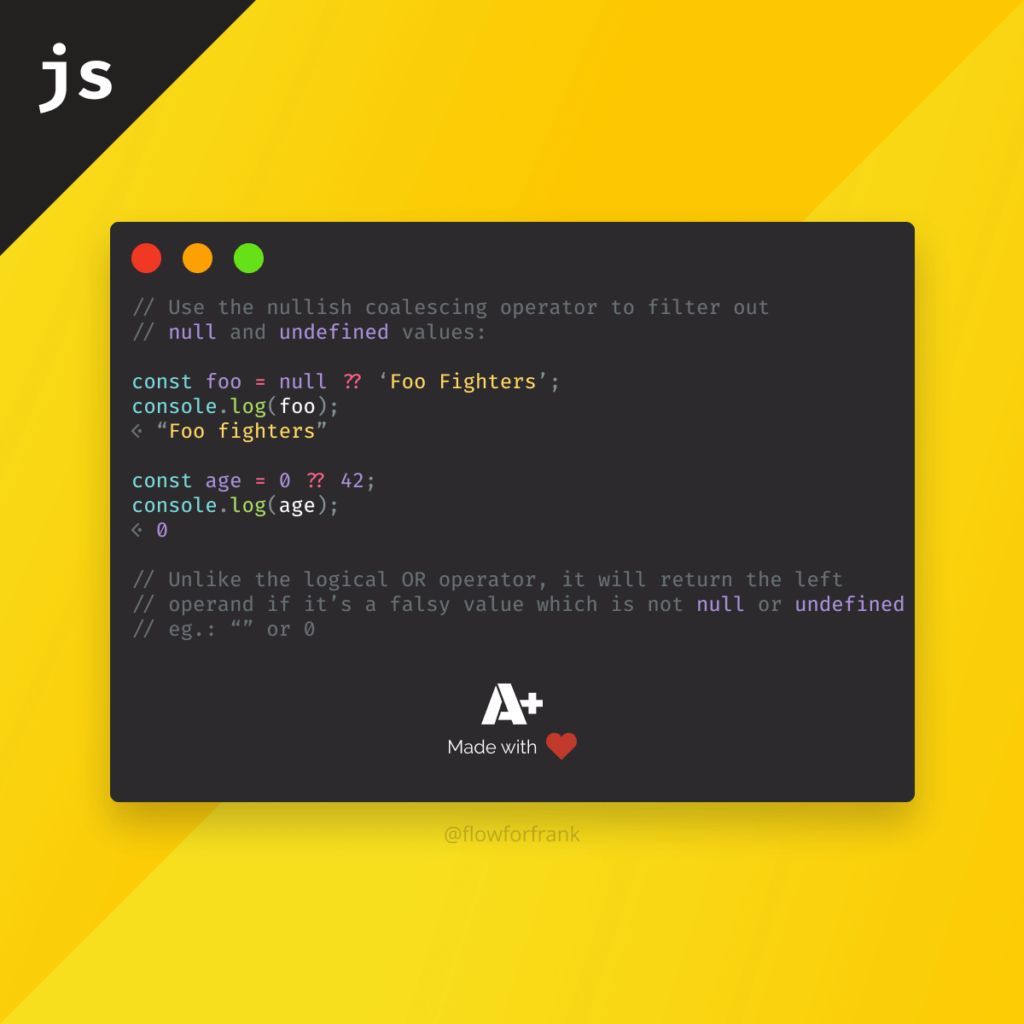
Resource
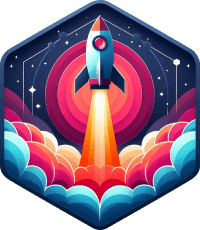
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: