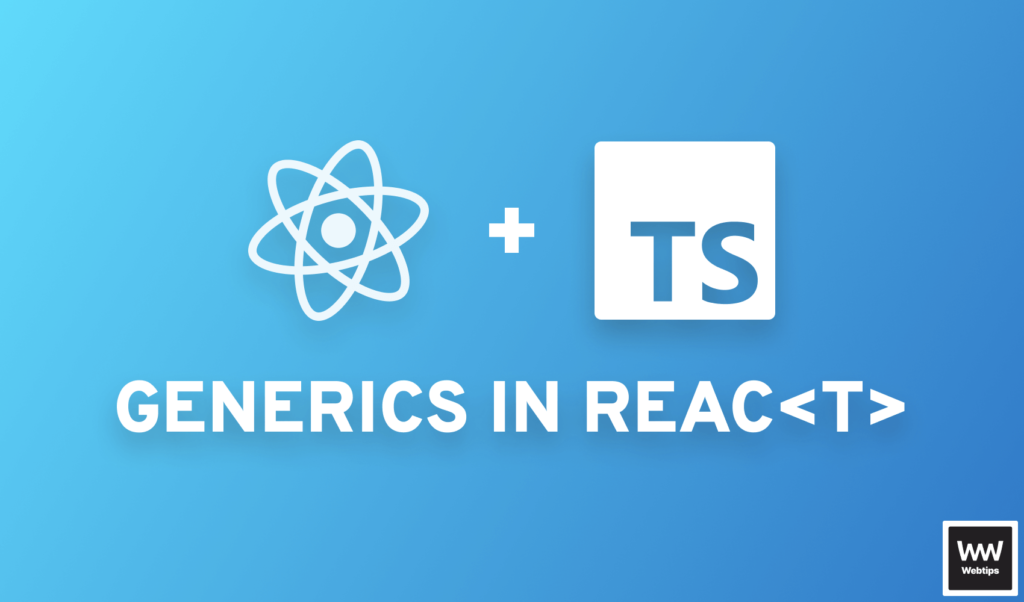
TypeScript
TypeScript is a statically typed superset of JavaScript that compiles to plain JavaScript. It adds optional static typing, classes, interfaces, and other features to JavaScript, making it a strongly typed language that can catch type-related issues early on.
With almost 40 million weekly downloads from NPM, TypeScript is one of the most popular packages for frontend developers and one of the most widely adopted flavors of JavaScript.
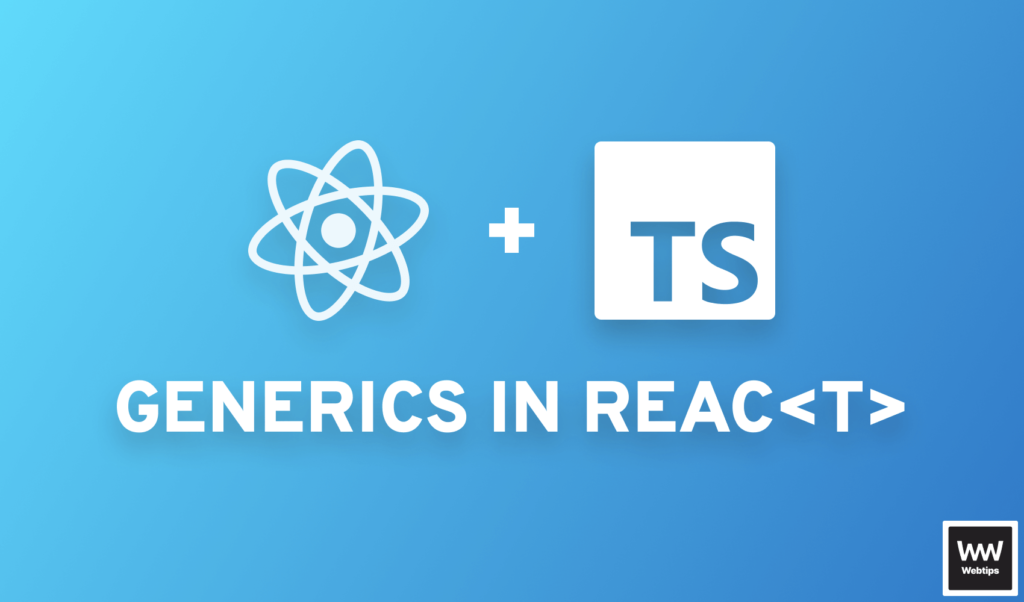
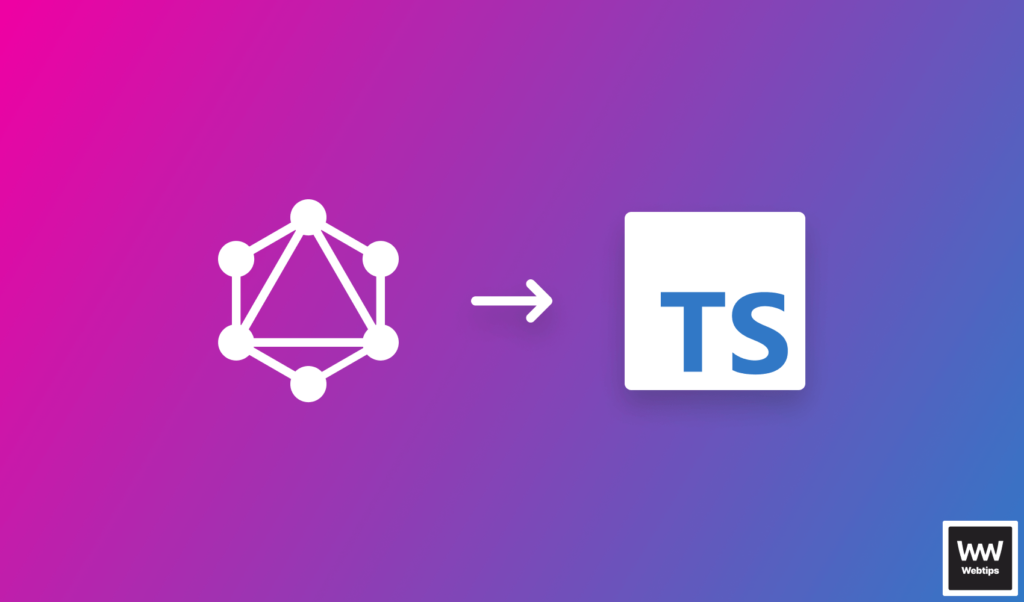
Why Should I Learn TypeScript?#
Still, the question remains: why should I care about learning TypeScript? What's in it for me? Here are five reasons to help you decide whether you should invest your time in learning TypeScript:
- Compatibility: TypeScript is a superset of JavaScript, which means that any valid JavaScript code is also valid TypeScript code. This makes it easy to adopt TypeScript gradually and incrementally, without having to rewrite all your code at once.
- Type safety: As TypeScript is a strongly typed language, it helps with catching errors early on in the development cycle. This can save a lot of time and effort in debugging later on.
- Improved productivity: TypeScript's static typing also helps IDEs provide better code completion, refactoring tools, and other useful features. This can speed up your development process and improve your productivity.
- Better maintenance: With better types comes better maintenance. Type checking makes code more maintainable over time by providing clear documentation of types and function signatures. This can make it easier to refactor code without worrying about introducing new bugs to the system.
- Better error reporting: TypeScript's error reporting is often more helpful and detailed than plain JavaScript. This can help you quickly identify and fix issues in your code.
Show Me How TypeScript Works#
Let's take a look at a simple example to see how TypeScript works. In the following code, we have a clamp function that takes in a number, min, and max values, and returns a number:
// JavaScript implementation
const clamp = (num, min, max) => Math.min(Math.max(num, min), max);
// TypeScript implementation
const clamp = (
num: number,
min: number,
max: number
): number => Math.min(Math.max(num, min), max);
To turn this function from JavaScript to TypeScript, we simply added types to the parameters and the return value using the number
type. Types can be assigned using a colon, followed by the type, such as number
, string
, or boolean
.
Where Should I Start?#
If you are new to TypeScript, you should start with the basics. First, you need to familiarize yourself with the syntax and the different types that TypeScript offers.
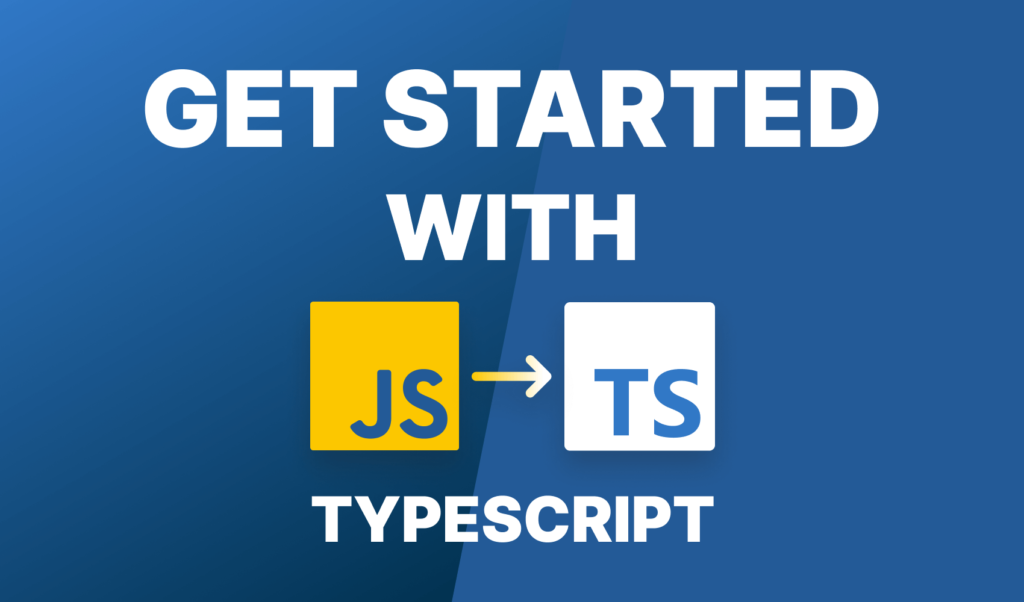
Working with function types, interfaces, enums, and type conversions should also be in your toolbelt. The official documentation of TypeScript can also guide you with full of examples.
Once you are familiar with the basics and you are comfortable building statically typed applications, you can start exploring more advanced concepts, such as generics or working with classes.
If you would like to learn more about TypeScript, you can find all of our latest tutorials below. Use the filters in the sidebar if you want to narrow down the results. Additionally, you can filter for TypeScript-related tutorials on our search page. I wish you good luck with learning TypeScript!
Keep on coding! π¨βπ»
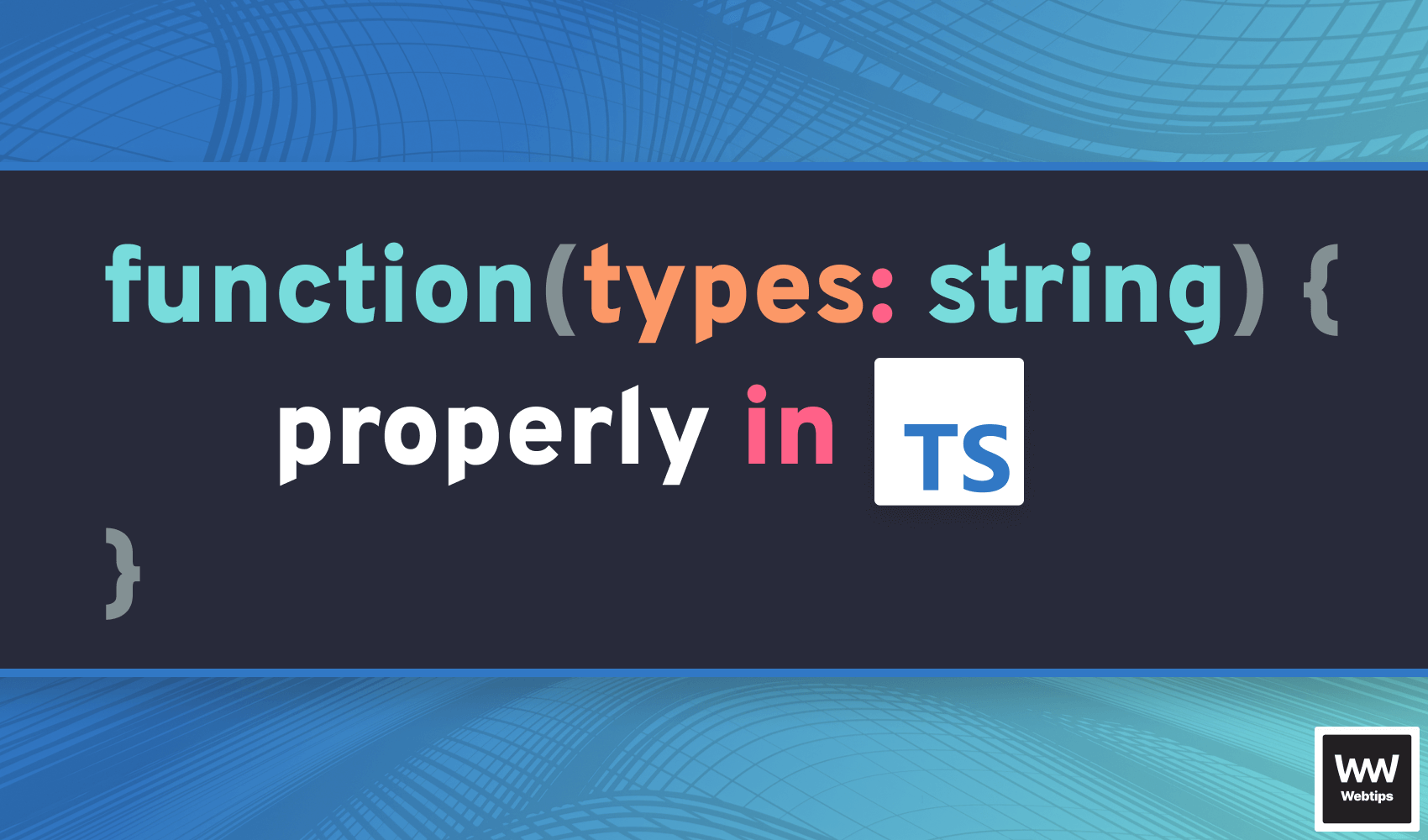
How to Type Functions in TypeScript
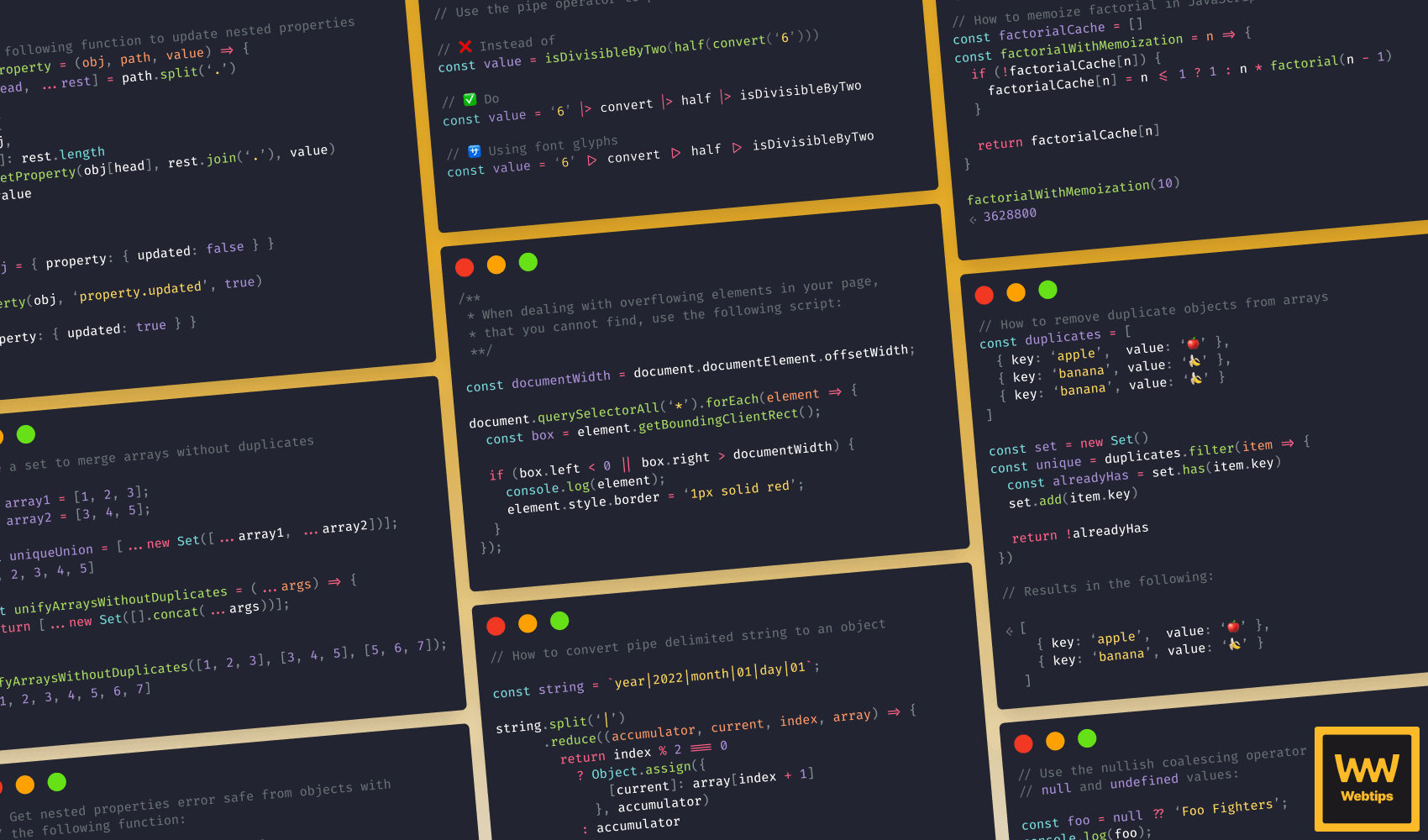
Two Ways to Get Enum Keys by Values in TypeScript
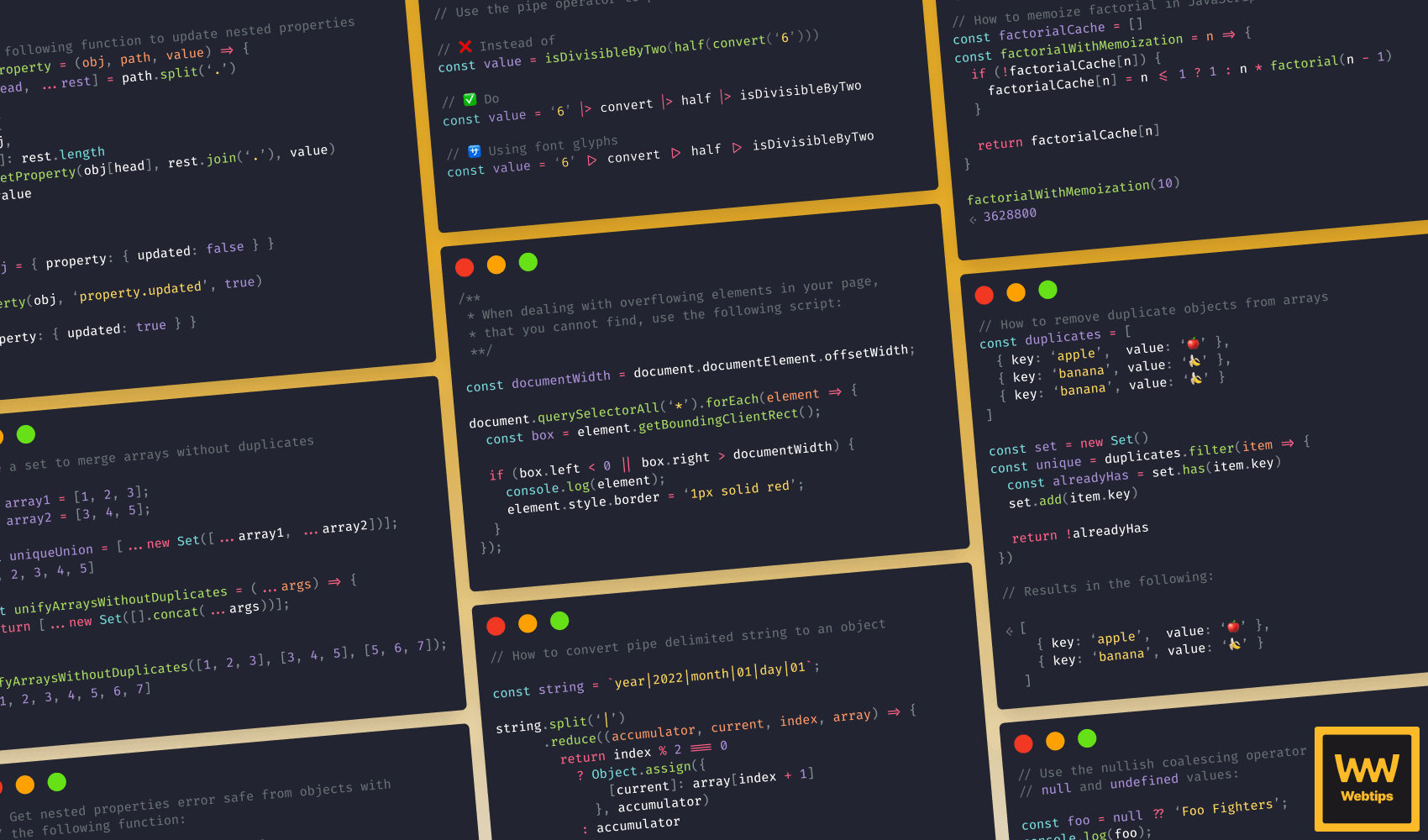
How to Extend Multiple Classes in TypeScript With Mixins
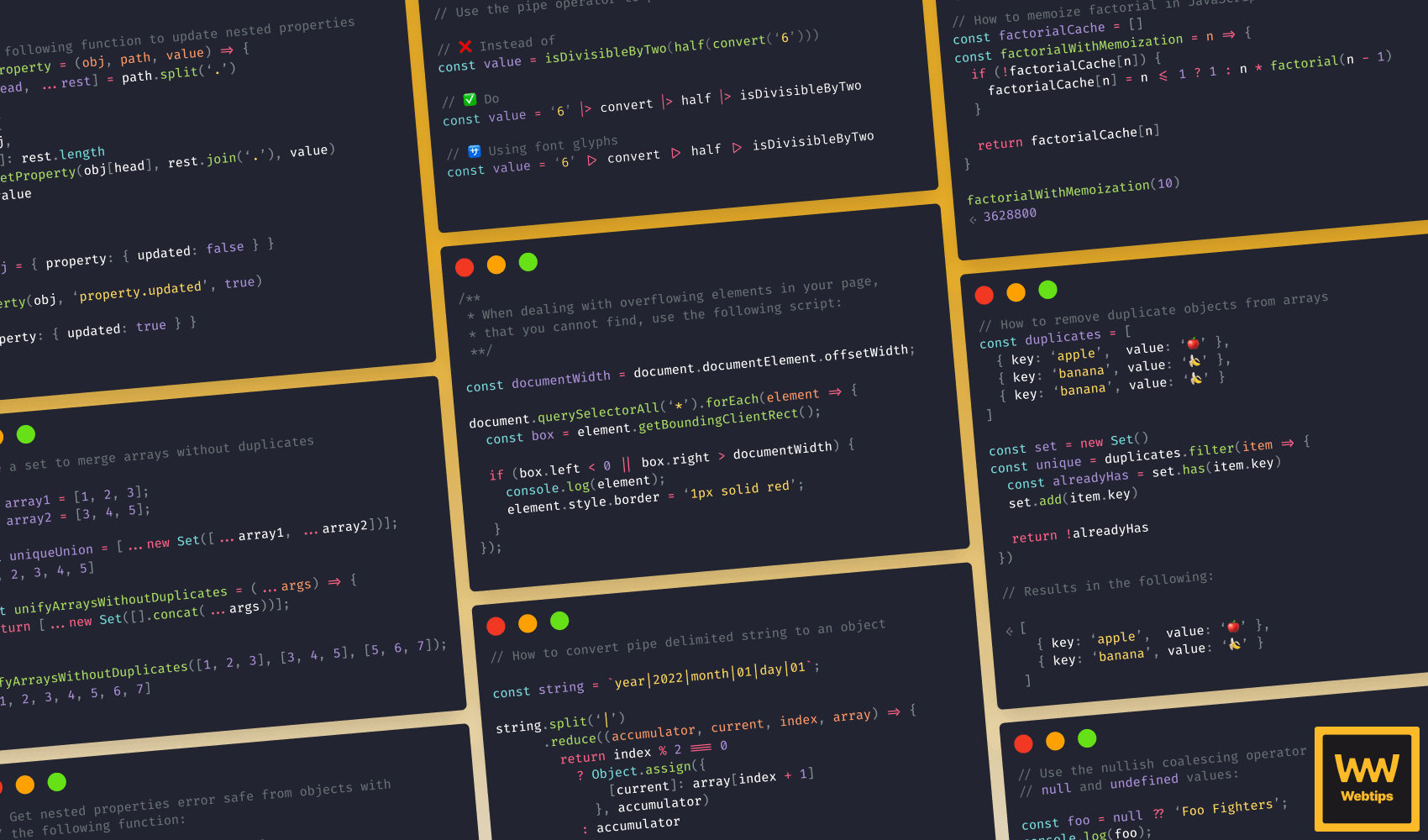
Two Ways to Force Types in TypeScript
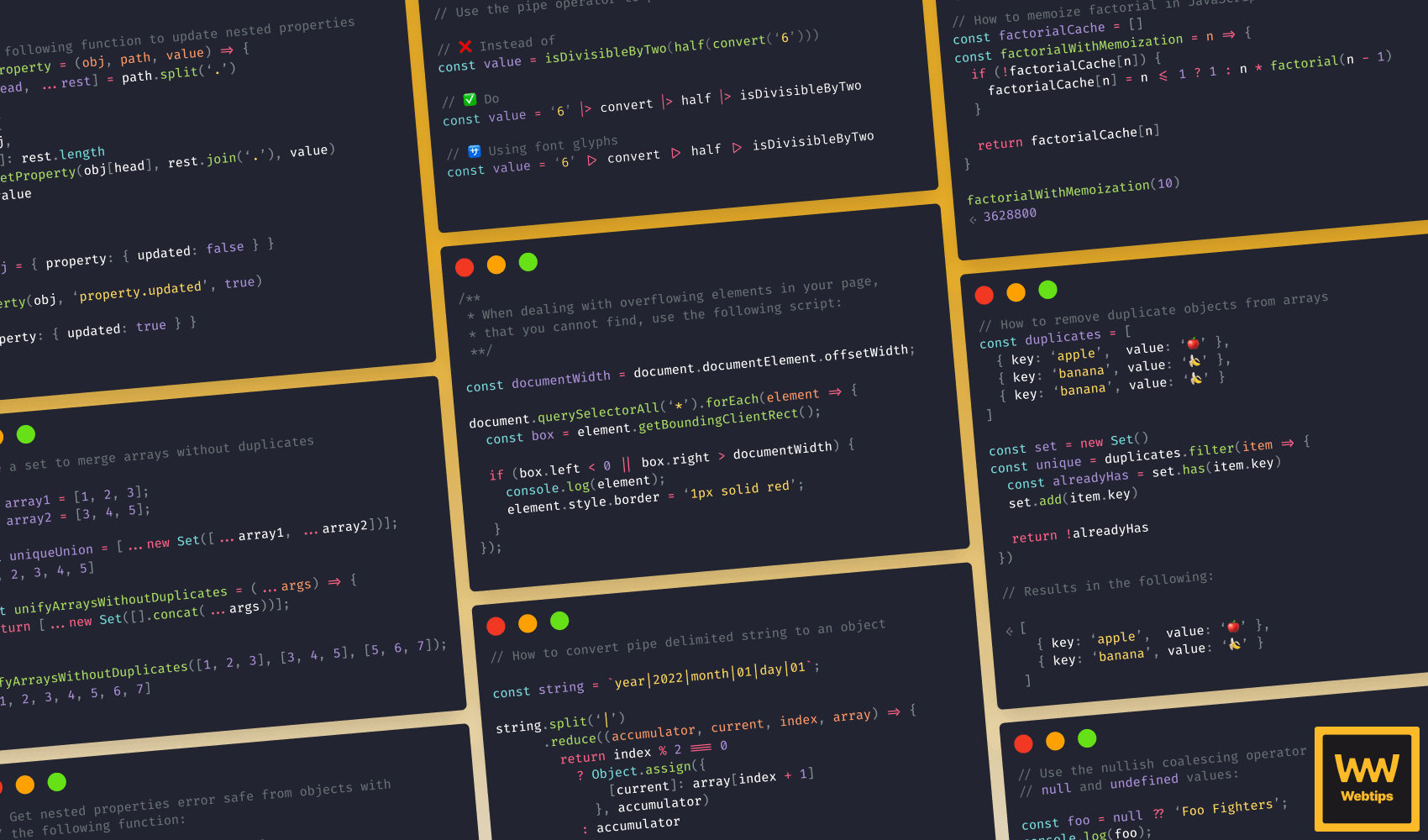
How to Easily Extend Interfaces in TypeScript
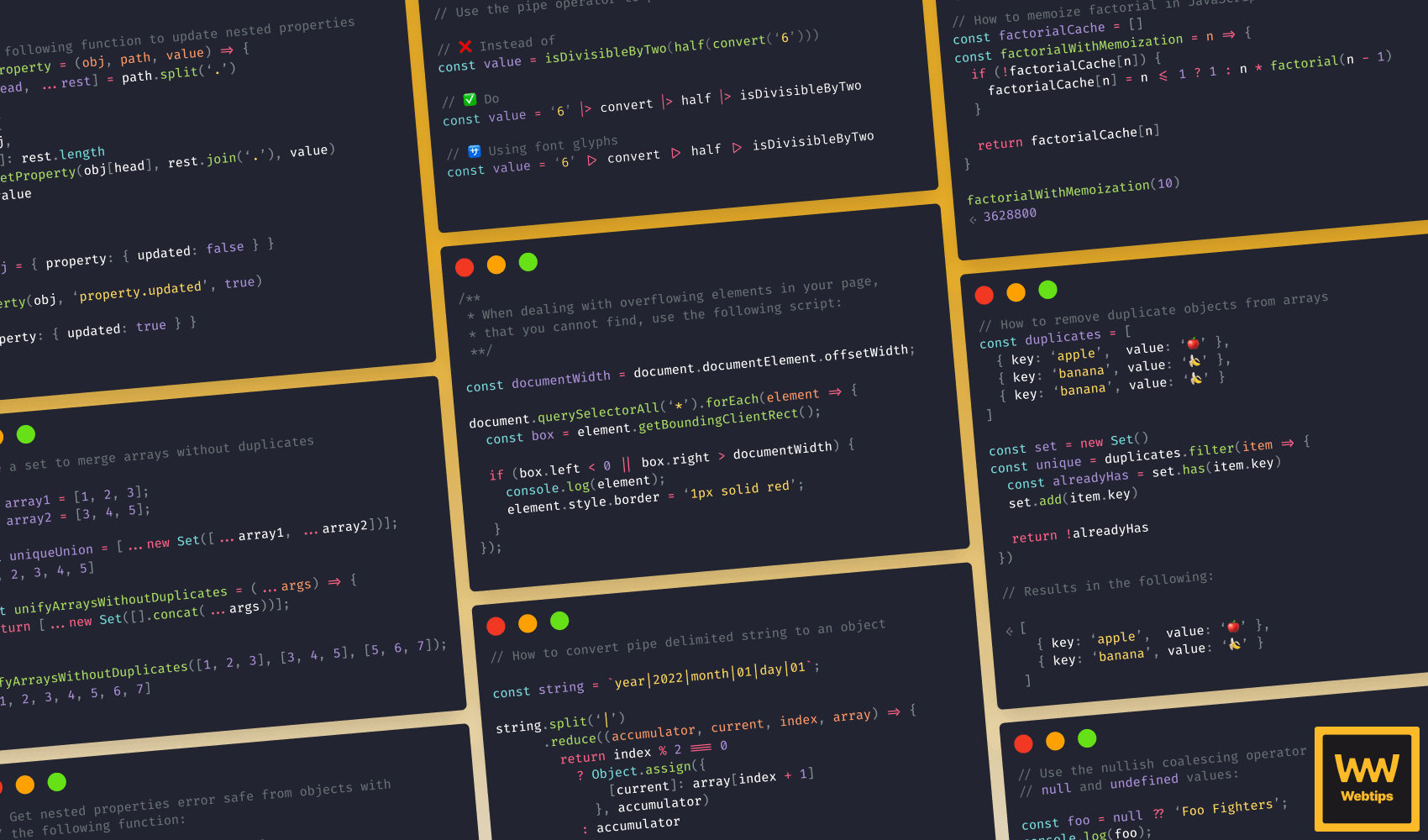
How to Correctly Check Undefined in TypeScript
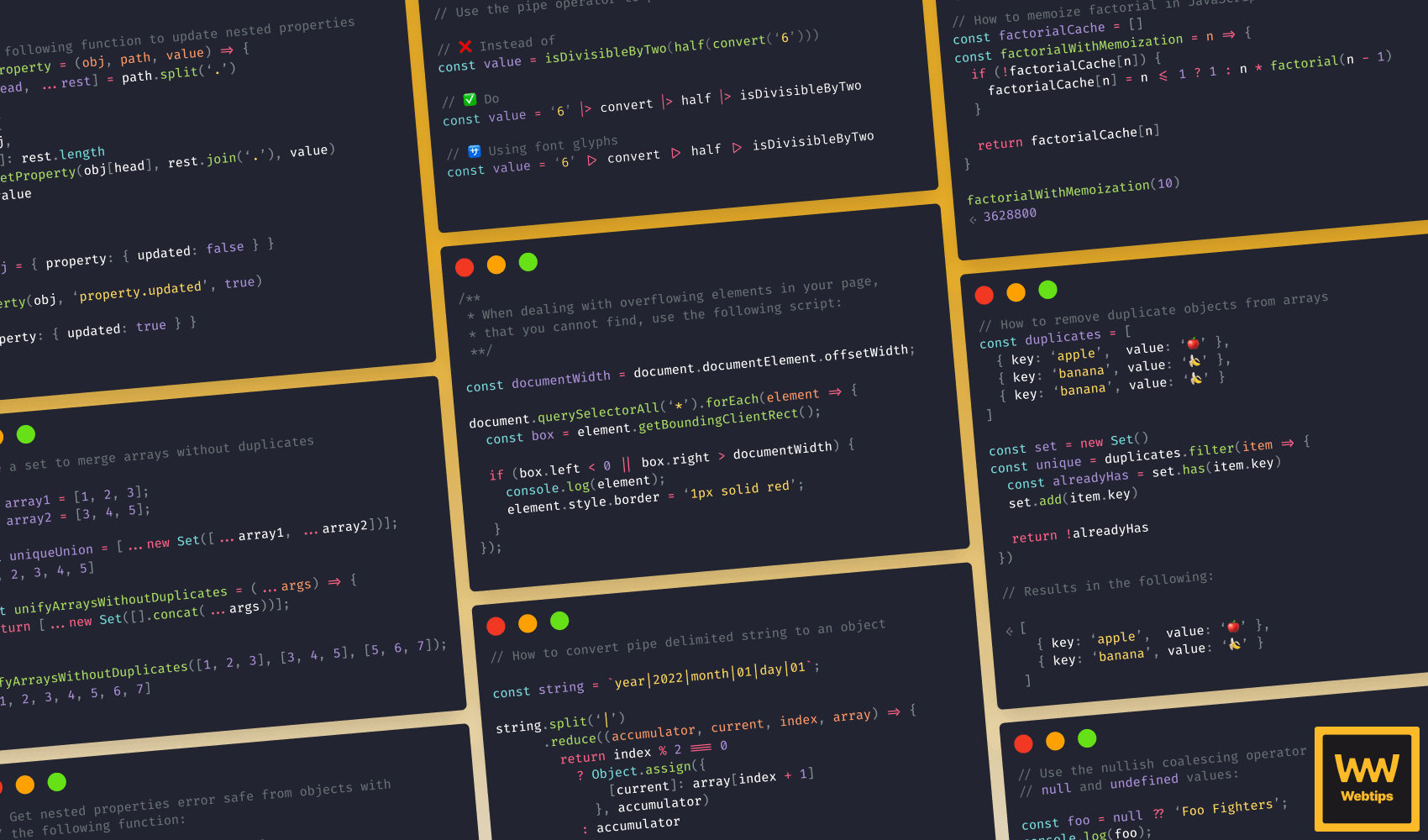
Extending Types in TypeScript The Right Way
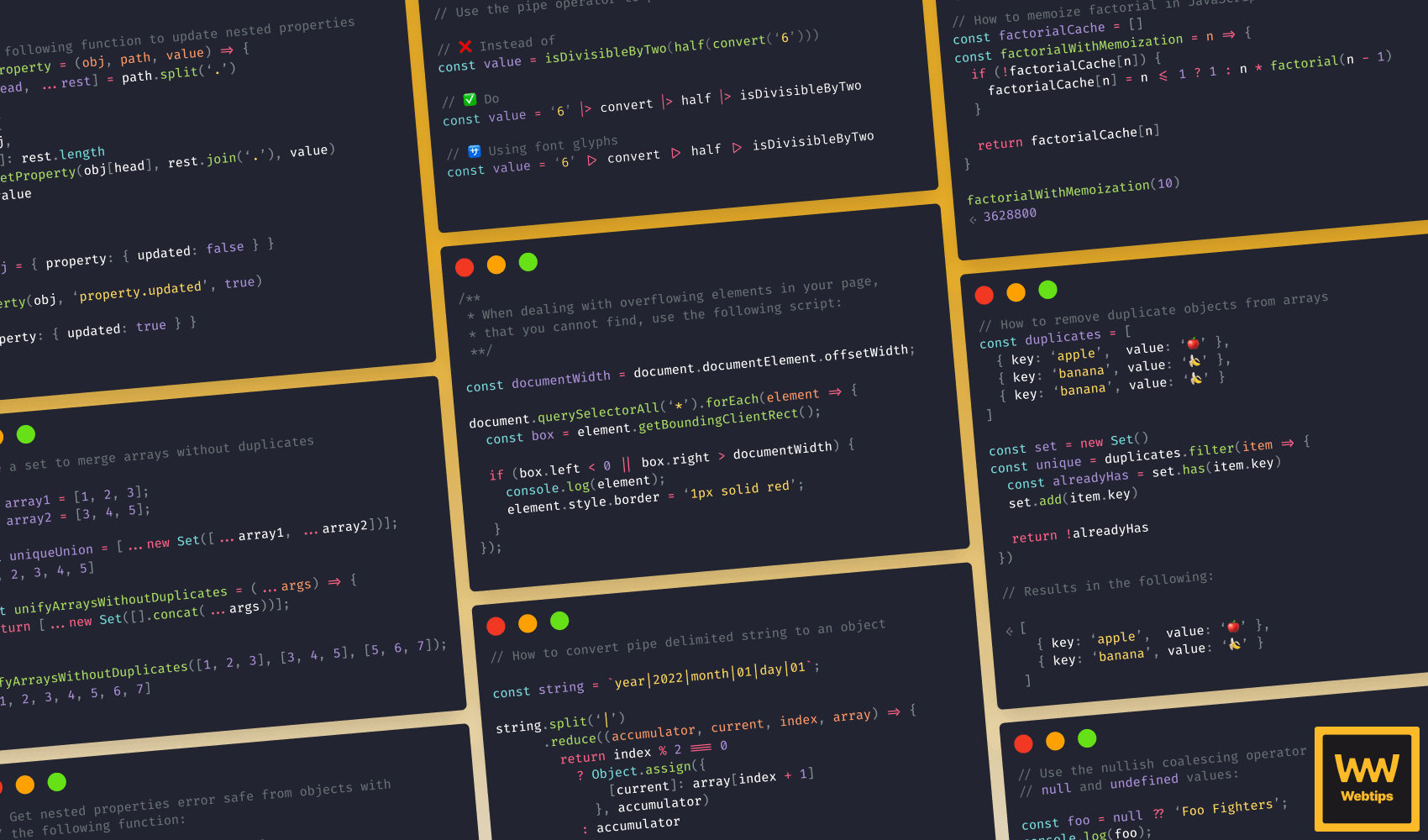
3+1 Ways to Declare Global Variables in TypeScript
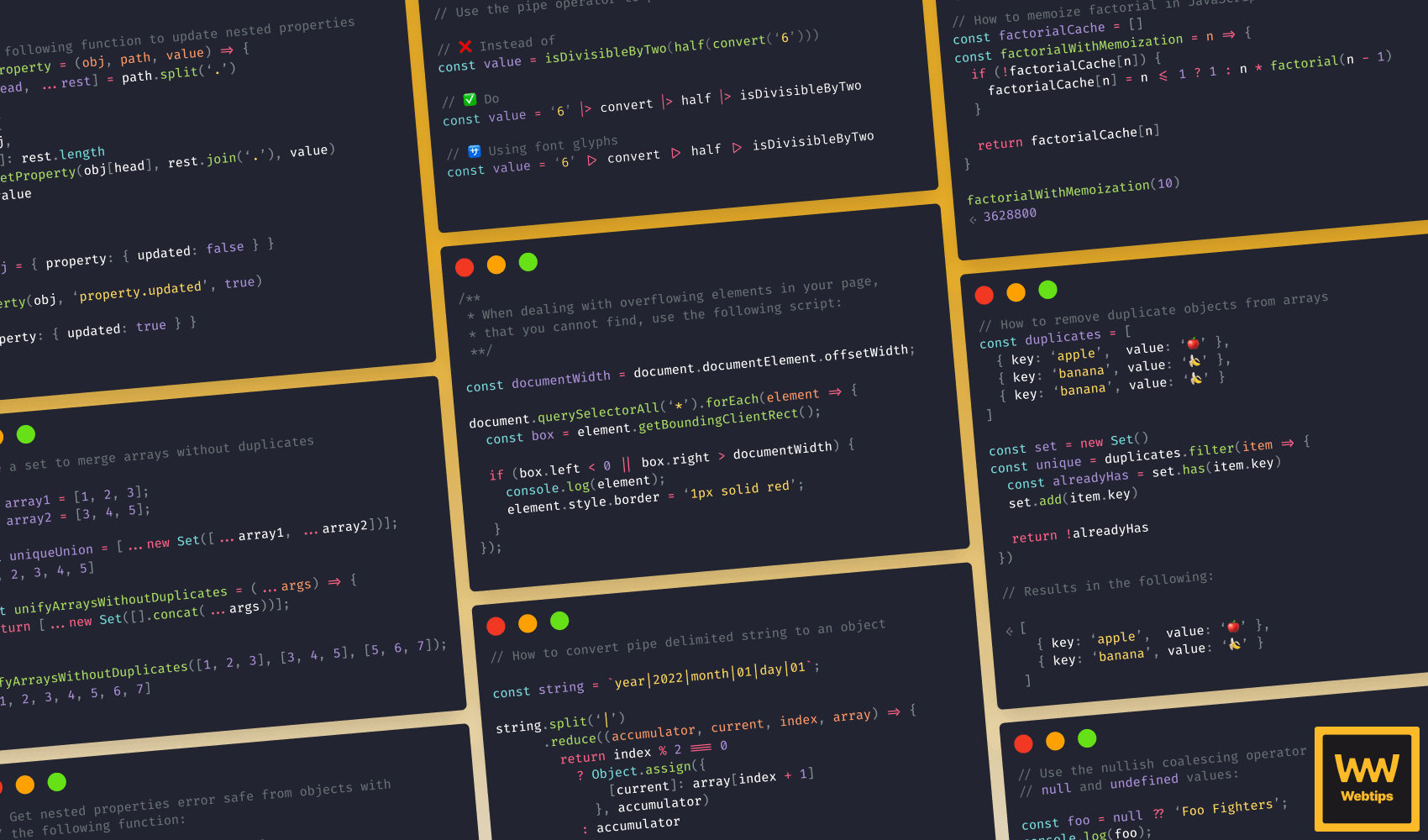
How to Check Variable Types in TypeScript
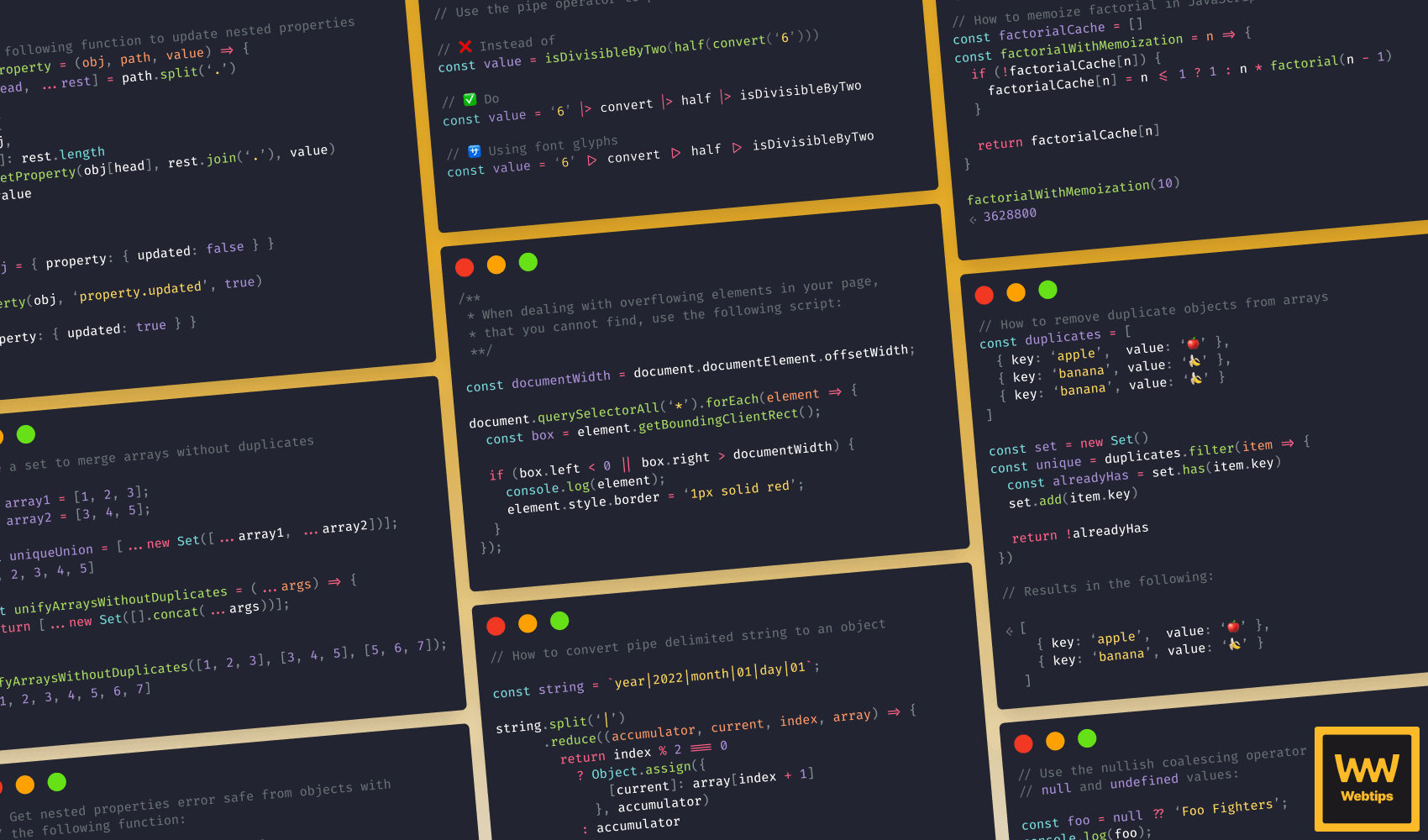
4 Ways to Convert Strings to Booleans in TypeScript
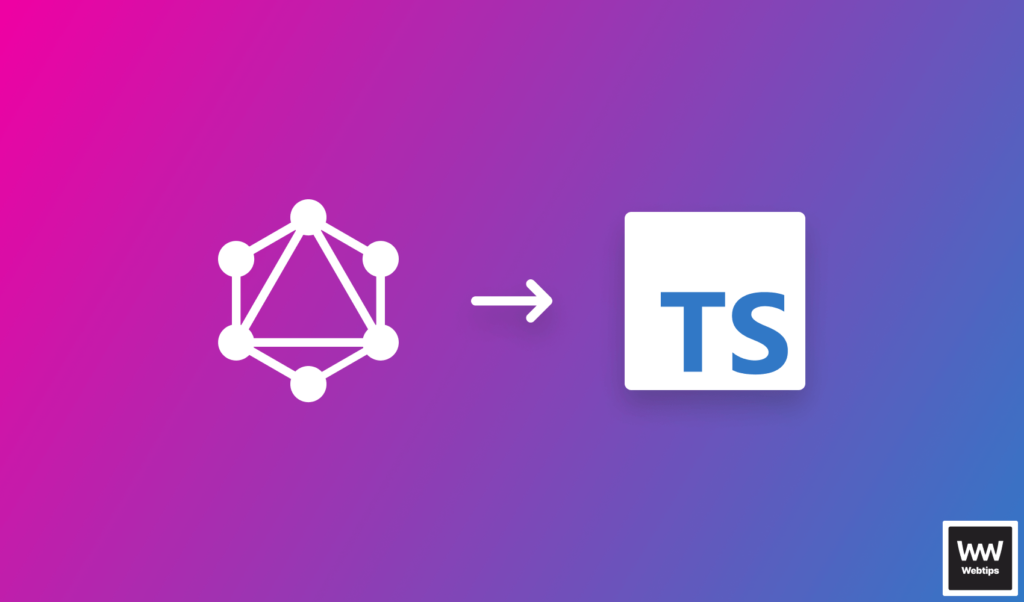
How to Generate TypeScript Types From GraphQL
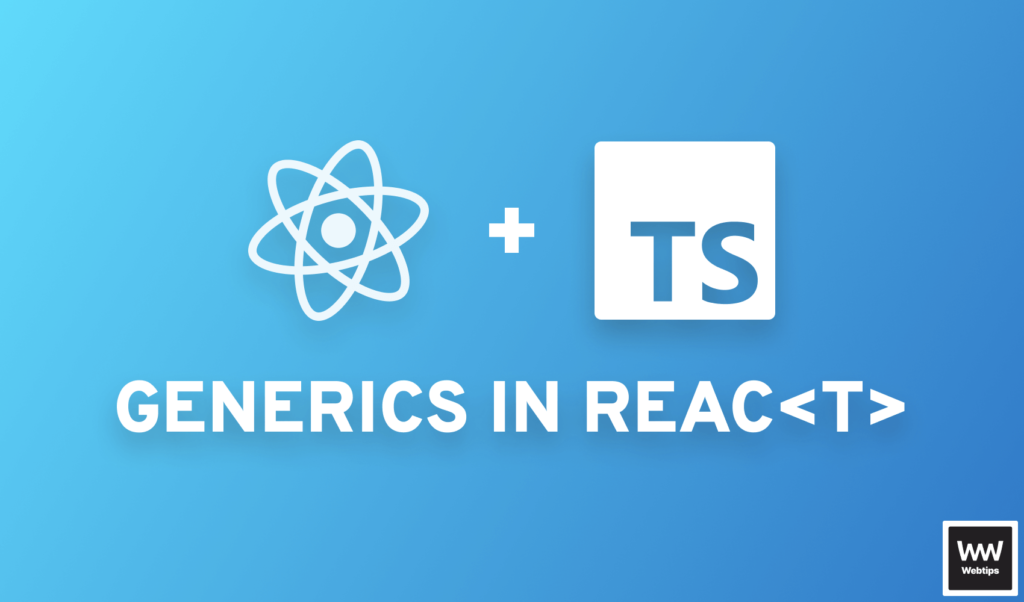
Generic React Components with TypeScript
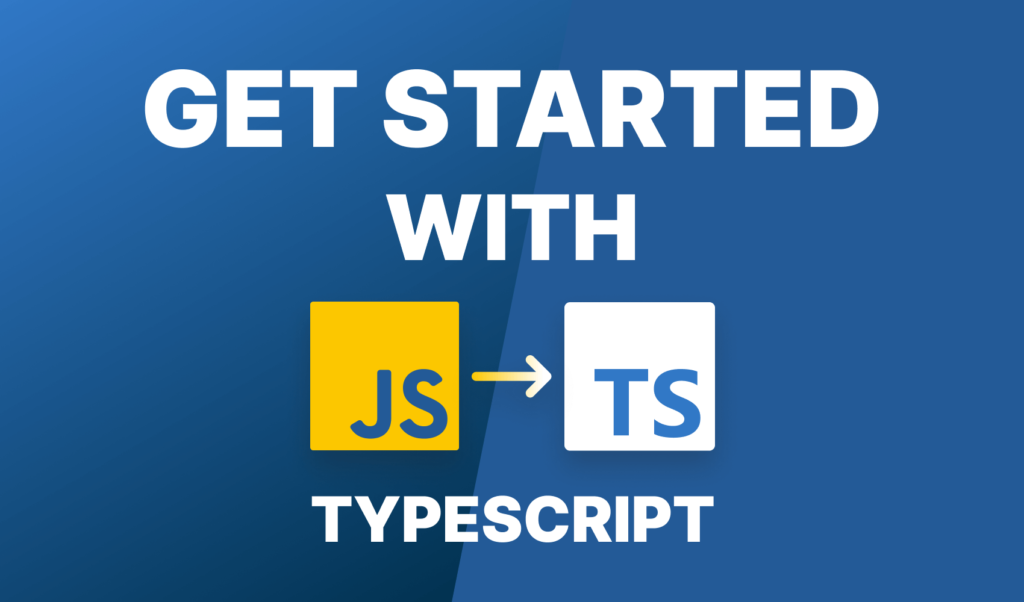
How to Get Started With TypeScript
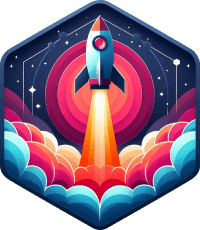
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
