How to Correctly Check Undefined in TypeScript
To check for undefined
values in TypeScript, you need to use the strict equal operator against the undefined
keyword.
type AuthorHandle = string | undefined
const twitter: AuthorHandle
// β This will throw an error (Cannot read properties of undefined)
const formattedHandle: string = twitter.toLowerCase()
// βοΈ Check if variable is not undefined
if (twitter !== undefined) {
const formattedHandle: string = twitter.toLowerCase()
}
In the above example, the if statement is called a type guard. It prevents toLowerCase
being called on undefined
. This way, TypeScript knows that the only other possible type is a string
type.
It is important to keep in mind that in case your variable can be null
, you want to check for a null
value too, as the above code example only caters to undefined
.
type AuthorHandle = string | undefined | null
const twitter: AuthorHandle
// β This will throw an error (Cannot read properties of undefined)
const formattedHandle: string = twitter.toLowerCase()
// β This will throw an error for null (Cannot read properties of null)
if (twitter !== undefined) {
const formattedHandle: string = twitter.toLowerCase()
}
// βοΈ Check if variable is not undefined OR null
if (twitter !== undefined && twitter !== null) {
const formattedHandle: string = twitter.toLowerCase()
}
// Note that null and undefined are not the same thing
undefined === null -> false
If you often have to work with types that can be either undefined
or null
, it is recommended to declare a generic often called Nullable
or Maybe
that can be reused throughout your application.
declare type Nullable<T> = T | undefined | null
// This will now be string | undefined | null
type AuthorHandle = Nullable<string>
Using Optional Chaining
Another approach to safeguard against undefined
values is using optional chaining. Optional chaining lets you use the ?.
notation to only call methods and properties if the variable is not undefined
or null
.
type AuthorHandle = Nullable<string>
const twitter: AuthorHandle
const formattedHandle: string = twitter?.toLowerCase()
This way, you can leave out the if statement, and be sure that your variable is checked against both undefined
and null
.
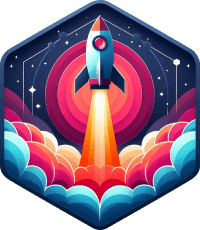
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

Understanding TypeScript

Mastering TypeScript
