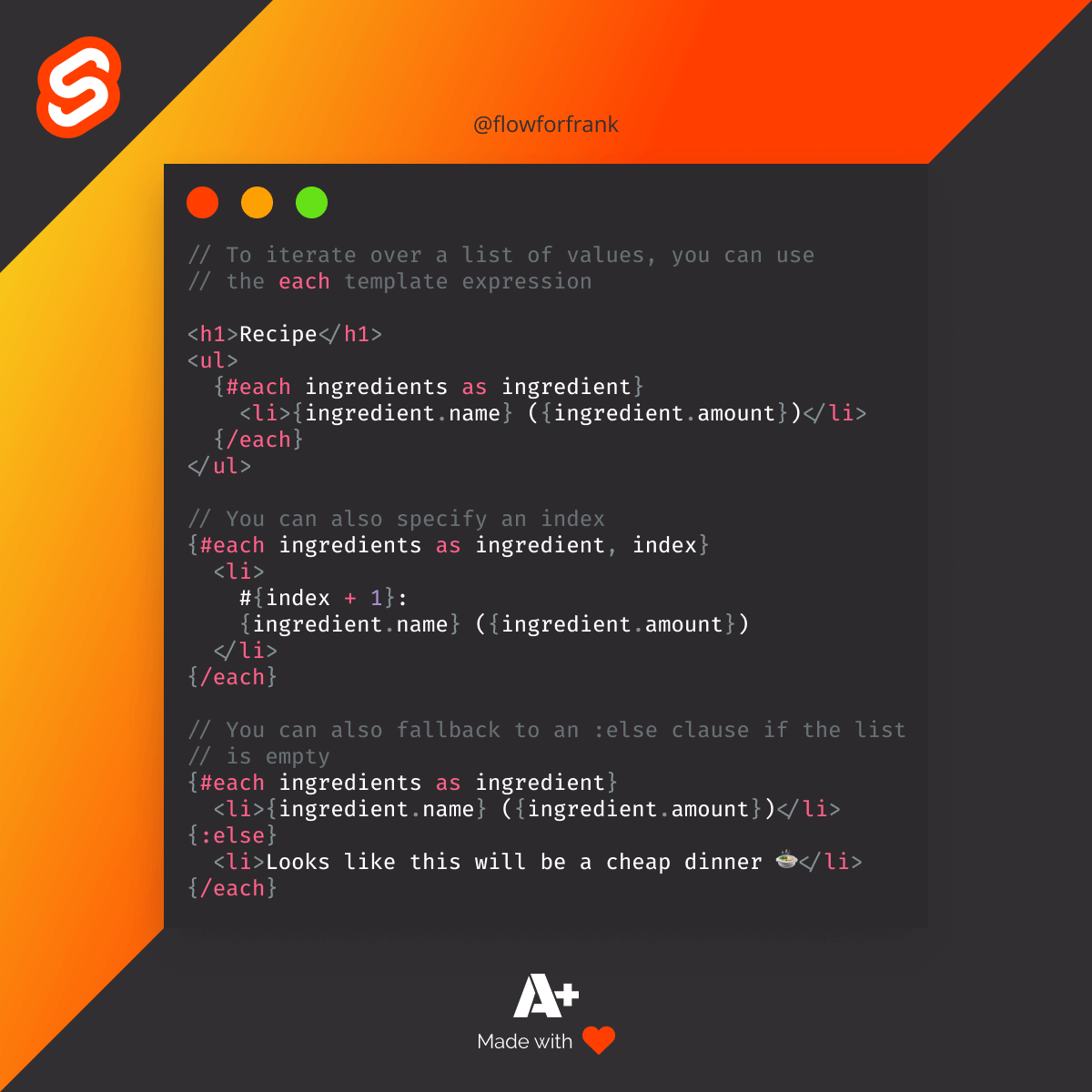
How to Use Loops in Svelte
In Svelte, you can iterate through a list using the each
template expression:
<h1>Recipe</h1>
<ul>
{#each ingredients as ingredient}
<li>{ingredient.name} ({ingredient.amount})</li>
{/each}
</ul>
Just as you would in other well-known frameworks, you can also specify the index
as a second parameter:
{#each ingredients as ingredient, index}
<li>
#{index + 1}:
{ingredient.name} ({ingredient.amount})
</li>
{/each}
Next to the index
, you can also specify a key
which identifies each list item:
{#each ingredients as ingredient, index (ingredient.id)}
<li>
#{index + 1}:
{ingredient.name} ({ingredient.amount})
</li>
{/each}
If a key
is provided, Svelte will diff changes, instead of removing or adding items at the end of the list. Svelte also allows the use of destructuring inside each
blocks:
{#each ingredients as { name, amount }, index (ingredient.id)}
<li>
#{index + 1}:
{name} ({amount})
</li>
{/each}
It also let's you use the spread operator:
{#each ingredients as { ...props }}
<Ingredient {...props} />
{/each}
You can also specify an else
clause. In case you don't have any elements inside the list, the content inside it will be displayed instead:
{#each ingredients as ingredient}
<li>{ingredient.name} ({ingredient.amount})</li>
{:else}
<li>Looks like this will be a cheap dinner π²</li>
{/each}
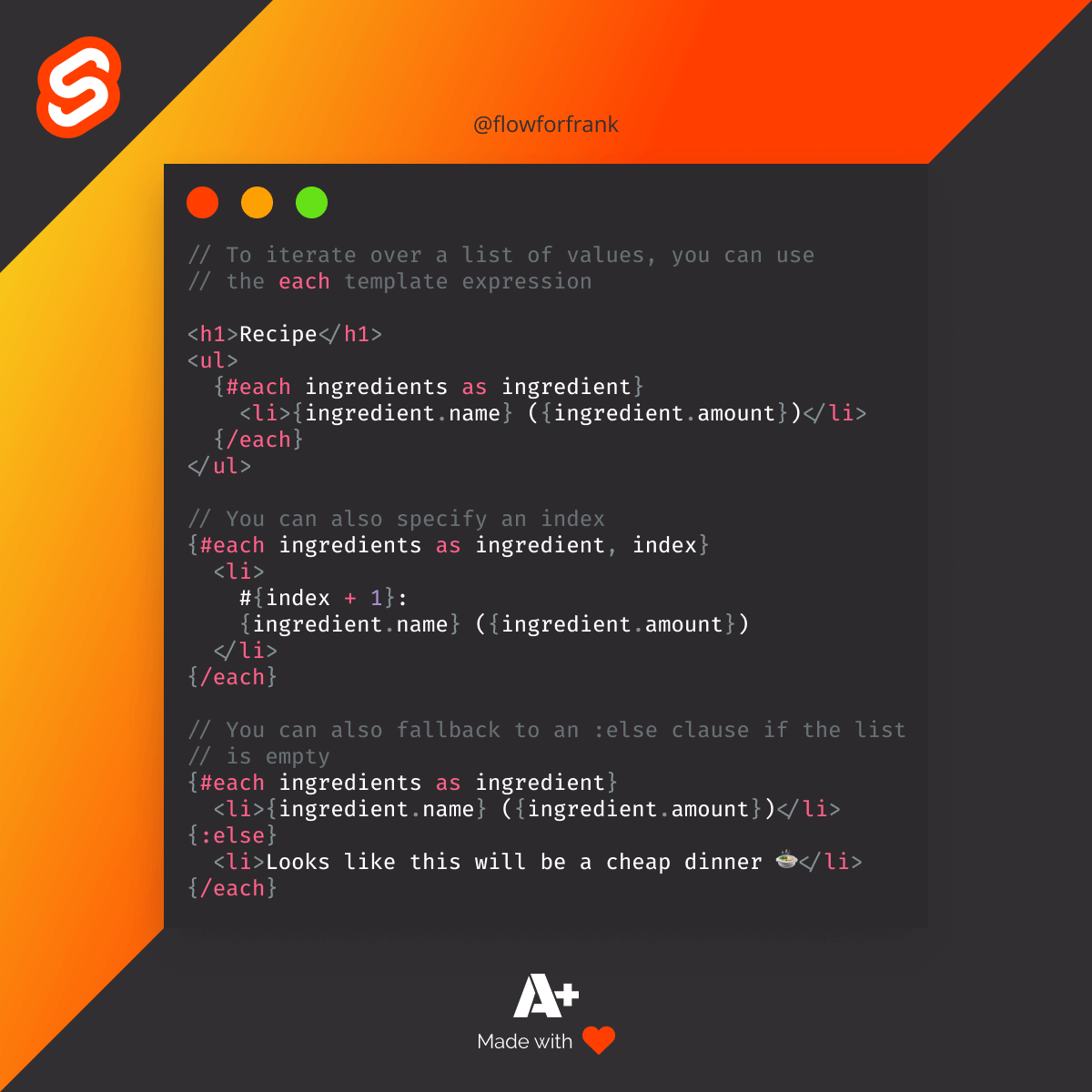
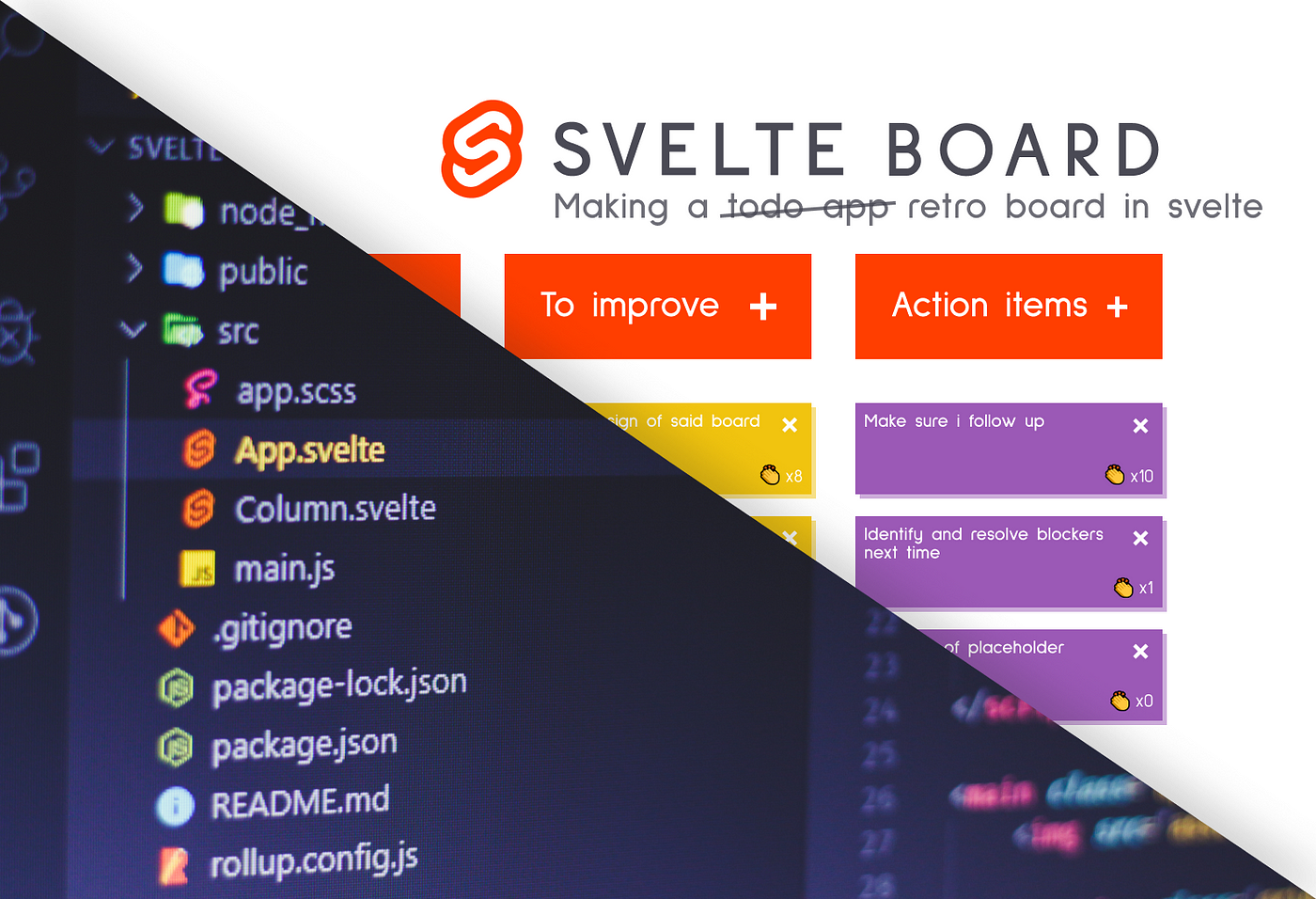
Resources:
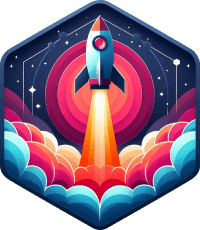
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: