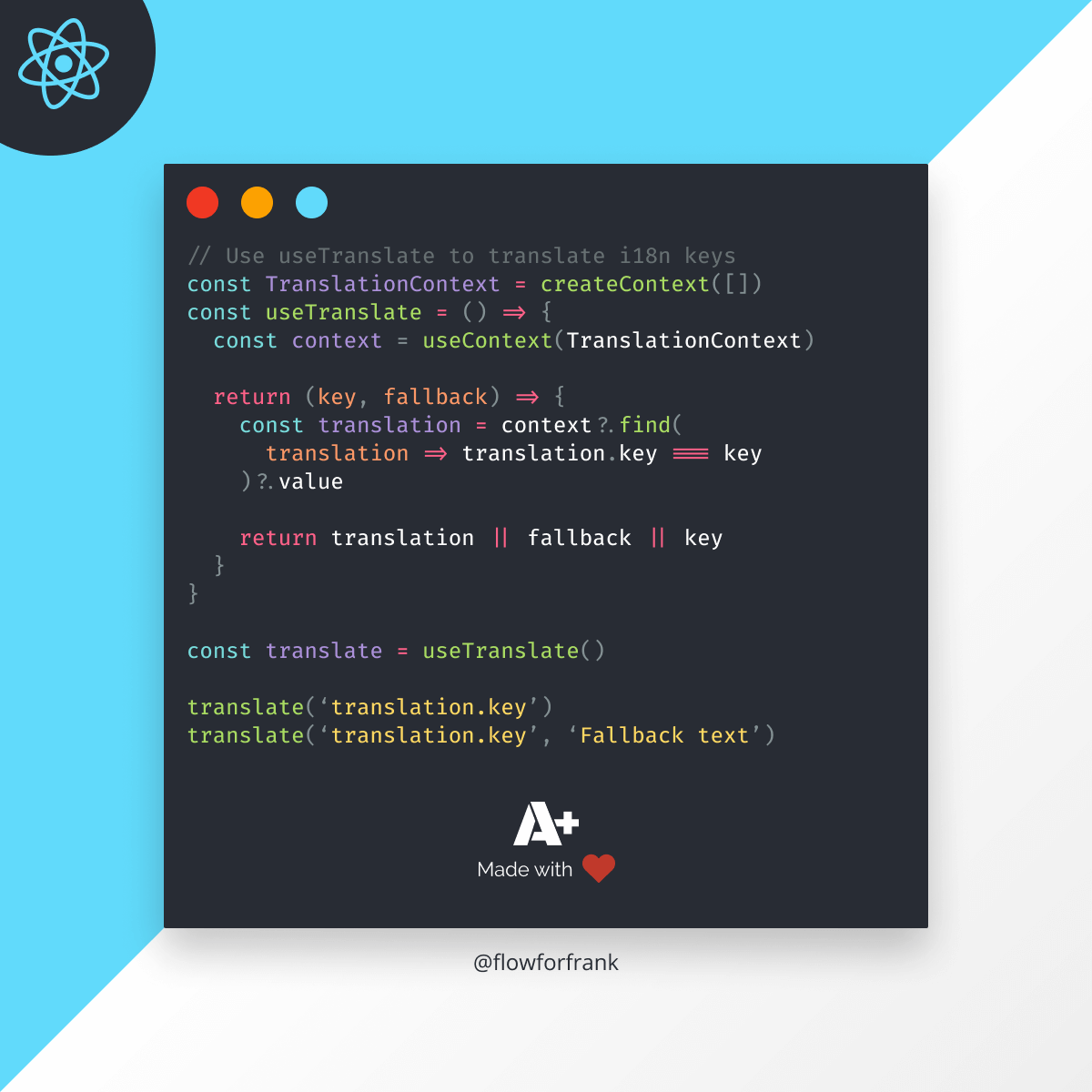
How to Create a useTranslate Hook in React
To create a useTranslate
hook in React that you can use for resolving translation keys, you first preferably want to create a context where you can store your translation keys to make it globally accessible in your React app:
import React, { createContext } from 'react'
const translations = [
{
key: 'hello',
value: 'Hello World! π'
}
]
export const TranslationContext = createContext([])
export const TranslationProvider = ({ children }) => (
<TranslationContext.Provider value={translations}>
{children}
</TranslationContext.Provider>
)
All translations can be stored in an array of objects, with the key for the translation, and its translated value. Usually, you will fetch this data from your backend. Make sure you wrap your app into this context, so we can use it later in our custom useTranslate
hook:
import ReactDOM from 'react-dom'
import App from './App'
import { TranslationProvider } from './context'
ReactDOM.render(
<TranslationProvider>
<App />
</TranslationProvider>,
document.getElementById("root")
)
Now let's create the actual hook in a new file:
import React, { useContext } from 'react'
import { TranslationContext } from './context'
const useTranslate = () => {
const context = useContext(TranslationContext)
return (key, fallback) => {
const translation = context?.find((translation) => translation.key === key)?.value
return translation || fallback || key
}
}
export default useTranslate
We need to use the built-in useContext
hook here to consume the value coming from the TranslationContext
. This hook returns another function that we can later call in our app with a translation key
and a fallback
text. To find a translation key, we can use Array.find
, and search for the key that we can pass to this hook.
If there is a match, its value
will be returned, otherwise, we can return a fallback text. If there is no fallback text either, we can return the translation key itself. To use this in your app, call the hook in the following way:
import useTranslate from './useTranslate'
export default function App() {
const translate = useTranslate()
return (
<div className="App">
<h1>{translate('hello')}</h1>
<h2>{translate('translation.unavailable', 'Showing fallback instead π')}</h2>
<h3>{translate('no.fallback.show.key.instead')}</h3>
</div>
)
}
If there is a match, it will show the translation. Otherwise, the hook will try to look for a fallback text. If it's not provided either, it will fall back to the translation. Now you can use a flag and request different translations from your backend to make your app multilingual. If you would like to tweak around with the project in one piece, try the hook on Codesandbox:

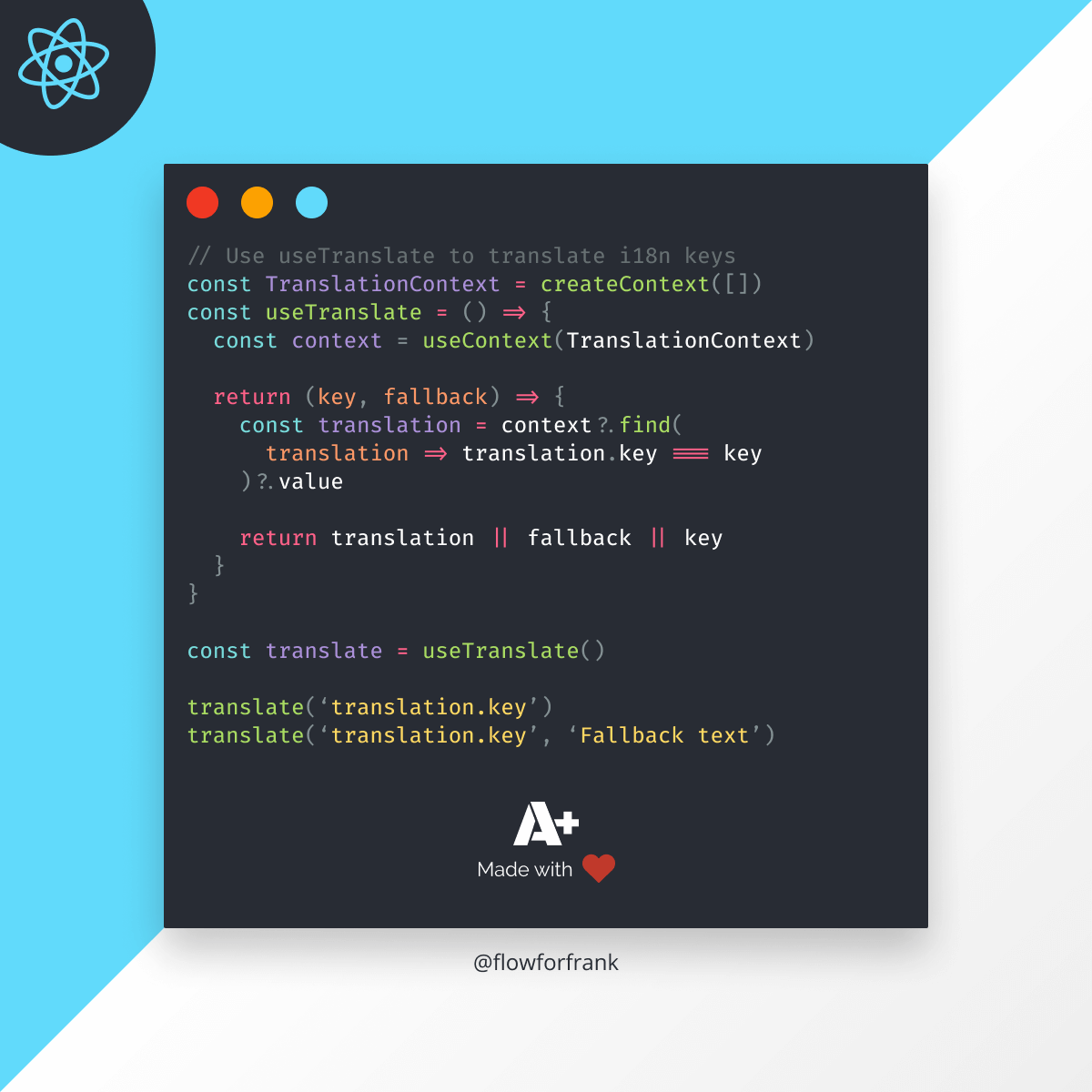
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
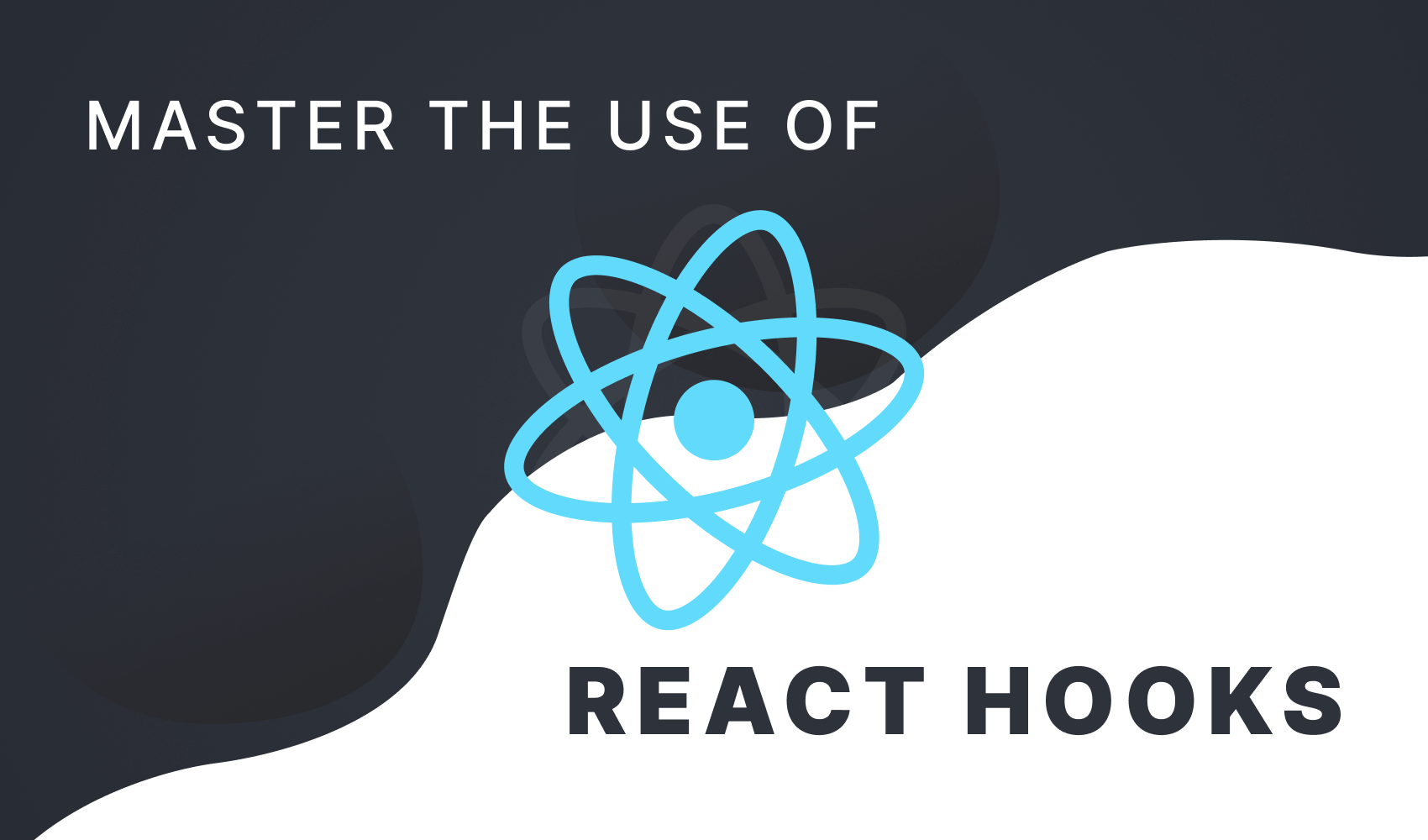
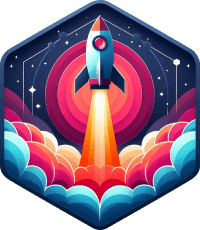
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: