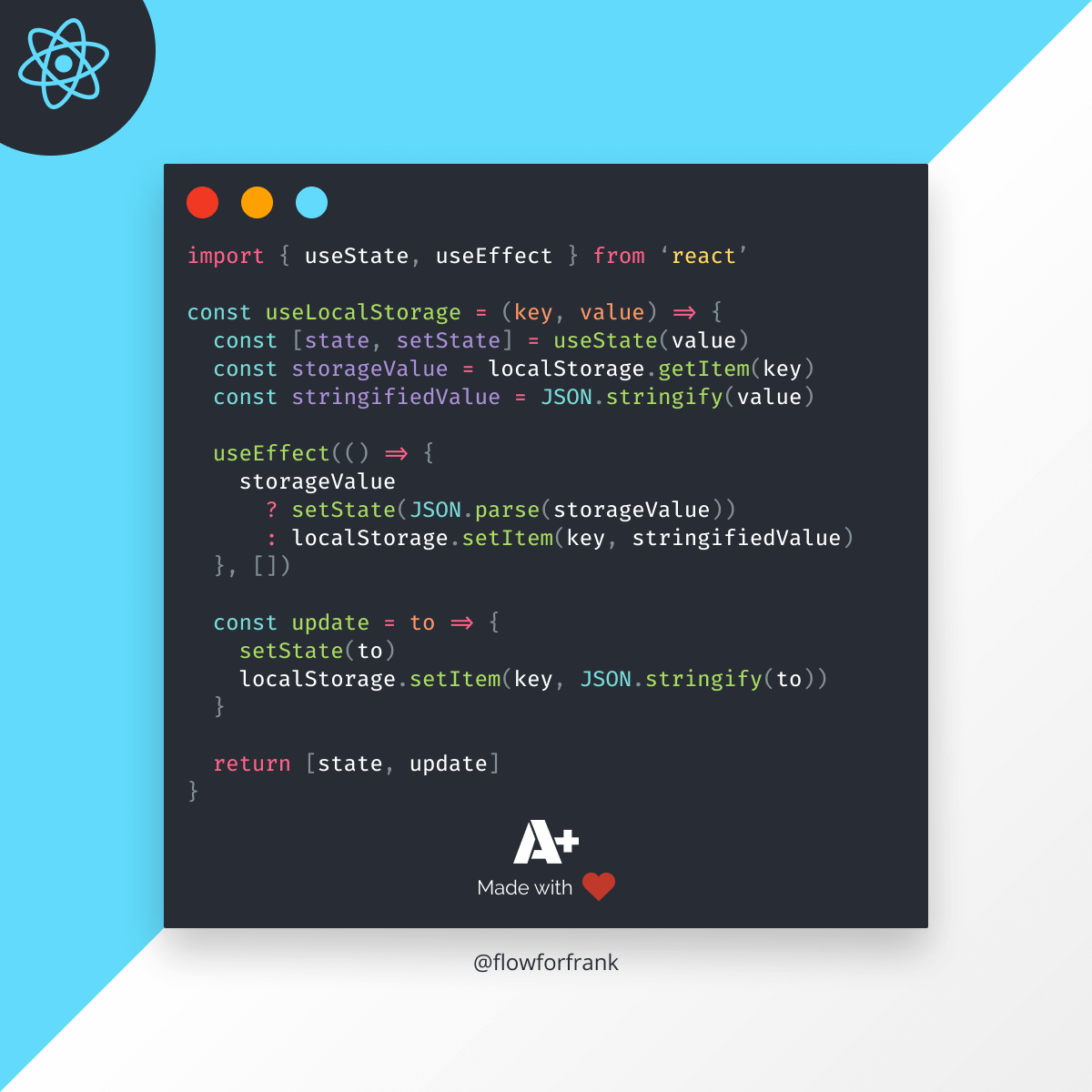
How to Create a useLocalStorage Hook in React
To use local storage with React, we can introduce a custom hook called useLocalStorage
that sets the appropriate keys in local storage, and retrieves them as JavaScript objects, all while keeping the state in sync:
import { useState, useEffect } from 'react'
const useLocalStorage = (key, value) => {
const [state, setState] = useState(value)
const localStorageValue = localStorage.getItem(key)
useEffect(() => {
if (localStorageValue) {
setState(JSON.parse(localStorageValue))
} else {
localStorage.setItem(key, JSON.stringify(value))
}
}, [])
const update = (to) => {
setState(to)
localStorage.setItem(key, JSON.stringify(to))
}
const remove = () => {
setState(null)
localStorage.removeItem(key)
}
return [state, update, remove]
}
First, we are going to need to import useState
and useEffect
, as we will be using those to set the state in React. This hook can accept a key (to target a local storage key), and a value (to store in the local storage key). If the key is already set in local storage, then it will be retrieved, otherwise, the hook will set it for you.
This hook also provides a way to update the state, as well as the local storage value, and a third option to remove the key. Also, instead of handling the values as strings, it converts them to JavaScript objects internally using JSON.parse
. To use this hook in our app, after importing it, we can call it in the following way:
// Set initial state, or retrieve the key if the state already exists in local storage
const [user, setUser, removeUser] = useLocalStorage('user', {
id: 1,
name: 'John Doe',
email: '[email protected]'
})
// Update both the state and local storage with the passed data
const update = () => {
setUser({
...user,
email: '[email protected]'
})
}
// Remove the 'user' key from local storage
removeUser();
If you would like to see it in action, give it a try on Codesandbox

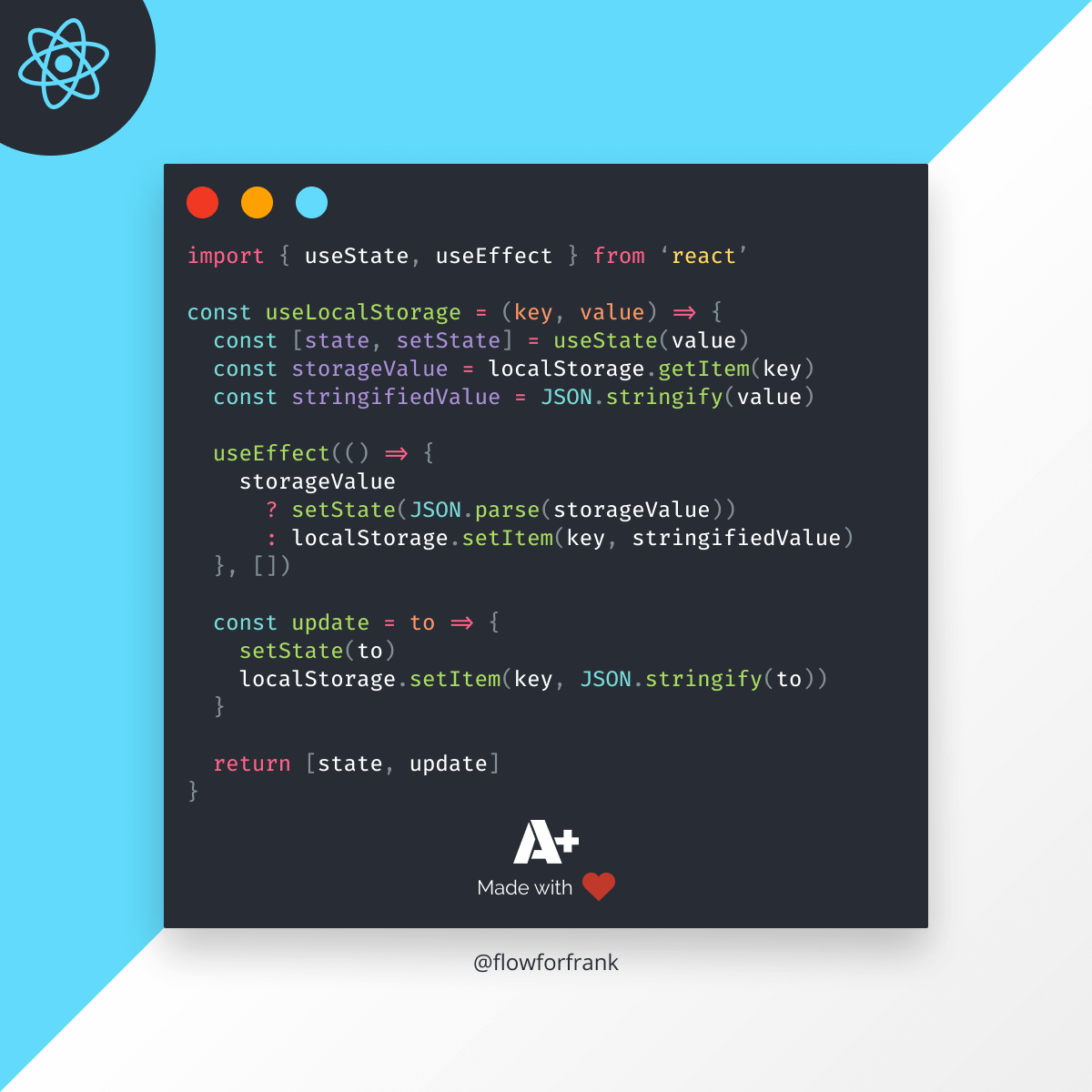
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
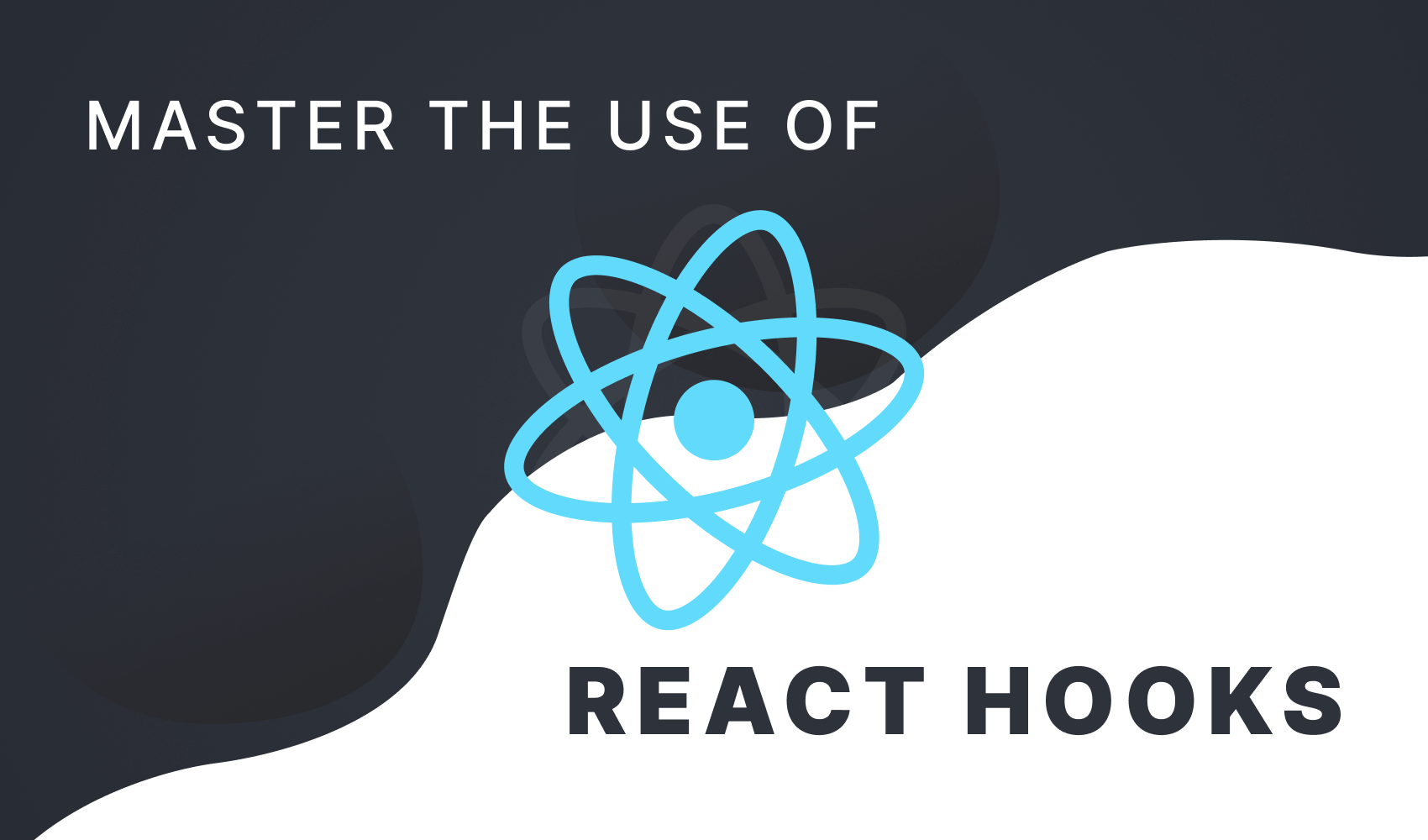
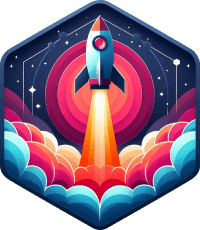
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: