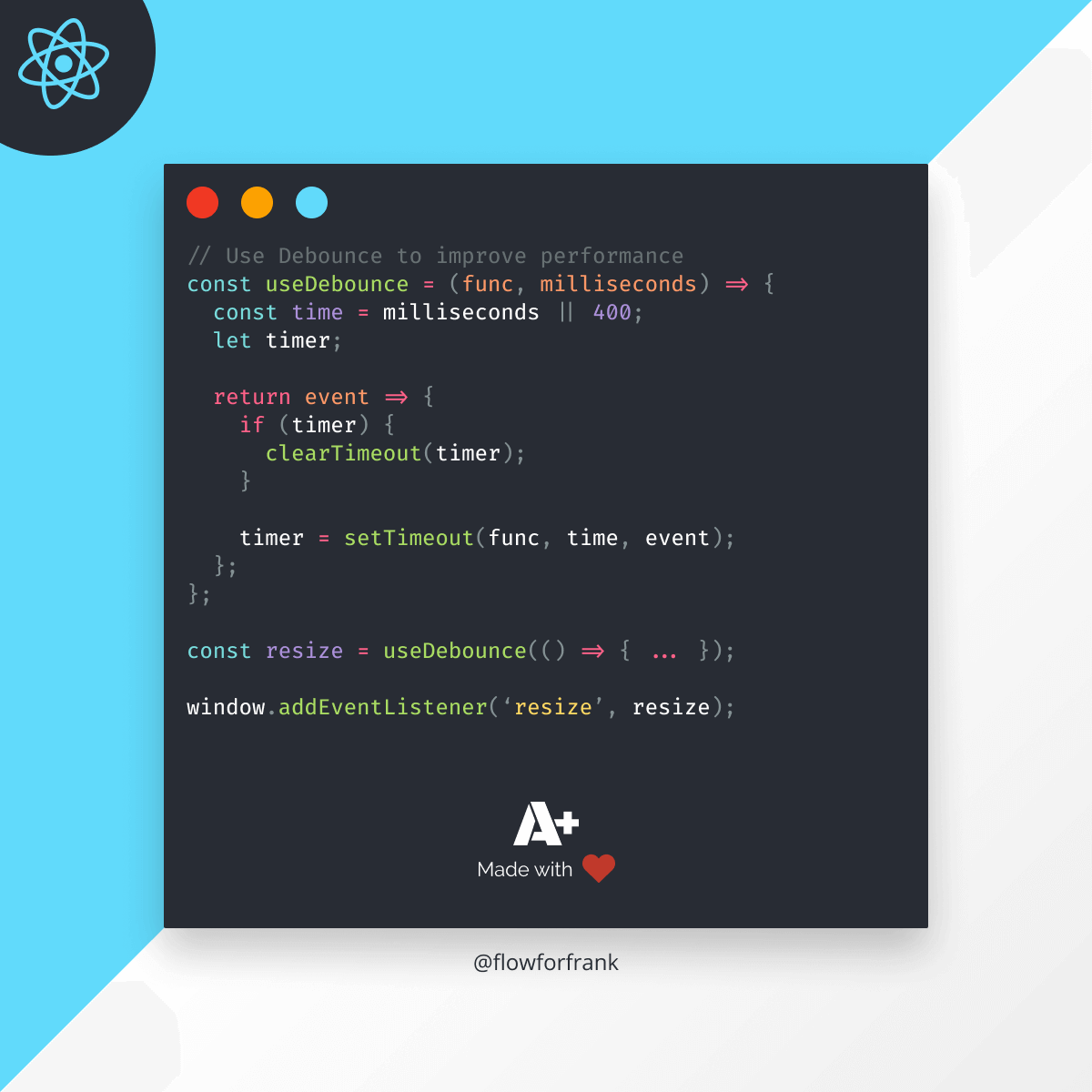
How to Create a useDebounce Hook in React
If you would like to create a useDebounce
hook in React, you can use setTimeout
and clearTimeout
together to fire events fewer times and improve the performance of your app:
const useDebounce = (func, milliseconds) => {
const time = milliseconds || 400
let timer
return event => {
if (timer) {
clearTimeout(timer)
}
timer = setTimeout(func, time, event)
}
}
As you can see, this function doesn't use any React hooks internally, so technically it may not be a hook, but it can still help us greatly reduce the number of times an event is fired, such as a scroll or a resize.
It returns a function, so you can wrap your callbacks inside this hook to get the desired effect. By default, it clears the timer every 400ms, but you can also decrease or increase this interval:
import useDebounce from 'hooks/useDebounce'
const App = () => {
const scroll = () => console.log('Scrolled without debounce')
const scrollWithDebounce = useDebounce(() => {
console.log('Scrolled with debounce fired')
})
const scrollWithMoreDebounce = useDebounce(() => {
console.log('Scrolled with debounce firing every 1000ms')
}, 1000)
window.addEventListener('scroll', scroll)
window.addEventListener('scroll', scrollWithDebounce)
window.addEventListener('scroll', scrollWithMoreDebounce)
}
In this example, the first function is fired countless times when the user scrolls the window, while the second callback is assigned to the useDebounce
hook, which will reduce the number of events fired every 400 milliseconds. The third example also sets the milliseconds to debounce the callback every 1000ms.
If you would like to see it in action, give it a try on Codesandbox

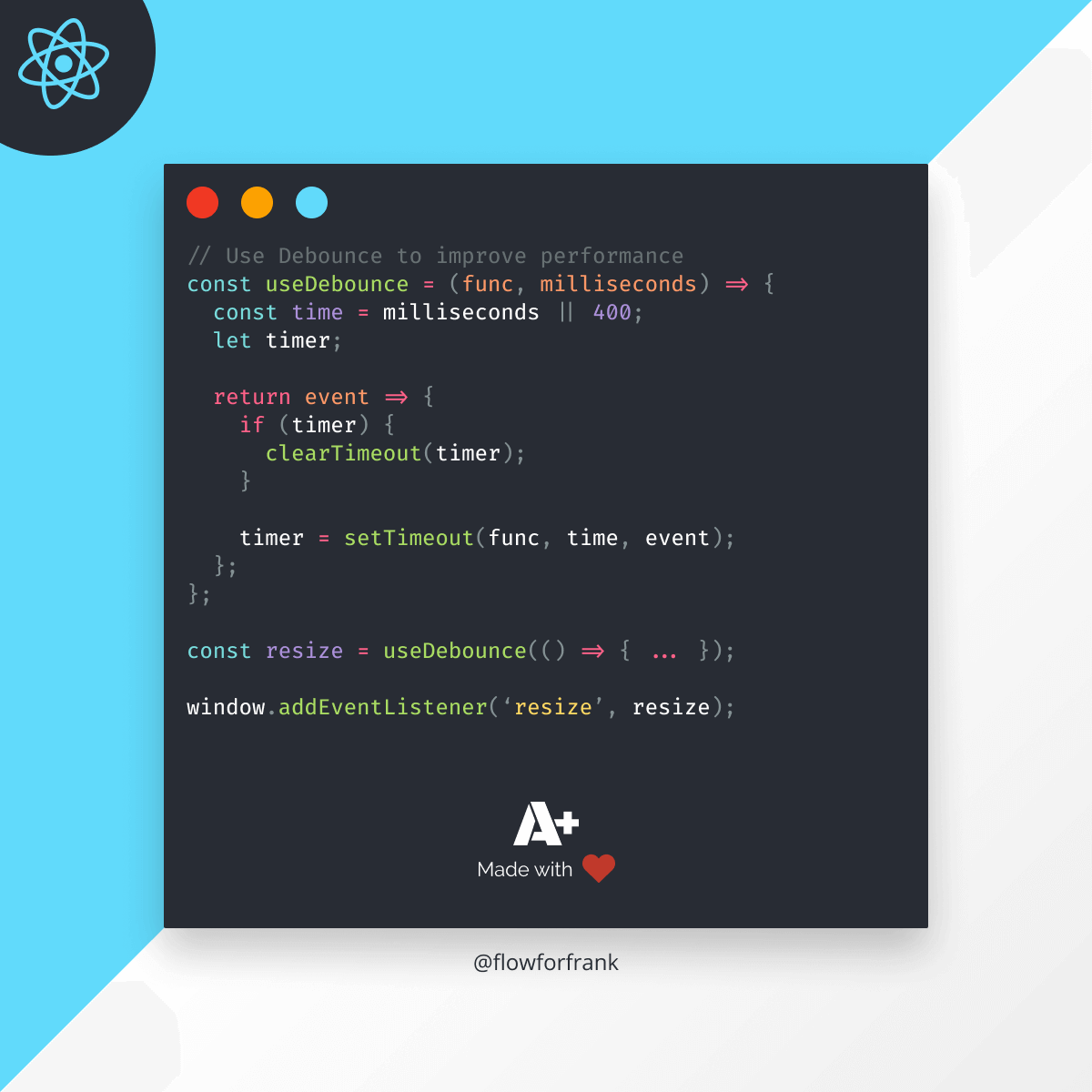
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
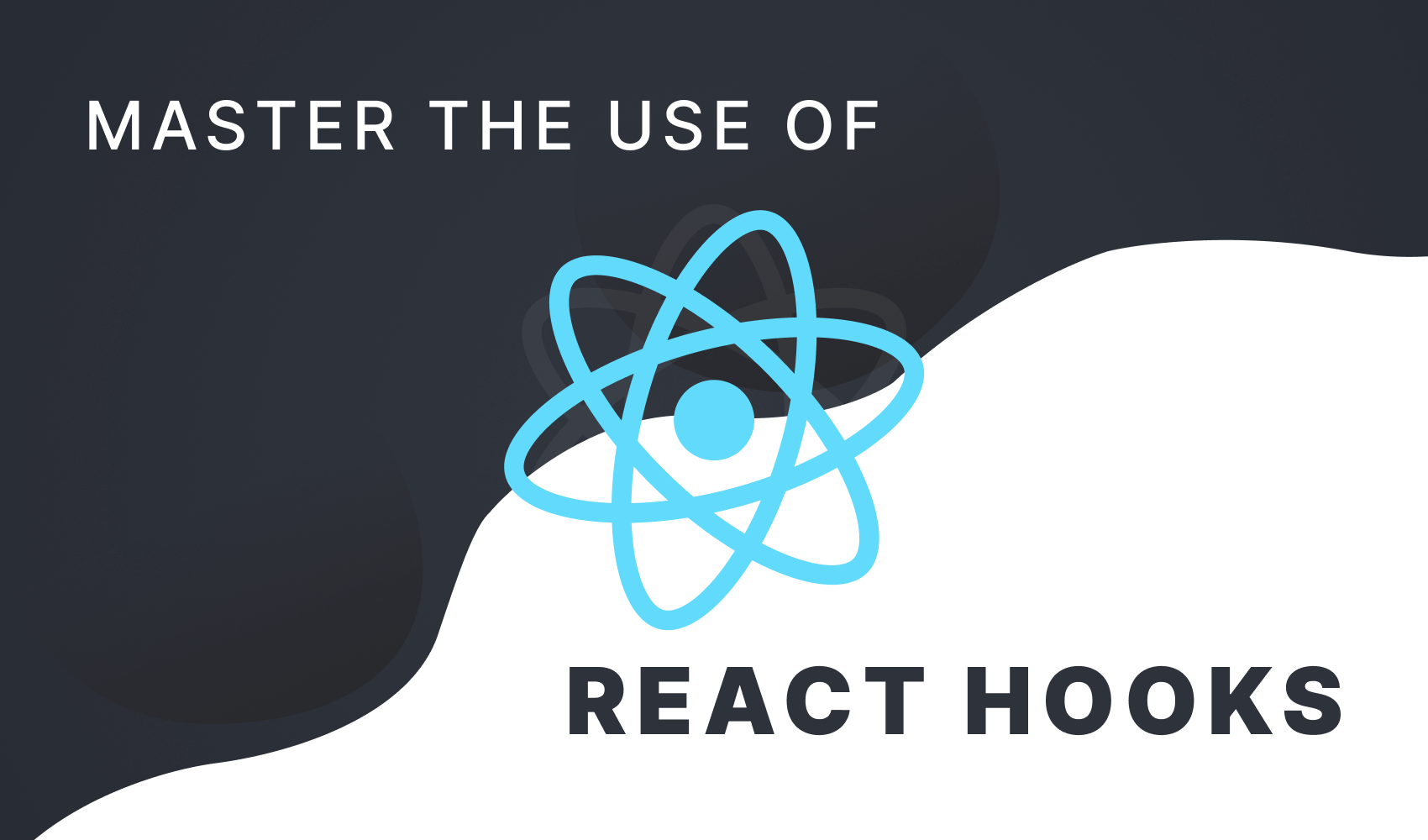
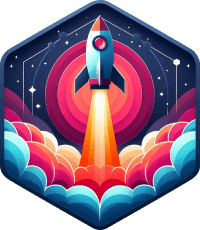
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: