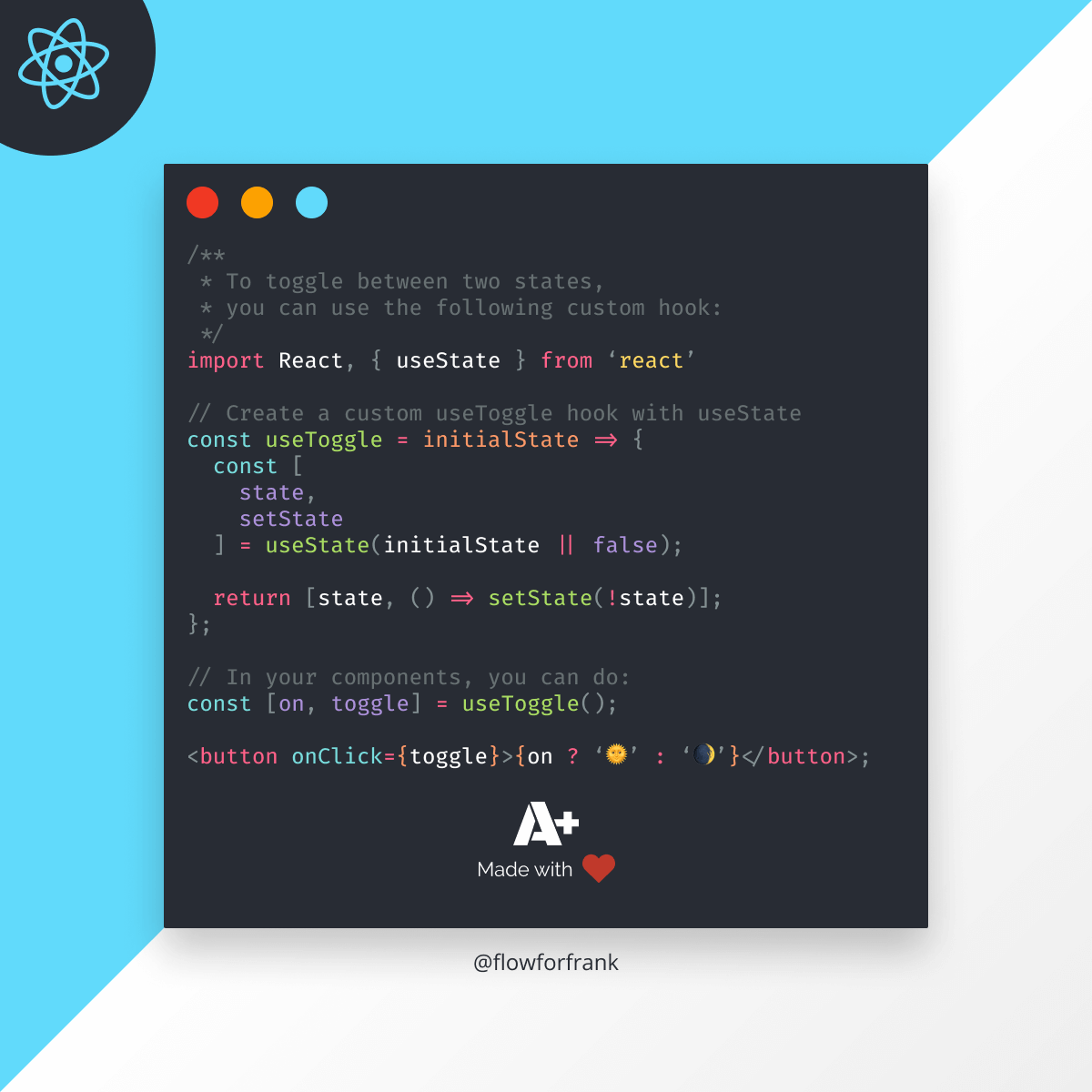
How to Make Toggles With React Hooks
To toggle between a boolean flag in React, you can use the below custom hook that uses setState
internally:
import { useState } from 'react'
const useToggle = initialState => {
const [state, setState] = useState(initialState || false);
return [state, () => setState(!state)];
};
Since the function returns an array, with a state and a callback function, you can use it in your component, just like you would for other built-in React hooks, like so:
import React, { useState } from 'react'
import useToggle from 'useToggle'
const toggleComponent = () => {
const [lightsOn, toggleLights] = useToggle();
const [isVisible, toggleSidebar] = useToggle();
return (
<React.Fragment>
<button onClick={toggleLights}>{lightsOn ? 'π' : 'π'}</button>;
<button onClick={toggleSidebar}>Toggle sidebar</button>;
<Sidebar visible={isVisible} />
</React.Fragment>
);
};
If you would like to see it in action, give it a try on Codesandbox

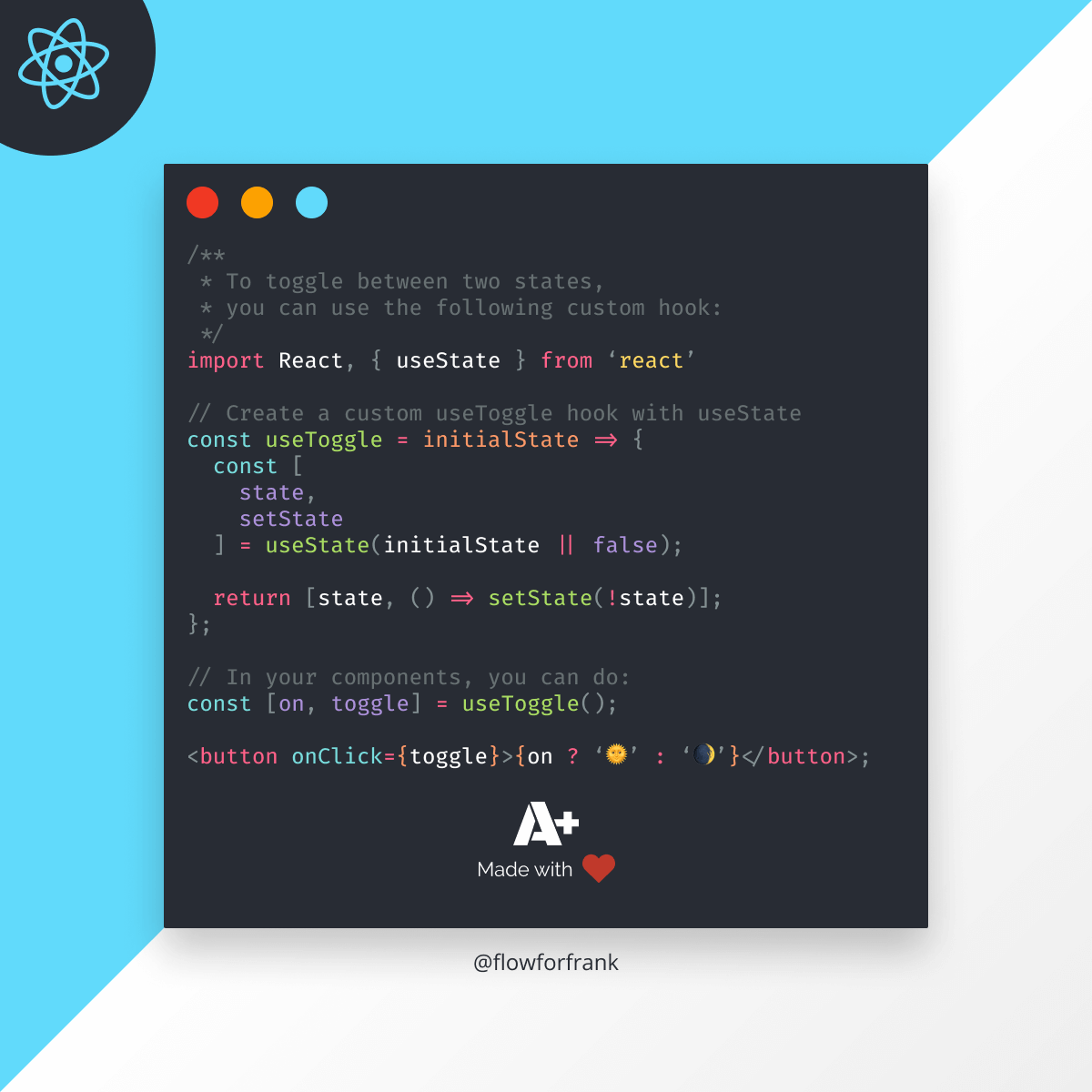
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
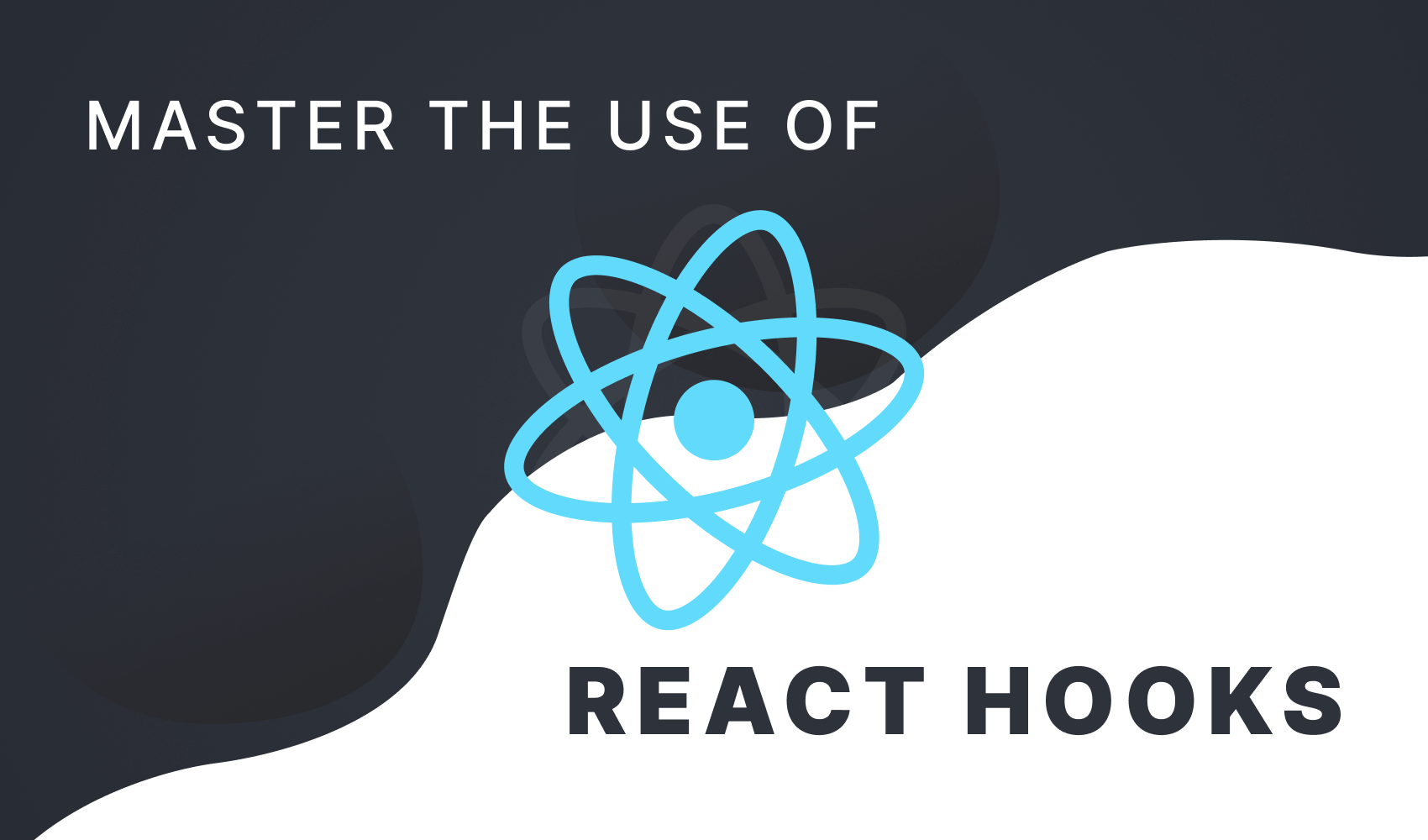
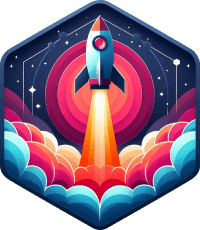
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: