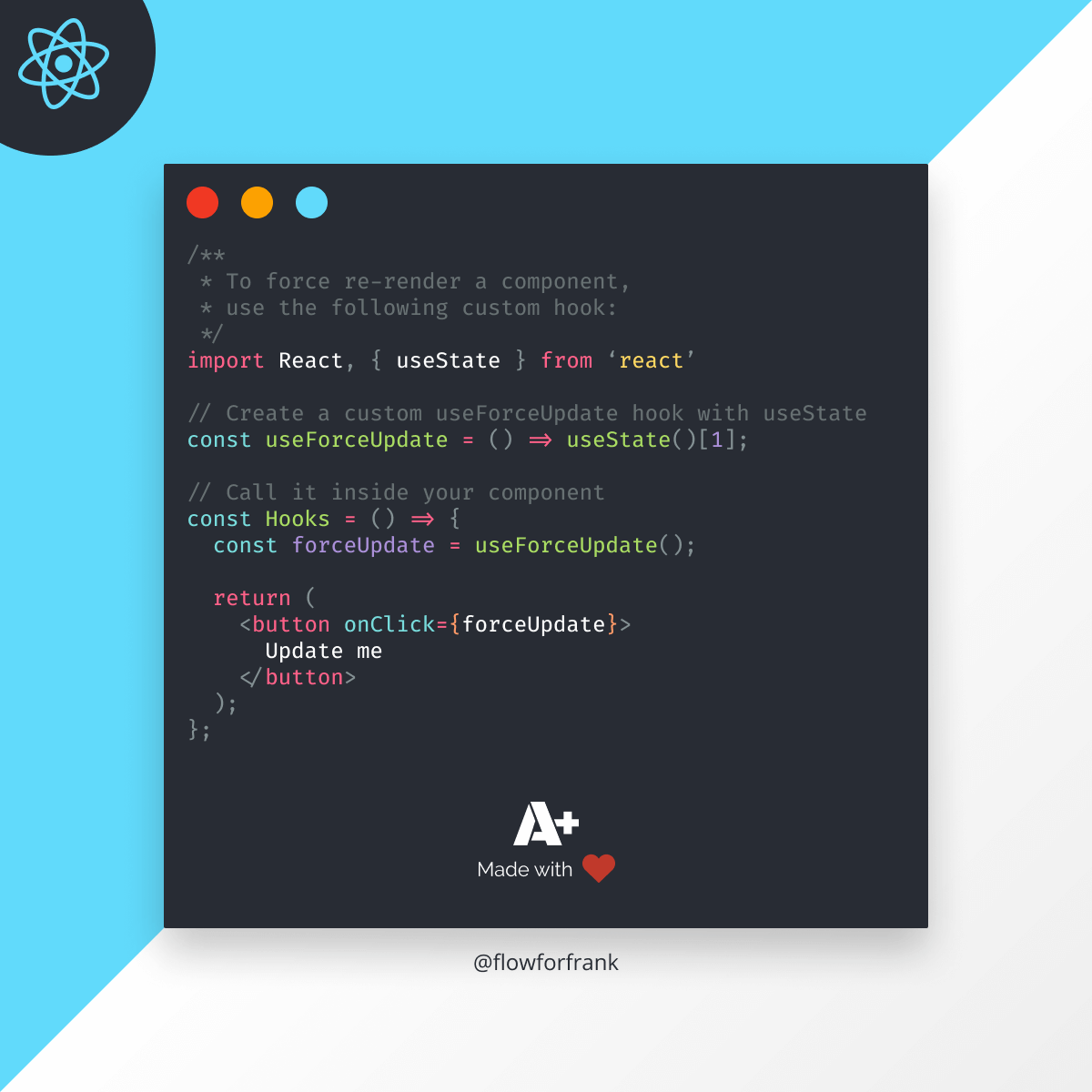
How to Force Rerender With Hooks in React
You can force re-renders of your components in React with a custom hook that uses the built-in useState
hook:
The following hook should only be used in exceptional cases. Only re-render your component when state changes.
import React, { useState } from 'react'
// Create a custom useForceUpdate hook with useState
const useForceUpdate = () => useState()[1];
// Call it inside your component
const Hooks = () => {
const forceUpdate = useForceUpdate();
return (
<button onClick={forceUpdate}>
Update me
</button>
);
};
The example above is equivalent to the functionality of the forceUpdate
method in class-based components. This hook works in the following way:
- The
useState
hook β and any other hook for that matter β returns an array with two elements, a value (with the initial value being the one you pass to the hook function) and an updater function. - In the above example, we are instantly calling the updater function, which in this case is called with
undefined
, so it is the same as callingupdater(undefined)
.
If you would like to see it in action, give it a try on Codesandbox

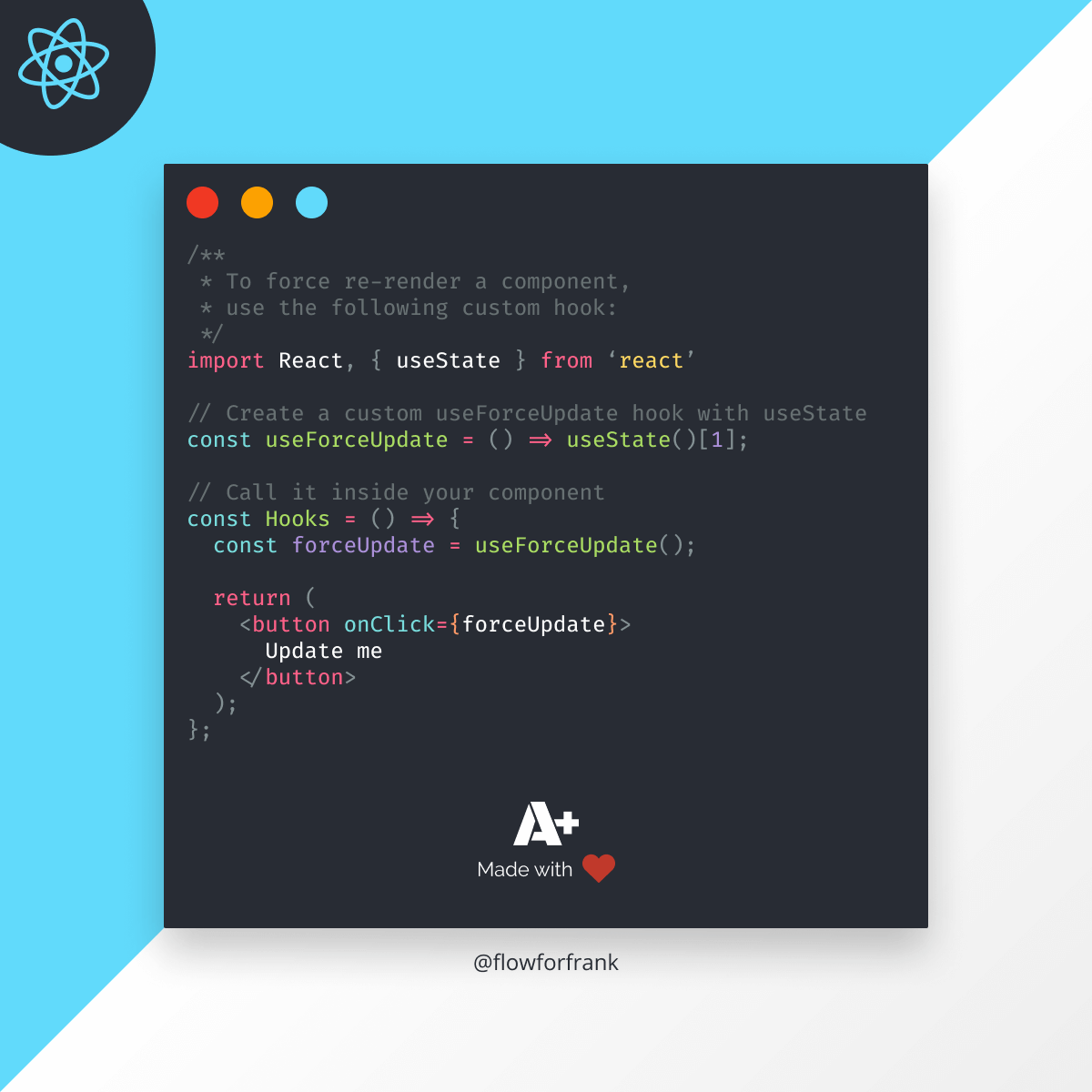
If you are interested in reading more about React hooks, see more examples for custom hooks or just learn about the basics, make sure to check out the article below.
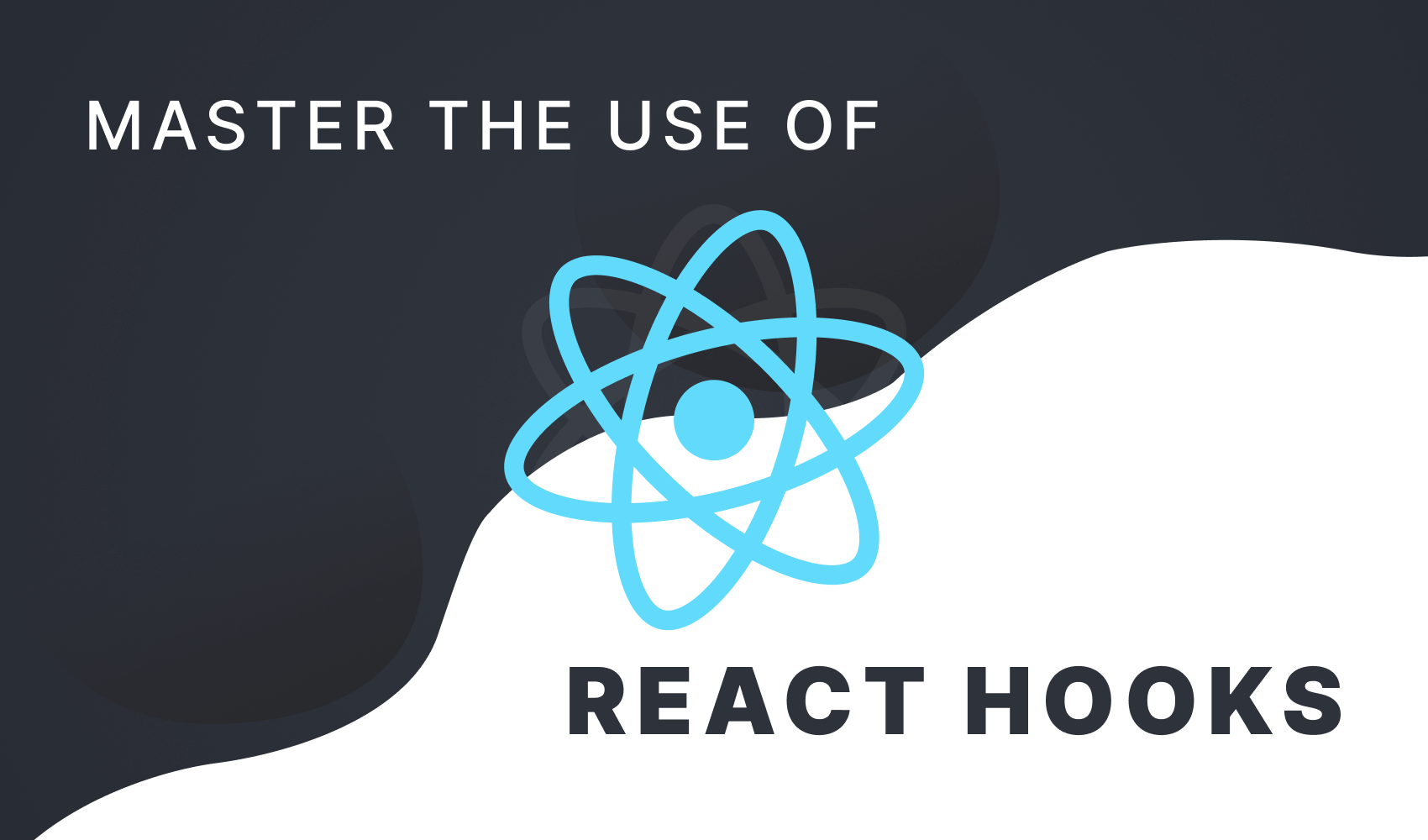
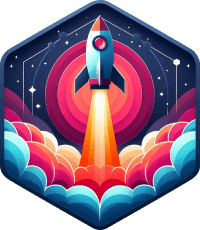
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: