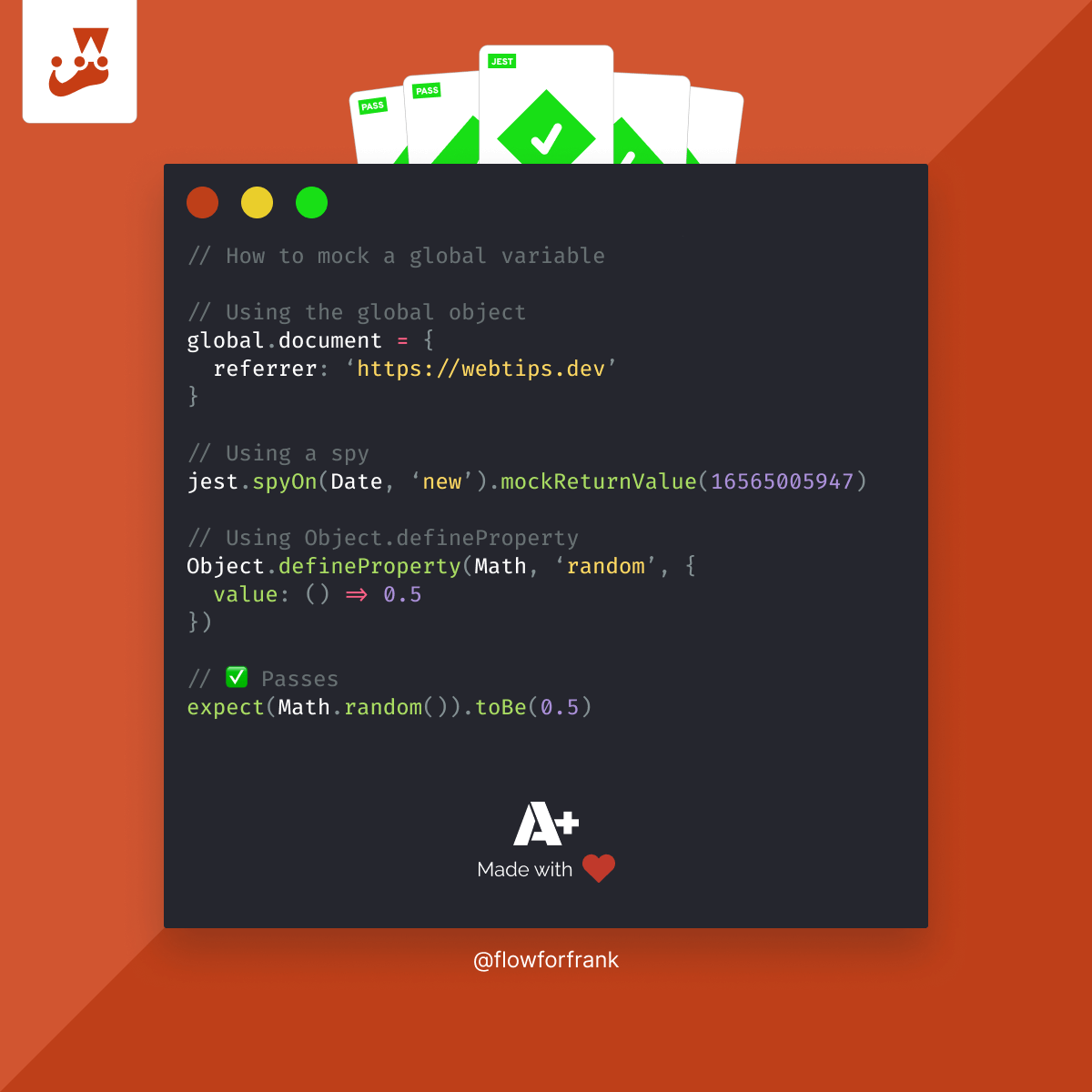
3 Ways to Mock Global Variables in Jest
There are many different ways to mock global variables in Jest. Here are three different ways you can mock global variables like Math.random
or Date.now
.
// Using the global object
global.document = {
referrer: 'https://webtips.dev'
}
// Using a spy
jest.spyOn(Date, 'new').mockReturnValue(16565005947)
// Using Object.defineProperty
Object.defineProperty(Math, 'random', {
value: () => 0.5
})
The first solution uses the global
object on which you can reassign values to mock them. The overwrite only works for the current test suite and will not affect other tests.
Need to mock process.env
in Jest? Check out this tutorial.
If you need to mock a global variable for all of your tests, you can use the setupFiles
in your Jest config and point it to a file that mocks the necessary variables. This way, you will have the global variable mocked globally for all test suites.
module.exports = {
setupFiles: ['<rootDir>/setup-mock.js']
}
// Inside setup-mock.js
global.document = {
referrer: 'https://webtips.dev'
}
In case you are running into issues using the global
object, you can also use a spy with mockReturnValue
by passing the object as the first parameter, and the method as a string for the second parameter:
jest.spyOn(Date, 'new').mockReturnValue(16565005947)
// β
Passes
expect(Date.now()).toBe(16565005947)
Lastly, if none of the above solutions are suitable for you, you can also use Object.defineProperty
similarly to jest.spyOn
. It accepts a configuration object as the third parameter where you can set the value through the value
property.
Object.defineProperty(Math, 'random', {
value: () => 0.5,
writable: true
})
// β
Passes
expect(Math.random()).toBe(0.5)
To avoid getting TypeError: Cannot redefine property
, you will need to set writable
to true
on the property. This will enable the property to be redefinable.
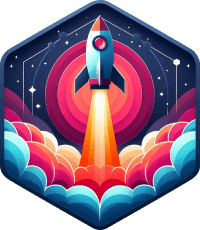
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

JavaScript Unit Testing

Unit Testing for TypeScript and Node.js Developers
