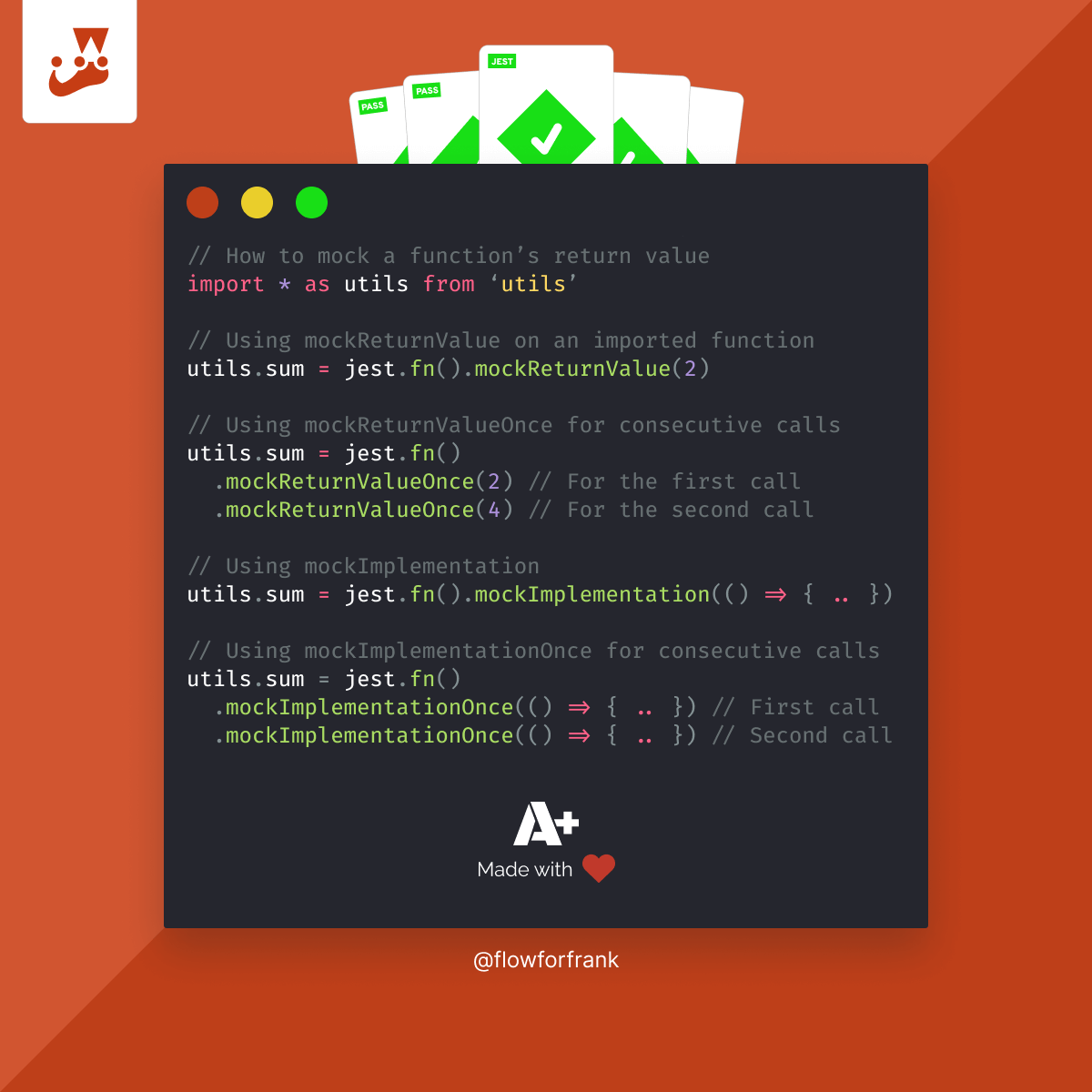
4 Ways to Mock Function Return Values in Jest
Mocking functions is a core part of unit testing. Oftentimes, your original functions may have side effects that can break your test suite if not handled the right way. You can mock these functions to avoid any side effects, but sometimes you may only want to mock the return value of these functions.
To mock a function's return value in Jest, you first need to import all named exports from a module, then use mockReturnValue
on the imported function.
// First, import all named exports from the module
import * as utils from 'utils'
utils.sum = jest.fn().mockReturnValue(2)
describe('Mock return value', () => {
it('Should mock the return value of sum', () => {
expect(utils.sum()).toBe(2)
})
})
You can use the * as <alias>
inside an import
statement to import all named exports.
Then, you need to chain mockReturnValue
off of jest.fn
. In the above example, the return value of the sum
function will be mocked to 2.
Mocking consecutive calls with different return values
In case you need to mock the return value of a function differently for each consecutive call, you can use a chain of mockReturnValueOnce
.
it('Should mock the return value of consecutive calls differently', () => {
utils.sum = jest.fn()
.mockReturnValue(0) // For default mocks
.mockReturnValueOnce(2) // For the first call
.mockReturnValueOnce(4) // For the second call
expect(utils.sum()).toBe(2)
expect(utils.sum()).toBe(4)
expect(utils.sum()).toBe(0)
})
In the above example, the return value of the mocked function will be different for the first two calls. For the first call, it will return 2, for the second call, it will return 4, while for every other call, it will return 0. You can chain mockReturnValueOnce
as many times as necessary, and create a default mocked value using mockReturnValue
.
Mocking return values using mockImplementation
Another way to mock the return value of your function is using the mockImplementation
call. Unlike mockReturnValue
, this can also be used to mock the entire implementation of your functions, not just their return values.
utils.sum = jest.fn().mockImplementation(() => {
// You can include your mock implementation here
// Then mock the return value using a return statement
return 2
})
// Or simply
utils.sum = jest.fn(() => {
// You can also handle mock implementations this way
})
Note that you can also use jest.fn(implementation)
in place of mockImplementation
. It is only a shorthand, therefore the functionality remains the same. Lastly, you can also use mockImplementationOnce
to mock the return value differently for each consecutive call, just like with mockReturnValueOnce
.
it('Should mock the return value of consecutive calls differently', () => {
utils.sum = jest.fn()
.mockImplementation(() => 0) // For default mocks
.mockImplementationOnce(() => 2) // For the first call
.mockImplementationOnce(() => 4) // For the second call
expect(utils.sum()).toBe(2)
expect(utils.sum()).toBe(4)
expect(utils.sum()).toBe(0)
})
This works in a very similar way to mockReturnValueOnce
, except it also mocks the implementation of your function.
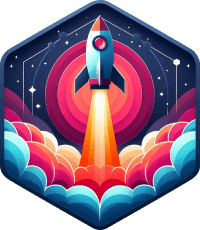
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies:
Courses

JavaScript Unit Testing

Unit Testing for TypeScript and Node.js Developers
