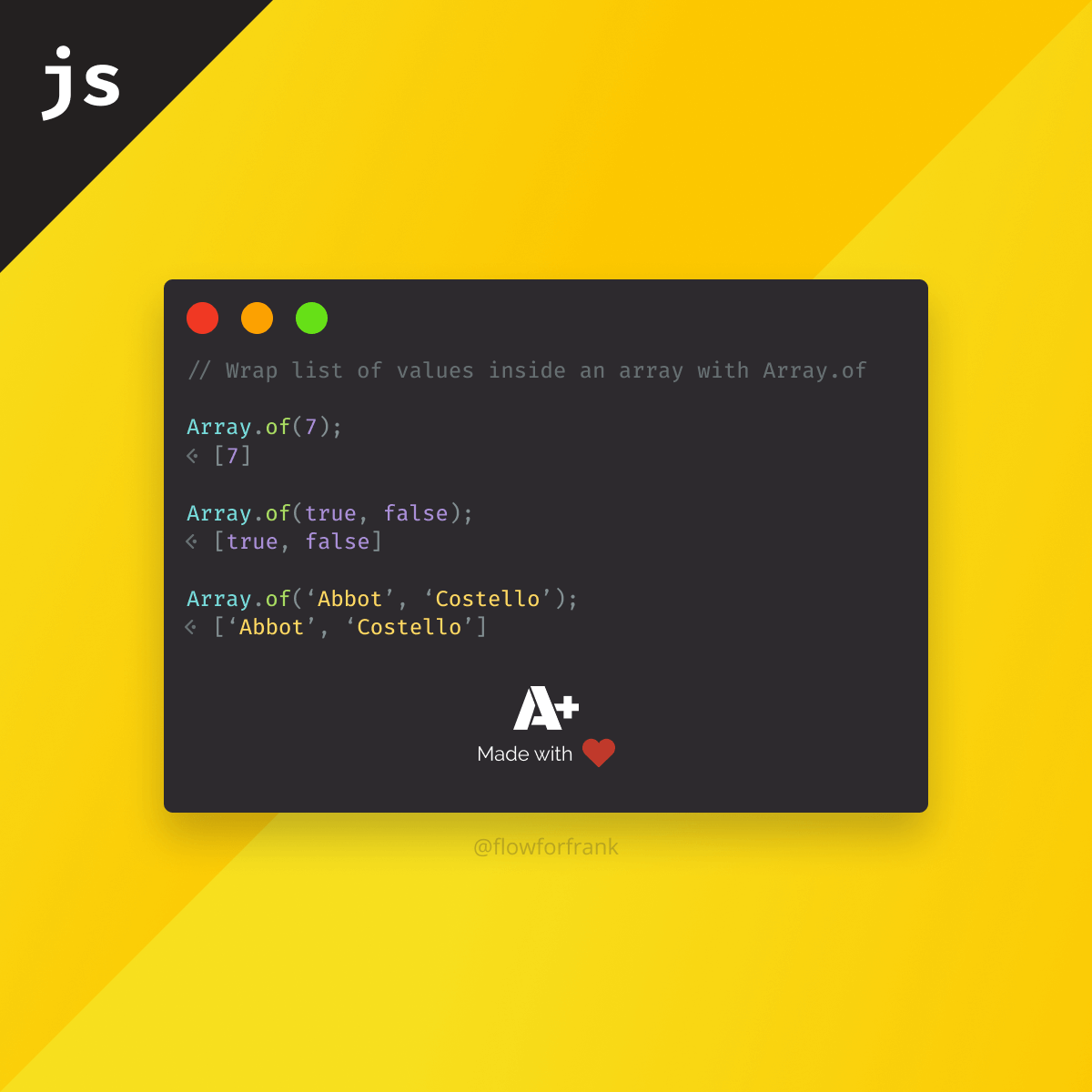
How to Turn Values Into Arrays in JavaScript
You can easily turn any value into an array in JavaScript by wrapping it into an Array.of
array method call:
Array.of(7); // Returns [7]
Array.of(true, false); // Returns [true, false]
Array.of('Abbot', 'Costello'); // Returns ['Abbot', 'Costello']
No matter how many arguments you want to work with, Array.of
accepts a variable number of arguments, meaning you can play with any number of values. You can also simply use the Array
object as a wrapper, but note that it won't work for single numbers:
Array.of(7); // Returns [7]
Array(7); // Returns [empty x 7]
Array(true); // Returns [true]
Array('Abbot') // Returns ['Abbot']
Have a long string that you also want to turn into numbers before turning it into an array? You can use the split
method in combination with a map:
'123456789'.split('').map(item => Number(item)) // Returns [1, 2, 3, 4, 5, 6, 7, 8, 9]
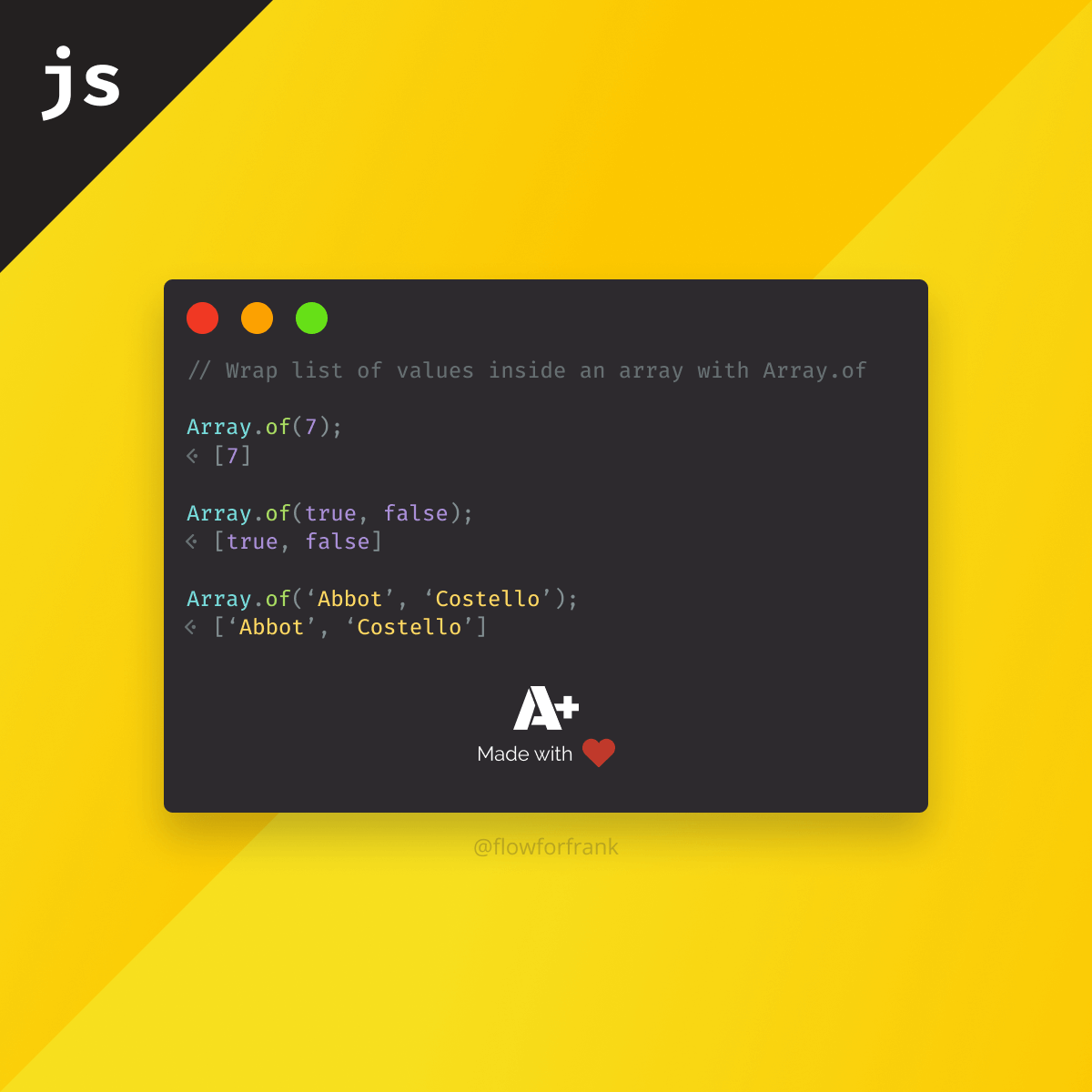
Resources:
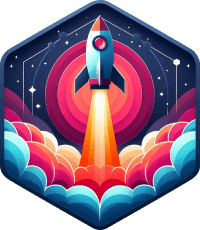
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: