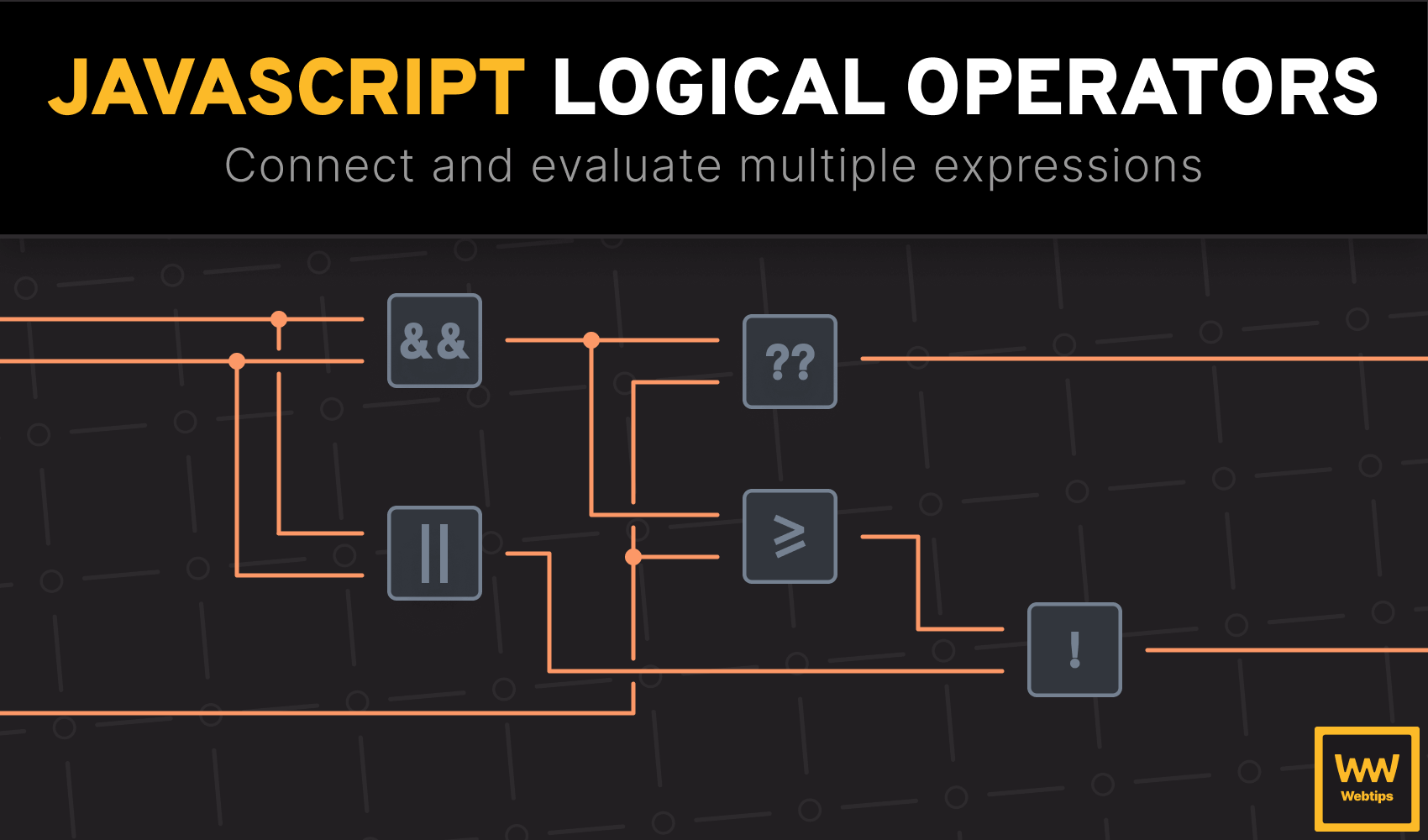
Logical Operators Explained in JavaScript
This lesson is a preview from our interactive course
Say that we want to check two things at a time. We want to check if a number is greater than 10, but less than 100. How would we do that? That is where logical operators come into place. Letβs see what kind of logical operators we have in JavaScript.
Logical AND#
First, we have the logical AND operator. As in the imaginary situation above, it can be used exactly to combine multiple comparisons together. If all comparisons are true
, then the statement is true
as well. Letβs see what it would look like in terms of code:
const x = 50;
x > 10 && x < 100;
// Or inside an if statement
if (x > 10 && x < 100) {
}
To use the logical AND, we need to use two ampersands next to each other: &&
. This means, that we only want the code to run inside the if
statement if x
is greater than 10, but less than 100.
Combining multiple comparisons together will create a boolean. If all statements are true
, then the output will be true
as well. If just one of the statements is false
, then the output will be false
too. Here is the result table for the logical AND:
Expression | Output |
---|---|
true && true | true |
true && false | false |
false && true | false |
false && false | false |
Note that only two true
values will produce a true
output. In every other case, it'll be evaluated as false
. We can also combine as many statements together as we would like. We may also see this for variables when we try to define a boolean flag:
const x = 50;
const isTrue = x
&& x > 10
&& x < 100
&& x !== 49;
console.log(isTrue);
Take note that for readability, it's best if we break multiple comparisons into new lines, each one starting with the logical AND operator. If you have too many conditions, it's also recommended to use multiple variables instead of one.
Logical OR#
What happens if we only want one statement to be true, but not all? Thatβs when we need to use the logical OR operator. If one of the operands is evaluated to true
when using logical OR, then the expression itself will also be evaluated to true
. To use logical OR, we need to use two pipes like so:
const x = 10;
x > 10 || x < 100;
// Or inside an if statement
if (x > 10 || x < 100) {
}
This means that even though x
is not greater than 10, the expressions will still be evaluated to true
because the second part is true. Letβs see how the result table looks like for the logical OR operator:
Expression | Output |
---|---|
true && true | true |
true && false | true |
false && true | true |
false && false | false |
Note that when using logical OR, we'll only get a false
value if all operands are false
. We can also combine different logical operators, so we can have the following:
const x = 10;
const isTrue = x
&& x > 10
|| x < 100
&& x !== 49;
console.log(isTrue);
The logical OR operator is also commonly used during variable assignments to provide fallback values. Take the following as an example:
const uploadedFileName = null;
const fileName = uploadedFileName || 'unnamed';
console.log(fileName);
Here we say that the fileName
should match the uploaded file's name. In case it's not provided (just like in the example above), then use a hard-coded string as the filename: "unnamed". We can chain this with multiple logical ORs, which will go from left to right until it finds a truthy value:
const uploadedFileName = null;
const defaultFileName = 'default';
const fileName = uploadedFileName || defaultFileName || 'unnamed';
console.log(fileName);
Nullish Coalescing#
There is also an operator in JavaScript called the nullish coalescing operator that works in a similar way to logical OR. It can be used during variable assignments, just like the above, but this time, it's denoted with two question marks:
const foo = null ?? 'Foo Fighters';
const age = 0 ?? 42;
// console.log(foo);
console.log(age);
Using the nullish coalescing operator, we can return the left-hand side of the operand if it's falsy, but not null
or undefined
. This means that for foo
, it'll return the string (the right-hand side of the operand), but for the age
, it'll return 0 (the left-hand side of the operand).
This can be used to only filter null
and undefined
values. It's useful if we still want to work with "valid" falsy values such as false
, or 0
.

Logical NOT#
Lastly, we have the logical NOT operator. It's for negating values. This can be achieved by using an exclamation mark in front of a value. We have already seen this when we briefly touched it in the conditions and loops lesson:
!true;
We can also prefix entire expressions with it as well, by wrapping the expression into parentheses:
const x = 50;
const expression = !(x > 10 && x < 100);
console.log(expression)
We have also talked about double negation before. This is negating the negated value into its original state, while also converting it to a boolean in the process:
// Copy the code to the console to see its value
!!''
// Try to double negate the following values too:
// !!false
// !!null
// !![]
The result table for logical NOT is much simpler since we can only have two options:
Expression | Output |
---|---|
!true | false |
!false | true |
Ternary#
These are all the logical operators in JavaScript that we need to know about. There is also a conditional operator we need to cover, called a ternary operator. It's used for conditionally assigning a value to a variable in the following way:
const age = 21;
const eligible = age > 21 ? 'yes' : 'no';
console.log(eligible);
So what does this mean exactly? It means that eligible
should have the value of βyesβ, in case age
is greater than 21. If it's less than 21, it'll have the value of βnoβ. We can also simply assign a comparison to a variable to create a boolean flag. So in the above example, if we only need a true
or false
value for the eligible
, we can simply say the following:
const age = 21;
const eligible = age > 21;
console.log(eligible);
If we need a somewhat more elaborate condition and we still want to use a ternary, we can wrap the condition into parentheses to make use of both a logical and the ternary operator:
const age = 21;
const eligible = (age > 21 && age < 60) ? 'yes' : 'no';
console.log(eligible);
Make sure you donβt overdo writing everything in one line, otherwise your code will quickly become unreadable. Only use ternary for simple conditions. If you need more elaborate logic, use an if
or switch
statement.
Short Circuit Evaluation#
There's one last thing we haven't touched on in this lesson. Short-circuit evaluation, or short-circuiting for brevity, is a technique that uses logical operators to simplify code, make it easier to understand, and can even help you avoid running into type errors in some cases. We can use logical AND and logical OR operators to create short circuits. Take the following as an example:
const useLogs = true;
// Short circuit with logical AND
useLogs && console.log('Logs are enabled');
// The above is equivalent to:
if (useLogs) {
console.log('Logs are enabled');
}
We can see that using a logical AND shortens our code, and with its help, we can avoid using an if statement. Both expressions will achieve the same result. We can also use a ternary operator to introduce an else branch in the following way:
const useLogs = true;
// Using ternary for an else branch
useLogs ? console.log('Logs are enabled') : console.log('Logs are disabled');
// The above can be refactored to:
console.log(useLogs ? 'Logs are enabled' : 'Logs are disabled');
// The above is equivalent to:
if (useLogs) {
console.log('Logs are enabled');
} else {
console.log('Logs are disabled');
}
If the useLogs
variable is true
, it'll execute the first console.log
statement; otherwise, it'll execute the second. Note that we can use a single console.log
statement by moving the variable inside the parentheses. Prefer this solution to reduce code complexity.
We have already seen how the logical OR can be used for short circuits as well during variable assignments:
const uploadedFileName = null;
const defaultFileName = 'default';
// Short circuit with logical OR
const fileName = uploadedFileName || defaultFileName || 'unnamed';
// The above is equivalent to:
if (uploadedFileName) {
fileName = uploadedFileName;
} else if (defaultFileName) {
fileName = defaultFileName;
} else {
fileName = 'unnamed';
}
We can see that using a logical OR operator creates much shorter and simpler code as opposed to using a list of if-else statements. It's readable, less prone to bugs, and easier to test and maintain.
Note that you should only use short circuits for simple expressions. More complex expressions can remain verbose for improved readability.
Test Your Skills!#
To reinforce your knowledge and test your skills, run the following example to execute the tests, then combine the highlighted variables in a way that produces the expected values.
import { expect, it } from 'vitest';
// Test #1:
it('Combine the variables so that it produces "true"', () => {
const a = 1;
const b = true;
const c = false;
// Replace the question marks with the right operator
const result = a ?? b ?? c;
expect(result).toBe(true);
});
// Test #2:
it('Combine the variables so that it produces "false"', () => {
const a = false;
const b = true;
// Replace the question marks with the right operator
const result = !a ?? !b;
expect(result).toBe(false);
});
// Test #3:
it('Combine the variables so that it produces "1"', () => {
const a = true;
const b = 1;
const c = false;
// Replace the question marks with the right operator
const result = a ?? b ?? !c;
expect(result).toBe(1);
});
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews