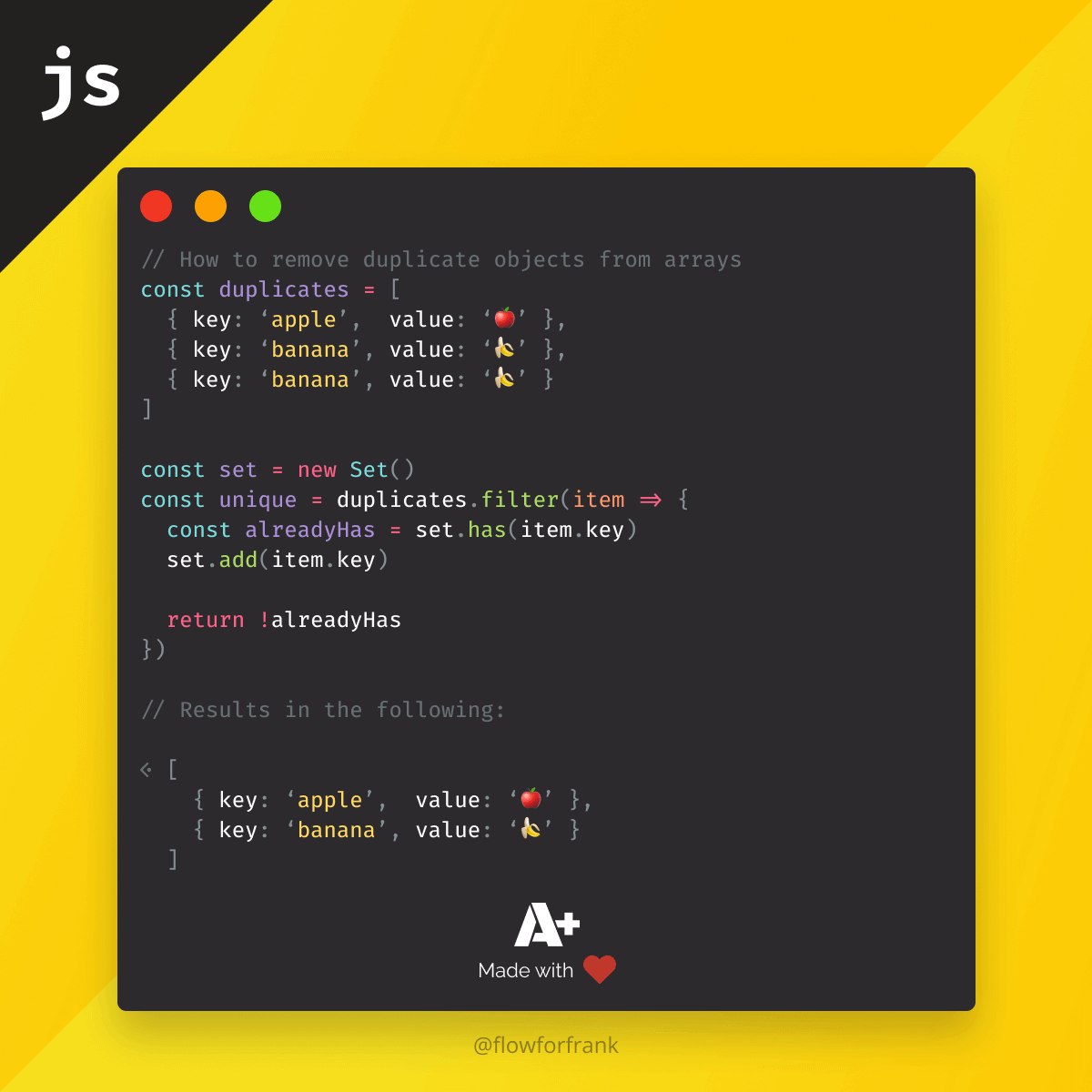
How to Remove Duplicate Objects from Arrays in JavaScript
To remove duplicate objects from arrays in JavaScript, we can use a combination of sets and Array.filter
:
const duplicates = [
{ key: 'apple', value: 'π' },
{ key: 'banana', value: 'π' },
{ key: 'banana', value: 'π' }
]
const set = new Set()
const unique = duplicates.filter(item => {
const alreadyHas = set.has(item.key)
set.add(item.key)
return !alreadyHas
})
This works by filtering through the array and adding one of the properties of the objects to a new set. The reason we are adding this to a new set is that sets are unique by nature. We then filter out those values that have already been added to the set. This way, we end up with an array of unique objects.
Unfortunately, since each object has a different reference, even if their properties match, we cannot simply do something like this:
// β This won't work
[...new Set(duplicates)]
// Because the reference will be different, even if the properties match
{ id: 1 } !== { id: 1 }
You can also copy the below function to your codebase to use it as an exported utility function in other places:
const removeDuplicateObjects = (array, key) => {
const set = new Set()
return array.filter(item => {
const alreadyHas = set.has(item[key])
set.add(item[key])
return !alreadyHas
})
}
// Later call it in your app like so:
removeDuplicateObjects(array, 'key') <- looks for the "key" property
removeDuplicateObjects(array, 'value') <- looks for the "value" property
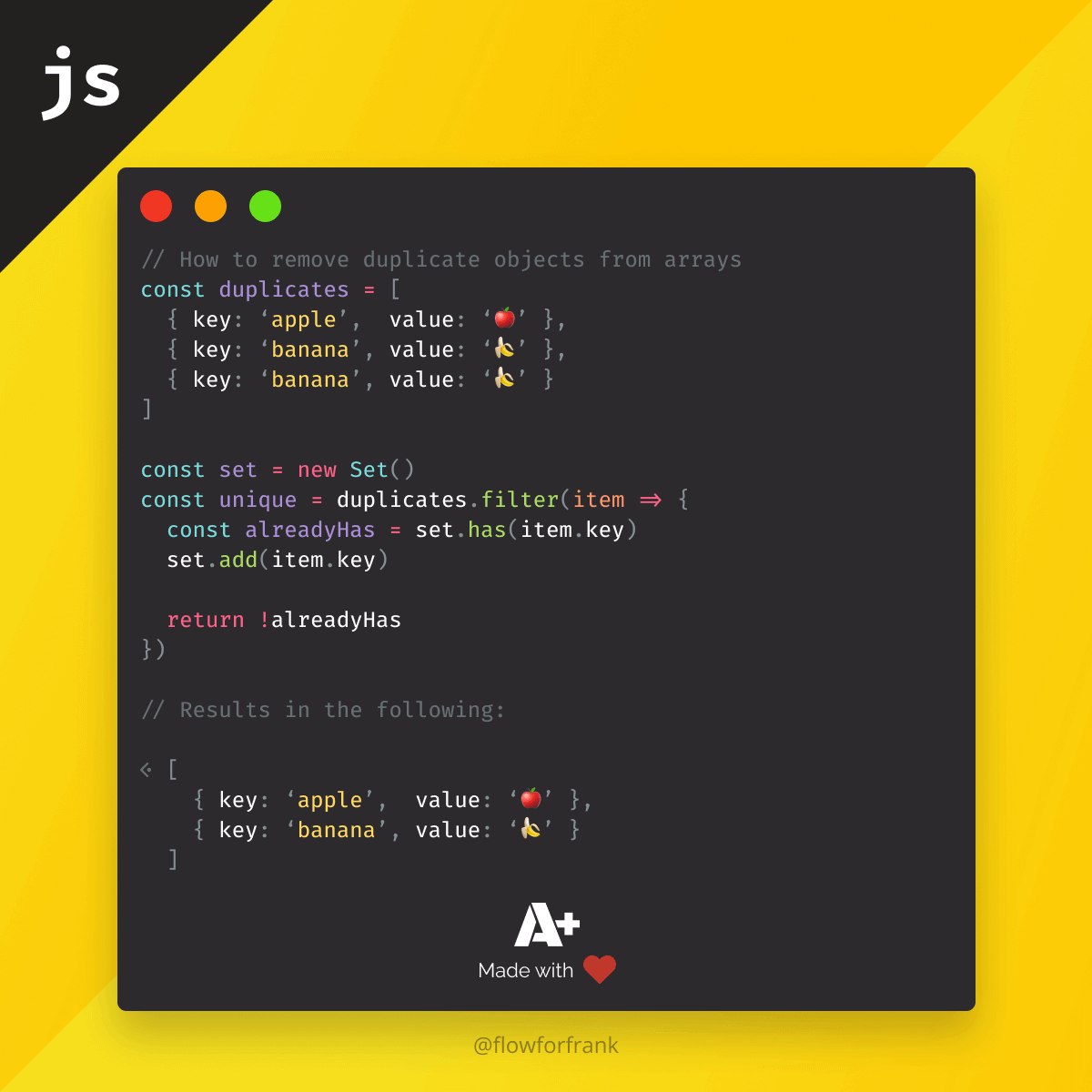
Resources:
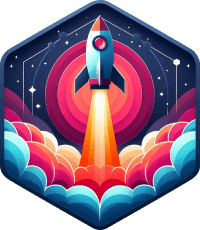
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: