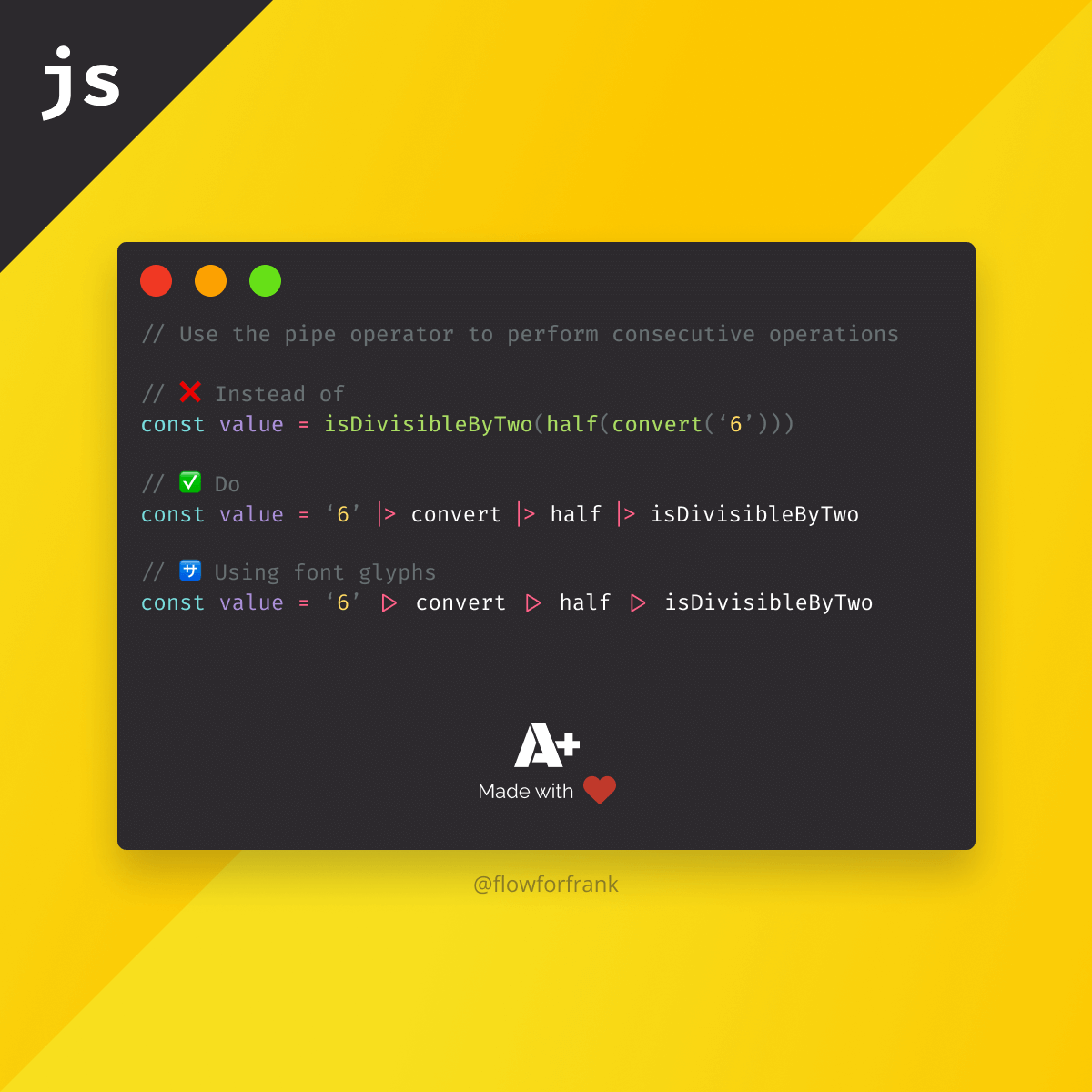
Taking a Look at the New Pipe Operator in JavaScript
The pipe operator (|>) is a new proposal for JavaScript (currently in stage 2) that was introduced in order to simplify how consecutive operations are performed.
The pipe operator (also called a pipeline expression) can be used to pipe the value of an expression into consecutive function calls. The value that is piped is passed as an argument to the functions. The functions are called in order from left to right. This makes function chaining more readable. The expression looks like the following:
expression |> function(%) |> function(%) |> function(%)
The expression also contains a special placeholder that could be used in place of the value. The documentation currently uses %
as the placeholder, but in the final implementation, this token may be different.
Let's see a practical example of how you would use three different functions on a value using nesting, and how you can achieve the same thing using the pipe operator:
const convert = x => Number(x)
const half = x => x / 2
const isDivisibleByTwo = x => x % 2 === 0
// β Instead of
const value = isDivisibleByTwo(half(convert('6')))
// β
οΈ Do
const value = '6' |> convert(%) |> half(%) |> isDivisibleByTwo(%)
The pipe operator reads from left to right. First, we feed the value of "6" to the convert
function, and the output value of the convert
function is passed over to the half
function. This goes on until the end of the pipeline is reached, and the final value is returned.
Why the Pipe Operator was Proposed?
According to the 2020 State of JS survey, the 4th most missed feature from JavaScript was a pipe operator. Currently, there are two ways to handle consecutive operations in JavaScript:
// Nesting
isDivisibleByTwo(half(convert('6')))
// Chaining
'6'.convert().half().isDivisbleByTwo()
Both of them bring their own problems. Nesting quickly becomes hard to read, making maintenance and refactoring hard. Instead of reading from left to right, you need to interpret the code the other way around. Hard readability becomes especially noticeable when you need to work with multiple arguments.
On the other hand, while chaining is much more readable, its use case is somewhat limited. You can only use this style on objects where the methods are available. It's also limited in the way you can handle await
or yield
with it. On the other hand, the new pipe operator would solve these problems.
// function calls
value |> fn(%)
// Arithmetic operators and template literals
value |> % + 1
value |> `${%}`
// arrays and objects
value |> [0, %]
value |> { key: % }
// Await and yield
value |> await %
value |> yield %
How to Try Out the New Pipe Operator
The pipe operator is still in stage 2, meaning it is an experimental feature. However, you can still try it out and use it today by using Babel. If you just want to have a quick look, you can try it out using the online compiler. If you however would like to give it a try in your codebase, you will need to install the following Babel plugin:
npm i --save-dev @babel/plugin-proposal-pipeline-operator
Then, you will need to call the plugin inside your plugins array in your .babelrc
config file:
{
"plugins": [
"@babel/plugin-proposal-pipeline-operator"
]
}
If you would like to find out more about the new pipe operator in JavaScript, make sure you have a read on the proposal page on GitHub for more documentation. What are your thoughts on the new operator? Let us know in the comments below! Thank you for reading through, happy coding! π¨βπ»
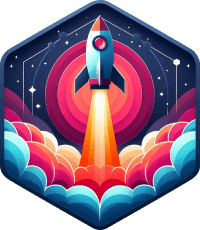
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: