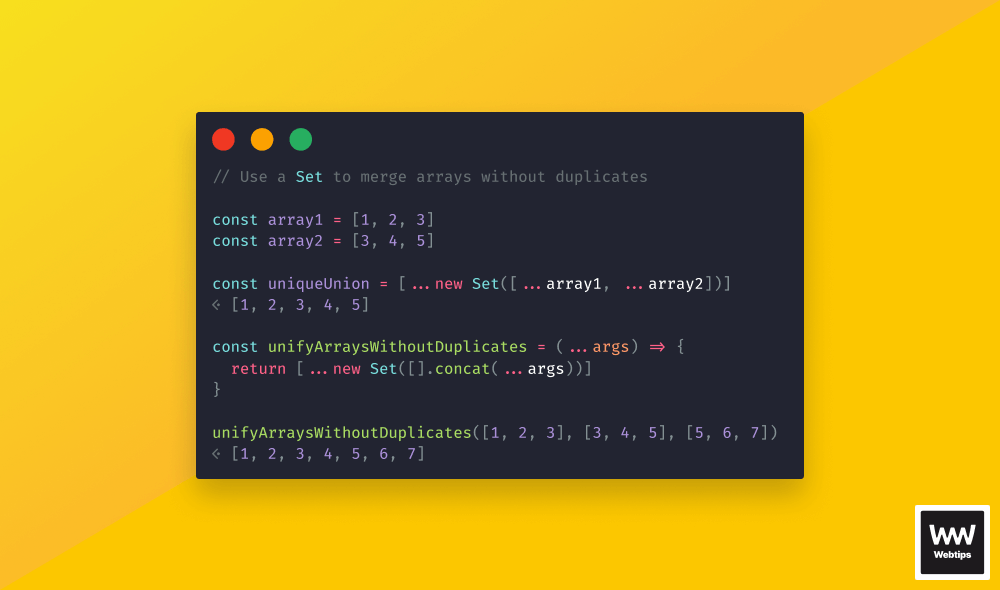
How to Merge Arrays Without Duplicates in JavaScript
There are multiple ways to merge arrays in JavaScript. Usually, we can use Array.concat
or the spread operator. However, none of these methods filter out duplicate values. We could use the filter
method to remove duplicate values after combining the arrays, but there is a cleaner and faster solution using Sets and the spread operator:
const array1 = [1, 2, 3]
const array2 = [3, 4, 5]
// This will return [1, 2, 3, 4, 5]
const merge = [...new Set([...array1, ...array2])]
// --- Explanation ---
// 1. step: use the spread operator the merge the arrays
[...array1, ...array2] -> [1, 2, 3, 3, 4, 5]
// 2. step: wrap the merged array into a set to filter out duplicates
new Set([1, 2, 3, 3, 4, 5]) -> Set(5) {1, 2, 3, 4, 5}
// 3. step: Convert the set back to an array
[...new Set([1, 2, 3, 3, 4, 5])] -> [1, 2, 3, 4, 5]
- Line 9: First, we need to spread the values of the array into a new array. This will merge the two arrays into one with duplicate values.
- Line 12: In the next step, we wrap the created array into a new Set. Since sets can only contain unique values, it filters out duplicates automatically, leaving only unique values in the array.
- Line 15: As the last step, we need to convert the Set back into an array using another spread operator.
Creating a Reusable Function
However, this only works by manually specifying the arrays. What if you don't know the number of arrays you want to combine upfront? We can easily outsource this into a function that we can reuse, which can take in any number of arrays using a rest parameter:
This is done by using concat
on a new empty array. By destructuring the arguments, we essentially merge the different arrays into one and pass it to a Set, which then filters out the duplicate values. By using a rest parameter, we can tell the function that it can accept an indefinite number of arrays.
Other Ways to Merge Arrays
If we don't need to remove duplicates, we can use either the concat array method or the spread operator without a Set:
// Using Array.concat
const array1 = [1, 2]
const array2 = [3, 4]
array1.concat(array2)
// Use concat on multiple arrays
const first = [1, 2]
const second = [3, 4]
const third = [5, 6]
[].concat(first, second, third)
// Using the spread operator
const array1 = [1, 2]
const array2 = [3, 4]
[...array1, ...array2]
- Lines 2-5: We can use the
concat
method on any array and pass a second array as an argument to merge it into the first. This will create a new array. - Lines 8-12: We can use
concat
on an empty array and pass a list of arguments to merge as many arrays as necessary. - Lines 15-18: A cleaner and more modern approach is to use the spread operator (
...
) inside an array. This will spread the values of thearray1
andarray2
variables into a new array, merging the two in the process.
Each of the above solutions will create a new array instead of modifying the original arrays (they don't have side effects). Just as with Sets, we can create a reusable function to merge as many arrays as necessary using the following function:
function merge() {
const array = []
[...arguments].forEach(arg => {
array.push(...arg)
})
return array
}
merge([1]) // returns [1]
merge([1], [2]) // returns [1, 2]
merge([1], [2], [3]) // returns [1, 2, 3]
This works by taking advantage of the special arguments
object, which contains the passed arguments to the function. We can use a simple forEach
to loop through the passed arguments and merge the values into the array variable. Note that we need to define the function using the function
keyword, as the arguments object is not available on arrow functions.

Summary
In summary, use a Set with the spread operator in case you need to merge arrays without duplicates. For merging arrays while keeping duplicate values, we can use the concat
method or the spread operator without a Set.
Is there anything else you'd like to know about merging arrays in JavaScript? Let us know in the comments below! Thank you for reading, and happy coding! To learn more about JavaScript, continue with our guided roadmap:
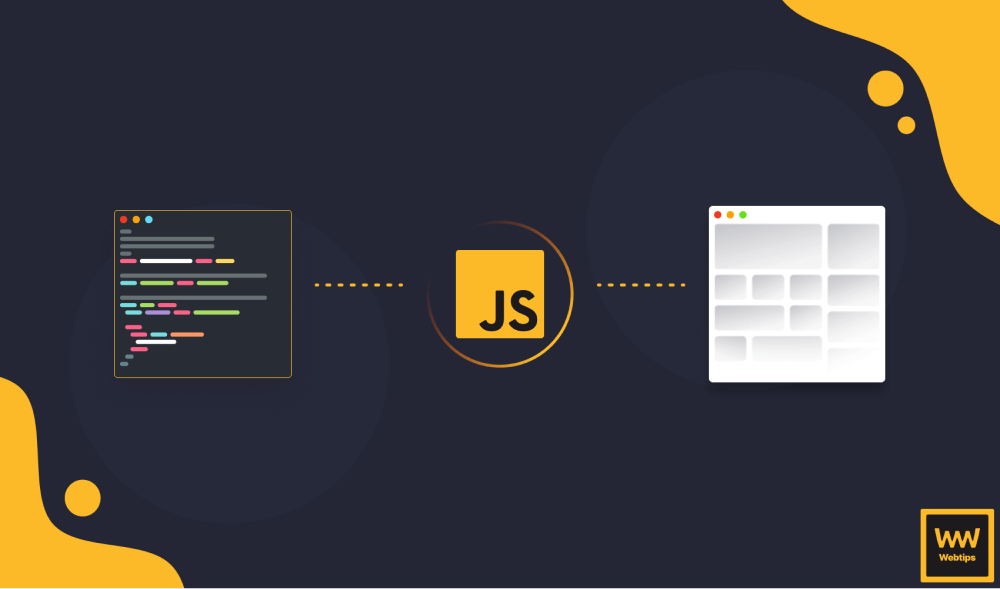
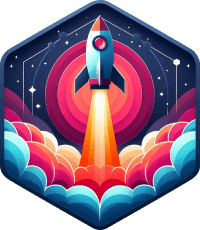
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: