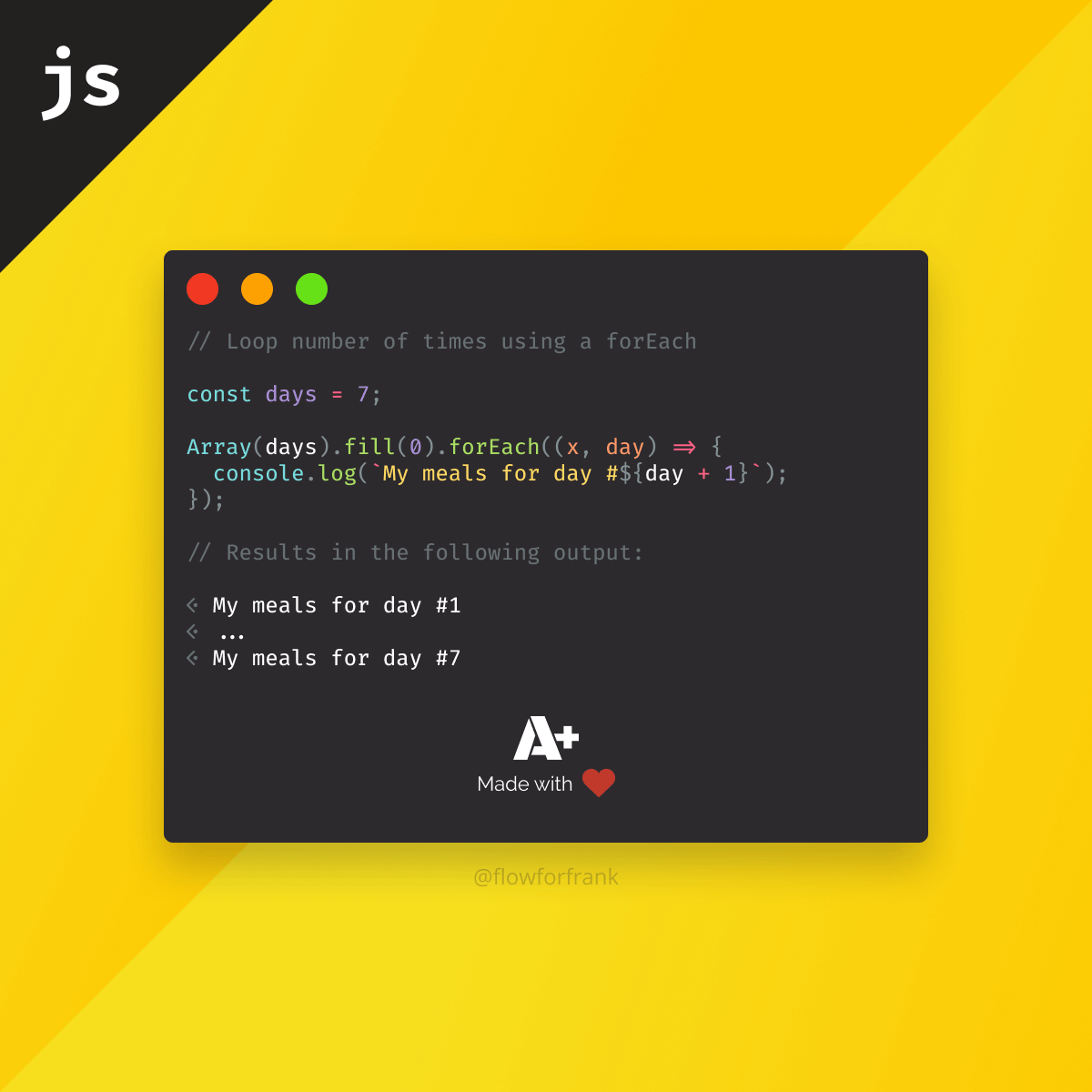
How to Loop Number of Times in JavaScript
The general way to loop x
number of times in JavaScript is using a simple for
loop, where you can define how many times you want to loop over something:
const loopTime = 5
for (let i = 0; i < loopTime; i++) {
console.log(`Iteration is #${i}`)
}
let i = 0
: The first statement is executed before the loop just once. This is where we define a counter, usually named byi
. Here we say thati
should be equal to 0.i < loopTime
: The second defines the condition for how long the loop should run. Here we can set the number of loops. In the above example, it means the code should be executed as long asi
is less thanloopTime
. Essentially, this means that we want the code to be executed 5 times.i++
: The third is executed every time after the block of code inside the curly braces has been executed. It means that we want to incrementi
by one. It's the same as sayingi = i + 1
. This way, the condition will be met in the second statement afteri
becomes 5.
Loop Number of Times Using Functions
Using a for
loop works just fine and is the most performant and simplest solution of all, but we can make things a bit more readable using functions. To loop a number of times using a function, we can introduce a loop
function that executes a for
loop internally:
const loop = (times, callback) => {
for (let i = 0; i < times; i++) {
callback(i)
}
}
Using the above function, we can loop over things over a predefined number of times. Note that we have passed the i
variable to the callback
function, so that whenever we call the loop
function, we'll have access to the current loop's iteration. We can use the loop
function in the following way:
loop(5, i => {
console.log(`Iteration is #${i}`)
})
Improving Loop Readability
We can further make things more readable, by using a helper array instead of the for
loop:
const loop = (times, callback) => {
Array(times).fill(0).forEach((item, i) => callback(i))
};
This will work just like using the for
loop. If you call the loop
function with a value of 5, it'll create an array of 5 with zeroes inside it, so we actually have something to loop over.
Note that in terms of performance, using for
loops will win over using array methods.
Using the spread operator
We can further shorten this code by using the spread operator and omitting the fill
method:
const loop = (times, callback) => {
[...Array(times)].forEach((item, i) => callback(i));
};
The reason we don't need to use fill
this time is because we get an array with the values of undefined
, instead of empty x 5
, meaning we'll have the correct length without having to use fill
. Changing callback(i)
to callback(i + 1)
, we can start the counter from 1
instead of 0
if necessary. You can try out the function with the editor below:

Resources
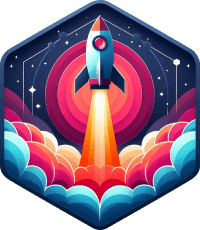
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: