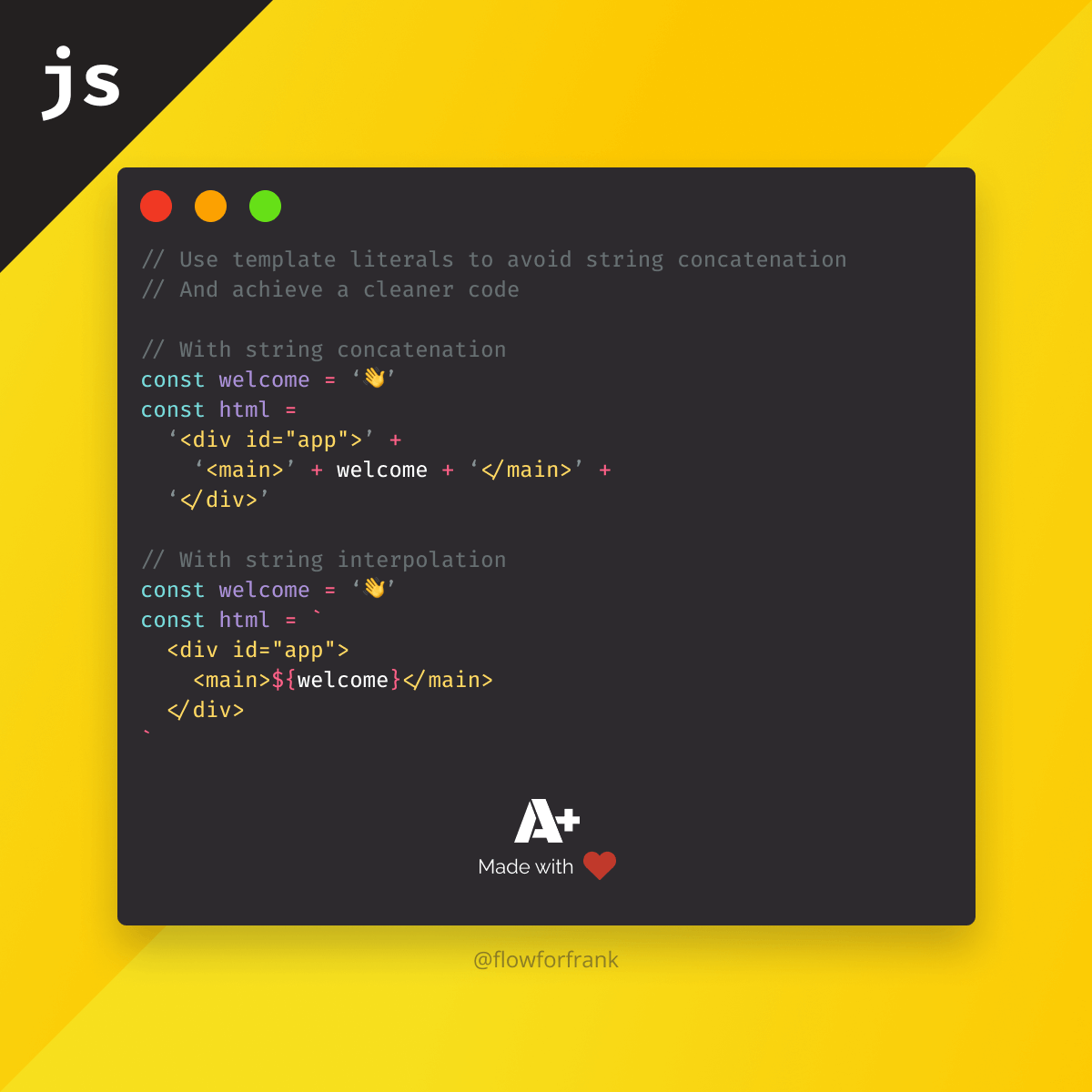
Javascript String Interpolation vs Concatenation
String interpolation and concatenation are two different ways in JavaScript to connect strings together. Originally, string concatenation was used, then ES6 introduced string interpolation. Note the difference between the two:
// Using string concatenation
const welcome = 'π'
const html =
'<div id="app">' +
'<main>' + welcome + '</main>' +
'</div>'
// Using string interpolation
const welcome = 'π'
const html = `
<div id="app">
<main>${welcome}</main>
</div>
`
In the first example, we used string concatenation. We can concatenate multiple strings together in JavaScript using a plus sign. We can also insert variables inside the string this way.
In the second example, we used string interpolation to insert the variable. This is called a template literal. Notice that to use template literals, we need to use backticks. This has the added advantage of writing multiline strings without the need to use any plus signs. Variables can also be inserted into the string more intuitively, and we don't need to worry about escaping quotation marks.
To insert variables (or expressions), we can use a dollar sign followed by curly braces. Anything that goes inside the curly braces will be executed. Therefore, we could just as well insert expressions inside (or even call functions). For example, we could do the following:
`<main>${1 + 1}</main>` // Will be evaluated to 2
`<main>${injectApp()}</main>` // Calling a function
Tagged Template Literals
We can also combine template literals with functions to create tagged template literals. This looks like the following:
const tagged = (string, ...values) => {
// Do whatever is needed with the string and the passed expressions
console.log(string, values);
}
tagged`You can call the function without parentheses and with params: ${1}, ${2}`
Notice that we can omit the parentheses when calling the function. Instead, we can call the string right after the function's name. This function expects the string itself as the first parameter, and we can use the spread operator to pass as many values as the second parameter as we would like (the values will be stored inside an array). This will produce the following:
(3) ['You can call the function without parentheses and with params: ', ', ', '', raw: Array(3)] (2) [1, 2]
On a final note, as using string interpolation is easier to read and maintain, it is recommended to use template literals over to string concatenation. Want to learn more about strings? Check out our other webtips on JavaScript.
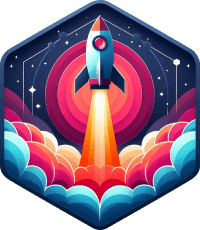
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: