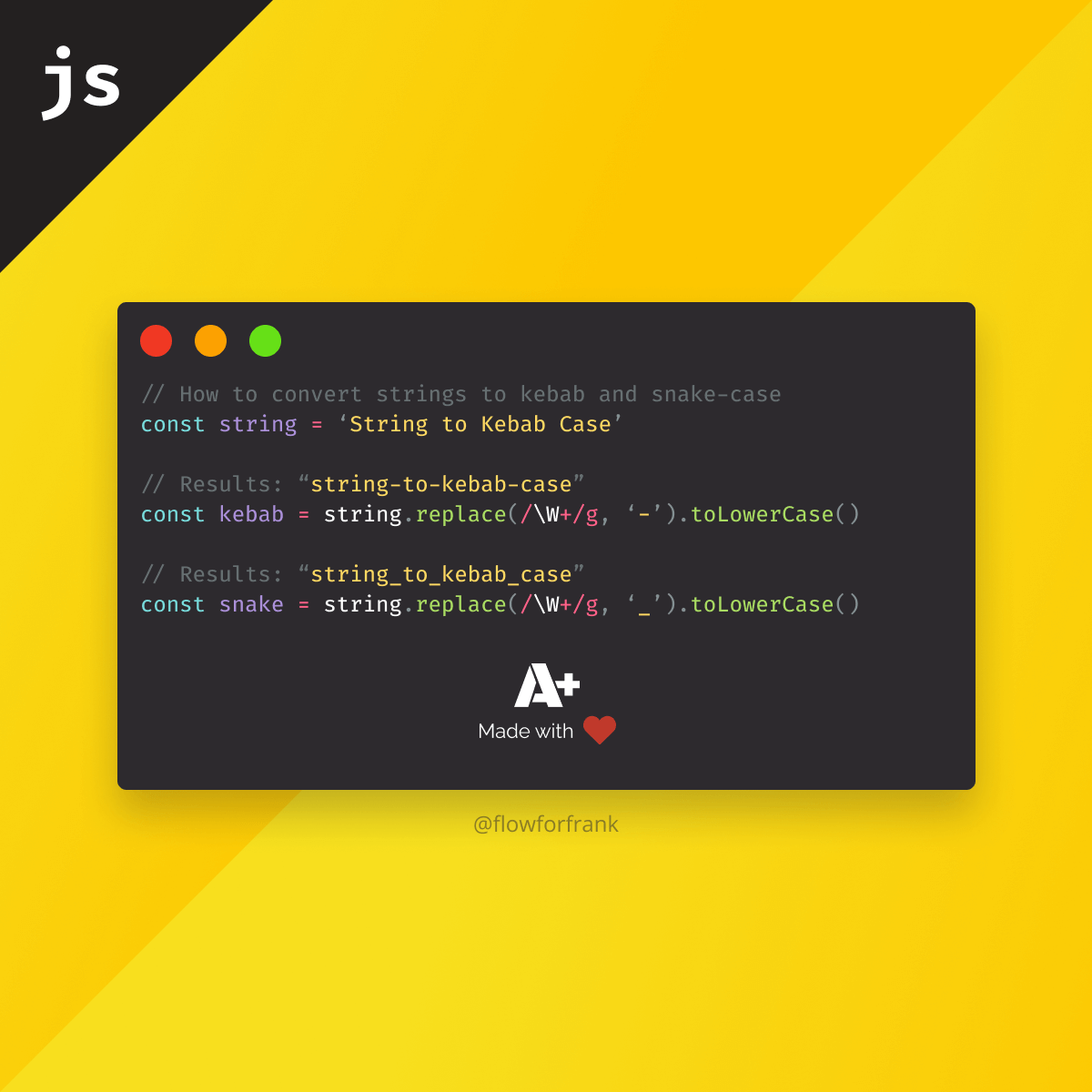
JavaScript Lowercase and Replace Spaces
We can use a combination of .replace
with a regex and .toLowerCase
to convert a string into lowercase and replace spaces as well in the following way:
const string = 'String to Kebab Case'
// Results: βstring-to-kebab-caseβ
const kebab = string.replace(/\W+/g, '-').toLowerCase()
// Results: βstring_to_kebab_caseβ
const snake = string.replace(/\W+/g, '_').toLowerCase()
There are two things to note here for the regex:
- First, we used the "not word" selector, which will match any character that is not a word. (notice that we have used a plus sign to match 1 or more words)
- Secondly, we used the
g
(global) flag to match more for multiple results.
This will select the whitespaces, which then we can replace with either a dash or an underscore, depending on whether we want to go with kebab-case or snake-case. This also works with multiple whitespaces, as well as other signs that should be stripped from the end string, such as the following:
'String with multiple spaces' -> 'string-with-multiple-spaces'
'A & B' -> 'a-b'
Hence, it is more flexible than using the whitespace character (\s
). Did you know you can also use variables inside regular expressions to create dynamic expressions? Check out the following tutorial:
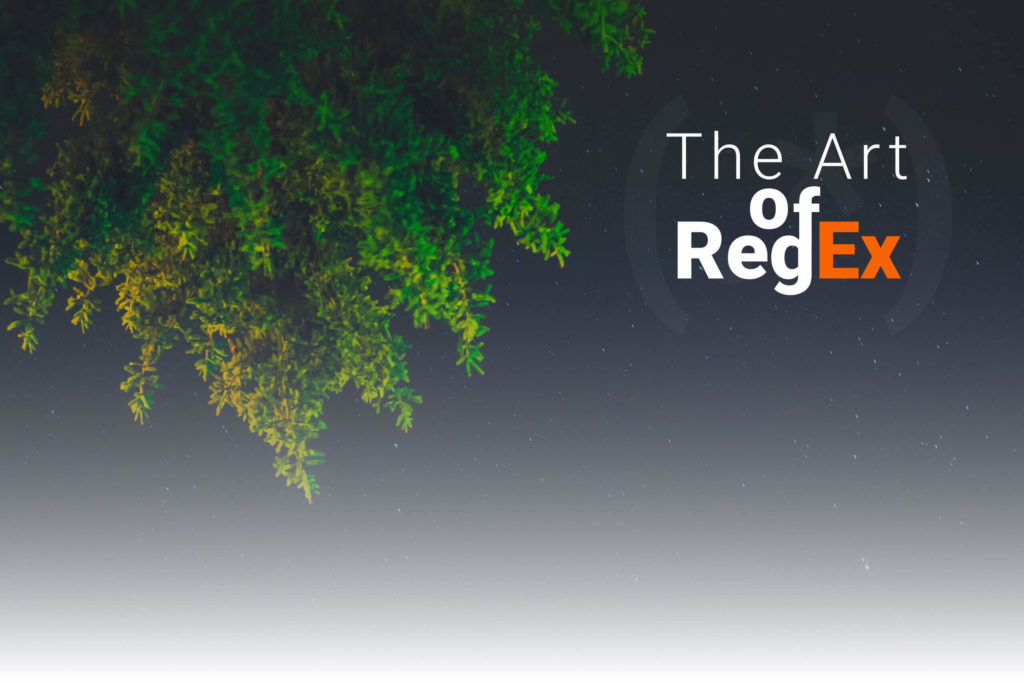
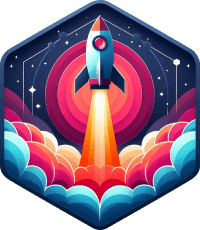
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: