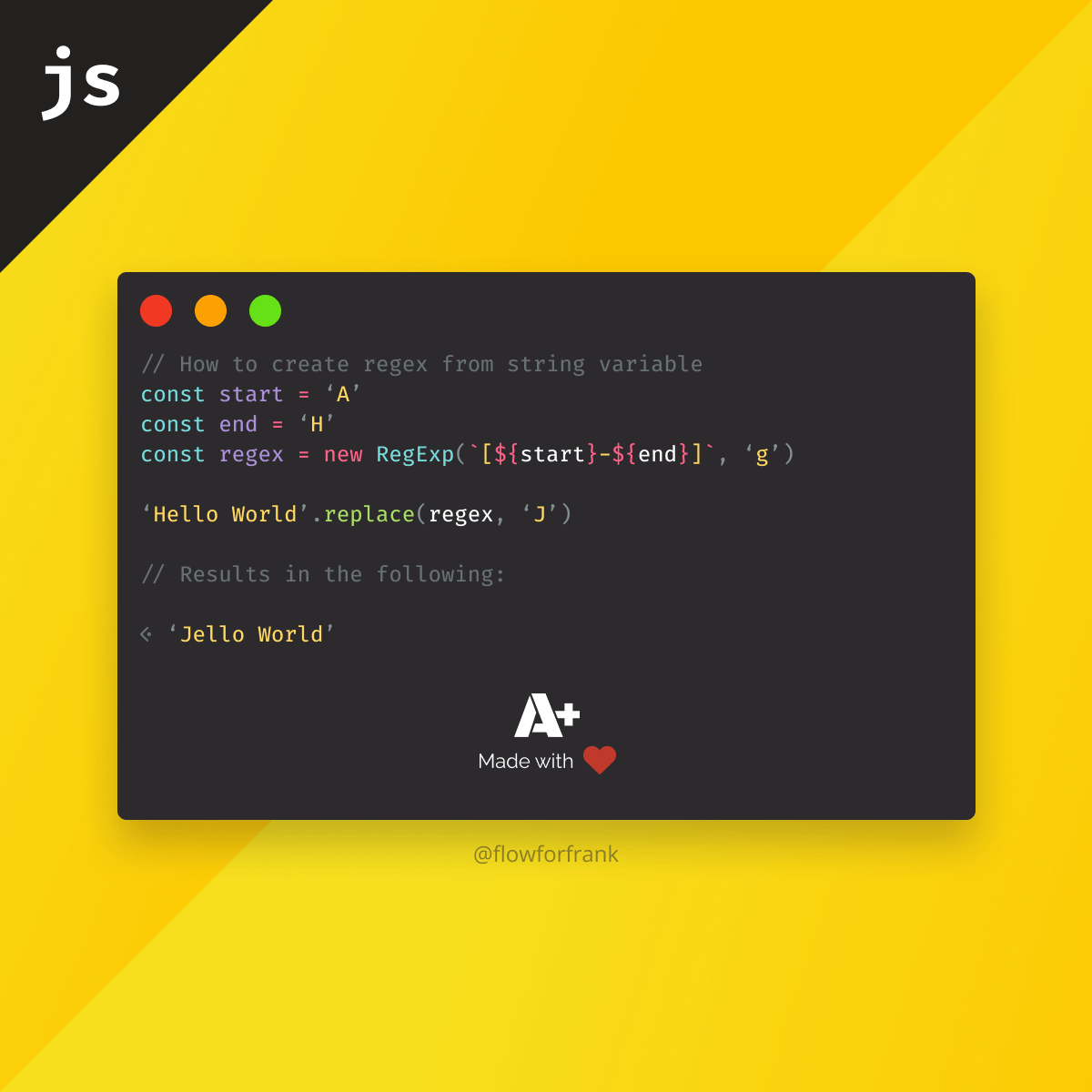
JavaScript Create Regex From String Variable
To create a regex from string variables in JavaScript, we can use a new RegExp
object to pass a variable as a regex pattern:
const string = '[A-Z]'
const regex = new RegExp(string, 'g')
'Hello World'.replace(regex, '_')
The RegExp
object takes in the regular expression as the first argument, while for the second argument, we can pass flags to be used with the expression, such as:
g
: for matching patterns globally (match pattern multiple times)i
: for case insensitive matchingm
: for matching multiple linesu
: for unicode support
In the above code example, we have used g
to match both the letter "H" and the letter "W". If we were to omit the global flag, only the first uppercase letter would be matched.
Using variables, we could also combine multiple variables using string interpolation to get a more dynamic behavior, for example:
const startLetter = 'A'
const endLetter = 'H'
const regex = new RegExp(`[${startLetter}-${endLetter}]`, 'g')
'Hello World'.replace(regex, 'J')
In this example, only the letter "H" will be replaced, as we essentially created a regular expression that spans from "A" to "H" ([A-H]
). We could also use an array of strings to create more elaborate expressions, such as a dynamic regular expression pattern for a blacklist:
const blacklist = [
'suspicious-domain',
'untrusty-site',
'devious-page'
]
const regex = new RegExp(blacklist.join('|'), 'gi')
const isBlacklisted = regex.test(document.location.href)
Here we join the array of strings together using a pipe, which will result in the following expression that can be used to match multiple strings:
suspicious-domain|untrusty-site|devious-page
This can be easily expanded by simply adding new strings to an array to match against more patterns. Notice that you can pass multiple flags to the RegExp
object. Here, both the global and case insensitive flags were passed.
Would you like to learn more about how regular expressions work in JavaScript? Make sure to check out the tutorial below which goes into more details.
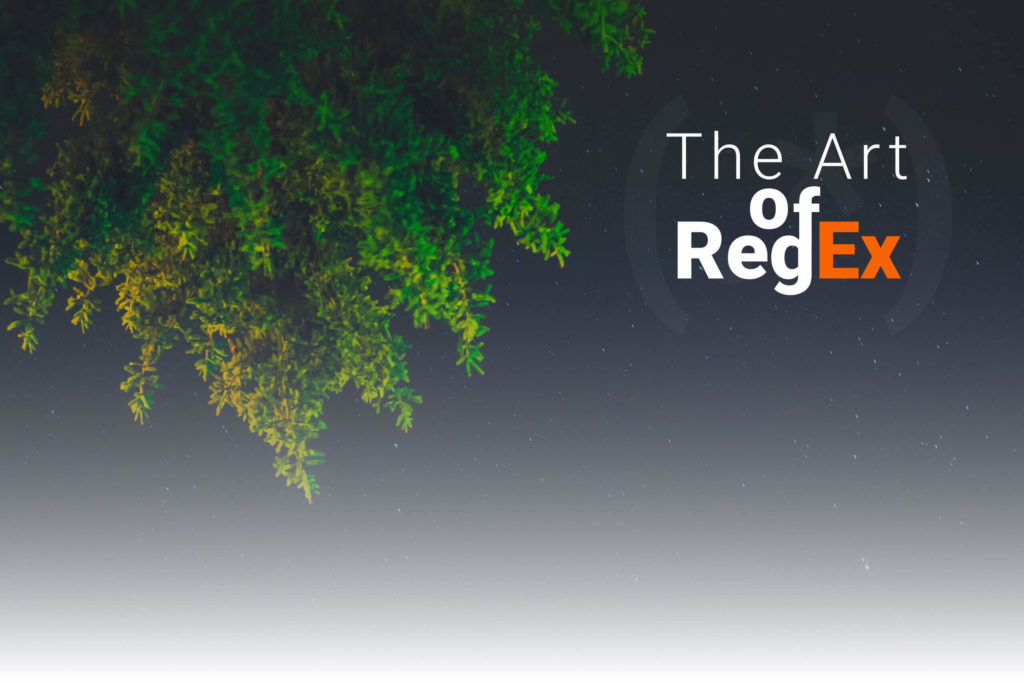
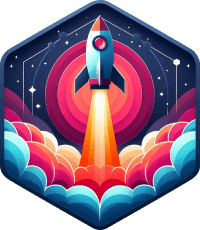
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: