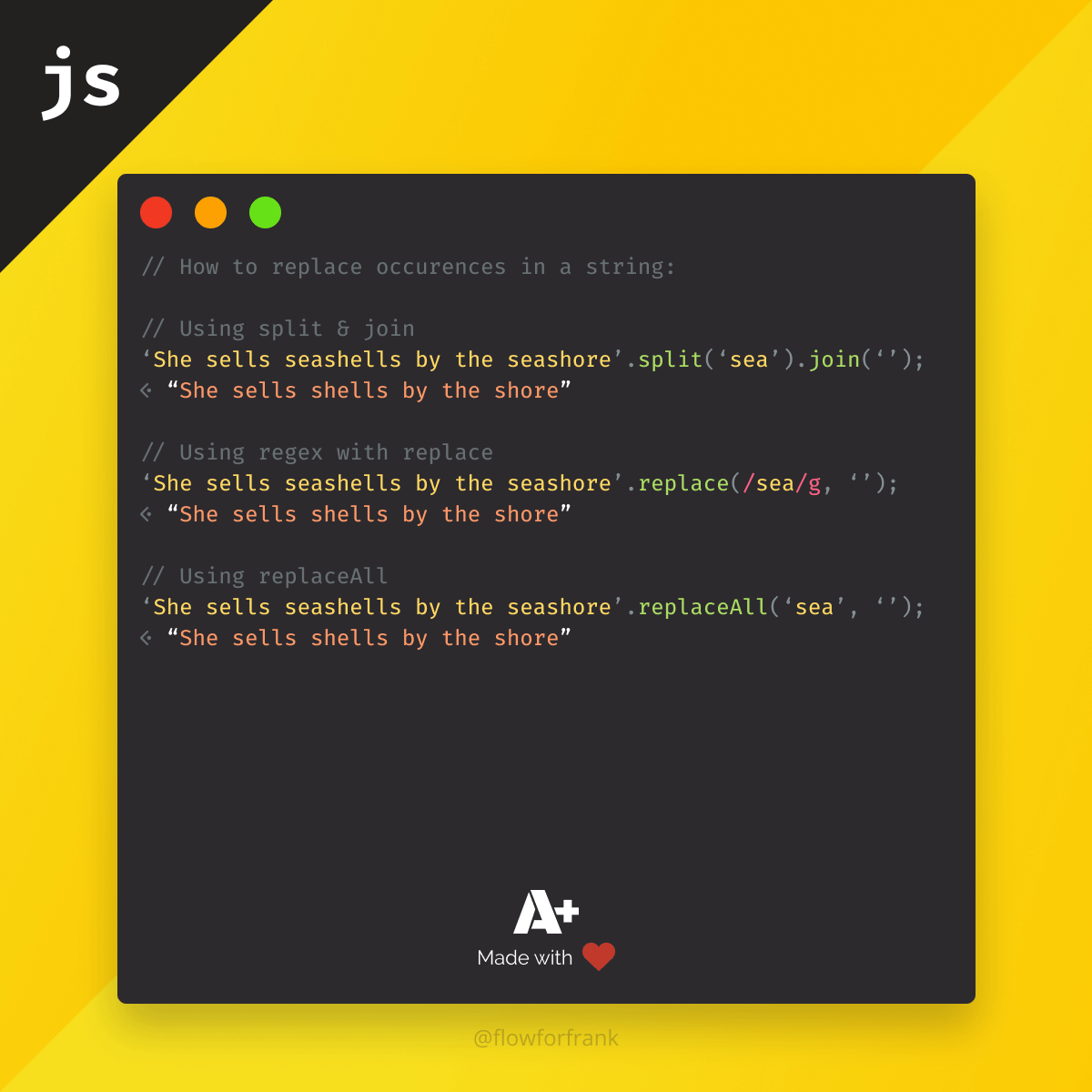
How to Replace All Occurrences of a String in JavaScript
In JavaScript, there are many different ways to do the same thing. Here are three different approaches to replace, or completely remove all occurrences in a string.
Using split & join
string.replace(search).join(replacement);
// eg.:
// Returns "She sells shells by the shore"
'She sells seashells by the seashore'.split('sea').join('');
This works because the string is split into three parts using the string "sea", which results in the following array:
'She sells seashells by the seashore'.split('sea');// Returns:(3) ["She sells ", "shells by the ", "shore"]
This is then joined together with an empty string using join
, essentially removing the string.
Using regex with replace
You can also use a regular expression, using the replace
method:
// Return "She sells shells by the shore"
'She sells seashells by the seashore'.replace(/sea/g, '');
Note that in order to replace every occurrence, you need to pass the global flag to the regex. Apart from the global flag, you can also use an i
to indicate you want matches to be case insensitive.
'No lemon, no melon'.replace(/no/g, ''); // Returns "No lemon, melon"
'No lemon, no melon'.replace(/no/gi, ''); // Returns " lemon, melon"
Note the difference between using a case insensitive flag, and omitting it.

Using the replaceAll method
Although global support is lacking completely for IE, and older versions of other browsers, it still has relatively wide support according to caniuse.com. This can be used to quickly and easily replace, or remove all occurrences in a string without using a regex.
// Return "She sells shells by the shore"
'She sells seashells by the seashore'.replaceAll('sea', '');
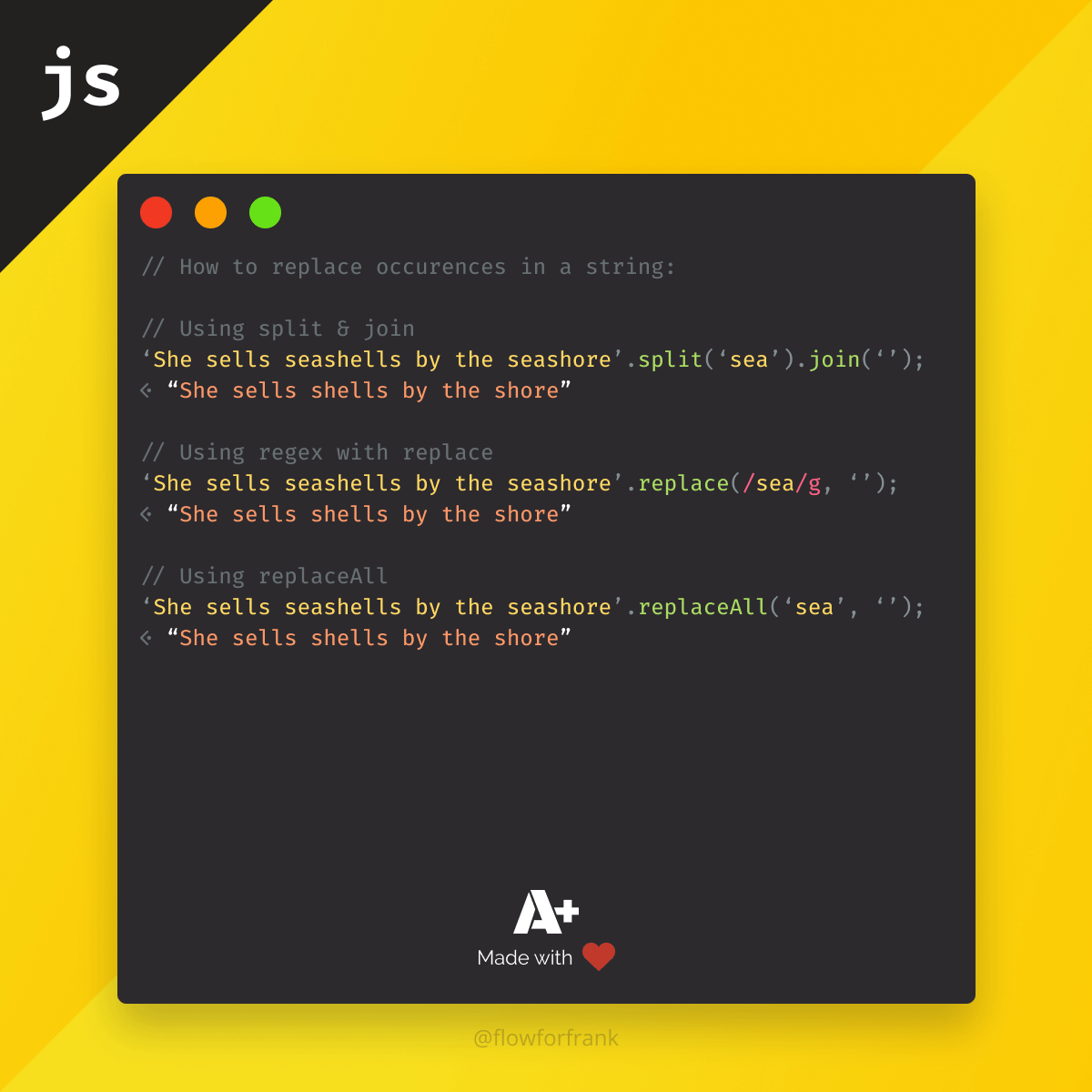
Resources:
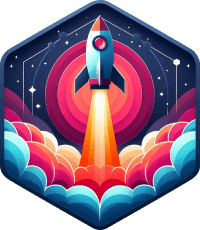
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: