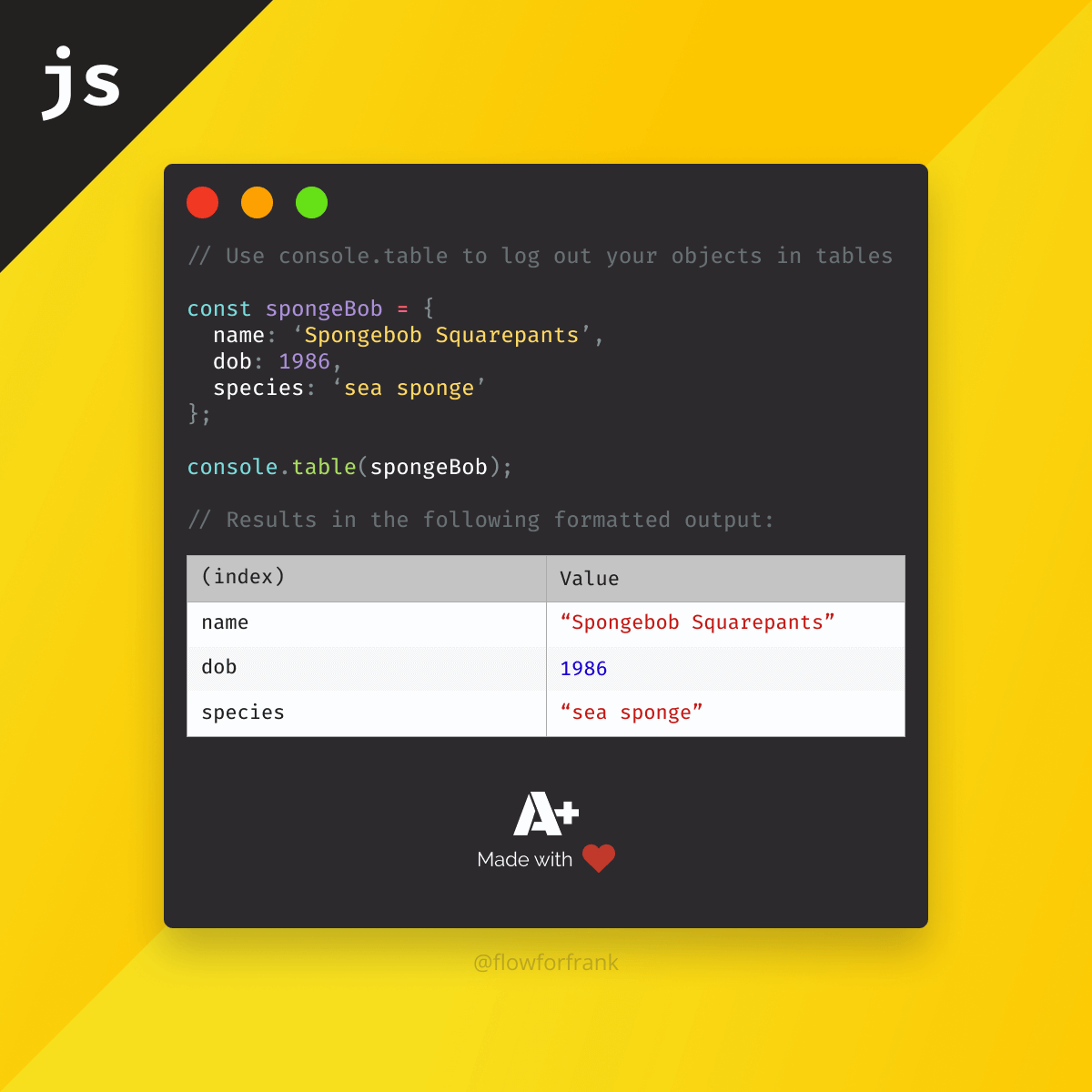
How to Pretty Print Objects in JavaScript
Ever had difficulties to navigate through complex objects in JavaScript during debug? - console.table
might be just what you're looking for.
With console.table
, you can display the passed data β which can be either an array or an object, or a combination of both β in a table.
// You can display arrays in a table
console.table('π', 'π', 'π', 'π');
// You can also display objects
const animals = [
{ icon: 'π', name: 'Pig' },
{ icon: 'π', name: 'Ox' },
{ icon: 'π', name: 'Ram' },
{ icon: 'π', name: 'Goat' },
];
console.table(animals);
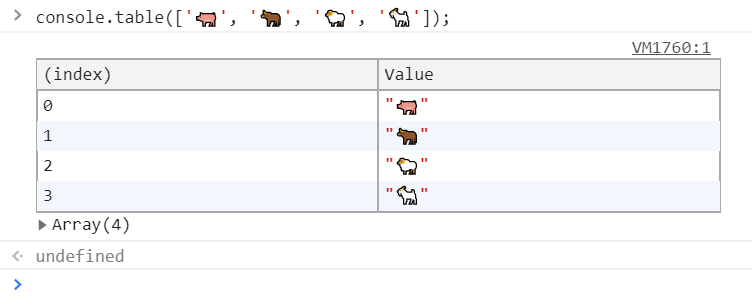
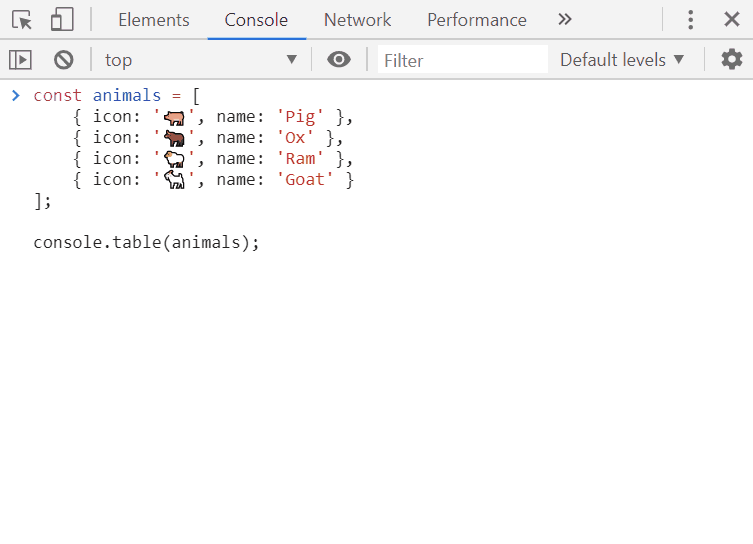
Besides displaying data, you can also pass a second argument to console.table
. It must be either an array or an object that can be used to restrict which columns to display. The below example will only display the name of the animals.
const animals = [
{ icon: 'π', name: 'Pig' },
{ icon: 'π', name: 'Ox' },
{ icon: 'π', name: 'Ram' },
{ icon: 'π', name: 'Goat' },
];
// Restrict to only display names
console.table(animals, ['name']);
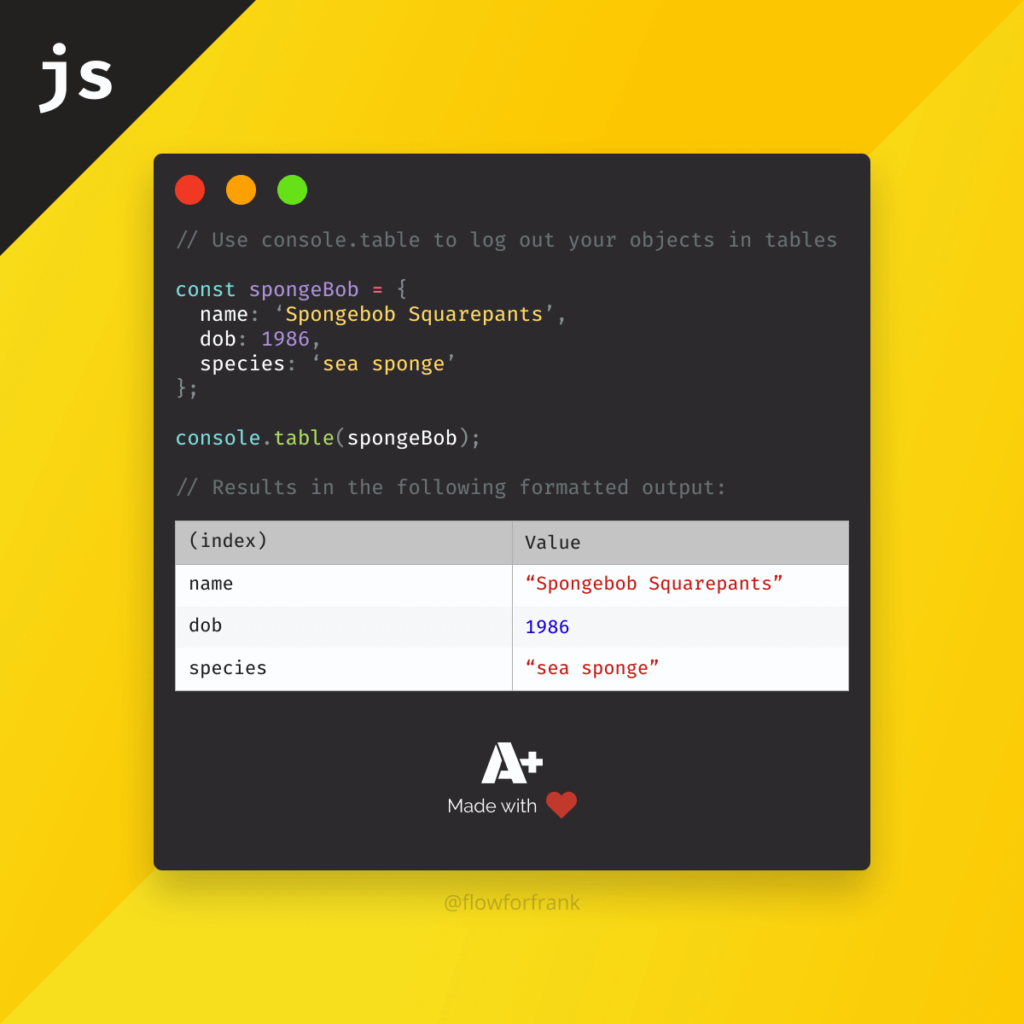
If you are also interested in how you can format stringify JSON outputs, check the article below:
How to Format JSON in JavaScript
Resources
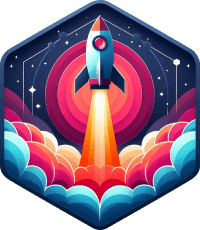
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: