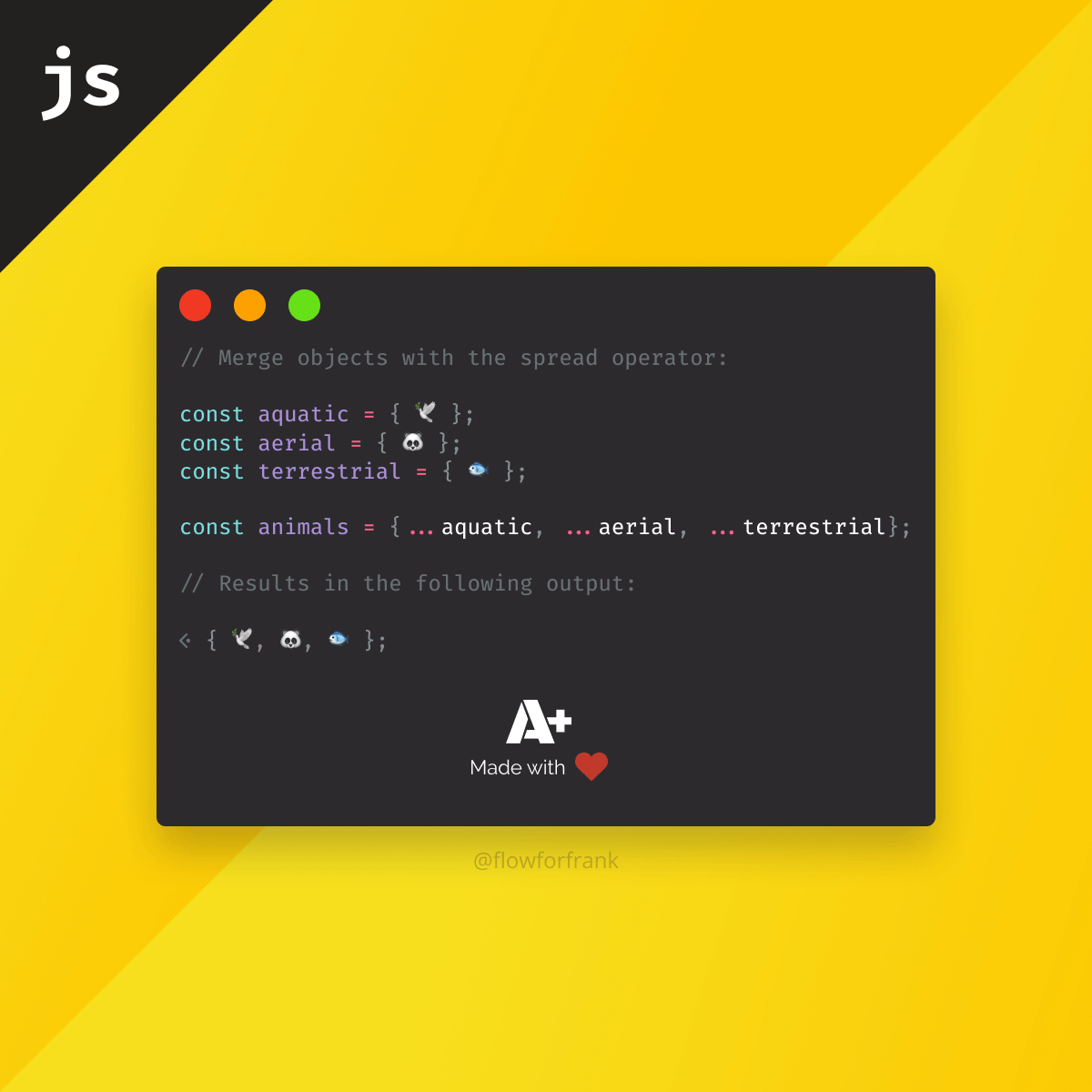
How to Merge Objects in JavaScript
From ECMAScript 2018 you can merge multiple objects together using the spread operator:
Copied to clipboard! Playground
// Merge objects with the spread operator:
const aquatic = { dove: 'π' };
const aerial = { panda: 'πΌ' };
const terrestrial = { fish: 'π' };
const animals = { ...aquatic, ...aerial, ...terrestrial };
// Results in the following output:
{
dove: 'π',
panda: 'πΌ',
fish: 'π'
}
But what if you try to merge two object which has the same property?
Copied to clipboard! Playground
const aquatic = { dove: 'πΌ' };
const aerial = { dove: 'π' };
const animals = { ...aquatic, ...aerial };
// Results in the following output:
{
dove: 'π'
}
The latter will rewrite the previous. If you don't have access to the spread operator in objects, you can also use Object.assign
:
Copied to clipboard! Playground
const aquatic = { dove: 'π' };
const aerial = { panda: 'πΌ' };
const terrestrial = { fish: 'π' };
// There is no limit to the amount of objects you can merge together
Object.assign(aquatic, aerial, terrestrial);
// If you want to copy everything into a brand new object,
// set the first param to an empty object:
Object.assign({}, aquatic, aerial, terrestrial);
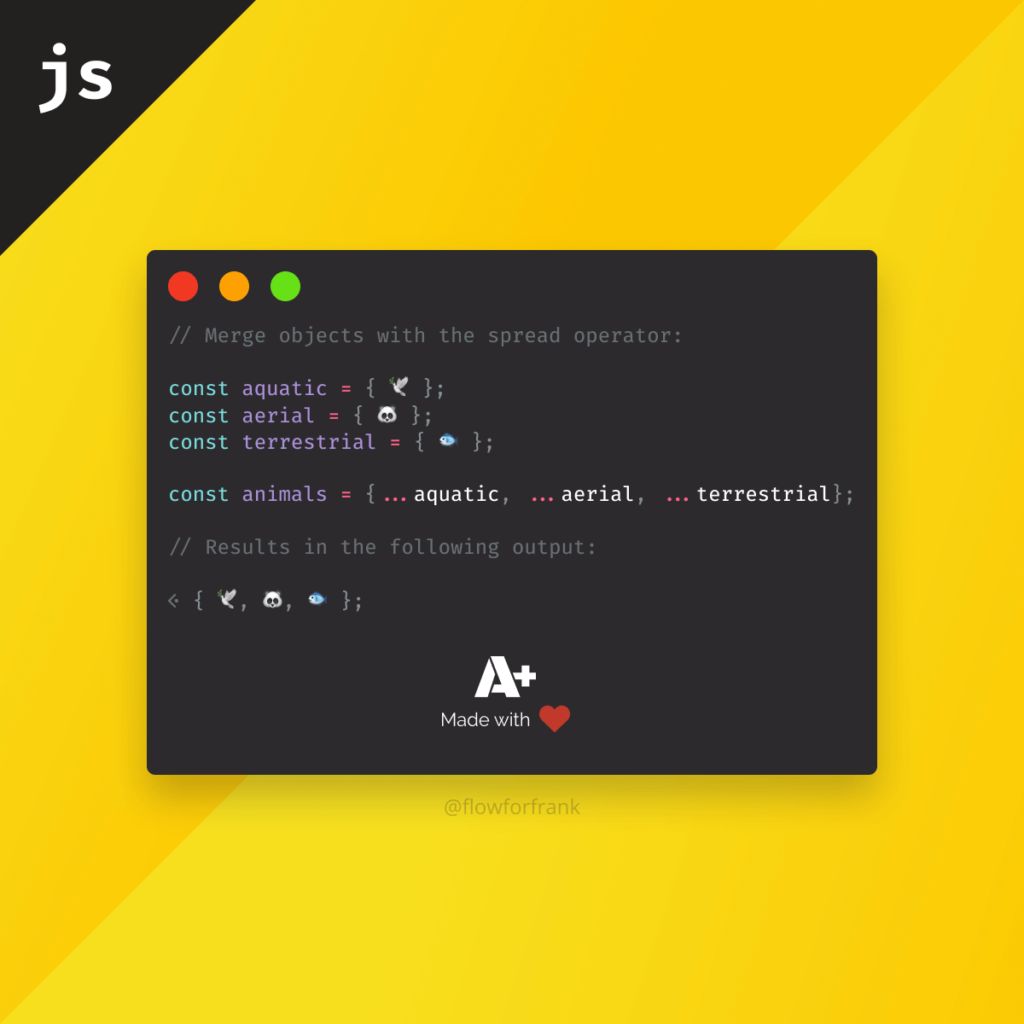
Resources:
π More Webtips
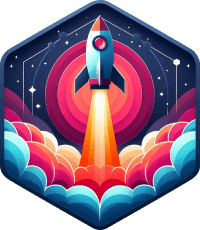
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: