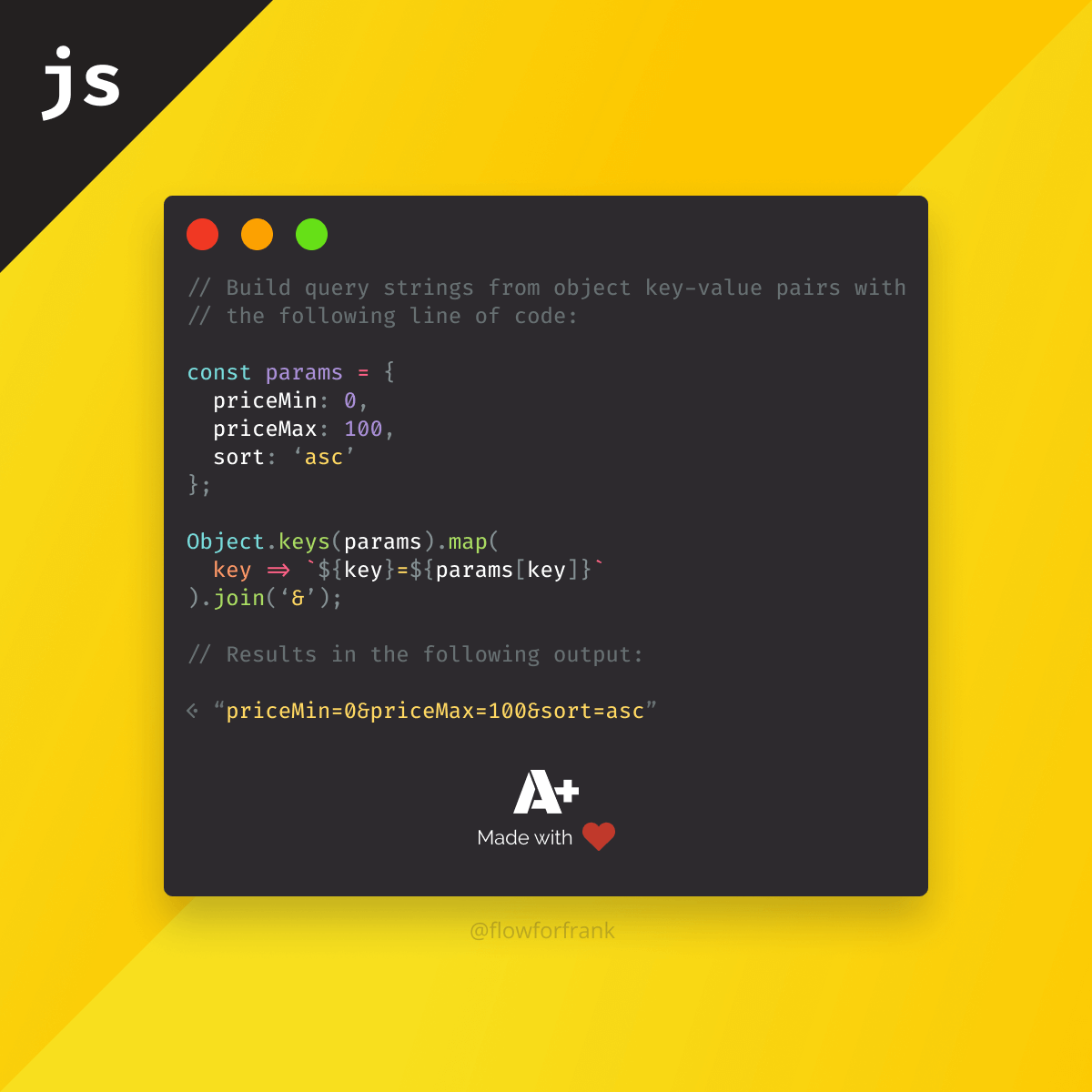
How to Make Query Strings From an Object in JavaScript
In JavaScript, you can generate query strings from an object's key-value pairs using a one-liner:
// Build query strings from object key-value pairs with
// the following line of code:
const params = {
priceMin: 0,
priceMax: 100,
sort: 'asc'
};
Object.keys(params).map(key => `${key}=${params[key]}`).join('&');
// Results in the following output:
"priceMin=0&priceMax=100&sort=asc"
You only need to use Object.keys
combined with a map
and join
. If you also need is a function, just copy the code below:
const querify = object => Object.keys(object).map(key => `${key}=${object[key]}`).join('&');
querify({
name: 'Patrick',
image: 'β'
});
How Does it Work?
To fully understand how it is working, let's go step by step and pick the function into pieces:
- First, we pass the object to
Object.keys
. This will return an array with each key name. - Next, we pass it to a
map
function, that transforms each of the array's element into a string, namely the key of the object equal to its value.
(${key}=${object[key]}
) β‘οΈ "name=Patrick" - Using
join
, these key-value pairs are joined together using the & sign.
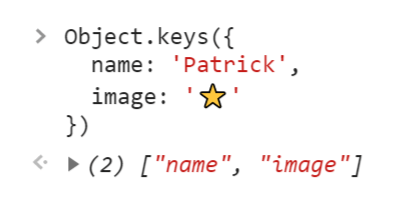
Want an Even Shorter Solution?
You can get things done even faster by using the URLSearchParams object:
new URLSearchParams({ priceMin: 0, priceMax: 100, sort: 'asc'}).toString();
You just need to convert it to a string afterwards.

Using jQuery
If you are still using jQuery, you can also achieve the same thing by using jQuery.param:
jQuery.param({ name: 'Patrick', image: 'β'});
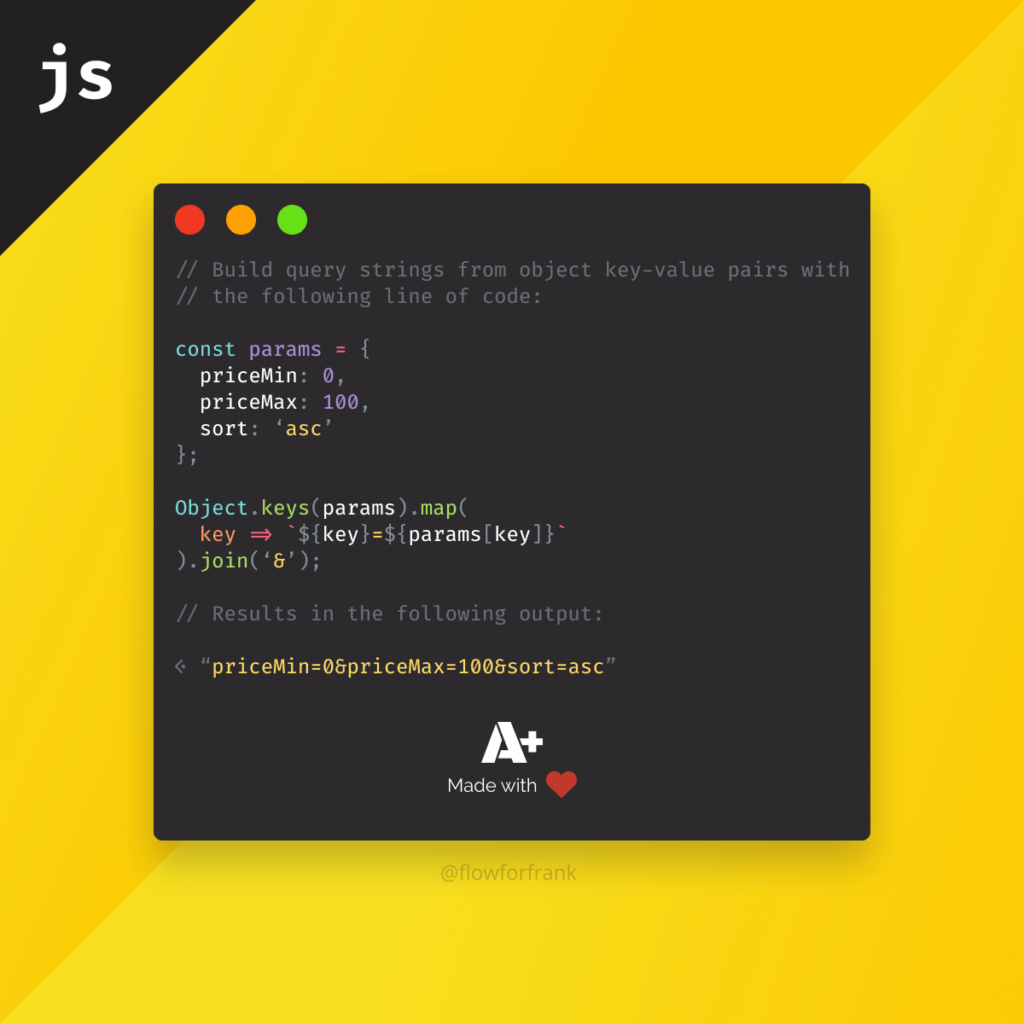
Resource
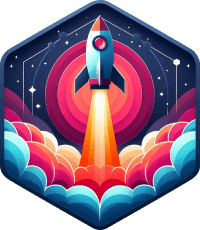
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: