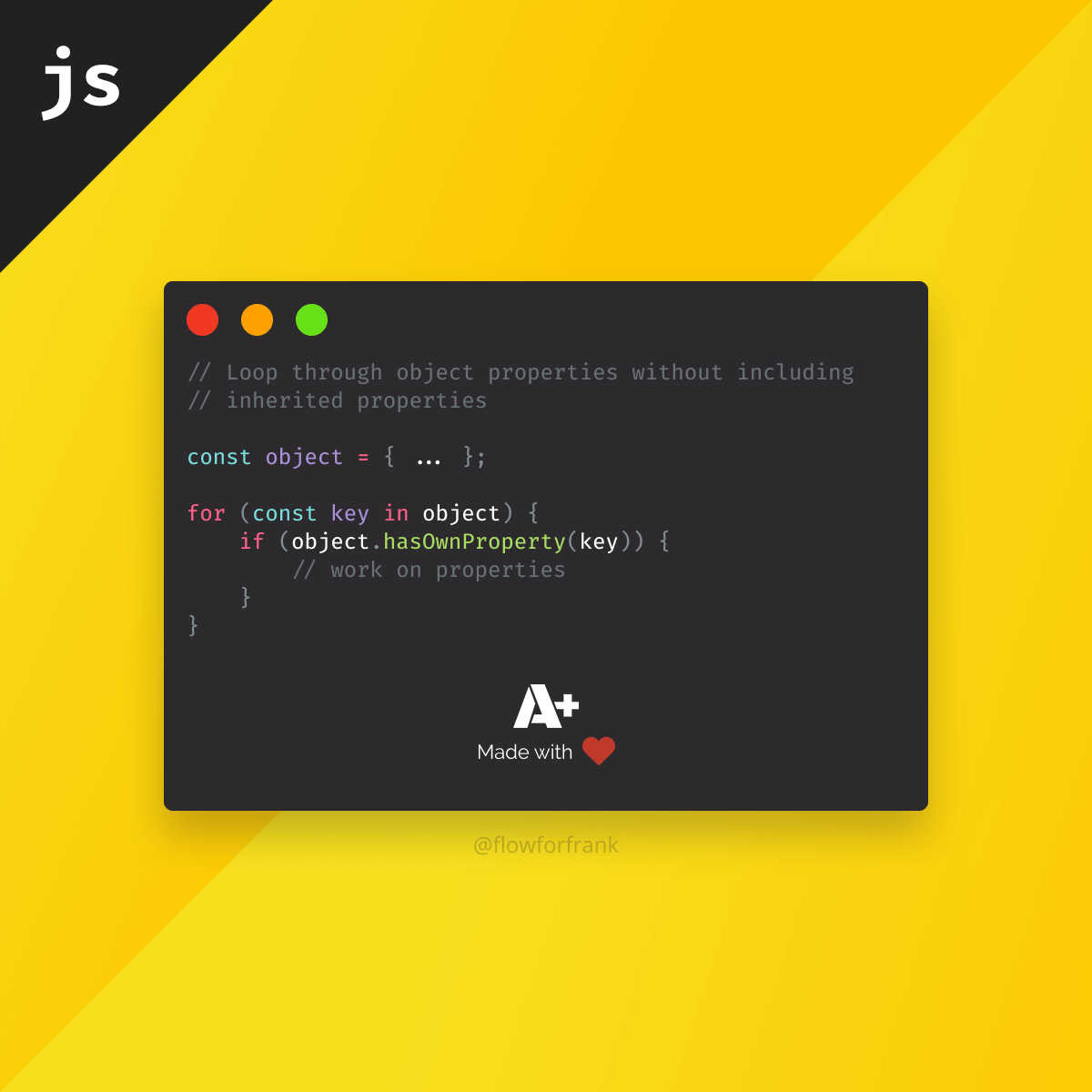
How to Loop Through Properties of an Object in JavaScript
Easily loop through the properties of an object without including inherited ones with the help of the hasOwnProperty method:
// Loop through object properties without including
// inherited properties
const object = { ... };
for (const key in object) {
if (object.hasOwnProperty(key)) {
// work on properties
}
}
As you can see, for the for
statement, the in
keyword is used. However, we also have an if statement that checks for hasOwnProperty
. This is because the in
keyword also includes inherited properties. To filter them out, we need the if
statement.
For a simpler solution, you can also use Object.keys
with a forEach
combined:
Object.keys(object).forEach((key, index) => {
console.log(`key: ${key}, value: ${object[key]}, index: ${index}`);
});
It has a fairly good global support, also supported in IE down to version 9.
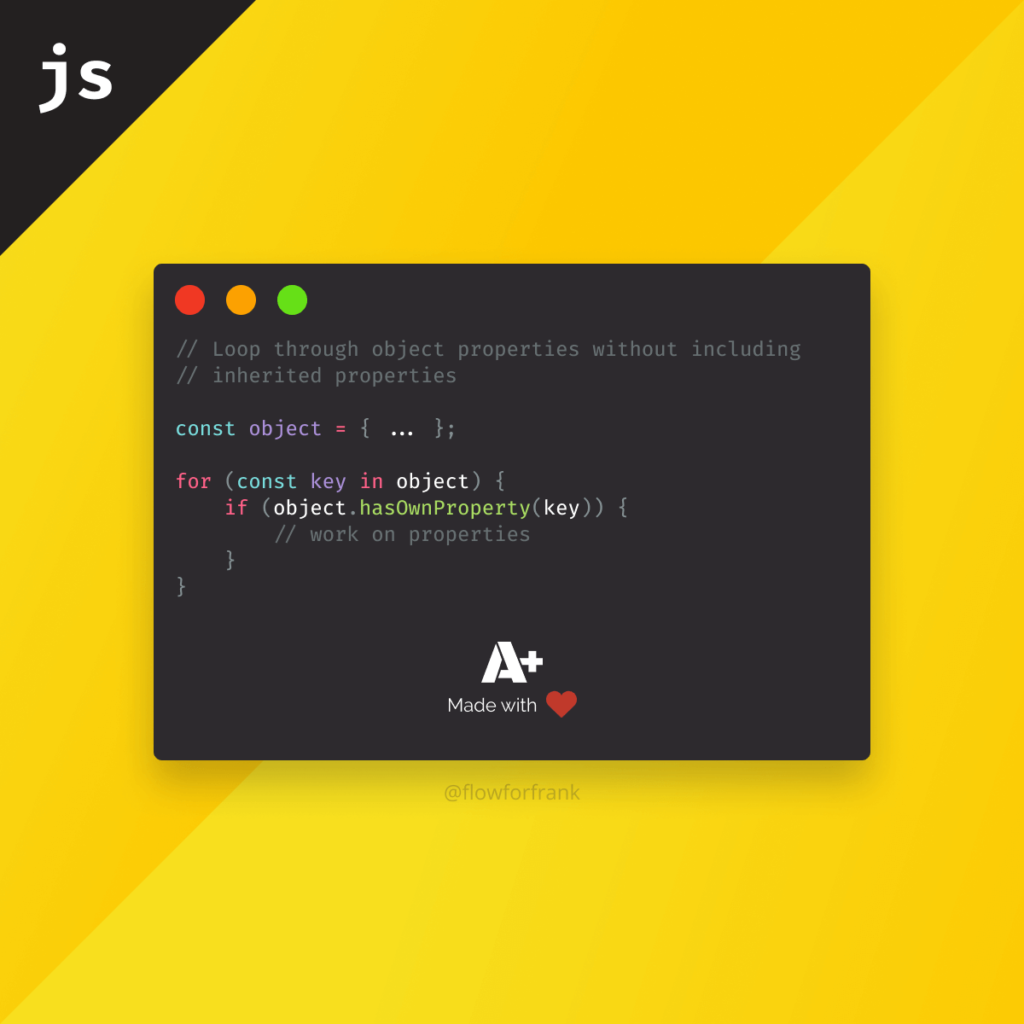
Resources:
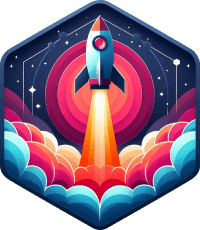
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: