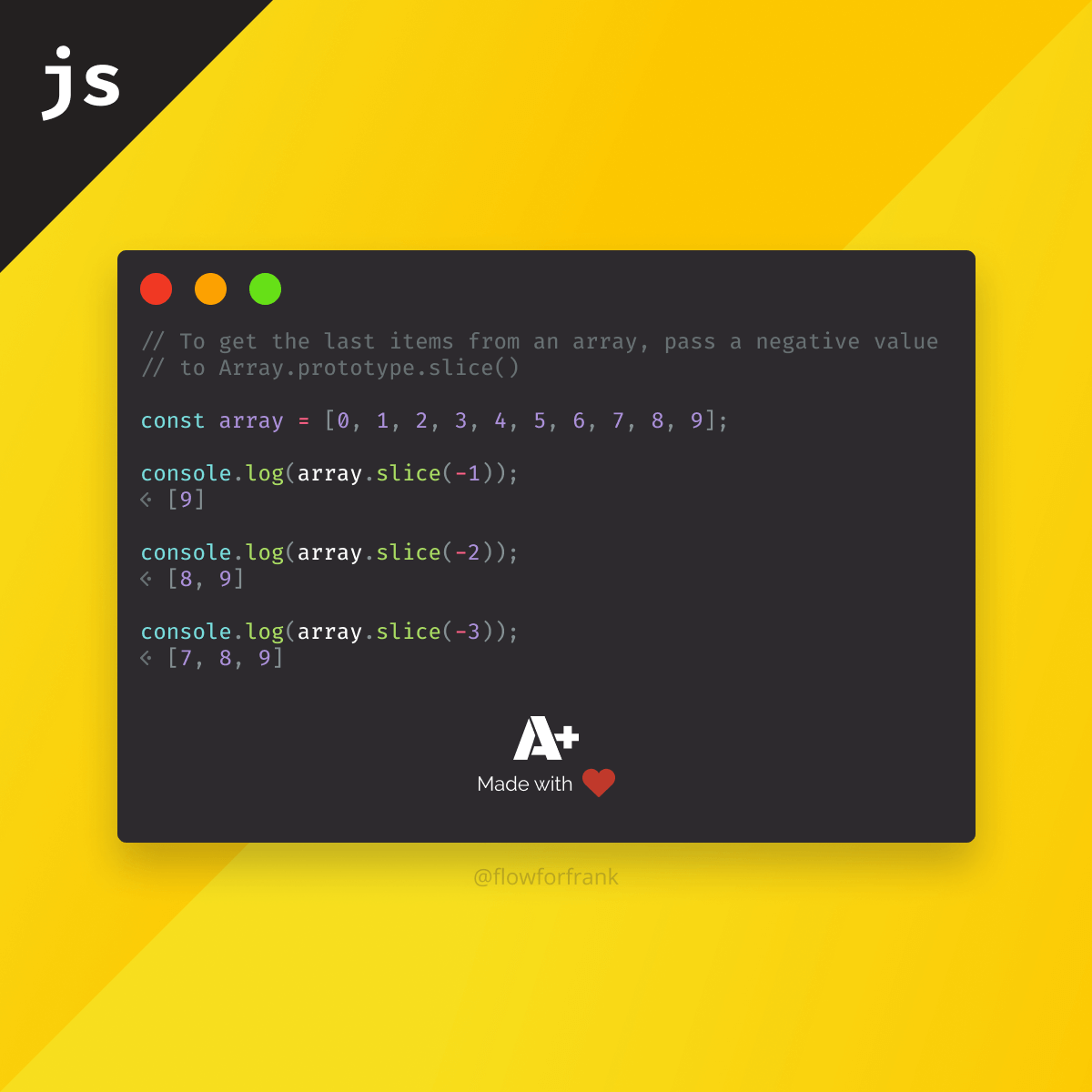
How to Get Last Items from Array in JavaScript
To get n number of last items from an array in JavaScript, simply pass a negative value to Array.slice
:
Copied to clipboard! Playground
const array = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
// This will return [9]
console.log(array.slice(-1));
// This will return [8, 9]
console.log(array.slice(-2));
// This will return [7, 8, 9]
console.log(array.slice(-3));
If you only interested in the returned value, you can also unwrap it with pop
:
Copied to clipboard!
// This will all return 0
array.slice(-1)[0];
array.slice(-1).pop();
array[array.length - 1];
In case your array is empty, undefined
will be returned.
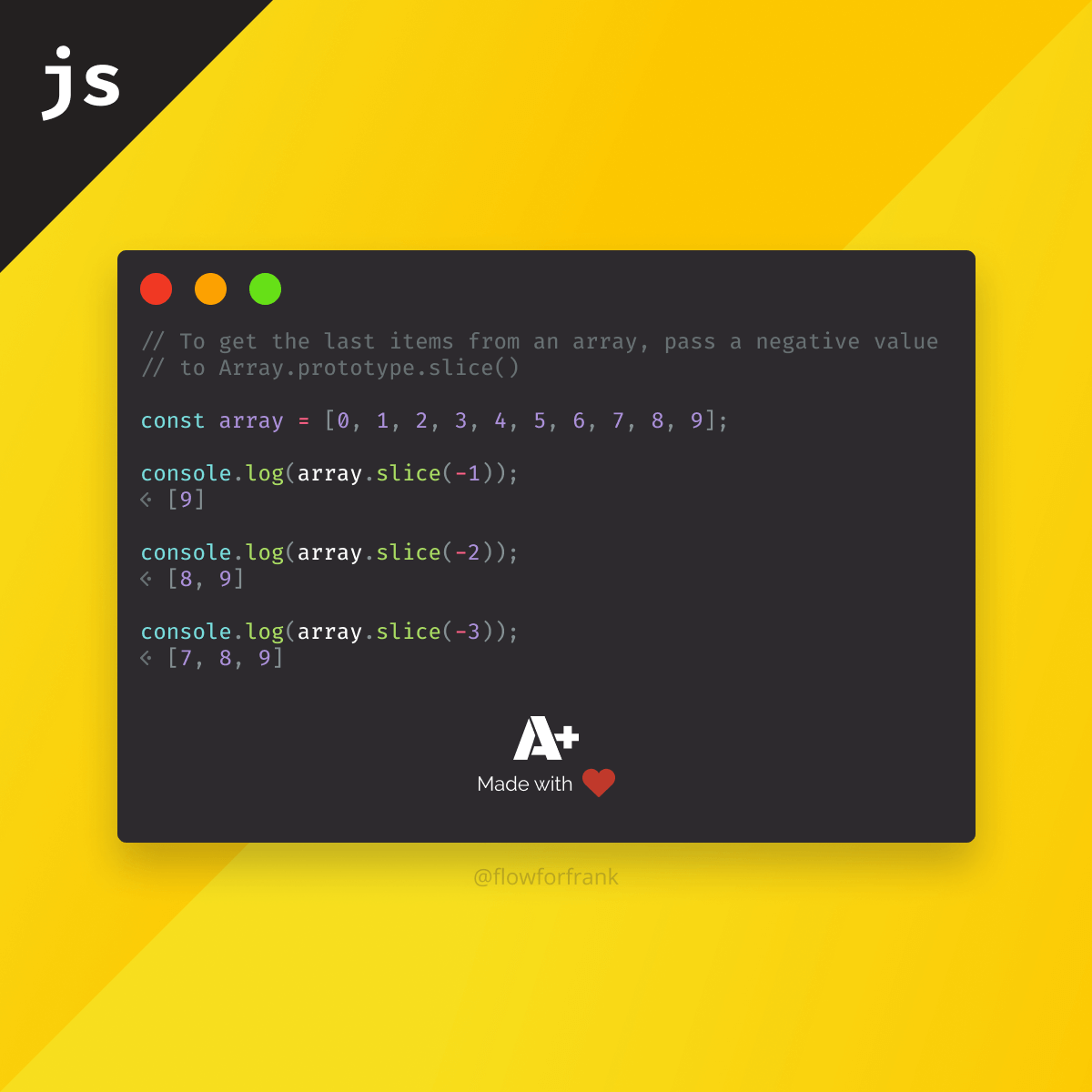
Resources:
π More Webtips
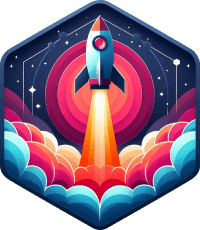
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: