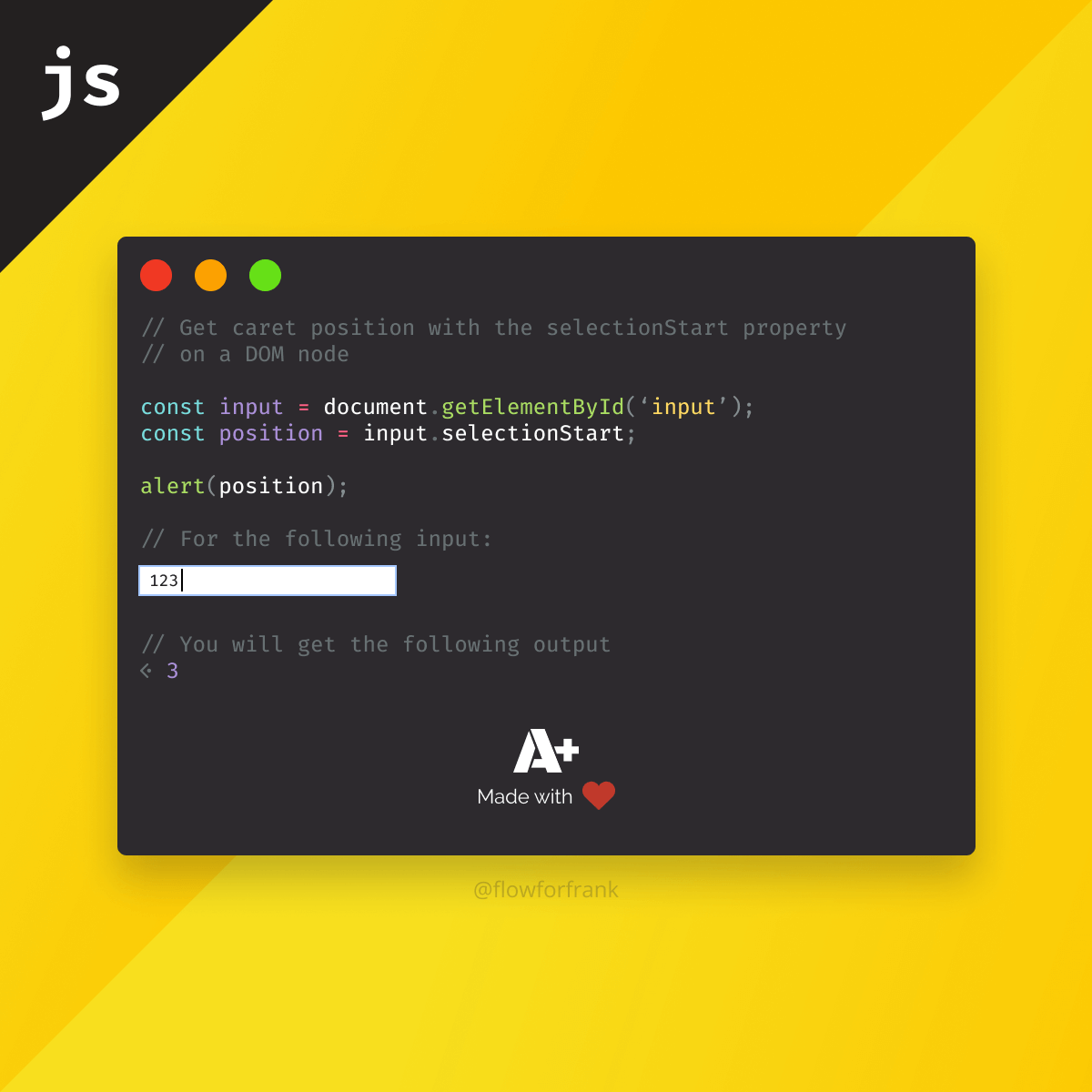
How to Get Cursor Position in JavaScript
If you ever had a specific case where you had to retrieve the position of a caret (your cursor's position) inside an HTML input field, you can do so using the selectionStart
property of an input's value.
<input type="text" id="input" value="123" />
<script>
const input = document.getElementById('input');
const position = input.selectionStart;
// If the position of the cursor is at the very last character inside the input,
// this will result in 3
alert(position);
</script>
You can also outsource this functionality to the following one-line function, to reuse it across your application:
const getCaretPosition = e => e && e.selectionStart || -1;
getCaretPosition(document.getElementById('input')); // Returns 3
getCaretPosition(document.getElementById('email')); // Returns -1
Keep in mind that selectionStart
can only be retrieved from the following list of input types:
text
password
search
tel
url
week
month
textarea
Therefore, in the example above, if you try to use it on an email
type, it will always return -1.
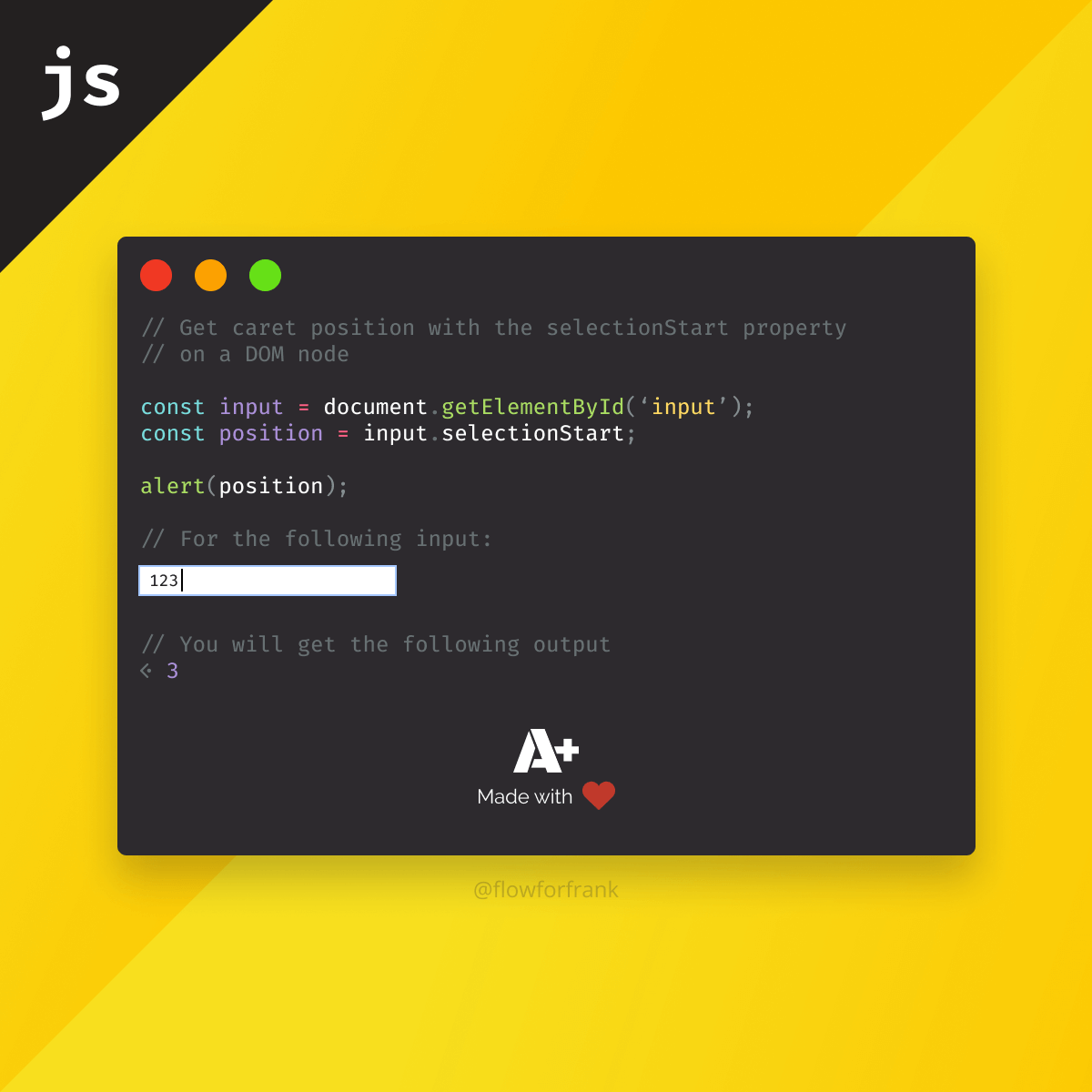
Resources
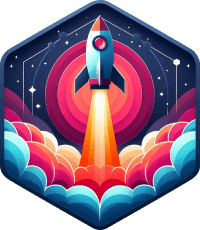
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: