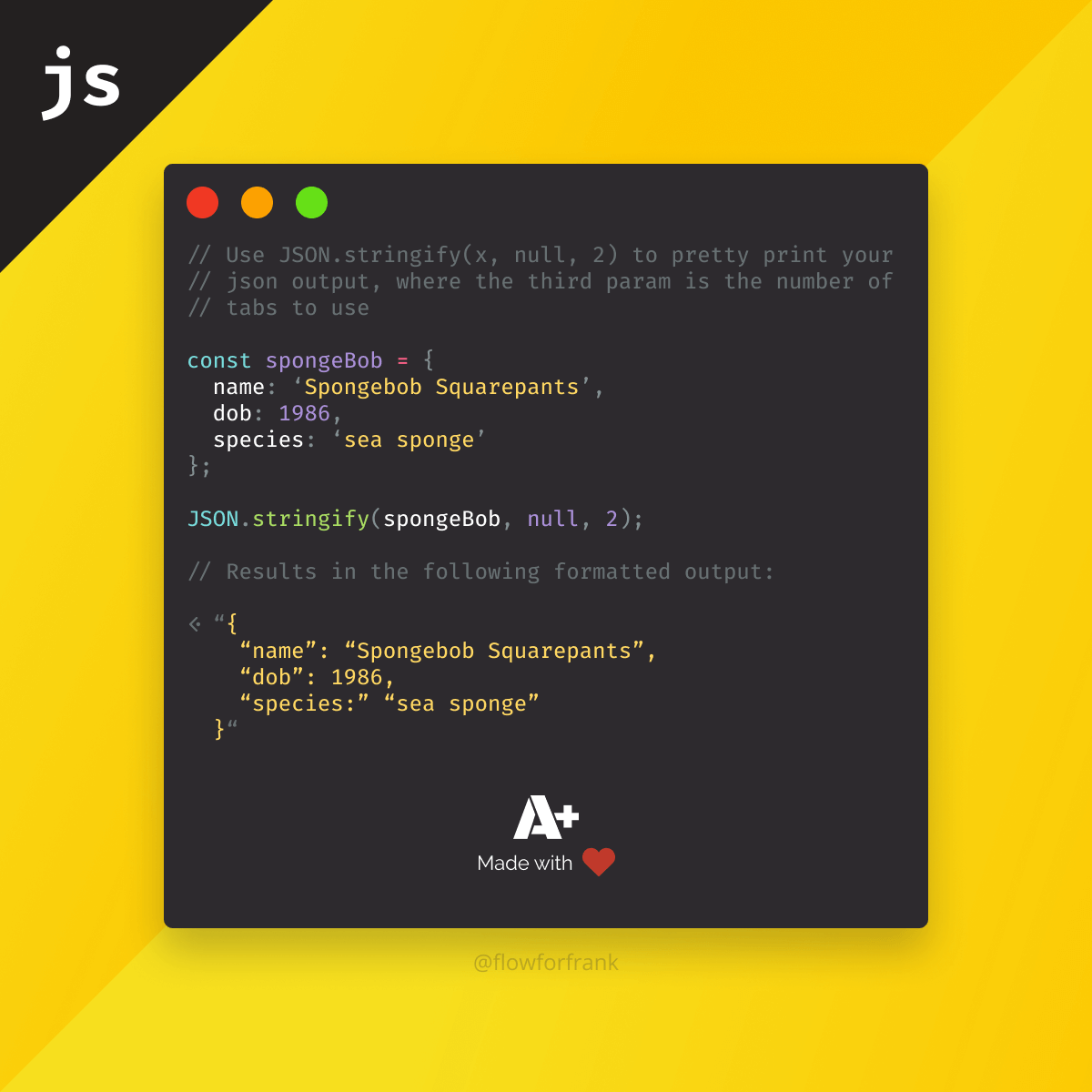
How To Format JSON in JavaScript
Ever had trouble getting through a JSON output during debug? You can use JSON.strinigfy with the number of spaces to use, passed as a 3rd parameterπ
But what is the second parameter then? Usually you pass null
, like so:
JSON.stringify(json, null, 2);
Examples
Let's see a couple of examples to break down what each param means:
const json = {
name: 'Spongebob Squarepants',
dob: 1986,
species: 'sea sponge'
};
// JSON.stringify without any optional params
JSON.stringify(json);
// Outputs:
// "{"name":"Spongebob Squarepants","dob":1986,"species":"sea sponge"}"
// JSON.stringify with optional params
JSON.stringify(json, null, 2);
// Outputs a formatted structure with 2 spaces:
// "{
// "name": "Spongebob Squarepants",
// "dob": 1986,
// "species": "sea sponge"
// }"
What is the Purpose of Params?
JSON.stringify
accepts 3 params. The first one is your data that you want to stringify. The other two are more interesting:
JSON.stringify(value, replacer, space);
replacer
: This is a function that you can use to change the stringification process. Instead of a function, you can also use an array to define which properties should be included in the result.space
: A string or number representing the number of spaces used for formatting the output.

The replacer Function
const json = {
name: 'Spongebob Squarepants',
dob: 1986,
species: 'sea sponge'
};
// Replacer function used for filtering
const replacer = (key, value) => typeof value === 'string' ? undefined : value;
JSON.stringify(json, replacer , 2);
// Outputs a filtered version of `json`:
// "{
// "dob": 1986
// }"
// Replacer function used as whitelist
JSON.stringify(json, ['name', 'species'], 2);
// Outputs a filtered version of `json`:
// "{
// "name": "Spongebob Squarepants",
// "species": "sea sponge"
// }"
In the first example, the function filters out everything from the object that is a string. Only dob
is left. In the second example, we've specified a list of properties we want to keep. The others al filtered out.
Now you know everything about JSON.stringify
. Do you know other tricks that can help debugging? Let us know in the comments!
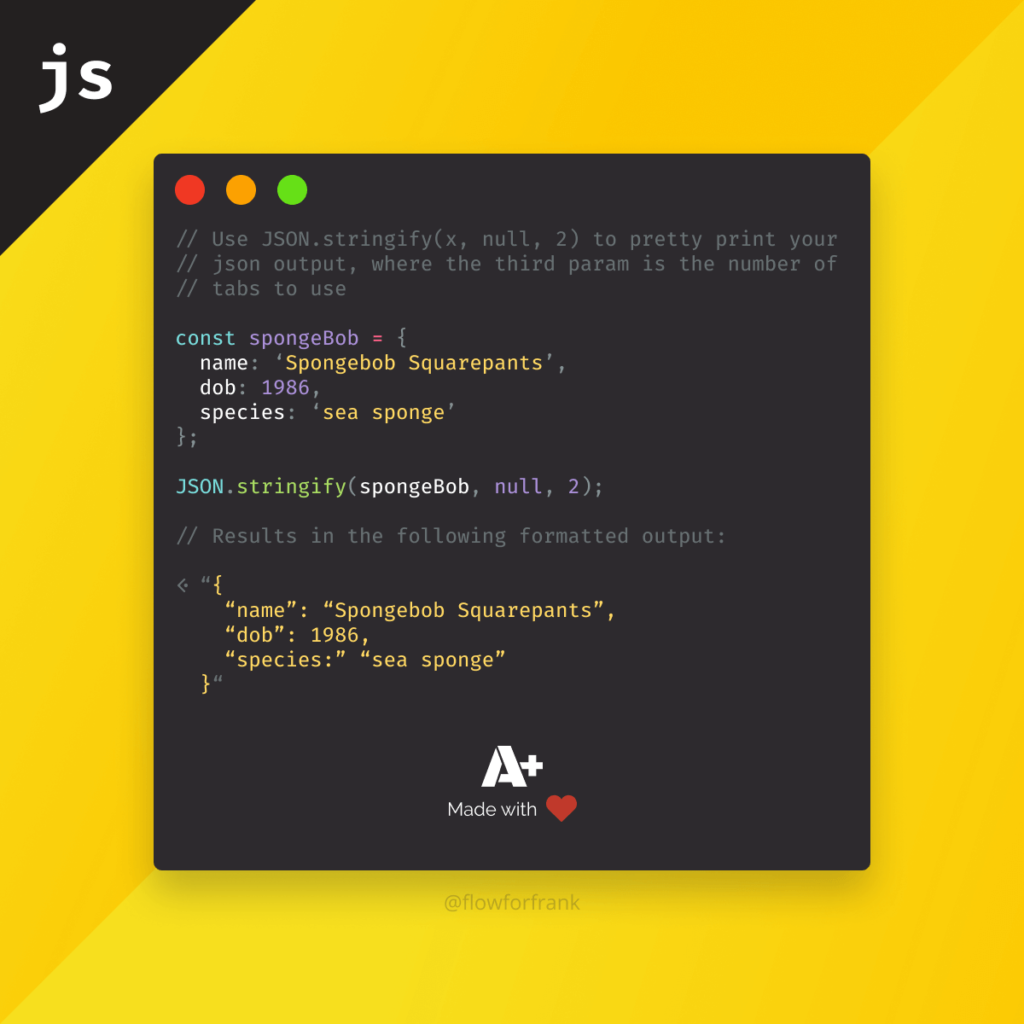
Resource
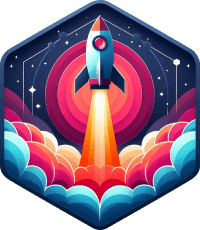
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: