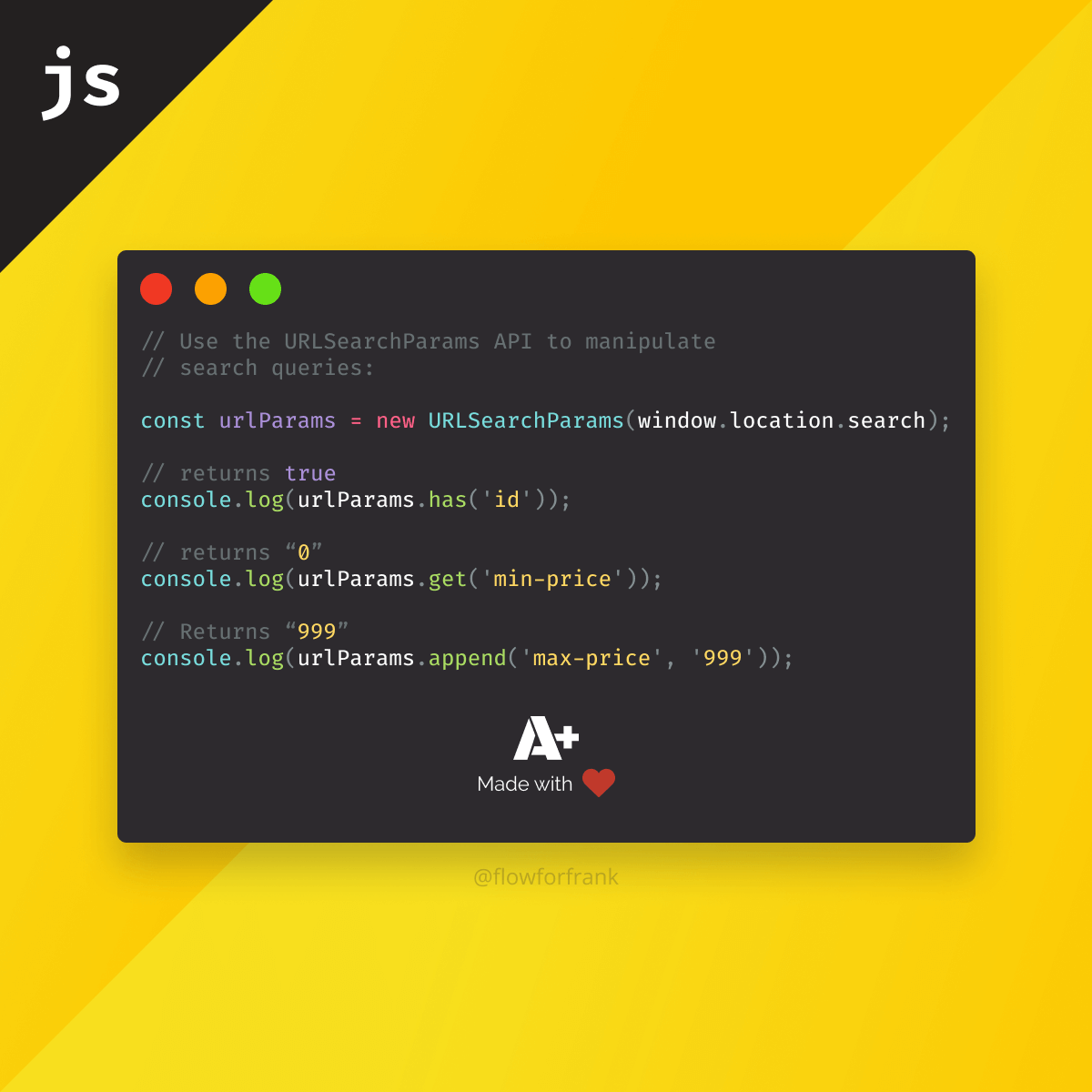
How to Easily Manipulate Search Queries in JavaScript
You don't have to write your own custom functions anymore to work with query string, JavaScript has the URLSearchParams
API which lets you interact and manipulate search queries with ease.
Copied to clipboard! Playground
// Use the URLSearchParams API to manipulate search queries:
const urlParams = new URLSearchParams(window.location.search);
console.log(urlParams.has('id')); // returns true
console.log(urlParams.get('min-price')); // returns β0β
console.log(urlParams.append('max-price', '999')); // Returns β999β
console.log(urlParams.toString()) // Returns βid=123&min-price=0&max-price=999β
So how well is it supported? According to caniuse.com, global support sits around ~95% at the writing of this article, with IE being unsupported.
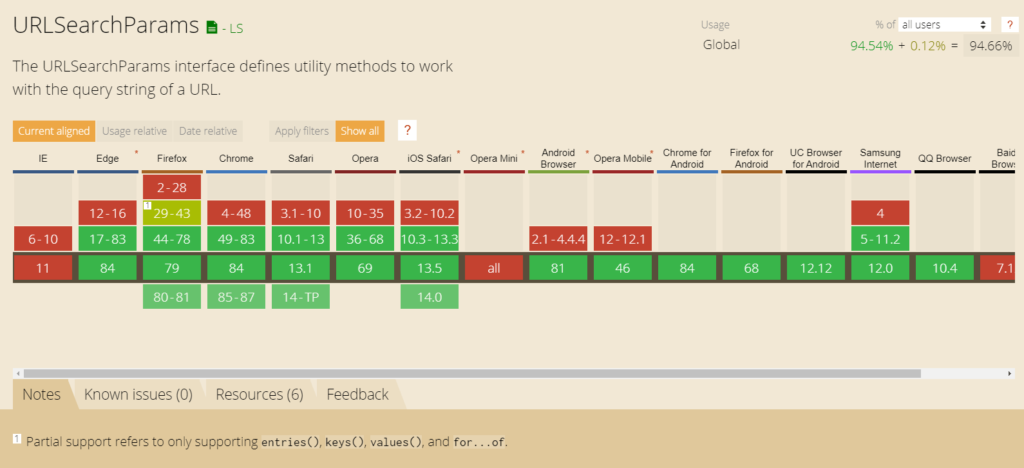
This means, if you need to cater for IE, you also need a fallback option. There is a polyfill for everything on NPMJS.
If you don't want to use a polyfill, but rather implement your own solution, there's also a URL API which has a slightly better support. It supports IE down to version 10.
Copied to clipboard! Playground
const url = new URL(window.location.href);
const searchParams = url.searchParams;
console.log(searchParams.has('id')); // returns true
console.log(searchParams.get('min-price')); // returns β0β
console.log(searchParams.append('max-price', '999')); // Returns β999β
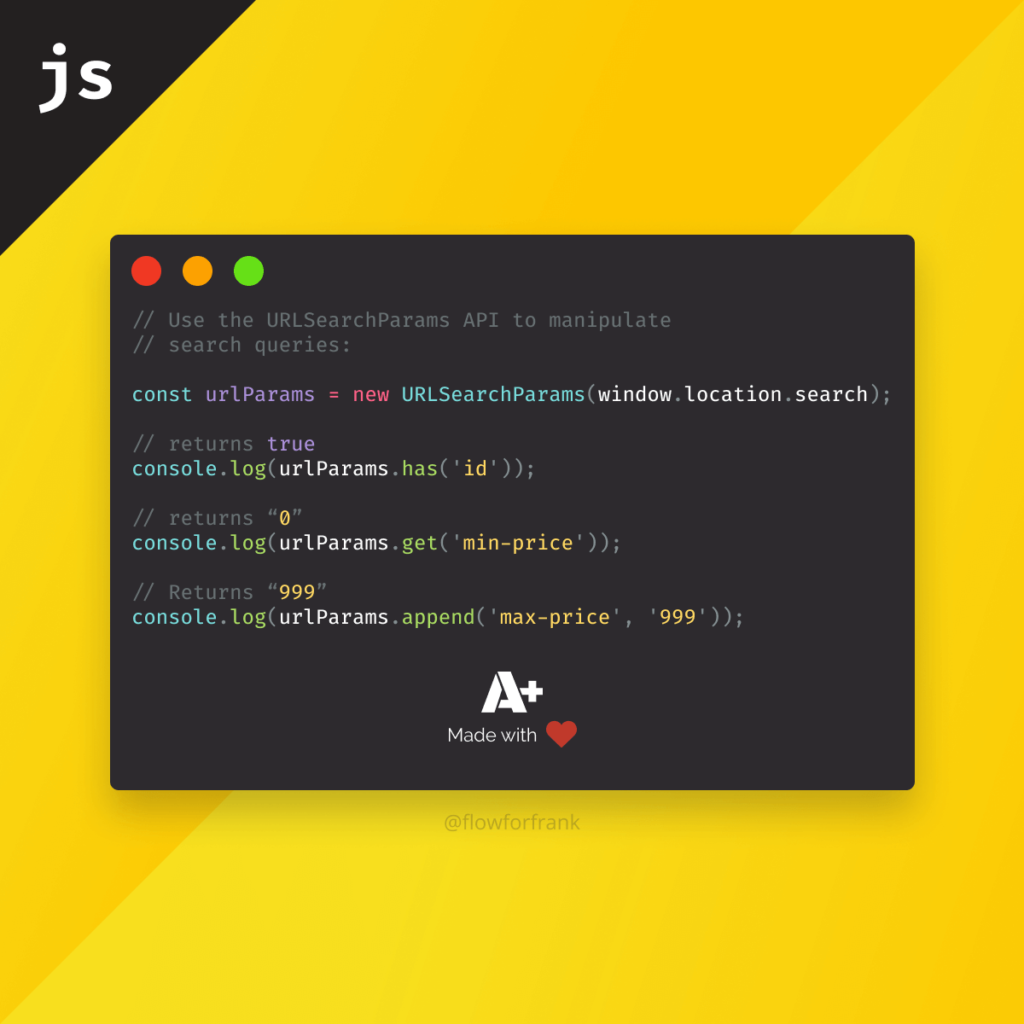
Resource
π More Webtips
Master the Art of Frontend
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews