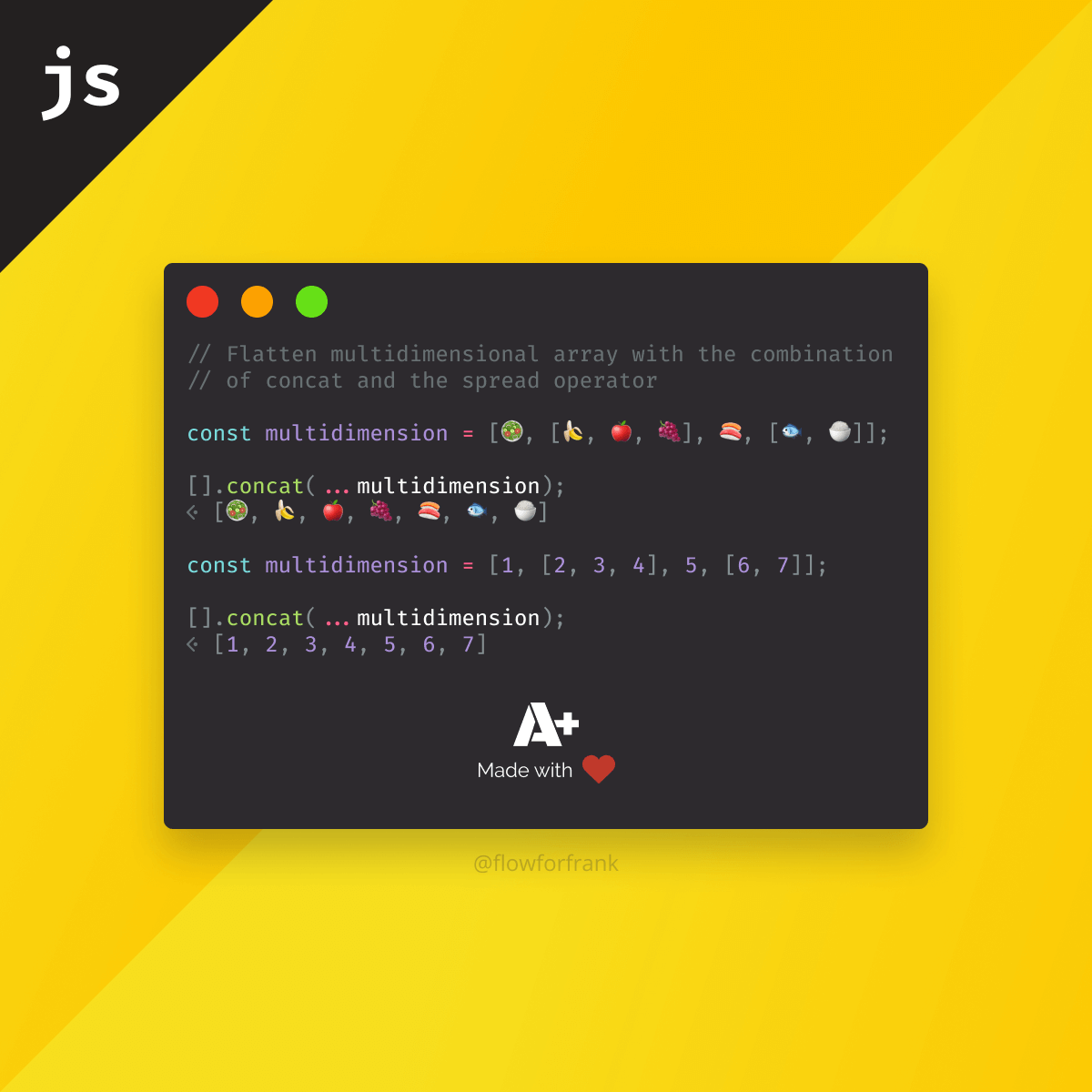
How to Easily Flatten Multidimensional Arrays in JavaScript
The spread operator comes to save us all once again. One of the cleanest ways to flatten a multidimensional array in JavaScript can be achieved with concat
and the spread
operator in the following way:
// Flatten multidimensional array with the combination
// of concat and the spread operator
const multidimension = [π₯, [π, π, π], π£, [π, π]];
[].concat(...multidimension); // This will return: [π₯, π, π, π, π£, π, π]
const multidimension = [1, [2, 3, 4], 5, [6, 7]];
[].concat(...multidimension); // This will return: [1, 2, 3, 4, 5, 6, 7]
However, this solution will only work if the depth level of the array is not greater than 1. To battle this, you could use Array.prototype.flat
:
// The depth of the array is 2
const multidimension = [1, [2, 3, 4], 5, [6, 7, [8, 9, 10]]];
[].concat(...multidimension); // Using the previous solution, this will return: [1, 2, 3, 4, 5, 6, 7, Array(3)]
multidimension.flat(2); // This will return: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
multidimension.flat(Infinity); // If you don't know the depth of the array, simply pass Infinity
The function takes in one parameter: the depth of the array. It defaults to 1. Keep in mind that this built-in function is not supported in IE. If you also need support for IE, you can either use a polyfill or the below function which achieves the same recursively:
const flatten = (array, output) => {
array.forEach(item => {
if(Array.isArray(item)) {
flatten(item, output);
} else {
output.push(item);
}
});
return output;
};
const output = [];
const multidimension = [1, [2, 3, 4], 5, [6, 7, [8, 9, 10]]];
flatten(multidimension, output); // This will return [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
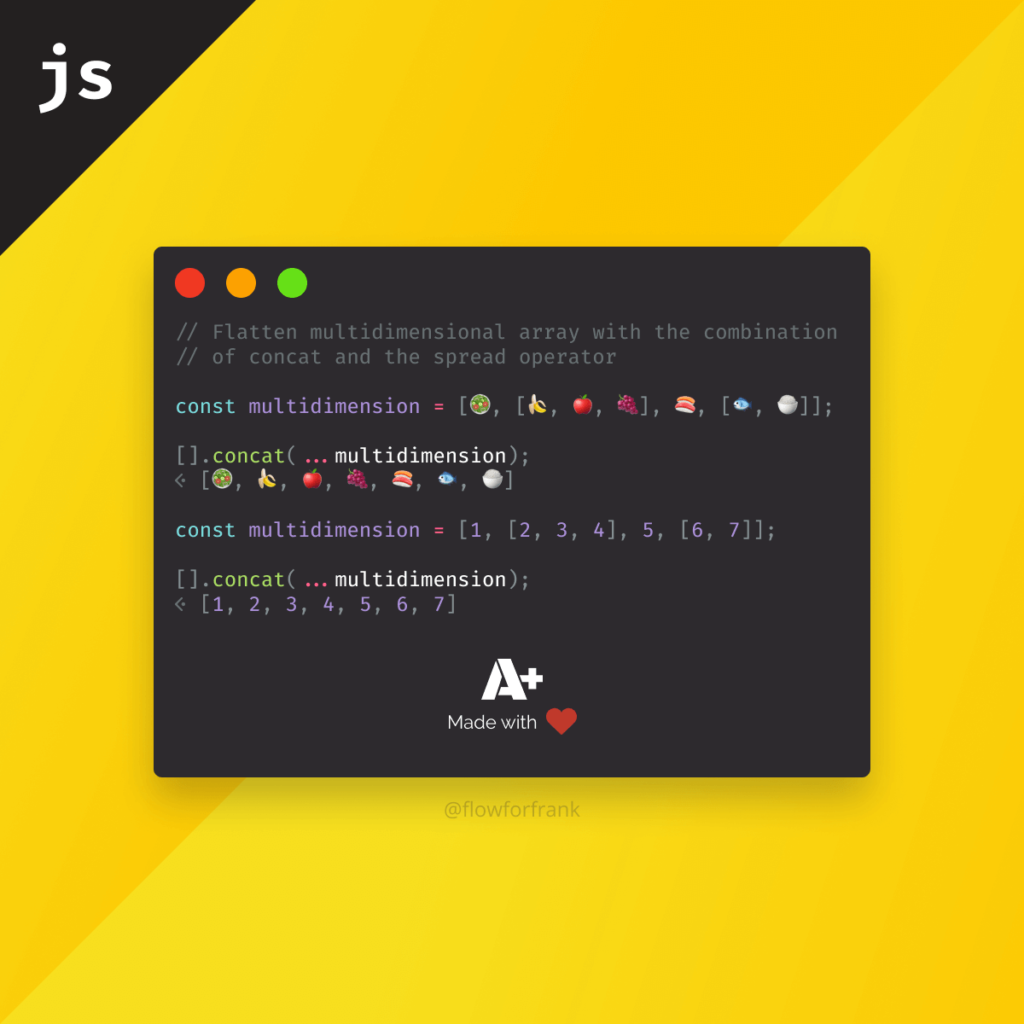
Resource
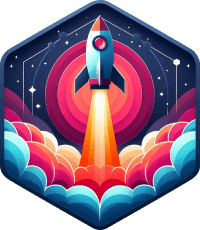
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: