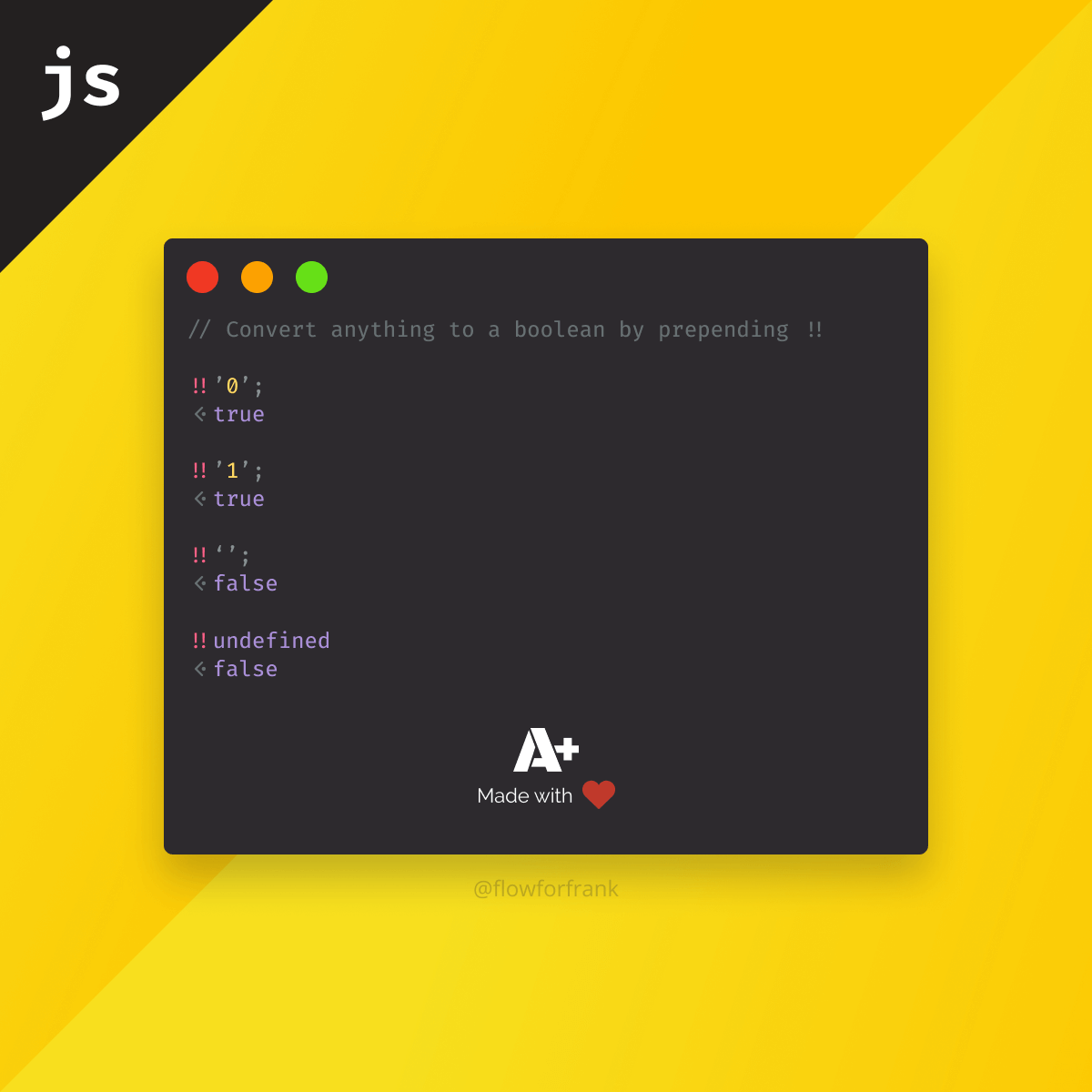
How to Convert Anything to Boolean in JavaScript
To convert anything to a boolean value in JavaScript, simply prepend it by two exclamation marks:
!!'0'; // returns true
!!'1'; // returns true
!!''; // returns false
!!undefined // returns false
This works by first negating the value, which turns it into a boolean, then this boolean is negated again to reflect the true state of the variable.
This solution, however, won't work, if you need to check for the string "true" or "false" specifically, as both will return true
. Instead, you can either use JSON.parse
or a custom function:
JSON.parse('true'); // returns true
JSON.parse('false'); // returns false
// To also cater for capitalization, use `toLowerCase`
JSON.parse('True'.toLowerCase());
In case you need to worry about 0
and 1
in string format, you can use a custom function:
const booleanify = val => {
if (typeof val === 'string') {
switch(val.toLowerCase()) {
case 'true':
case '1':
return true;
case 'false':
case '0':
return false;
default: return !!val;
}
} else {
return !!val;
}
};
booleanify(0); // returns false
booleanify('0'); // returns false
booleanify(1); // returns true
booleanify('1'); // returns true
booleanify(true); // returns true
booleanify('true'); // returns true
booleanify('True'); // returns true
booleanify(false); // returns false
booleanify('false'); // returns false
booleanify('False'); // returns false
booleanify(''); // returns false
booleanify('foo'); // returns true
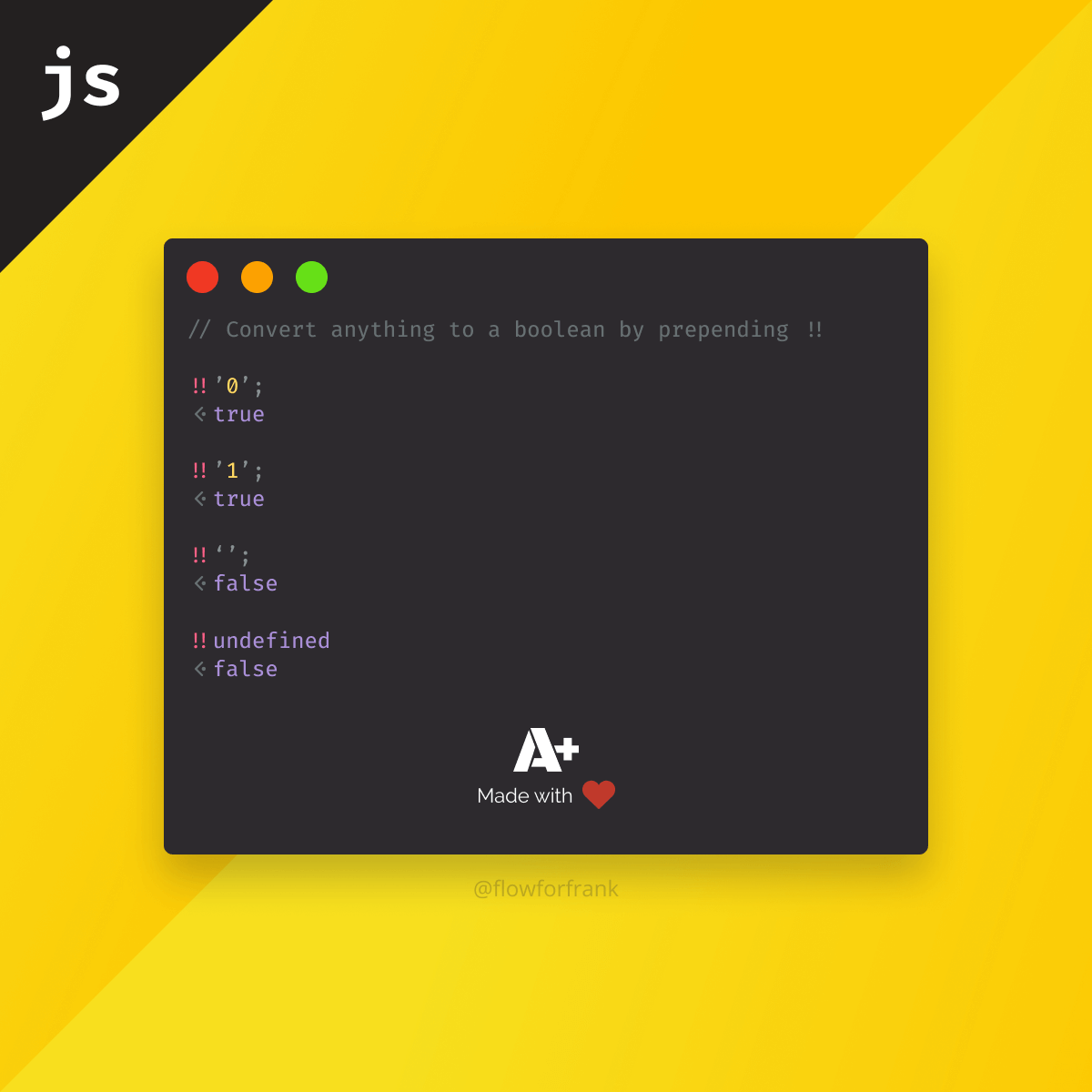
Resources:
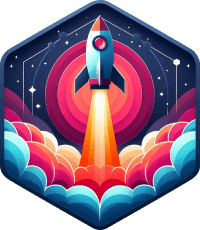
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: