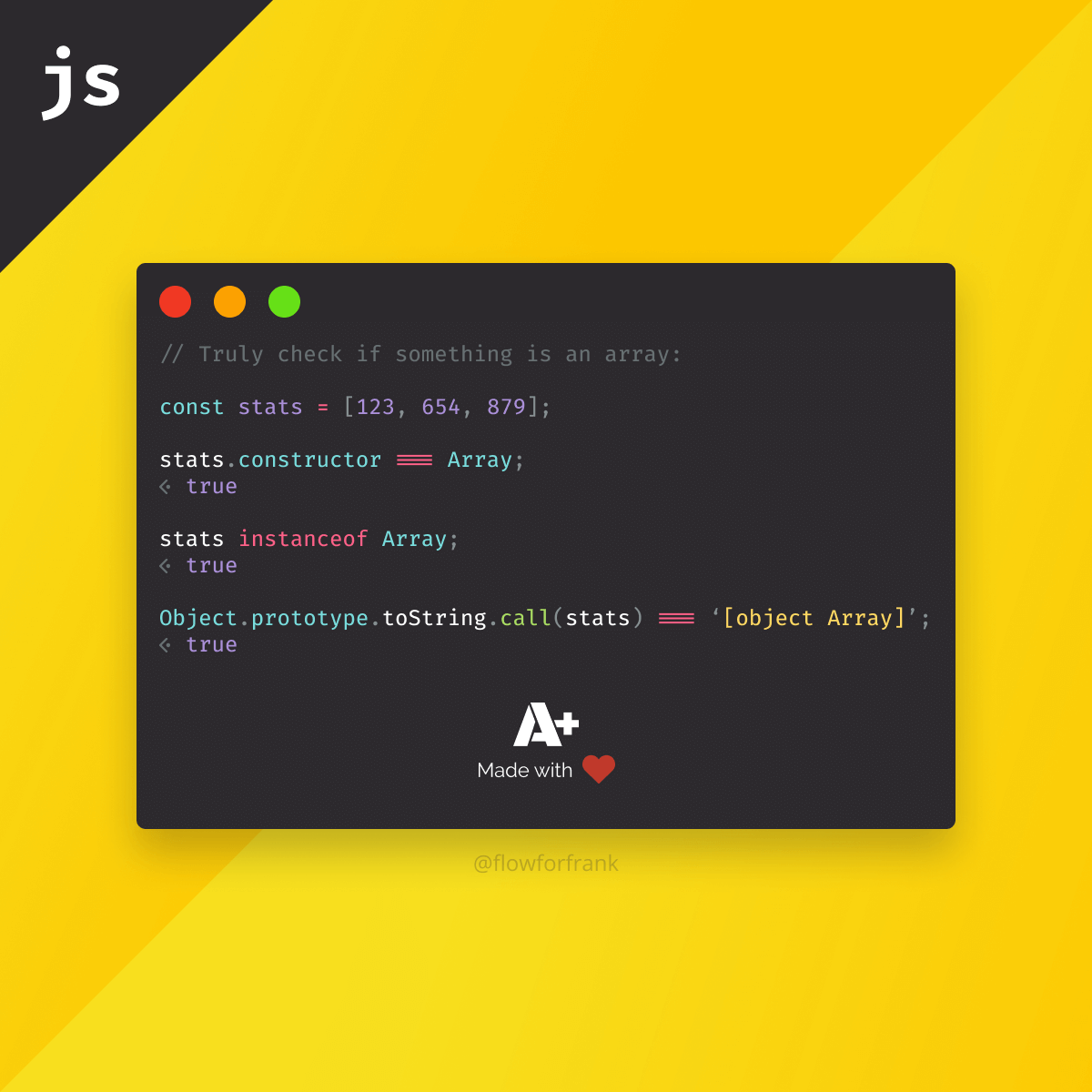
How to Check if Something is an Array in JavaScript?
When checking if something is an array in JavaScript, you can't rely on the typeof
operator because that just returns object.
Instead, here are four tips you can use to truly be sure you are dealing with an array:
// Truly check if something is an array in the following ways
const stats = [123, 654, 879];
stats.constructor === Array; // returns true
All objects in JavaScript have a constructor
property. Except the ones created with Object.create(null)
. It returns a reference to the Object constructor function that created the instance object. If this equals to Array, you can be sure your variable is an array as well.
stats instanceof Array; // returns true
You can also use the instanceof
operator to check whether Array
appears anywhere in the prototype chain. If it is, then it means your variable is an array.
Object.prototype.toString.call(stats) === '[object Array]'; // returns true
Calling Object.prototype.toString
, return the type of the object in a stringified version. Since you want to check the type of your variable, you need to call this method with the proper object. This is why you also need to append .call(stats)
. If this returns "[object Array]
", you can be sure, you are dealing with an array.
Array.isArray(stats) // returns true
Lastly, you can also use the built-in isArray
function to check whether your variable is an array or not. This is the simplest and most elegant solution. Whenever you can, always go with this.
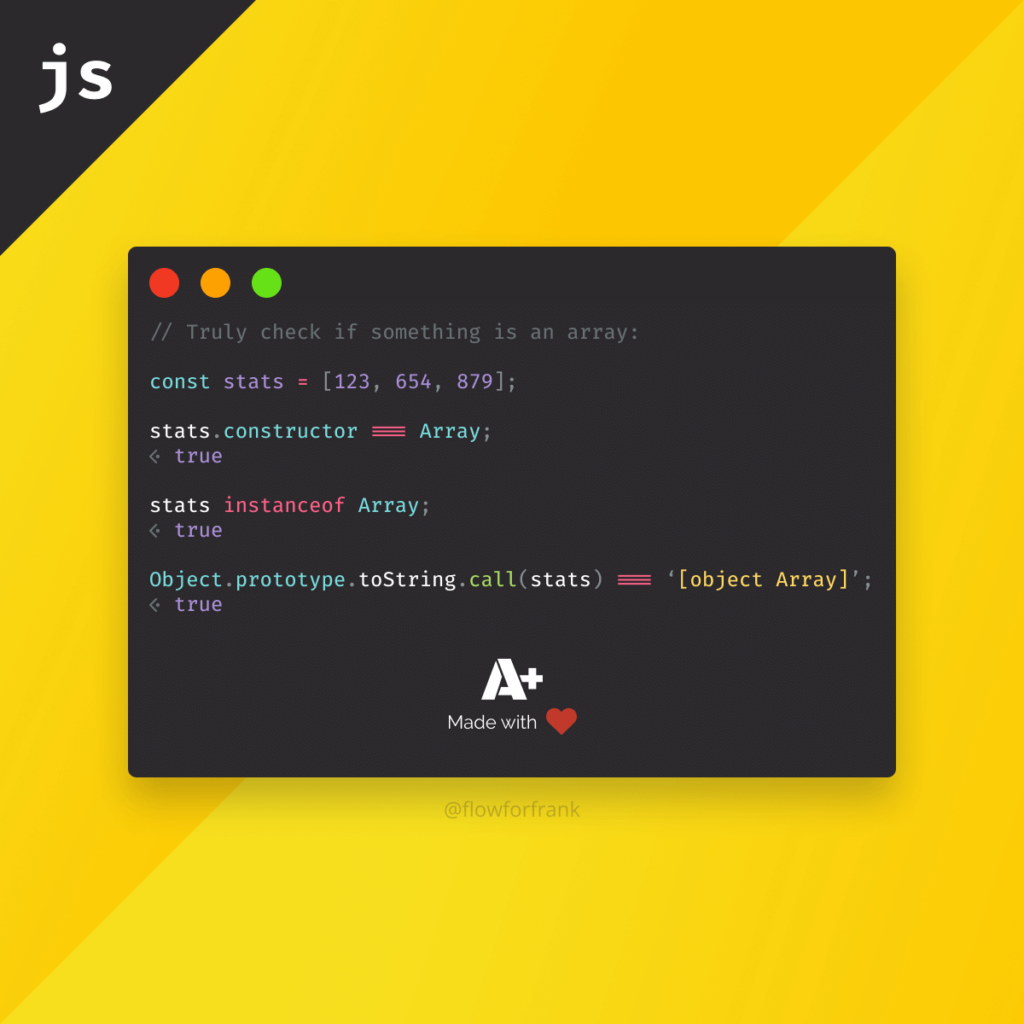
Resources:
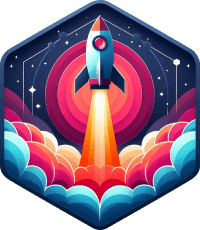
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: