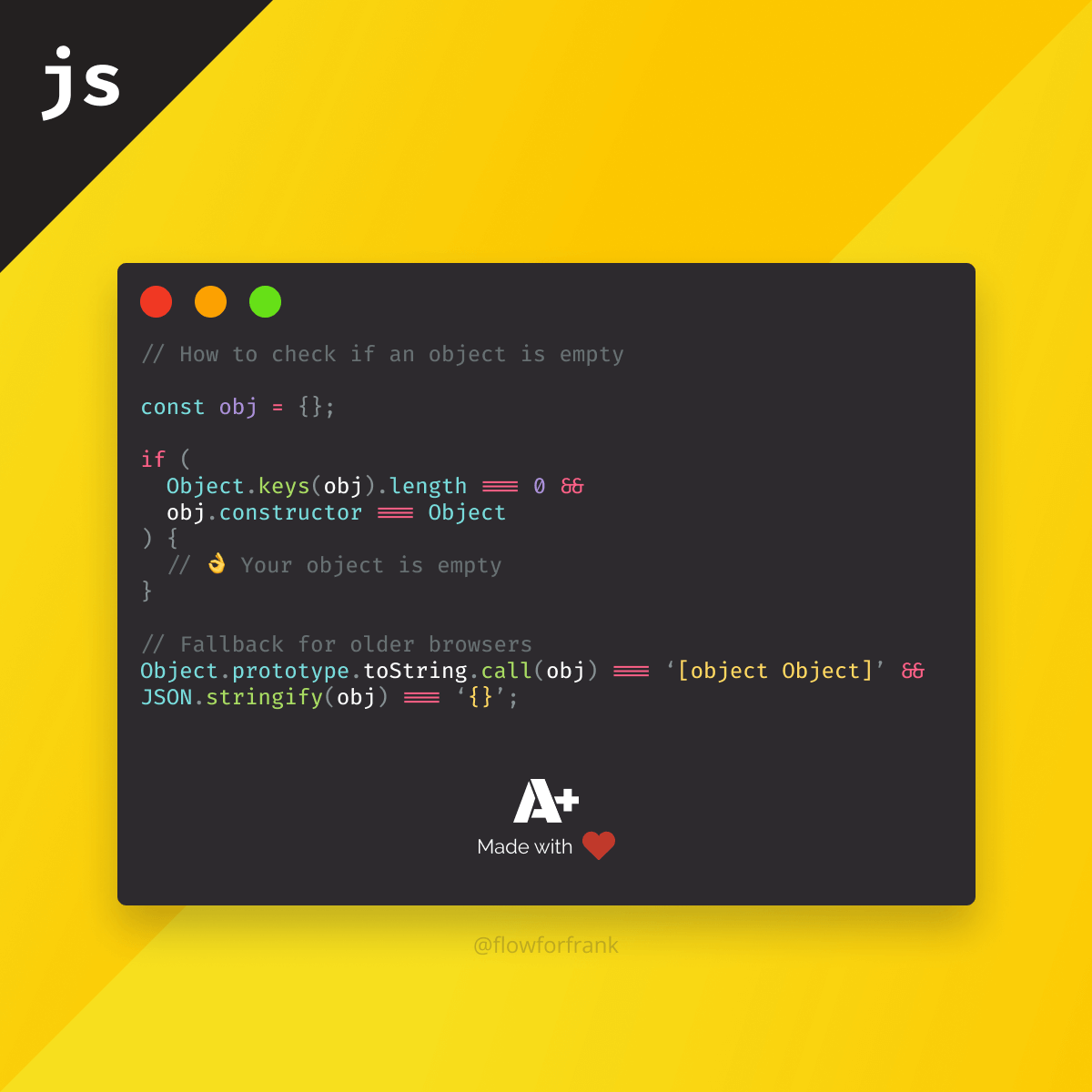
Simplest Way to Check for Empty Objects in JavaScript
The easiest way to check if an object is empty in JavaScript is to use a combination of Object.keys
and a constructor
check:
Object.keys(emptyObject).length === 0 && emptyObject.constructor === Object
// How to check if an object is empty using an if statement
const emptyObject = {};
if (Object.keys(emptyObject).length === 0 && emptyObject.constructor === Object) {
// π This means your object is empty
}
Object.keys
returns an array of the property names based on the passed object. But why do we need the second check for the constructor?
This is because Object.keys
alone will return true if you pass anything else than an object:
Object.keys(0).length === 0; // This will return true
Object.keys([]).length === 0; // This will return true
Object.keys(true).length === 0; // This will return true
Object.keys('').length === 0; // This will return true
Even though we are not checking against an object, but other arbitrary data, the equation will return true. This is why we must check if the constructor
is of type Object
.
Browser Support
While Object.keys
has fairly good support, if you need to fall back to older browsers, you can use the following solution:
// Fallback for older browsers
obj.constructor === Object && JSON.stringify(obj) === '{}';
// or
Object.prototype.toString.call(obj) === '[object Object]' && JSON.stringify(obj) === '{}';
This solution makes use of JSON.stringify
, which is used for converting objects to JSON strings.
Keep in mind that for both solutions, you will get an error if you try to use it on a null
or undefined
value.
Uncaught TypeError: Cannot convert undefined or null to object
To get around this, you first need to check if the variable exists:
// Prevent errors for null and undefined
const emptyObject = {};
// The first check here is used for filtering out null and undefined
if (emptyObject && Object.keys(emptyObject).length === 0 && emptyObject.constructor === Object) {
// π This means your object is empty
}
Or wrapping the whole thing into a function:
const isEmptyObject = object => {
if (Object.keys) {
return object &&
Object.keys(object).length === 0 &&
object.constructor === Object;
}
return obj.constructor === Object && JSON.stringify(obj) === '{}';
};
isEmptyObject({}); // Returns true
isEmptyObject([]); // Returns false
isEmptyObject(0); // Returns false
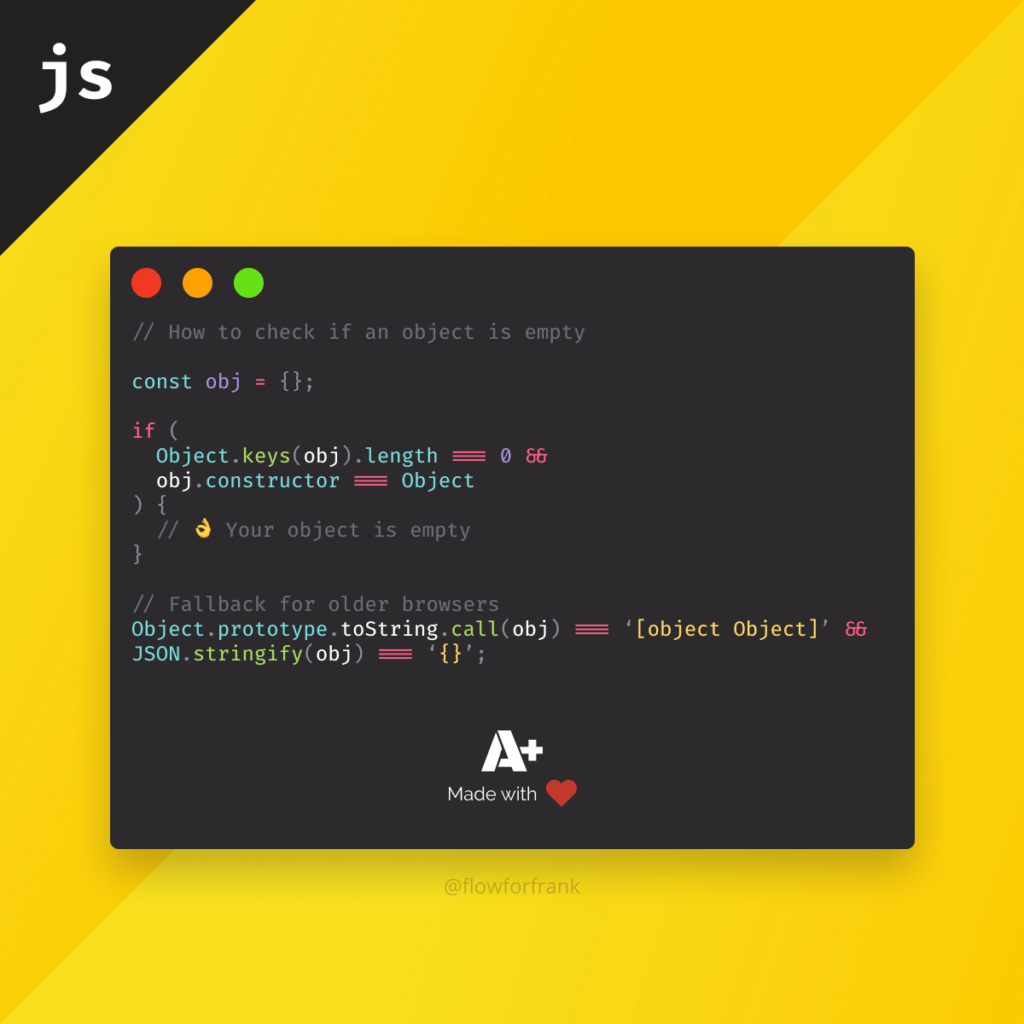
Resources:
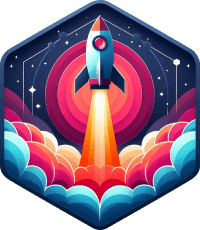
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: