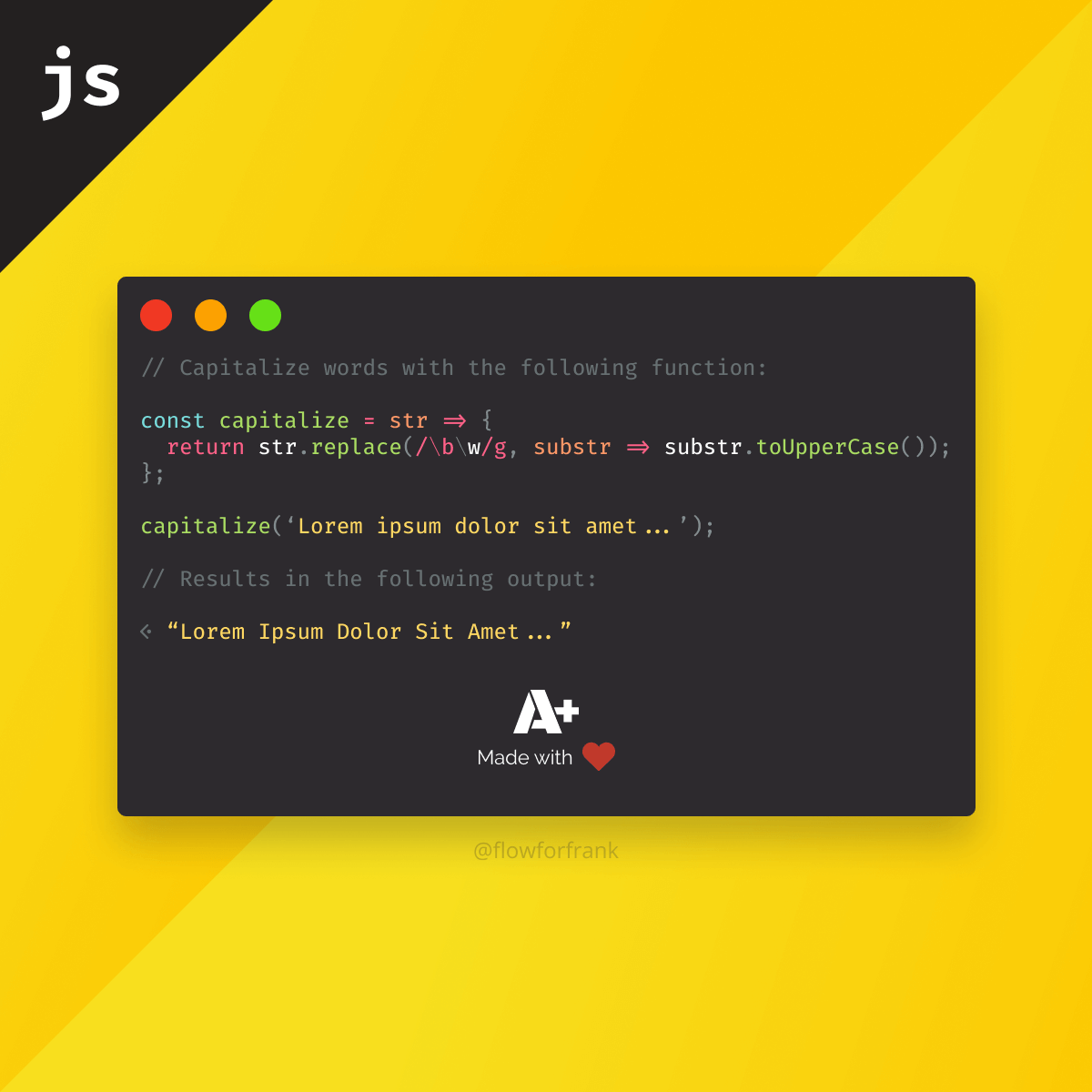
How to Capitalize Words in JavaScript
If you want to capitalize words in JavaScript, you can use the following function which makes use of replace
, toUpperCase
and some regex:
const capitalize = str => str.replace(/\b\w/g, substr => substr.toUpperCase());
// This will return: "Lorem Ipsum Dolor Sit Amet..."
capitalize('lorem ipsum dolor sit amet...');
The regex for the string replace uses an anchor and a word boundary to match the first character of every word. If you need to capitalize only the very first letter of your string, you can use the following solution:
const capitalize = str => str.charAt(0).toUpperCase() + str.slice(1).toLowerCase();
// This will return: "Lorem ipsum dolor sit amet..."
capitalize('lorem ipsum dolor sit amet...');
// This also works for wrong capitalizations:
capitalize('lOREM IPsUm dolor sit amet...');
This function will get the first character of your string (charAt(0)
), turn it into uppercased, and then slice(1)
returns the rest of the string, lowercased.
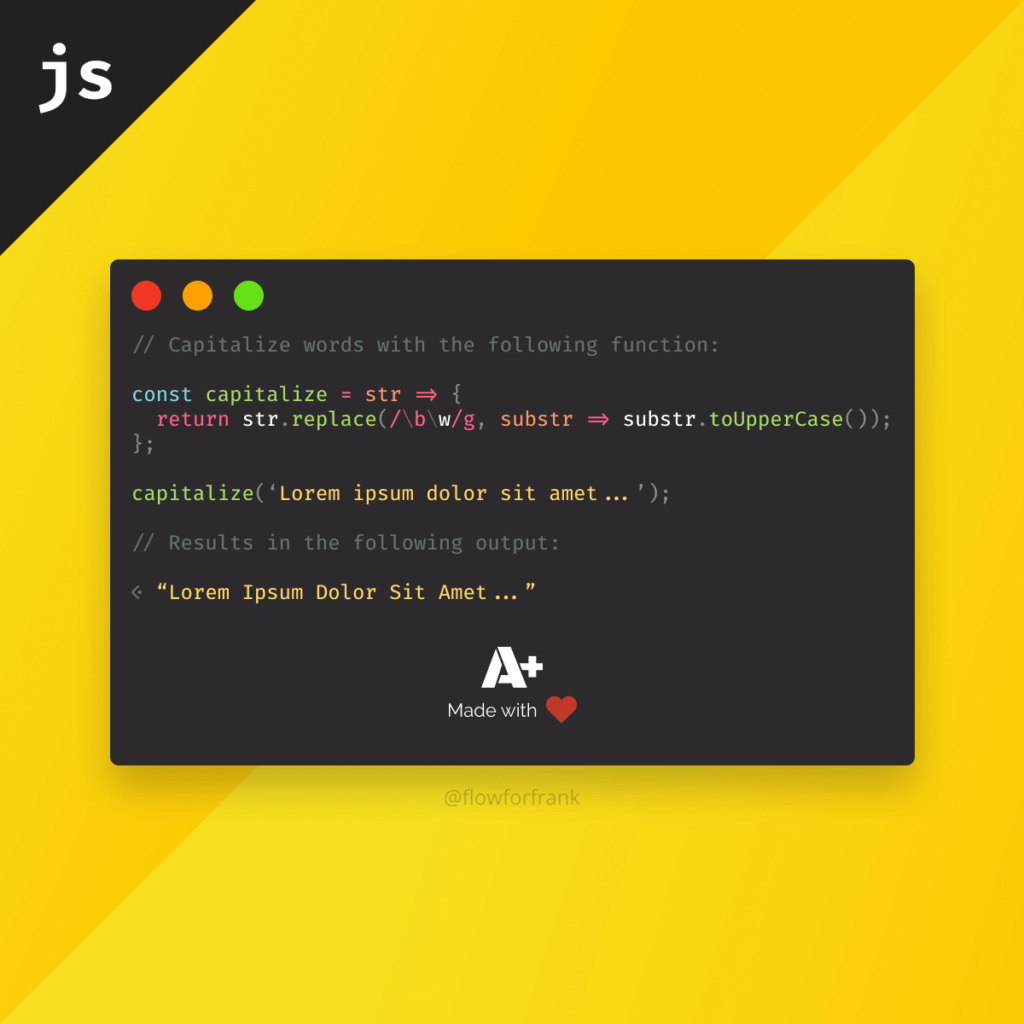
Resources:
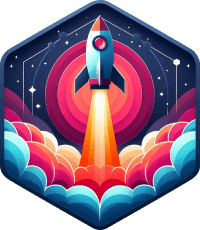
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: