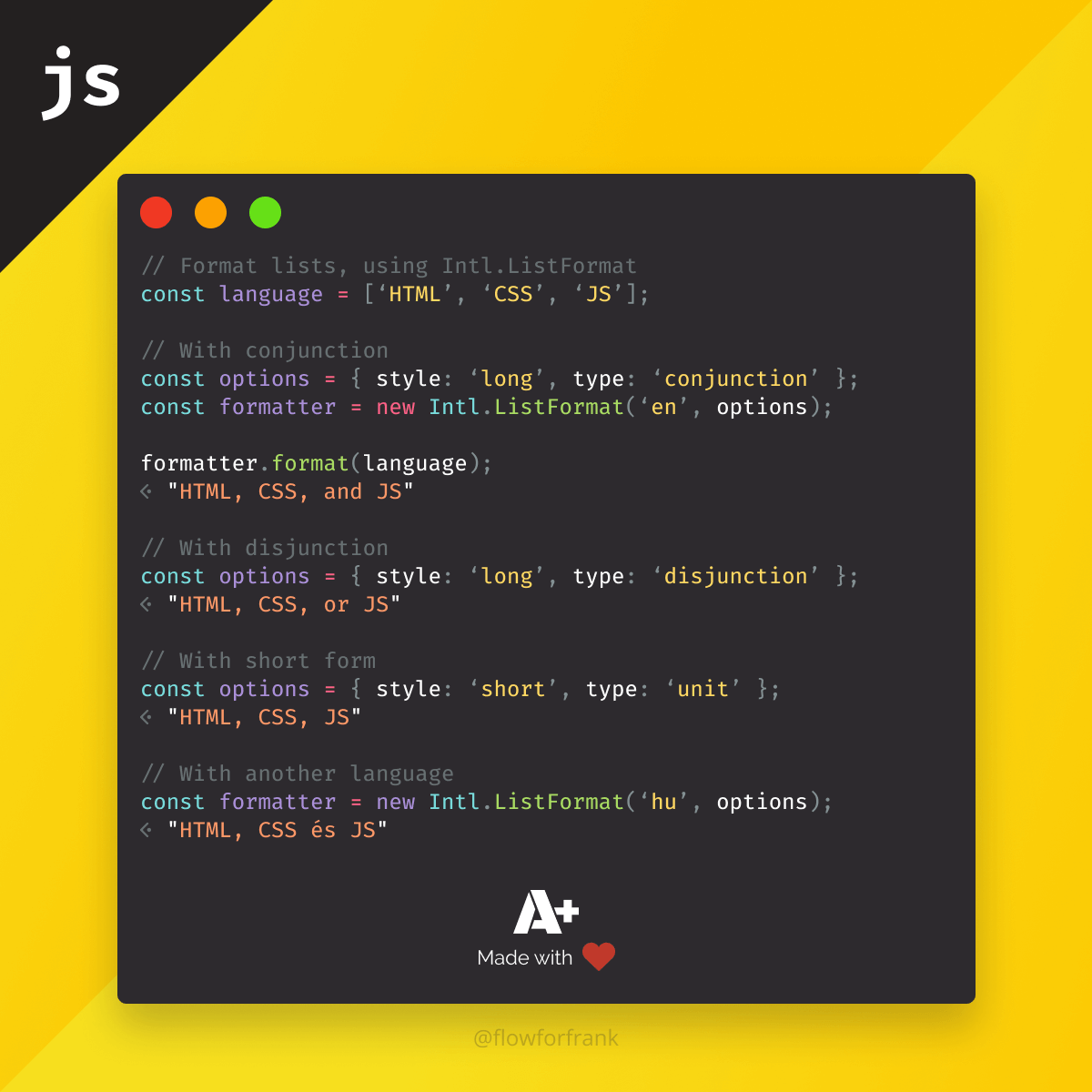
How to Format Lists in JavaScript based on Languages
Do you want to concatenate an array of strings in JavaScript and format them based on different languages? You can use the ListFormat
method of an Intl
object with various options to get different results. Take the following as the most basic example:
const language = ['HTML', 'CSS', 'JS'];
const options = {
style: 'short',
type: 'unit'
};
const formatter = new Intl.ListFormat('en', options);
formatter.format(language); // Will return "HTML, CSS, JS"
You can create a new ListFormat
using the new
keyword, passing in a locale (BCP 47 language tag) and an optional object of options. Calling formatter.format
on the array will format it according to the passed options
.
Available Options
You can also customize the way the string is translated. The method accepts the following properties inside the options
object:
localeMatcher
: The matching algorithm to use for locales. It can be one oflookup
, orbest fit
. The default value isbest fit
. You can learn more about the matching algorithm on MDN.style
: This option decides the length of the formatted text. It can be eitherlong
(the default value), orshort
, meaning you either get "HTML, CSS, and JS" forlong
, or "HTML, CSS, JS" forshort
.type
: The type of the list. It can be one ofconjunction
("and-based" list),disjunction
("or-based" list), orunit
for using a list of units. Note that whenshort
is used as astyle
, thetype
must be aunit
.
Using Conjunction
To use conjunctions, you want to use a long
style with the type of conjunction
, passed as options to ListFormat
. This produces an "and-based" list:
const options = { style: 'long', type: 'conjunction' };
const formatter = new Intl.ListFormat('en', options);
formatter.format(language); // Will return "HTML, CSS, and JS"

Using Disjunction
For using disjunctions, you want to use a long
style with the type of disjunction
, passed as options to ListFormat
. This produces an "or-based" list:
const options = { style: 'long', type: 'disjunction' };
const formatter = new Intl.ListFormat('en', options);
formatter.format(language); // Will return "HTML, CSS, or JS"
const formatter = new Intl.ListFormat('hu', options);
formatter.format(language); // Will return "HTML, CSS, vagy JS"
Support
It's important to also talk about support. Even though most major browsers do have support, there are exceptions. At the writing of this, it has a global support of around ~71%. For example, it is unsupported both in IE and Safari. For polyfilling, you can use Format.js.
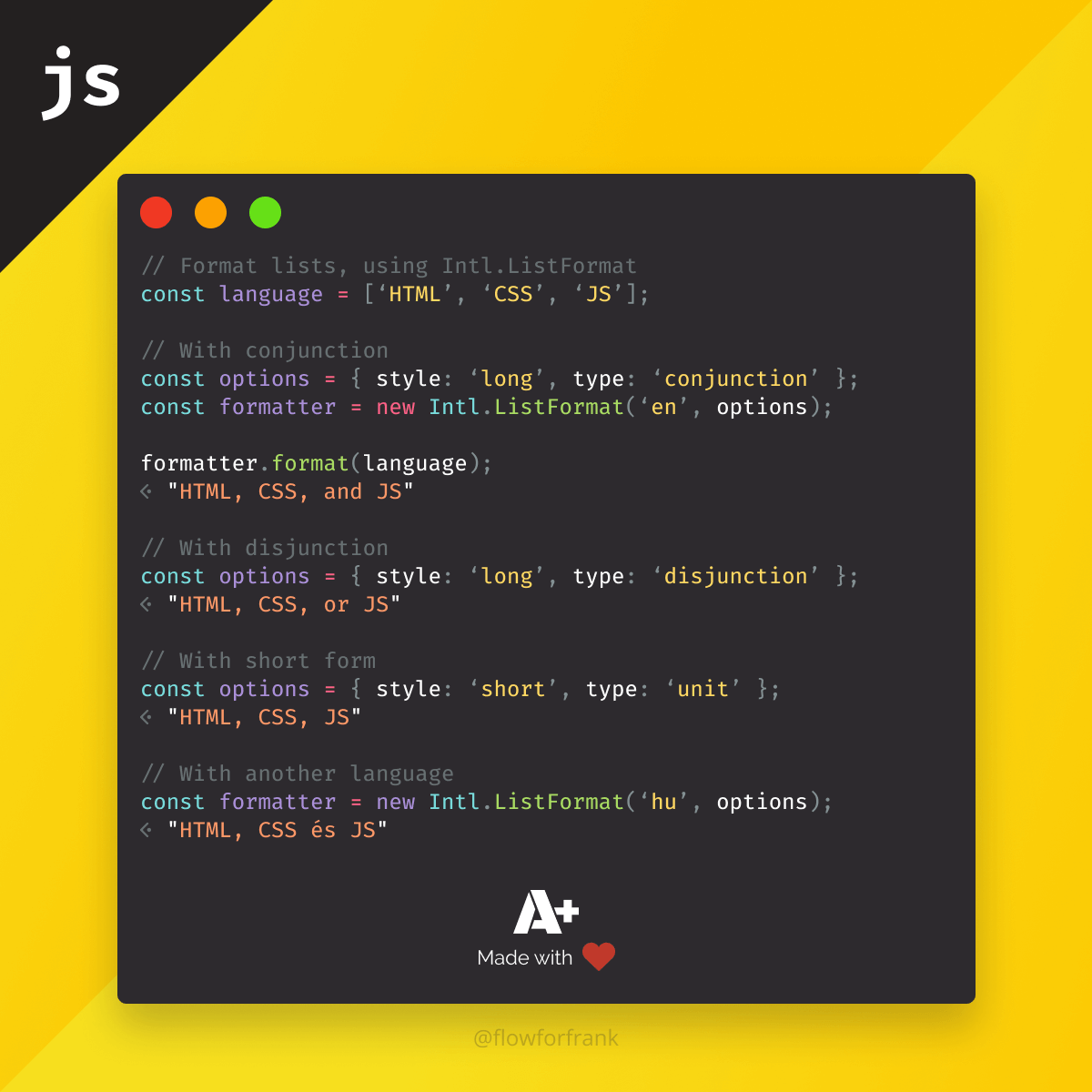
Resources:
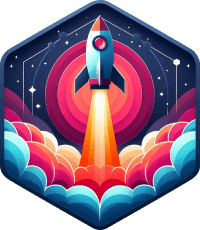
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: