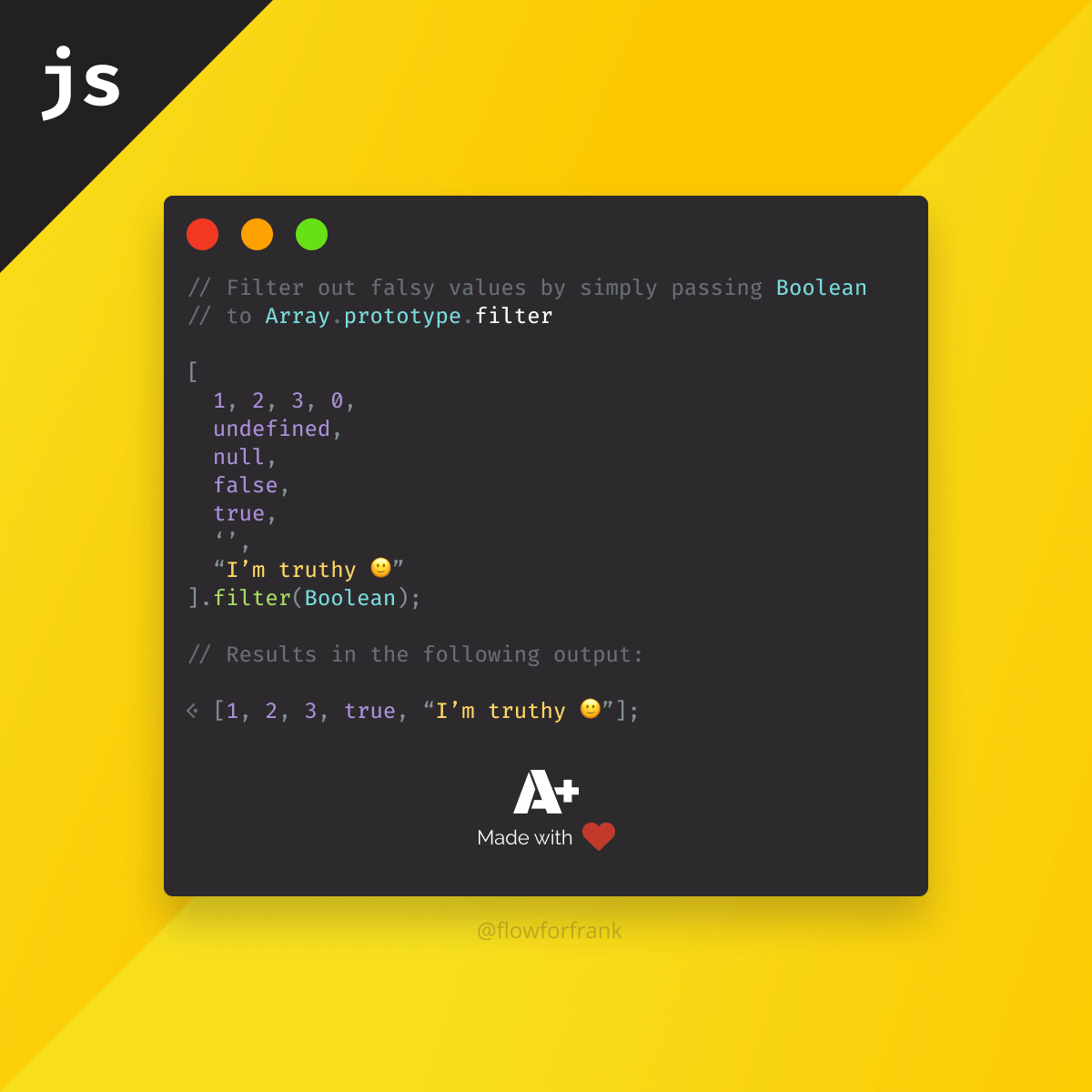
How to Filter for True Values in an Array in JavaScript
Probably one of the simplest and cleanest ways to get rid of falsy values in JavaScript is by utilizing the built-in filter array function the following way:
Copied to clipboard! Playground
// Filter out falsy values by simply passing Boolean to Array.prototype.filter
[
1, 2, 3, 0,
undefined,
null,
false,
true,
'',
'Iβm truthy π'
].filter(Boolean);
// Results in the following array:
[1, 2, 3, true, 'Iβm truthy π'];
The only problem is that this won't work for nested arrays. To get around this, you can use the below function which uses recursion to filter out falsy values from a nested array:
Copied to clipboard! Playground
const filterFalsy = array => array.filter(Boolean)
.map(item => Array.isArray(item) ? filterFalsy(item) : item);
const array = [
1, 2, 3, 0,
undefined,
null,
false,
true,
[0, 1, 2, '', [false, true]],
'',
'Iβm truthy π'
];
filterFalsy(array); // This will return the following array:
[1, 2, 3, true, [1, 2, [true]], "Iβm truthy π"]
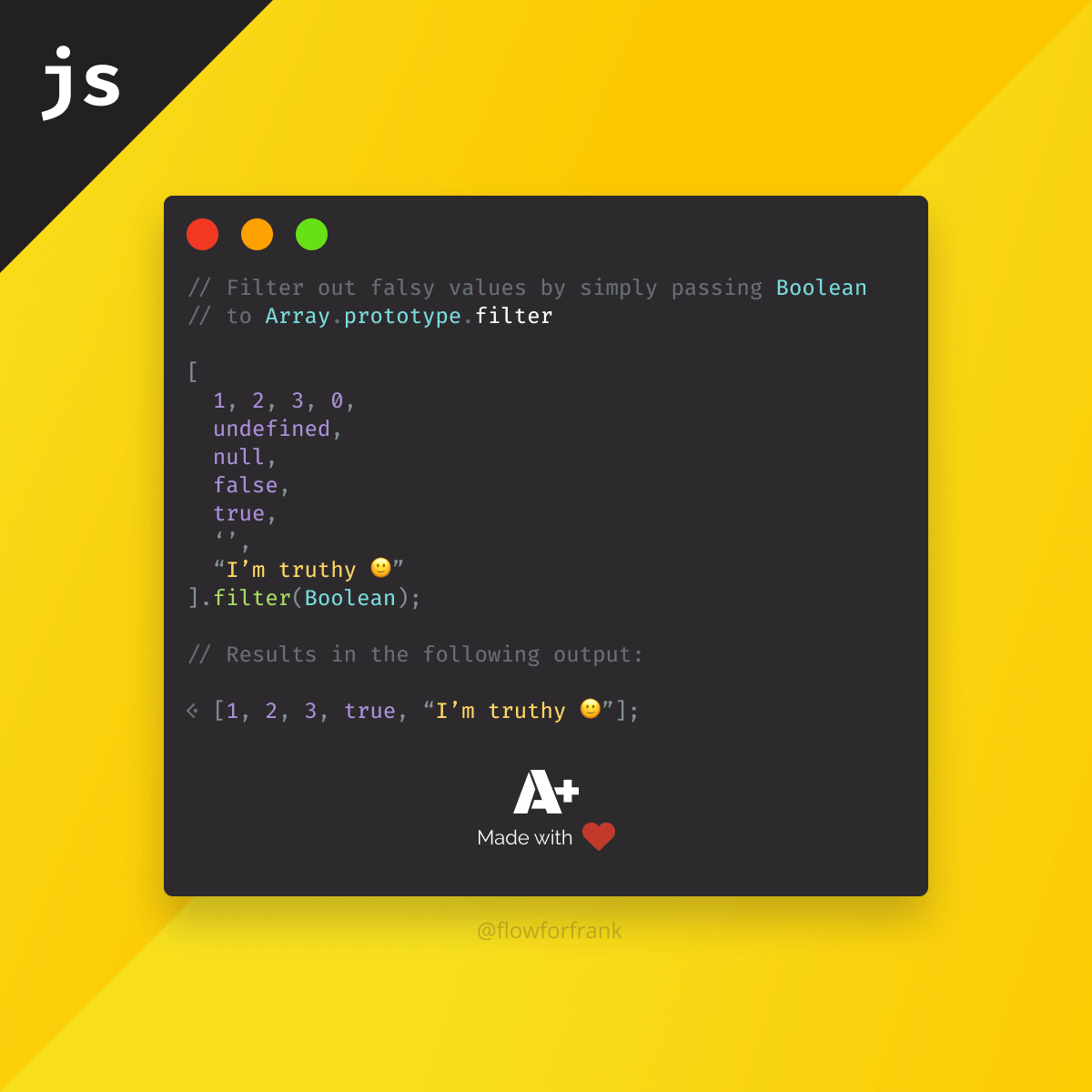
Resource
π More Webtips
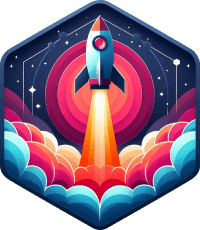
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: