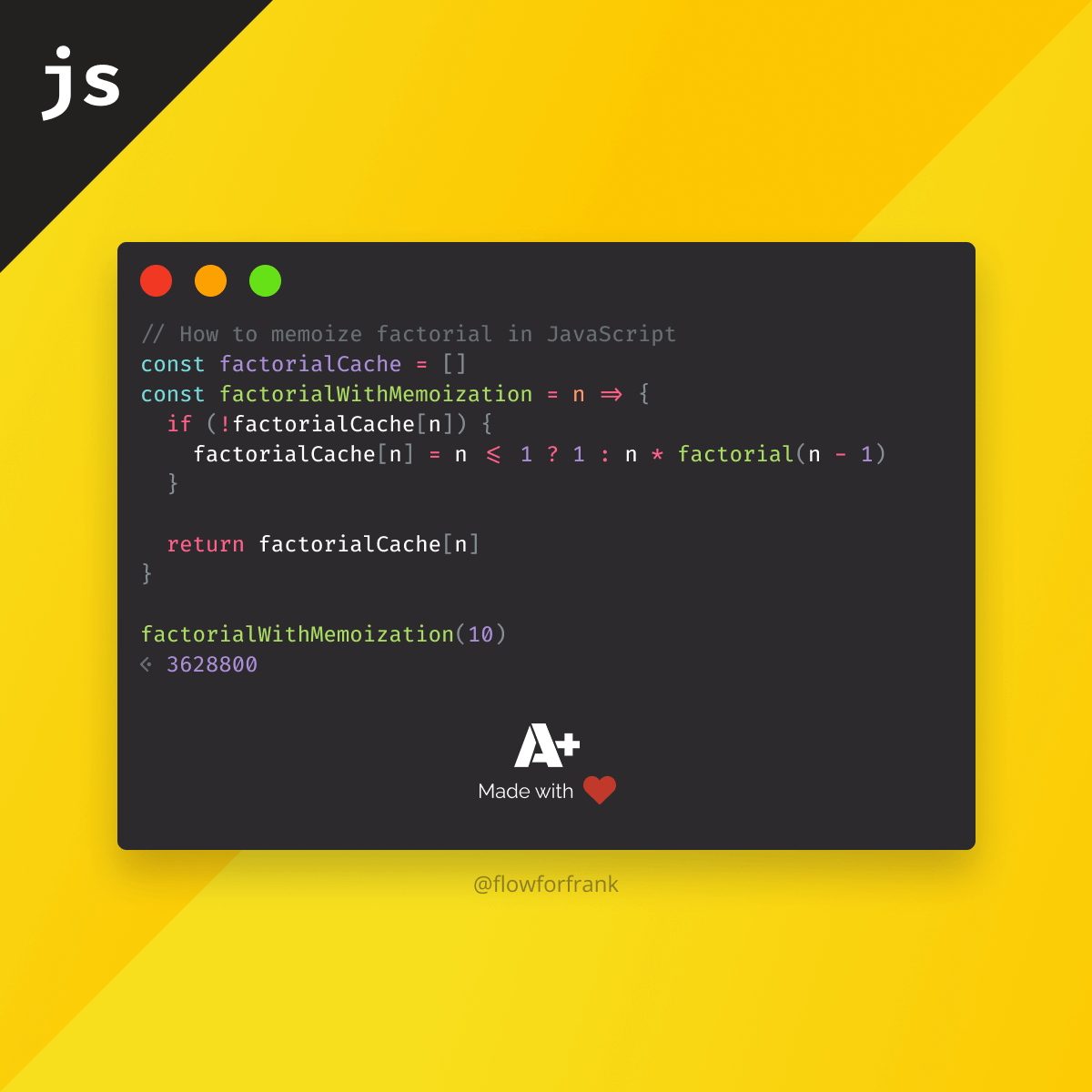
How to Do Factorial with Memoization in JavaScript
Factorial can be defined with a recursive function in JavaScript with a single line in the following way:
const factorial = n => n <= 1 ? 1 : n * factorial(n - 1)
// The above is equivalent to:
const factorial = (n) => {
if (n <= 1) {
return 1
}
return n * factorial(n - 1)
}
// Returns 3628800
factorial(10)
This means that if the passed number is less than or equal to 1, then the output will be 1, otherwise, it will be n * factorial(n-1)
. Based on the formula, we can define factorial as:
n! = n * (n - 1) * ... * 1
or
n! = n β factorial (n β 1)
To memoize a factorial function in JavaScript, we need to create an array to store previously calculated values inside it and return the value that already exists in the array (cache) rather than recalculating it.
const factorialCache = []
const factorialWithMemoization = n => {
if (!factorialCache[n]) {
factorialCache[n] = n <= 1 ? 1 : n * factorial(n - 1)
}
return factorialCache[n]
}
in case the value doesn't exist in the cache, we can create it at the given index, and return the cached value during the next call. This means that if we call the function with 10 again, the cache will be equal to the following:
(11) [empty Γ 10, 3628800]
To compare the performance difference between the two factorial functions, check out the benchmark created on jsben.ch.
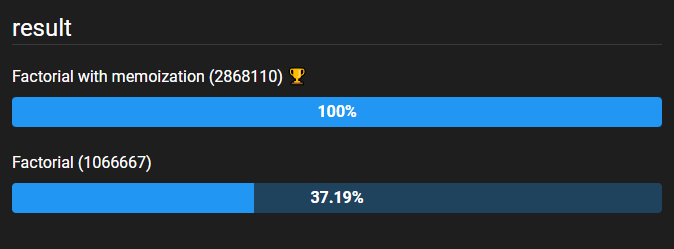
Make sure you never prematurely optimize code in JavaScript. Optimize when performance issues arise.
Want to learn more about how memoization works in JavaScript? Check out how you can create a memoize decorator in JavaScript in the following tutorial:
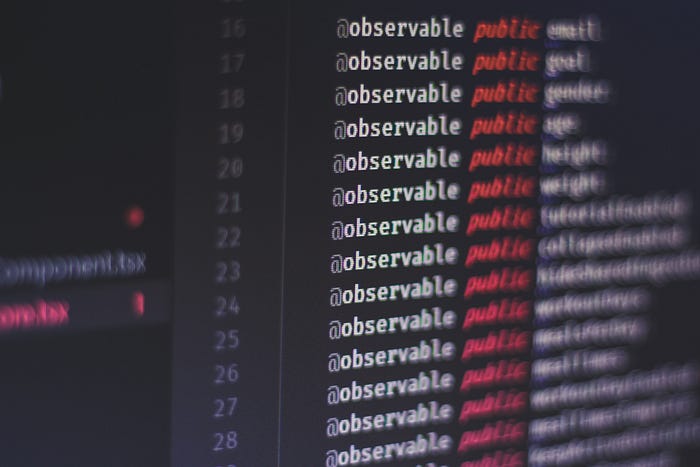
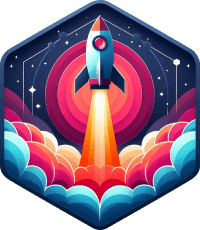
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: