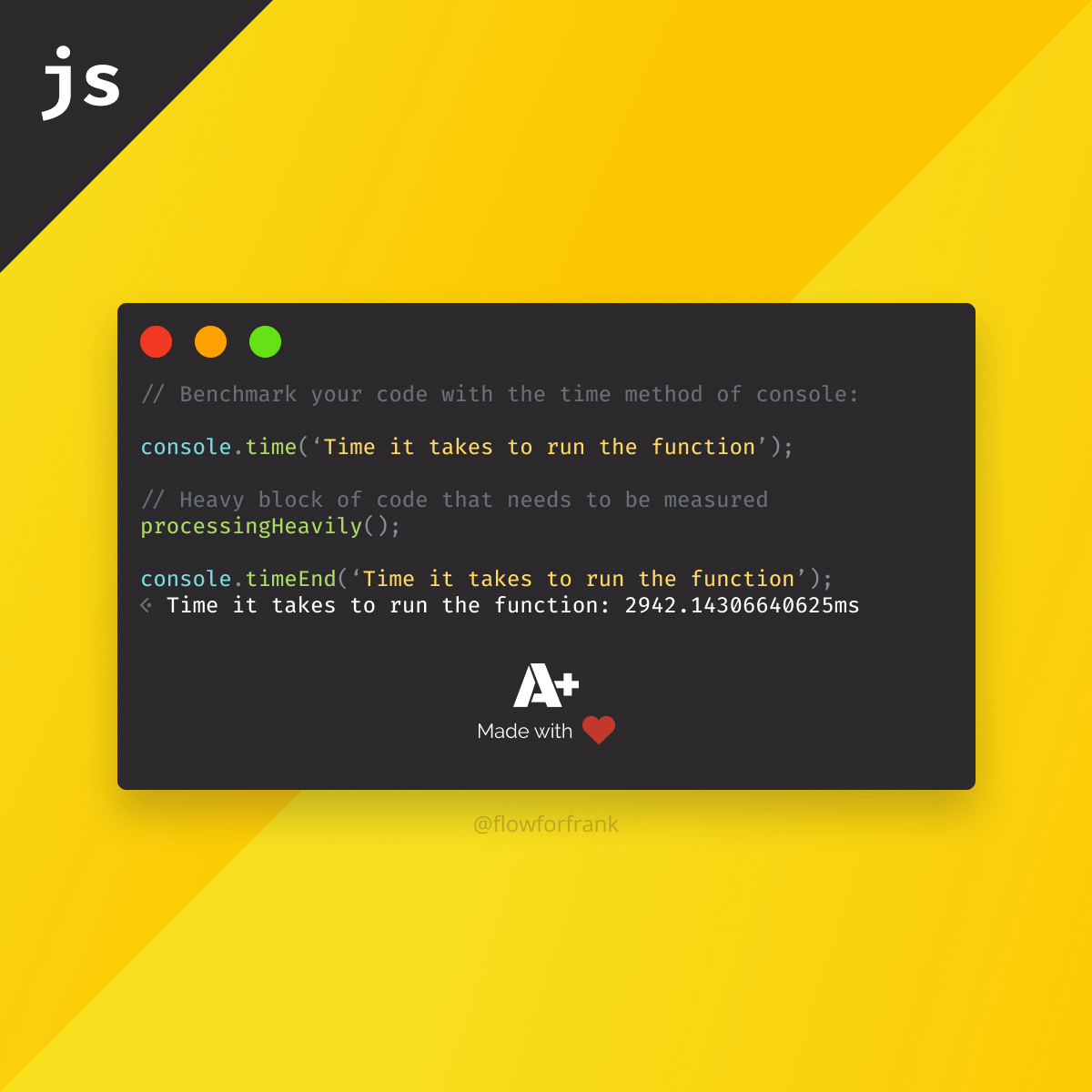
3 Ways to Benchmark Your JavaScript Code Without a Library
Good performance in JavaScript is a must if you want to ensure a great user experience on your site. Slow load and interaction times can drive users away, and users are less likely to return to a site that is known for being slow.
Measuring performance in JavaScript is a great way to spot potential bugs and bottlenecks, see where user experience can be improved by improving performance, or just simply compare implementations to evaluate which solution performs better.
In this tutorial, we are going to take a look at three different ways you can use to measure and benchmark the performance of your JavaScript code.
Benchmark using performance.now
First, we have the Performance API which exposes a performance.now
method that we can use to measure the execution time of a given code. This can be done by calling performance.now
twice:
const t0 = performance.now()
// block of code that needs to be measured
processingHeavily()
const t1 = performance.now()
console.log(`Time it takes to run the function: ${t1 - t0} ms`)
Once before the execution of the function that we want to measure, and once after the execution of the function (or any code for that matter). We need to save the result into variables, then subtracting the second variable from the first one we get the execution time in milliseconds.
Benchmark using performance.measure
The Performance API also provides two other methods that can be used in conjunction to benchmark your code. They are performance.mark
and performance.measure
. To convert the above example into using these two new methods, we can do the following:
performance.mark('start')
// block of code that needs to be measured
processingHeavily()
performance.mark('end')
performance.measure('Measurement', 'start', 'end')
First, we need to mark our code at a place where we want to start the measurement. We can give it a name by passing a string. I have named it "start". Then we need to make another mark at the end of the benchmark, I have called it "end". Then we can measure the performance between the two marks by calling performance.measure
with a name, and the starting and end mark. Make sure you pass the correct mark names as the second and third parameters. This will return a PerformanceMeasure
object:
{
detail: null
duration: 5.699999988079071
entryType: "measure"
name: "Measurement"
startTime: 132324.40000000596
}
We can also later grab it by its name and request the results again using the following line:
performance.getEntriesByName('Measurement')

Benchmark using console.time
The third option is to use the Console object, as it has a time
method that can be used to start a timer and track how long an operation takes. It looks very similar to the performance API. The previous examples would translate to the following:
console.time('Time it takes to run the function')
// block of code that needs to be measured
processingHeavily();
console.timeEnd('Time it takes to run the function')
Make sure you give the same name to both time
and timeEnd
so they are connected. This will generate the time it takes to run the operation between the two calls in a readable format:
Time it takes to run the function: 0.02099609375 ms
Benchmark with a custom function
Lastly, given the above methods, we can come up with a custom implementation to create a function specifically for measuring performance. Take a look at the following code example:
const benchmark = (func, params, iterations) => {
const t0 = performance.now()
for (let i = 0; i < iterations; i++) {
func(params)
}
const t1 = performance.now()
return `${t1 - t0}ms`
}
This function takes in another function as the first argument, its parameters as the second argument, and the number of iterations we want to run the function for. It will return the number of milliseconds it took for the function to run for x iterations. We can then later call this benchmark function in the following way:
// Run the callback function with no parameters for 10,000 iterations
benchmark(() => { ... }, null, 10_000)
// Returns
-> '5.200000017881393ms'
Things to keep in mind when measuring performance
There are a couple of things to keep in mind when it comes to measuring performance in JavaScript:
- Your performance results will vary based on device and browser. Each device is different, just like each browser. While a function may perform well in Chrome, it could perform slower in Safari, and the same is true the other way. This is because of different hardware and different JavaScript engines.
- The measurements will not be 100% precise. There is an intentionally reduced time precision when it comes to measuring performance due to protection against timing and fingerprinting attacks. This, however, should not stop you from making meaningful conclusions.
- Results are usually much slower for average users. Your development device is likely much faster then the device of an average user, whether it be mobile or desktop. This means you may get a better picture by enabling CPU throttle in your DevTools.
- Measure multiple times with multiple inputs. If the function you are measuring can have parameters, make sure you test it with different parameters, not just with one deterministic value. In a real scenario, inputs can and will change. Likewise, you want to execute benchmarks multiple times, and draw a conclusion for a resultset, instead of jumping to conclusions from one iteration. One iteration may perform better than the other, so rather look at averages.
- Focus on relative improvements. Performance is impacted by many things. That's why it is important to focus on relative improvements. You will not only experience different results based on different hardware and browser, the current load of your CPU can also affect the end results.
Do you have any tips on performance for JavaScript that are not mentioned here? Share it with us in the comments below! If you would like to learn more about performance, make sure you check out the tutorial below. Thank you for reading through, happy benchmarking!
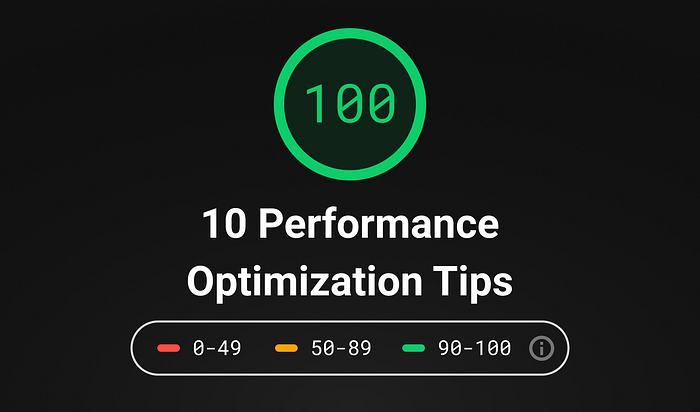
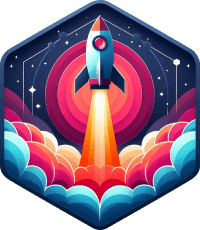
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: