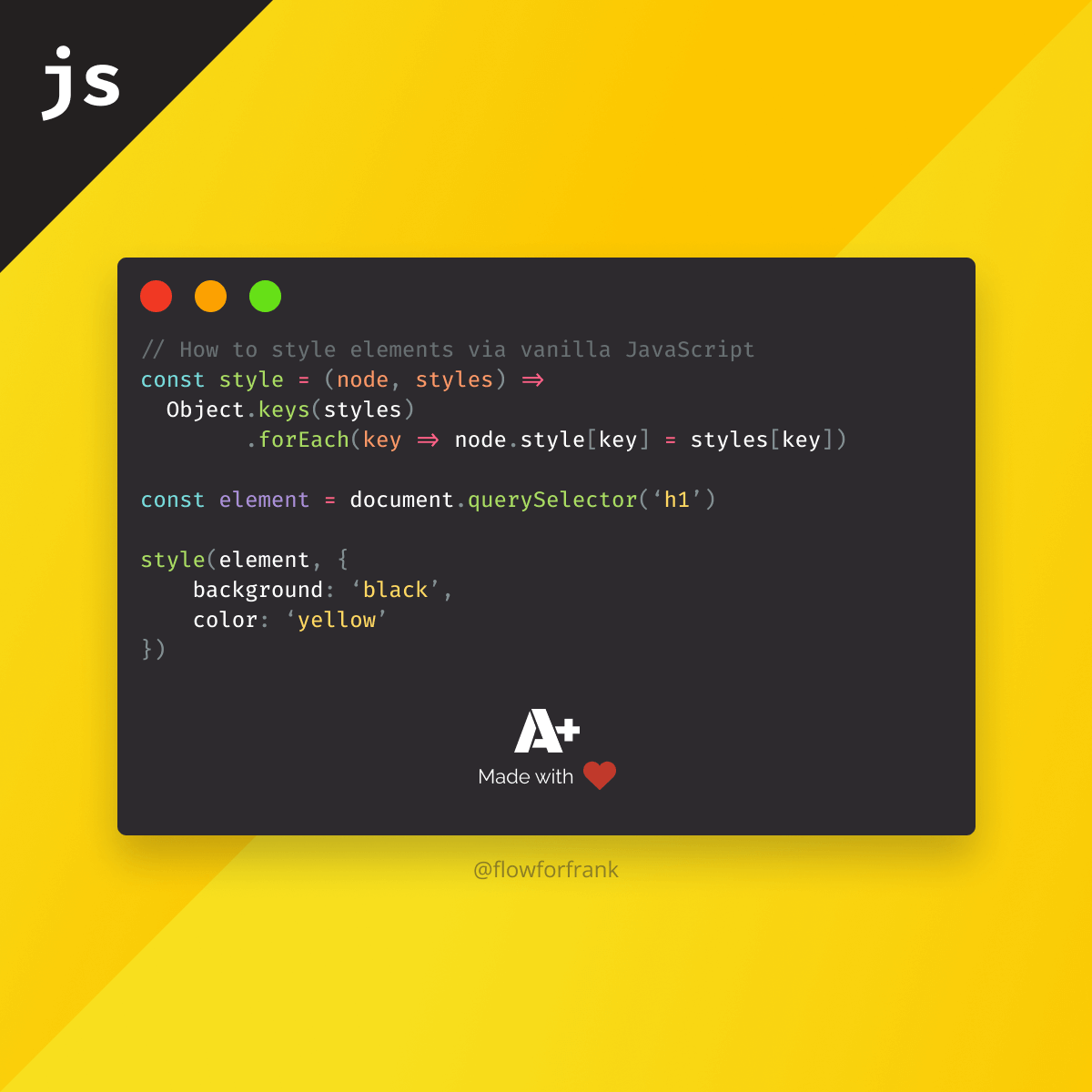
How to Add Styles to Element in Vanilla JavaScript
To add styles to an element in vanilla JavaScript, you can use the following helper function which expects a DOM element and a list of styles as an object:
const style = (node, styles) => Object.keys(styles).forEach(key => node.style[key] = styles[key])
// Use it in the following way:
const element = document.querySelector('h1')
style(element, {
background: 'black',
color: 'yellow'
})
This works by looping through the keys of the styles
object which it expects as a second parameter. In this case: background
and color
. Then, for each key, it sets the appropriate style property of the passed DOM element. This means you have to name the keys as valid CSS properties. Essentially, in the loop, this will translate to the following:
element.style.background = 'black'
element.style.color = 'yellow'
Manually set the style object of the element
This takes us to the second example, where you could also directly do the above and set the styles of an element by using assignments:
const element = document.querySelector('h1')
element.style.background = 'black'
element.style.color = 'yellow'
To find out what CSS properties are available on the element you are working with, simply grab an element and log its style
object to the console. This will give back a CSSStyleDeclaration
object:
<- element.style
// Gives back
-> CSSStyleDeclaration {
accentColor: ""
additiveSymbols: ""
alignContent: ""
...
}
Notice that styles that otherwise use dashes in CSS are converted into camelCase in JavaScript. For example, instead of "align-content", you can reference alignContent
.
Using the cssText property
There is also a cssText
property on the style
object. This contains all the styles represented as a single string. Keeping with the above example, our element's cssText
would be the following:
'background: black; color: yellow;'
// Or you can overwrite it in the following way:
const element = document.querySelector('h1')
element.style.cssText = 'background: black; color: yellow;'
Make sure you pass a string to this property. All CSS properties must be separated by a semi-colon. You can also combine this approach with a more declarative function that generates the necessary styles out of JavaScript objects:
const book = {
content: 'π',
display: 'block',
padding: '20px',
background: 'yellow'
};
const getStyles = obj => Object.keys(obj).map(key => `${key}:${obj[key]}`).join(';');
// Later you can call the function to inline the styles
getStyles(book);
// Or assign it directly to your cssText
element.style.cssText = getStyles(book)
This function works by (similarly to the previous helper function):
- First looping through the keys of the object with
Object.keys
- For each key, it returns with a new string (the key and value combined), by calling
map
- Lastly, it joins everything together with a semicolon, using
join
If you have multiple styles to take care of, you can also take this one step further and use the below assignment to generate them in one go:
const styles = {
heroCTA: {
display: 'block'
},
heroImage: {
display: 'block',
width: '100%'
}
};
const getStyles = obj => Object.keys(obj).map(key => `${key}:${obj[key]}`).join(';');
const generatedStyles = Object.fromEntries(
Object.entries(styles)
.map(([element, style]) => [element, getStyles(style)])
);
// This will generate the following object:
{
heroCTA: 'display:block',
heroImage: 'display:block;width:100%'
}

Using a style tag
Lastly, you can also dynamically create a style tag and append it to the body to add global styles to your app via JavaScript. To do this, you can use the following code snippet:
const style = document.createElement('style')
style.innerHTML = `
h1 {
background: 'black';
color: 'yellow';
}
`
document.head.appendChild(style)
You can use a template literal to break your CSS into multiple lines for improved readability. Don't forget to actually append the element to your head after you created the CSS.
To add styles to elements in JavaScript, you can use:
element.style[property]
, eg.:element.style.color
element.style.cssText
, eg.:'color: yellow;'
- A dynamically created style tag for global styles
- A helper function for looping through object of styles
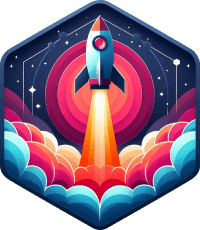
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: