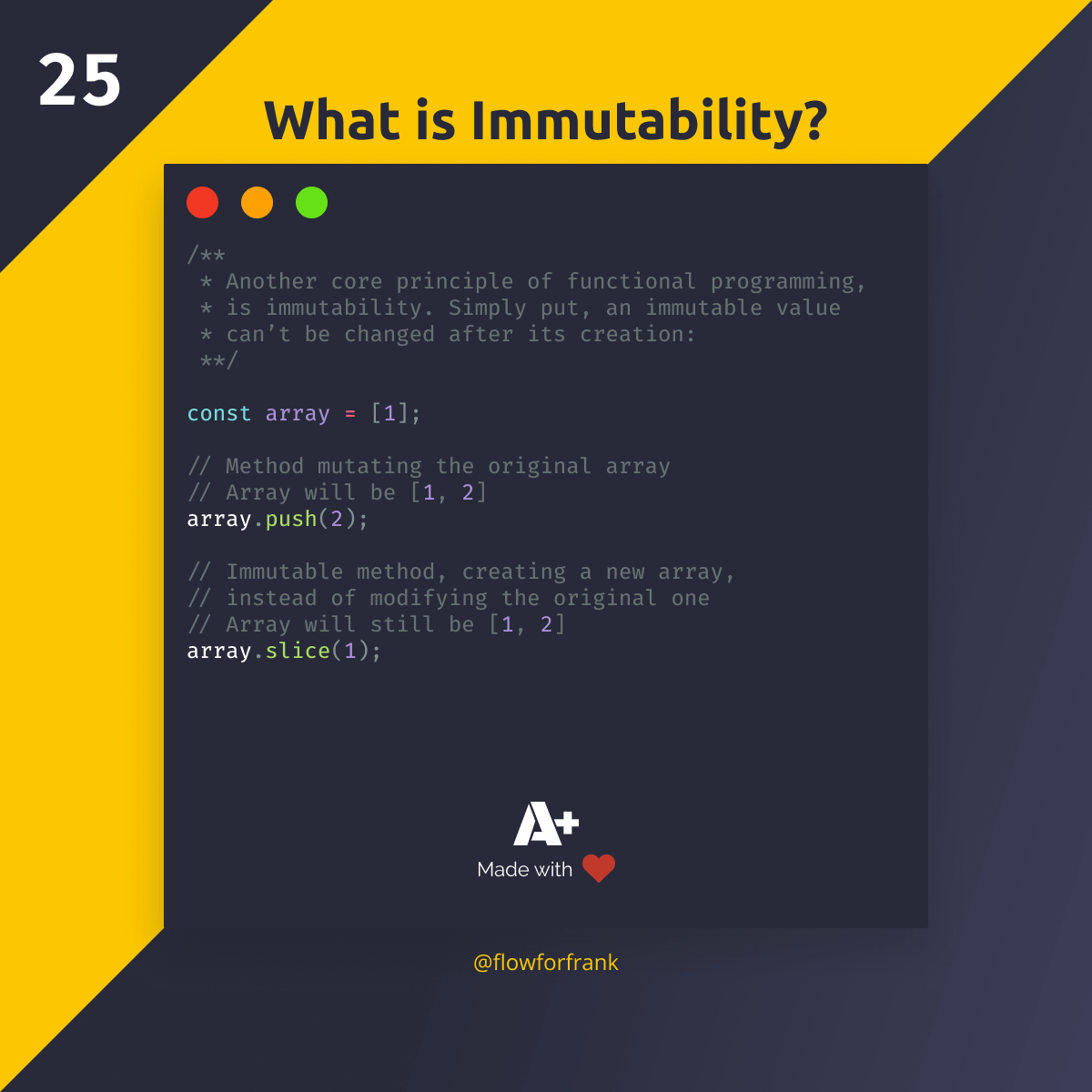
What is Immutability?
Another core principle of functional programming is immutability. When we talk about immutable variables and objects, we simply mean that once declared, their value canβt be changed. This can reduce the complexity of your code and make your implementation less prone to errors.
To demonstrate immutability through an example, letβs say you have an array where you need to remove the first item. Take a look at the differences below:
const presents = ['π', 'π¦', 'π', 'π', 'π'];
// --- Mutable solution ---
// we get back π
// and presents will be equal to ['π¦', 'π', 'π', 'π'];
presents.shift();
// --- Immutable solution ---
// newPresents will be equal to π¦ π π π
// and presents will be still equal to ['π', 'π¦', 'π', 'π', 'π'];
const newPresents = presents.slice(1);
In the first example, we modify the original array with the shift
function. If we want to achieve the same but keep the original array intact, we can use slice instead. This way you can avoid having unforeseen bugs in your application where you unintentionally modify data that should be kept in pristine condition.
One downside of immutability is performance. If you create too many copies you will run into memory issues so in case you operate on a large data set, you need to think about performance.
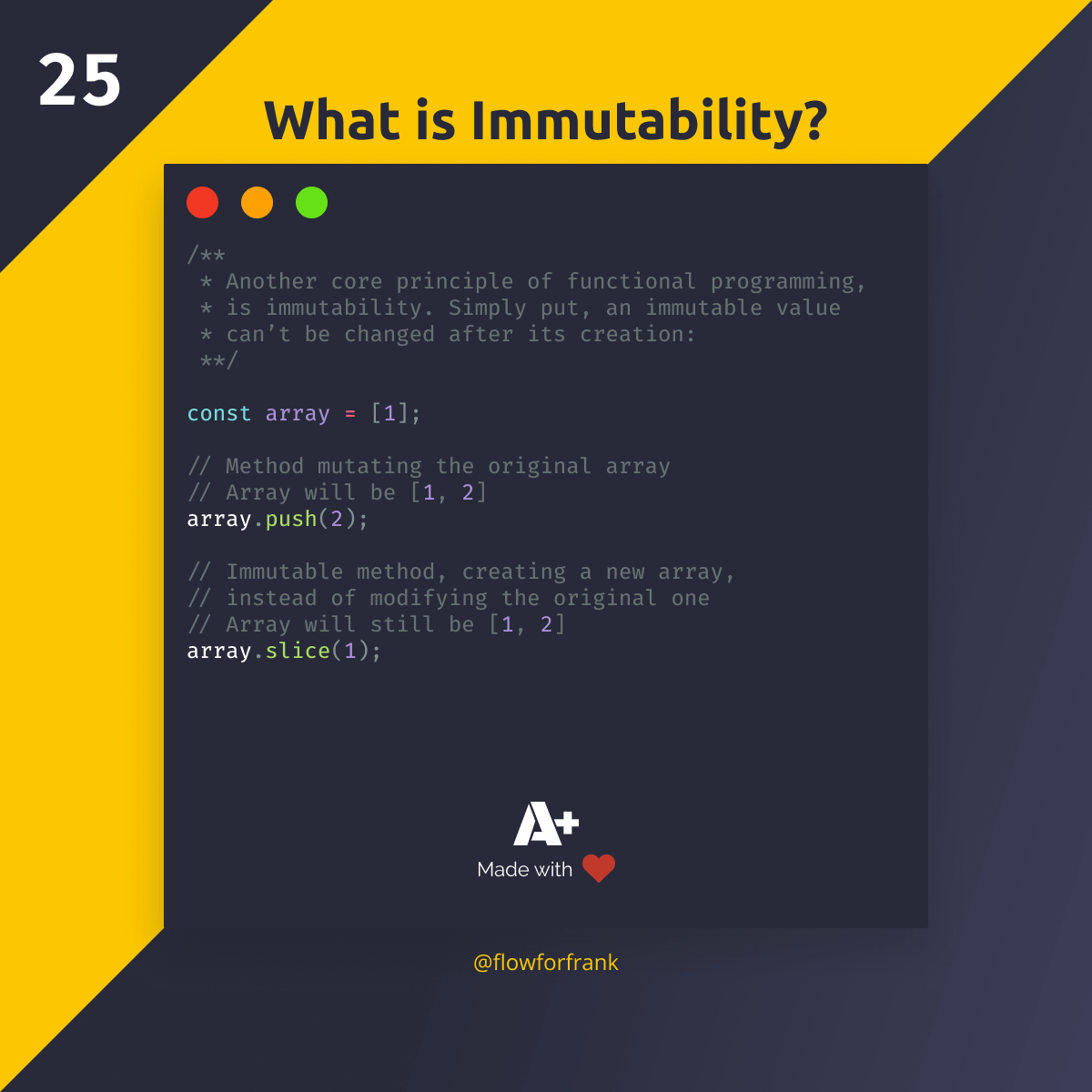
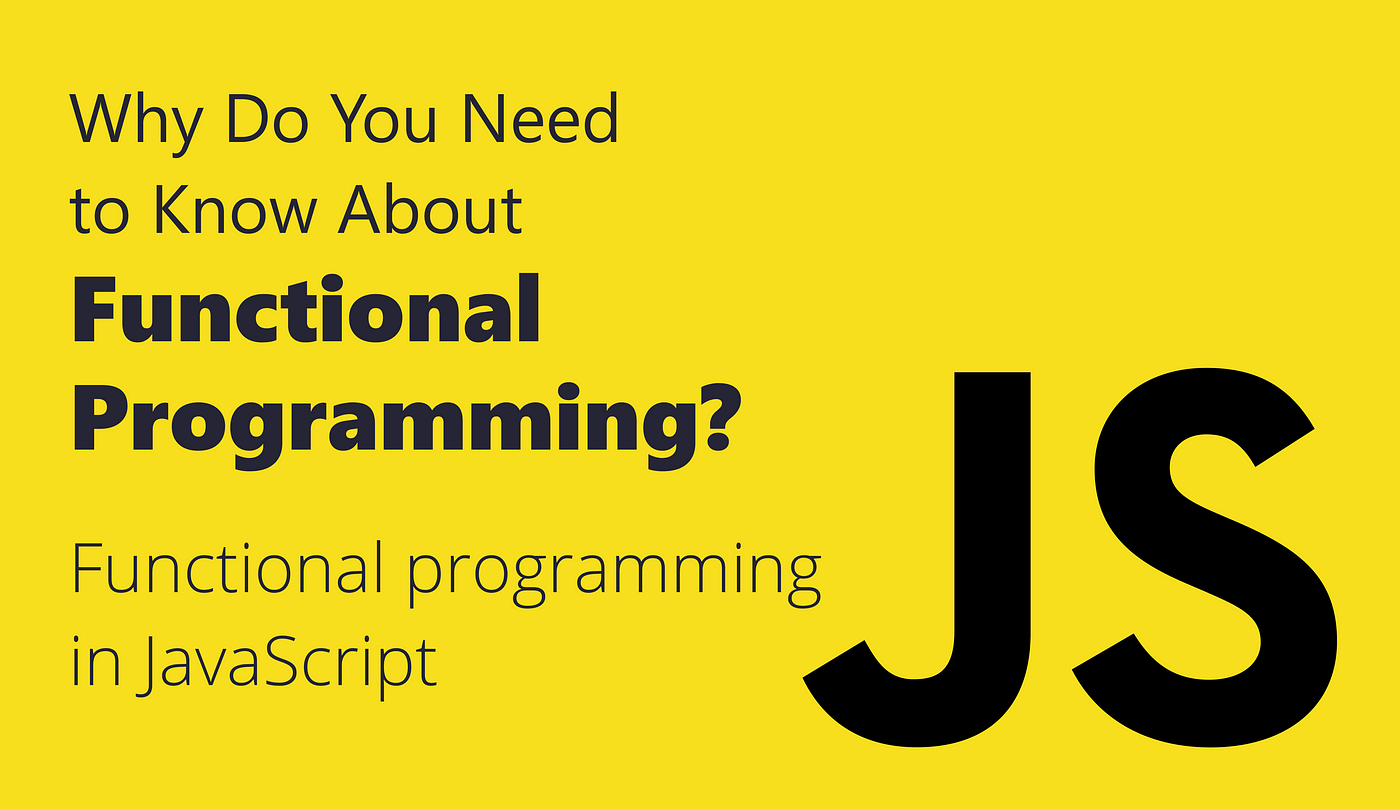
Resources:
Access 100+ interactive lessons
Unlimited access to hundreds of tutorials
Prepare for technical interviews