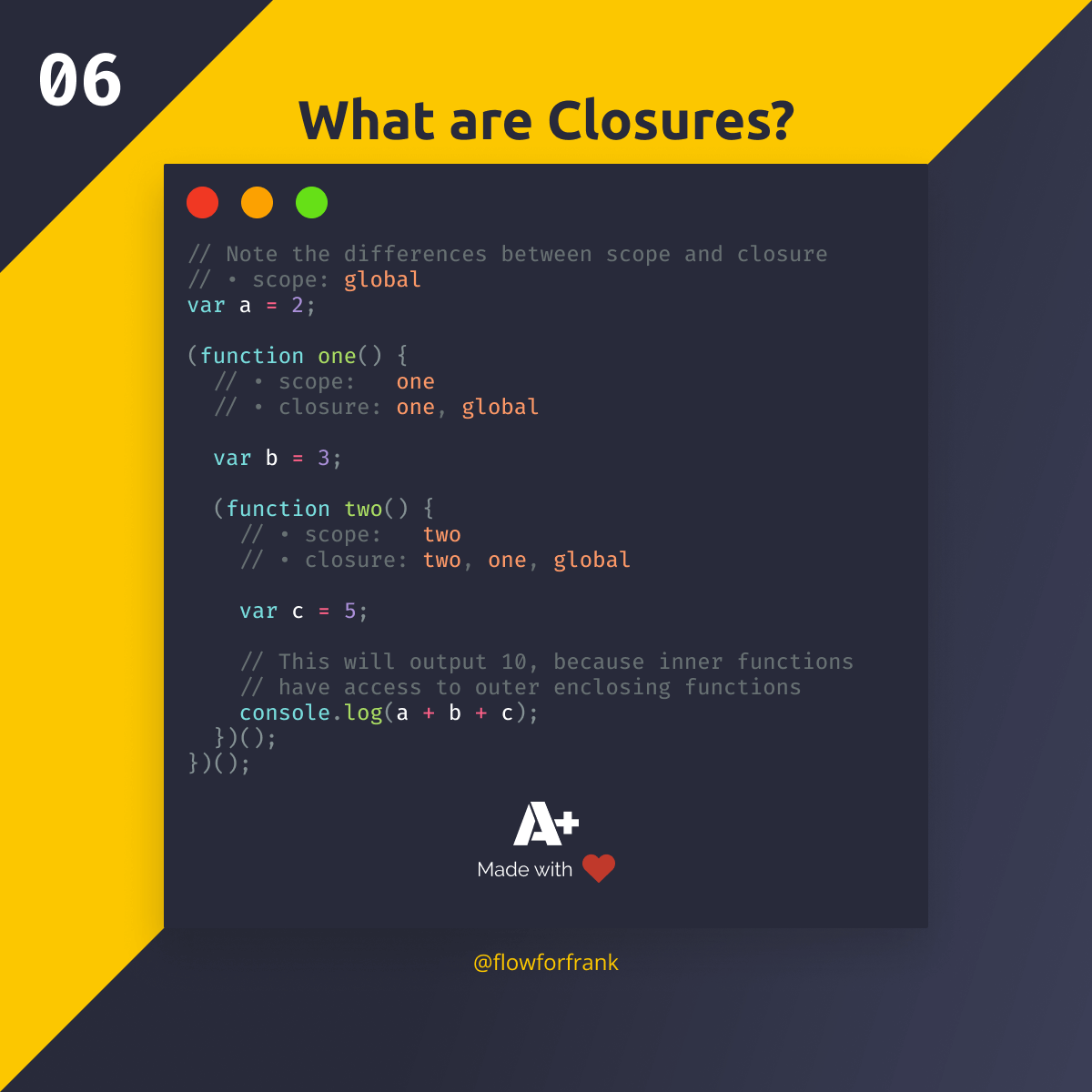
What are Closures in JavaScript?
Closure often confuse people in JavaScript. In one sentence: It is an inner function that has access to the other function's variables. Itβs a combination of a function and the lexical environment in which it was declared.
A great example of closures are callbacks. Note the differences between scopes and closures in the following example:
Copied to clipboard! Playground
// Note the differences between scope and closure
// scope: global
var a = 1;
(function one() {
// scope: one
// closure: one, global
var b = 2;
(function two() {
// scope: two
// closure: two, one, global
var c = 3;
(function three() {
// scope: three
// closure: three, two, one, global
var d = 4;
// This will output 10 as we have access to outer enclosing function variables
console.log(a + b + c + d);
})();
})();
})();
- The scope refers to the lexical environment of the function
- While closure encompasses the lexical scope of other enclosing function as well
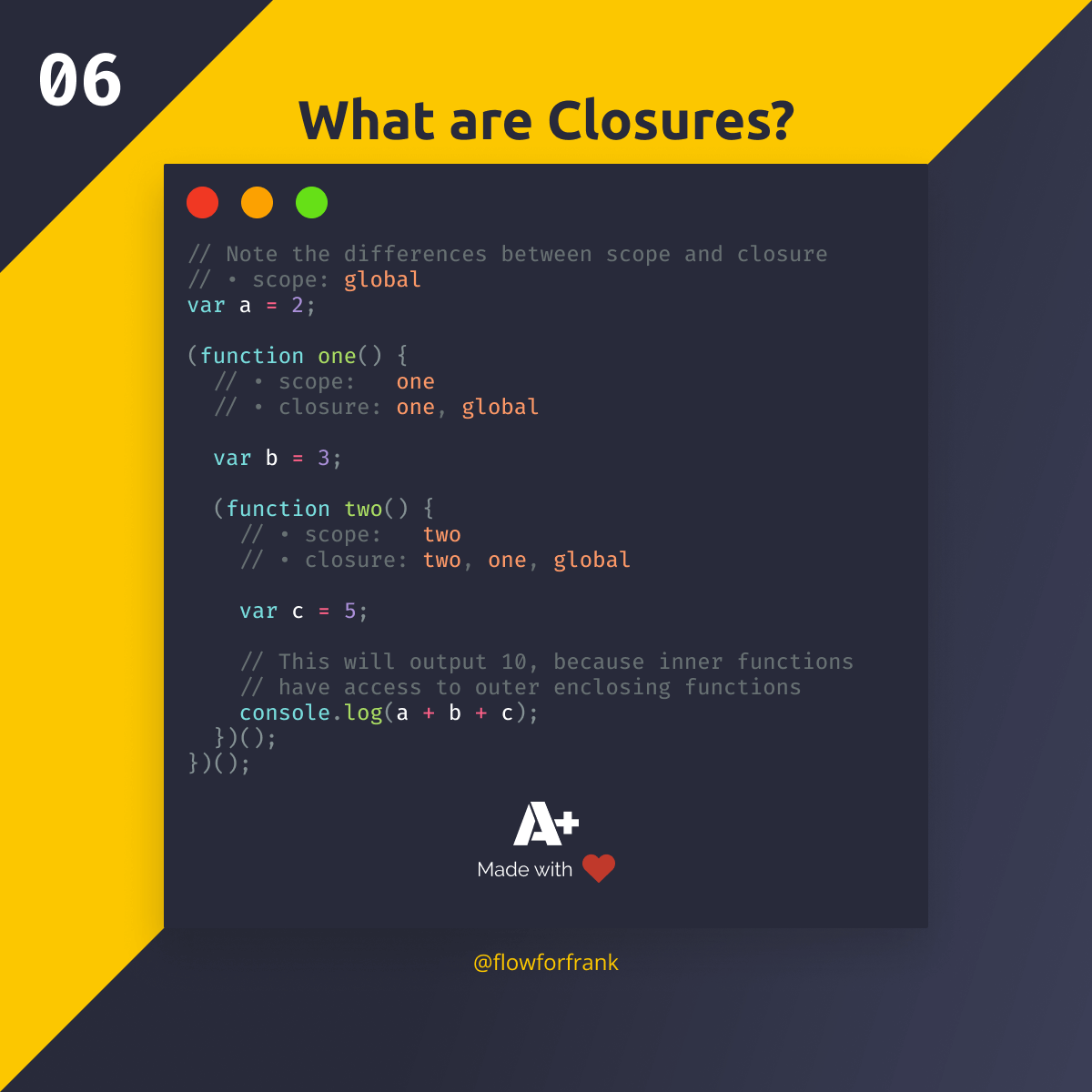
Resources:
π More Webtips
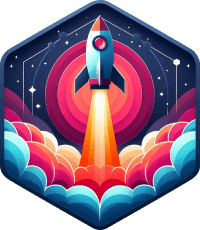
Rocket Launch Your Career
Speed up your learning progress with our mentorship program. Join as a mentee to unlock the full potential of Webtips and get a personalized learning experience by experts to master the following frontend technologies: